Show More
@@ -0,0 +1,137 b'' | |||||
|
1 | from converters.markdown import ConverterMarkdown | |||
|
2 | import io | |||
|
3 | import os | |||
|
4 | ||||
|
5 | class ConverterSlider(ConverterMarkdown): | |||
|
6 | """Convert a notebook to html suitable for easy pasting into Blogger. | |||
|
7 | ||||
|
8 | It generates an html file that has *only* the pure HTML contents, and a | |||
|
9 | separate file with `_header` appended to the name with all header contents. | |||
|
10 | Typically, the header file only needs to be used once when setting up a | |||
|
11 | blog, as the CSS for all posts is stored in a single location in Blogger. | |||
|
12 | """ | |||
|
13 | ||||
|
14 | def __init__(self, infile, highlight_source=False, show_prompts=True, | |||
|
15 | inline_prompt=True): | |||
|
16 | super(ConverterMarkdown, self).__init__(infile) | |||
|
17 | self.highlight_source = highlight_source | |||
|
18 | self.show_prompts = show_prompts | |||
|
19 | self.inline_prompt = inline_prompt | |||
|
20 | ||||
|
21 | def convert(self, cell_separator='\n'): | |||
|
22 | """ | |||
|
23 | Generic method to converts notebook to a string representation. | |||
|
24 | ||||
|
25 | This is accomplished by dispatching on the cell_type, so subclasses of | |||
|
26 | Convereter class do not need to re-implement this method, but just | |||
|
27 | need implementation for the methods that will be dispatched. | |||
|
28 | ||||
|
29 | Parameters | |||
|
30 | ---------- | |||
|
31 | cell_separator : string | |||
|
32 | Character or string to join cells with. Default is "\n" | |||
|
33 | ||||
|
34 | Returns | |||
|
35 | ------- | |||
|
36 | out : string | |||
|
37 | """ | |||
|
38 | lines = [] | |||
|
39 | lines.extend(self.optional_header()) | |||
|
40 | top = '<section data-markdown><script type="text/template">' | |||
|
41 | bottom = '</script></section>' | |||
|
42 | text = self.main_body(cell_separator) | |||
|
43 | for i,j in enumerate(text): | |||
|
44 | if j == u'---': | |||
|
45 | text[i] = bottom + top | |||
|
46 | lines.extend(text) | |||
|
47 | lines.extend(self.optional_footer()) | |||
|
48 | return u'\n'.join(lines) | |||
|
49 | ||||
|
50 | def save(self, outfile=None, encoding=None): | |||
|
51 | "read and parse notebook into self.nb" | |||
|
52 | if outfile is None: | |||
|
53 | outfile = self.outbase + '_slides.' + 'html' | |||
|
54 | if encoding is None: | |||
|
55 | encoding = self.default_encoding | |||
|
56 | with io.open(outfile, 'w', encoding=encoding) as f: | |||
|
57 | f.write(self.output) | |||
|
58 | return os.path.abspath(outfile) | |||
|
59 | ||||
|
60 | def optional_header(self): | |||
|
61 | optional_header_body = [\ | |||
|
62 | ''' | |||
|
63 | <!DOCTYPE html> | |||
|
64 | <html> | |||
|
65 | <head> | |||
|
66 | <meta charset="utf-8" /> | |||
|
67 | <meta http-equiv="X-UA-Compatible" content="chrome=1"> | |||
|
68 | ||||
|
69 | <meta name="apple-mobile-web-app-capable" content="yes" /> | |||
|
70 | <meta name="apple-mobile-web-app-status-bar-style" content="black-translucent" /> | |||
|
71 | ||||
|
72 | <link rel="stylesheet" href="reveal/css/reveal.css"> | |||
|
73 | <link rel="stylesheet" href="reveal/css/theme/default.css" id="theme"> | |||
|
74 | ||||
|
75 | <!-- For syntax highlighting --> | |||
|
76 | <link rel="stylesheet" href="reveal/lib/css/zenburn.css"> | |||
|
77 | ||||
|
78 | <!-- If the query includes 'print-pdf', use the PDF print sheet --> | |||
|
79 | <script> | |||
|
80 | document.write( '<link rel="stylesheet" href="reveal/css/print/' + ( window.location.search.match( /print-pdf/gi ) ? 'pdf' : 'paper' ) + '.css" type="text/css" media="print">' ); | |||
|
81 | </script> | |||
|
82 | ||||
|
83 | <!--[if lt IE 9]> | |||
|
84 | <script src="reveal/lib/js/html5shiv.js"></script> | |||
|
85 | <![endif]--> | |||
|
86 | ||||
|
87 | </head> | |||
|
88 | <body><div class="reveal"><div class="slides"> | |||
|
89 | <section data-markdown><script type="text/template"> | |||
|
90 | '''] | |||
|
91 | return optional_header_body | |||
|
92 | ||||
|
93 | def optional_footer(self): | |||
|
94 | optional_footer_body = [\ | |||
|
95 | ''' | |||
|
96 | </script></section> | |||
|
97 | </div></div> | |||
|
98 | ||||
|
99 | <script src="reveal/lib/js/head.min.js"></script> | |||
|
100 | ||||
|
101 | <script src="reveal/js/reveal.min.js"></script> | |||
|
102 | ||||
|
103 | <script> | |||
|
104 | ||||
|
105 | // Full list of configuration options available here: https://github.com/hakimel/reveal.js#configuration | |||
|
106 | Reveal.initialize({ | |||
|
107 | controls: true, | |||
|
108 | progress: true, | |||
|
109 | history: true, | |||
|
110 | ||||
|
111 | theme: Reveal.getQueryHash().theme, // available themes are in /css/theme | |||
|
112 | transition: Reveal.getQueryHash().transition || 'page', // default/cube/page/concave/zoom/linear/none | |||
|
113 | ||||
|
114 | // Optional libraries used to extend on reveal.js | |||
|
115 | dependencies: [ | |||
|
116 | { src: 'reveal/lib/js/classList.js', condition: function() { return !document.body.classList; } }, | |||
|
117 | { src: 'reveal/plugin/markdown/showdown.js', condition: function() { return !!document.querySelector( '[data-markdown]' ); } }, | |||
|
118 | { src: 'reveal/plugin/markdown/markdown.js', condition: function() { return !!document.querySelector( '[data-markdown]' ); } }, | |||
|
119 | { src: 'reveal/plugin/highlight/highlight.js', async: true, callback: function() { hljs.initHighlightingOnLoad(); } }, | |||
|
120 | { src: 'reveal/plugin/zoom-js/zoom.js', async: true, condition: function() { return !!document.body.classList; } }, | |||
|
121 | { src: 'reveal/plugin/notes/notes.js', async: true, condition: function() { return !!document.body.classList; } }, | |||
|
122 | { src: 'https://c328740.ssl.cf1.rackcdn.com/mathjax/latest/MathJax.js?config=TeX-AMS_HTML', async: true }, | |||
|
123 | { src: 'js/initmathjax.js', async: true} | |||
|
124 | ] | |||
|
125 | }); | |||
|
126 | </script> | |||
|
127 | ||||
|
128 | <script> | |||
|
129 | Reveal.addEventListener( 'slidechanged', function( event ) { | |||
|
130 | MathJax.Hub.Rerender(); | |||
|
131 | }); | |||
|
132 | </script> | |||
|
133 | ||||
|
134 | </body> | |||
|
135 | </html> | |||
|
136 | '''] | |||
|
137 | return optional_footer_body |
@@ -0,0 +1,468 b'' | |||||
|
1 | { | |||
|
2 | "metadata": { | |||
|
3 | "name": "test_example_slide" | |||
|
4 | }, | |||
|
5 | "nbformat": 3, | |||
|
6 | "nbformat_minor": 0, | |||
|
7 | "worksheets": [ | |||
|
8 | { | |||
|
9 | "cells": [ | |||
|
10 | { | |||
|
11 | "cell_type": "heading", | |||
|
12 | "level": 1, | |||
|
13 | "metadata": {}, | |||
|
14 | "source": [ | |||
|
15 | "Notebook Slide Example" | |||
|
16 | ] | |||
|
17 | }, | |||
|
18 | { | |||
|
19 | "cell_type": "markdown", | |||
|
20 | "metadata": {}, | |||
|
21 | "source": [ | |||
|
22 | "Rendered by vIPer using [Reveal.js](http://lab.hakim.se/reveal-js)!" | |||
|
23 | ] | |||
|
24 | }, | |||
|
25 | { | |||
|
26 | "cell_type": "markdown", | |||
|
27 | "metadata": {}, | |||
|
28 | "source": [ | |||
|
29 | "(also you can get a [pdf](http://www.slideviper.oquanta.info/reveal/slides.pdf) version with chrome!)" | |||
|
30 | ] | |||
|
31 | }, | |||
|
32 | { | |||
|
33 | "cell_type": "markdown", | |||
|
34 | "metadata": {}, | |||
|
35 | "source": [ | |||
|
36 | "---" | |||
|
37 | ] | |||
|
38 | }, | |||
|
39 | { | |||
|
40 | "cell_type": "heading", | |||
|
41 | "level": 2, | |||
|
42 | "metadata": {}, | |||
|
43 | "source": [ | |||
|
44 | "NOTE: This notebook was modified from a Fernando's one" | |||
|
45 | ] | |||
|
46 | }, | |||
|
47 | { | |||
|
48 | "cell_type": "markdown", | |||
|
49 | "metadata": {}, | |||
|
50 | "source": [ | |||
|
51 | "Since the notebook was introduced with [IPython 0.12](http://ipython.org/ipython-doc/rel-0.12.1/whatsnew/version0.12.html), it has proved to be very popular, and we are seeing great adoption of the tool and the underlying file format in research and education. One persistent question we've had since the beginning (even prior to its official release) was whether it would be possible to easily write blog posts using the notebook." | |||
|
52 | ] | |||
|
53 | }, | |||
|
54 | { | |||
|
55 | "cell_type": "markdown", | |||
|
56 | "metadata": {}, | |||
|
57 | "source": [ | |||
|
58 | "---" | |||
|
59 | ] | |||
|
60 | }, | |||
|
61 | { | |||
|
62 | "cell_type": "markdown", | |||
|
63 | "metadata": {}, | |||
|
64 | "source": [ | |||
|
65 | "The combination of easy editing in markdown with the notebook's ability to contain code, figures and results, makes it an ideal platform for quick authoring of technical documents, so being able to post to a blog is a natural request." | |||
|
66 | ] | |||
|
67 | }, | |||
|
68 | { | |||
|
69 | "cell_type": "markdown", | |||
|
70 | "metadata": {}, | |||
|
71 | "source": [ | |||
|
72 | "---" | |||
|
73 | ] | |||
|
74 | }, | |||
|
75 | { | |||
|
76 | "cell_type": "markdown", | |||
|
77 | "metadata": {}, | |||
|
78 | "source": [ | |||
|
79 | "Today, in answering a query about this from a colleague, I decided to try again the status of our conversion pipeline, and I'm happy to report that with a bit of elbow-grease, at least on Blogger things work pretty well! \n", | |||
|
80 | "\n", | |||
|
81 | "This post was *entirely* written as a notebook, and in fact I have now created a [github repo](https://github.com/fperez/blog), which means that you can see it directly [rendered in IPyhton's nbviewer app](http://nbviewer.ipython.org/urls/raw.github.com/fperez/blog/master/120907-Blogging with the IPython Notebook.ipynb)." | |||
|
82 | ] | |||
|
83 | }, | |||
|
84 | { | |||
|
85 | "cell_type": "markdown", | |||
|
86 | "metadata": {}, | |||
|
87 | "source": [ | |||
|
88 | "---" | |||
|
89 | ] | |||
|
90 | }, | |||
|
91 | { | |||
|
92 | "cell_type": "heading", | |||
|
93 | "level": 2, | |||
|
94 | "metadata": {}, | |||
|
95 | "source": [ | |||
|
96 | "Converting your notebook to html with nbconvert" | |||
|
97 | ] | |||
|
98 | }, | |||
|
99 | { | |||
|
100 | "cell_type": "markdown", | |||
|
101 | "metadata": {}, | |||
|
102 | "source": [ | |||
|
103 | "The first thing you will need is our [nbconvert](https://github.com/ipython/nbconvert) tool that converts notebooks across formats. The README file in the repo contains the requirements for nbconvert (basically [python-markdown](http://pypi.python.org/pypi/Markdown/), [pandoc](http://johnmacfarlane.net/pandoc), [docutils from SVN](http://docutils.svn.sourceforge.net/viewvc/docutils/trunk/docutils/?view=tar) and [pygments](http://pygments.org)).\n", | |||
|
104 | "\n", | |||
|
105 | "Once you have nbconvert installed, you can convert your notebook to Blogger-friendly html with:\n", | |||
|
106 | "\n", | |||
|
107 | " nbconvert -f blogger-html your_notebook.ipynb" | |||
|
108 | ] | |||
|
109 | }, | |||
|
110 | { | |||
|
111 | "cell_type": "markdown", | |||
|
112 | "metadata": {}, | |||
|
113 | "source": [ | |||
|
114 | "---" | |||
|
115 | ] | |||
|
116 | }, | |||
|
117 | { | |||
|
118 | "cell_type": "markdown", | |||
|
119 | "metadata": {}, | |||
|
120 | "source": [ | |||
|
121 | "This will leave two files in your computer, one named `your_notebook.html` and one named `your_noteboook_header.html`; it might also create a directory called `your_notebook_files` if needed for ancillary files. The first file will contain the body of your post and can be pasted wholesale into the Blogger editing area. The second file contains the CSS and Javascript material needed for the notebook to display correctly, you should only need to use this once to configure your blogger setup (see next):" | |||
|
122 | ] | |||
|
123 | }, | |||
|
124 | { | |||
|
125 | "cell_type": "markdown", | |||
|
126 | "metadata": {}, | |||
|
127 | "source": [ | |||
|
128 | "---" | |||
|
129 | ] | |||
|
130 | }, | |||
|
131 | { | |||
|
132 | "cell_type": "markdown", | |||
|
133 | "metadata": {}, | |||
|
134 | "source": [ | |||
|
135 | " # Only one notebook so far\n", | |||
|
136 | " (master)longs[blog]> ls\n", | |||
|
137 | " 120907-Blogging with the IPython Notebook.ipynb fig/ old/\n", | |||
|
138 | "\n", | |||
|
139 | " # Now run the conversion:\n", | |||
|
140 | " (master)longs[blog]> nbconvert.py -f blogger-html 120907-Blogging\\ with\\ the\\ IPython\\ Notebook.ipynb\n", | |||
|
141 | " \n", | |||
|
142 | " # This creates the header and html body files\n", | |||
|
143 | " (master)longs[blog]> ls\n", | |||
|
144 | " 120907-Blogging with the IPython Notebook_header.html fig/\n", | |||
|
145 | " 120907-Blogging with the IPython Notebook.html old/\n", | |||
|
146 | " 120907-Blogging with the IPython Notebook.ipynb" | |||
|
147 | ] | |||
|
148 | }, | |||
|
149 | { | |||
|
150 | "cell_type": "markdown", | |||
|
151 | "metadata": {}, | |||
|
152 | "source": [ | |||
|
153 | "---" | |||
|
154 | ] | |||
|
155 | }, | |||
|
156 | { | |||
|
157 | "cell_type": "heading", | |||
|
158 | "level": 2, | |||
|
159 | "metadata": {}, | |||
|
160 | "source": [ | |||
|
161 | "Configuring your Blogger blog to accept notebooks" | |||
|
162 | ] | |||
|
163 | }, | |||
|
164 | { | |||
|
165 | "cell_type": "markdown", | |||
|
166 | "metadata": {}, | |||
|
167 | "source": [ | |||
|
168 | "The notebook uses a lot of custom CSS for formatting input and output, as well as Javascript from [MathJax](http://www.mathjax.org) to display mathematical notation. You will need all this CSS and the Javascript calls in your blog's configuration for your notebook-based posts to display correctly:" | |||
|
169 | ] | |||
|
170 | }, | |||
|
171 | { | |||
|
172 | "cell_type": "markdown", | |||
|
173 | "metadata": {}, | |||
|
174 | "source": [ | |||
|
175 | "---" | |||
|
176 | ] | |||
|
177 | }, | |||
|
178 | { | |||
|
179 | "cell_type": "markdown", | |||
|
180 | "metadata": {}, | |||
|
181 | "source": [ | |||
|
182 | "1. Once authenticated, go to your blog's overview page by clicking on its title.\n", | |||
|
183 | "2. Click on templates (left column) and customize using the Advanced options.\n", | |||
|
184 | "3. Scroll down the middle column until you see an \"Add CSS\" option.\n", | |||
|
185 | "4. Copy entire the contents of the `_header` file into the CSS box." | |||
|
186 | ] | |||
|
187 | }, | |||
|
188 | { | |||
|
189 | "cell_type": "markdown", | |||
|
190 | "metadata": {}, | |||
|
191 | "source": [ | |||
|
192 | "---" | |||
|
193 | ] | |||
|
194 | }, | |||
|
195 | { | |||
|
196 | "cell_type": "markdown", | |||
|
197 | "metadata": {}, | |||
|
198 | "source": [ | |||
|
199 | "That's it, and you shouldn't need to do anything else as long as the CSS we use in the notebooks doesn't drastically change. This customization of your blog needs to be done only once.\n", | |||
|
200 | "\n", | |||
|
201 | "While you are at it, I recommend you change the width of your blog so that cells have enough space for clean display; in experimenting I found out that the default template was too narrow to properly display code cells, producing a lot of text wrapping that impaired readability. I ended up using a layout with a single column for all blog contents, putting the blog archive at the bottom. Otherwise, if I kept the right sidebar, code cells got too squished in the post area." | |||
|
202 | ] | |||
|
203 | }, | |||
|
204 | { | |||
|
205 | "cell_type": "markdown", | |||
|
206 | "metadata": {}, | |||
|
207 | "source": [ | |||
|
208 | "---" | |||
|
209 | ] | |||
|
210 | }, | |||
|
211 | { | |||
|
212 | "cell_type": "markdown", | |||
|
213 | "metadata": {}, | |||
|
214 | "source": [ | |||
|
215 | "I also had problems using some of the fancier templates available from 'Dynamic Views', in that I could never get inline math to render. But sticking to those from the Simple or 'Picture Window' categories worked fine and they still allow for a lot of customization.\n", | |||
|
216 | "\n", | |||
|
217 | "*Note:* if you change blog templates, Blogger does destroy your custom CSS, so you may need to repeat the above steps in that case." | |||
|
218 | ] | |||
|
219 | }, | |||
|
220 | { | |||
|
221 | "cell_type": "markdown", | |||
|
222 | "metadata": {}, | |||
|
223 | "source": [ | |||
|
224 | "---" | |||
|
225 | ] | |||
|
226 | }, | |||
|
227 | { | |||
|
228 | "cell_type": "heading", | |||
|
229 | "level": 2, | |||
|
230 | "metadata": {}, | |||
|
231 | "source": [ | |||
|
232 | "Adding the actual posts" | |||
|
233 | ] | |||
|
234 | }, | |||
|
235 | { | |||
|
236 | "cell_type": "markdown", | |||
|
237 | "metadata": {}, | |||
|
238 | "source": [ | |||
|
239 | "Now, whenever you want to write a new post as a notebook, simply convert the `.ipynb` file to blogger-html and copy its entire contents to the clipboard. Then go to the 'raw html' view of the post, remove anything Blogger may have put there by default, and paste. You should also click on the 'options' tab (right hand side) and select both `Show HTML literally` and `Use <br> tag`, else your paragraph breaks will look all wrong.\n", | |||
|
240 | "\n", | |||
|
241 | "That's it!" | |||
|
242 | ] | |||
|
243 | }, | |||
|
244 | { | |||
|
245 | "cell_type": "markdown", | |||
|
246 | "metadata": {}, | |||
|
247 | "source": [ | |||
|
248 | "---" | |||
|
249 | ] | |||
|
250 | }, | |||
|
251 | { | |||
|
252 | "cell_type": "heading", | |||
|
253 | "level": 2, | |||
|
254 | "metadata": {}, | |||
|
255 | "source": [ | |||
|
256 | "What can you put in?" | |||
|
257 | ] | |||
|
258 | }, | |||
|
259 | { | |||
|
260 | "cell_type": "markdown", | |||
|
261 | "metadata": {}, | |||
|
262 | "source": [ | |||
|
263 | "I will now add a few bits of code, plots, math, etc, to show which kinds of content can be put in and work out of the box. These are mostly bits copied from our [example notebooks](https://github.com/ipython/ipython/tree/master/docs/examples/notebooks) so the actual content doesn't matter, I'm just illustrating the *kind* of content that works." | |||
|
264 | ] | |||
|
265 | }, | |||
|
266 | { | |||
|
267 | "cell_type": "code", | |||
|
268 | "collapsed": false, | |||
|
269 | "input": [ | |||
|
270 | "# Let's initialize pylab so we can plot later\n", | |||
|
271 | "%pylab inline" | |||
|
272 | ], | |||
|
273 | "language": "python", | |||
|
274 | "metadata": {}, | |||
|
275 | "outputs": [ | |||
|
276 | { | |||
|
277 | "output_type": "stream", | |||
|
278 | "stream": "stdout", | |||
|
279 | "text": [ | |||
|
280 | "\n", | |||
|
281 | "Welcome to pylab, a matplotlib-based Python environment [backend: module://IPython.zmq.pylab.backend_inline].\n", | |||
|
282 | "For more information, type 'help(pylab)'.\n" | |||
|
283 | ] | |||
|
284 | } | |||
|
285 | ], | |||
|
286 | "prompt_number": 1 | |||
|
287 | }, | |||
|
288 | { | |||
|
289 | "cell_type": "markdown", | |||
|
290 | "metadata": {}, | |||
|
291 | "source": [ | |||
|
292 | "---" | |||
|
293 | ] | |||
|
294 | }, | |||
|
295 | { | |||
|
296 | "cell_type": "markdown", | |||
|
297 | "metadata": {}, | |||
|
298 | "source": [ | |||
|
299 | "With pylab loaded, the usual matplotlib operations work" | |||
|
300 | ] | |||
|
301 | }, | |||
|
302 | { | |||
|
303 | "cell_type": "code", | |||
|
304 | "collapsed": false, | |||
|
305 | "input": [ | |||
|
306 | "x = linspace(0, 2*pi)\n", | |||
|
307 | "plot(x, sin(x), label=r'$\\sin(x)$')\n", | |||
|
308 | "plot(x, cos(x), 'ro', label=r'$\\cos(x)$')\n", | |||
|
309 | "title(r'Two familiar functions')\n", | |||
|
310 | "legend()" | |||
|
311 | ], | |||
|
312 | "language": "python", | |||
|
313 | "metadata": {}, | |||
|
314 | "outputs": [ | |||
|
315 | { | |||
|
316 | "output_type": "pyout", | |||
|
317 | "prompt_number": 2, | |||
|
318 | "text": [ | |||
|
319 | "<matplotlib.legend.Legend at 0xa90d90c>" | |||
|
320 | ] | |||
|
321 | }, | |||
|
322 | { | |||
|
323 | "output_type": "display_data", | |||
|
324 | "png": "iVBORw0KGgoAAAANSUhEUgAAAXoAAAEICAYAAABRSj9aAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\nAAALEgAACxIB0t1+/AAAIABJREFUeJzt3XlYVGX7wPEv4r4huGGioeCChmahtiFkIhauaaa55ZaV\n5pL101ATy0LbzC0zM3PJrXotA/cSNMt4c8t9X9DQUsREQhTO749HeUEGnBlmOGdm7s91cSlwmHPP\nmTM3h+fcz/24aZqmIYQQwmkV0zsAIYQQ9iWJXgghnJwkeiGEcHKS6IUQwslJohdCCCcniV4IIZyc\nJHphGP3798fLy4uHHnrI5o9doUIFTp06BcDzzz/PhAkTANi6dSsNGza06b7s+TwK8tRTT7F48eIi\n3adwDMX1DkAYV/ny5XFzcwPg2rVrlC5dGnd3d9zc3Jg7dy49e/a02b62bt3Kpk2b+PPPPyldurTN\nHve2q1evZv/fzc0t+3kFBwdz6NAhm+3H3s/jtqioKI4fP54rsa9Zs8Zu+xOOTRK9yFdqamr2/+vU\nqcP8+fNp3bq1XfZ1+vRpfH197Zocc7LFPMHMzEzc3d1zfa2on4cQ5pChG2GR9PR0ypQpQ3JyMgDv\nvPMOJUqUyP6lMGHCBEaNGgXAlStX6Nu3L9WqVcPX15d33nnHZIKdP38+gwcP5tdff6VChQpMmjSJ\nlJQU2rdvT7Vq1fDy8qJDhw6cO3cu+2dCQ0OZMGECjz76KBUqVKBjx45cvHiRXr164eHhQYsWLTh9\n+nT29sWKFePEiRN59h0XF0etWrWyP58yZQr+/v5UrFiRxo0b891332V/78svv+TRRx/l1VdfpUqV\nKkyaNKnA5xEVFcWXX35JcHBwru1yxvL8888zdOhQ2rdvT8WKFXnooYdyxbl//37CwsKoXLky3t7e\nREdHs379eqKjo1mxYgUVKlSgWbNm2cdk/vz5gPpFNnnyZHx9falevTr9+vXjn3/+AeDUqVMUK1aM\nRYsWce+991K1alXefffd7H0mJCQQFBSEh4cH3t7ejB49Os9xE45FEr2wSOnSpWnRogVxcXEAxMfH\n4+vry88//5z9eWhoKACvvPIKV69e5eTJk8THx7No0SIWLFiQ5zEHDhzIp59+ysMPP8zVq1eZOHEi\nWVlZDBw4kDNnznDmzBnKlCnDsGHDcv3cihUrWLJkCefOneP48eM8/PDDDBw4kOTkZAICAvIkYnP4\n+/vz888/888//zBx4kR69+7NhQsXsr+fkJCAn58ff/31F5GRkQU+j6ioKLP2uWLFCqKiorh8+TL+\n/v6MGzcOUMNNbdq04amnniIpKYljx47xxBNPEB4eTmRkJD169ODq1avs2rULyD0ktWDBAhYuXEhc\nXBwnTpwgNTU1z/Hbtm0bR44c4ccff+Stt97i8OHDAIwYMYJRo0Zx5coVTpw4Qffu3S0+jsJYJNEL\ni4WEhBAfH09mZiZ79+5l+PDhxMfHk56ezu+//06rVq3IzMxkxYoVREdHU65cOe69915Gjx6d783C\nO6/0vby86NKlC6VLl6Z8+fJERkYSHx+f/X03Nzf69+9PnTp1qFixIk8++ST169endevWuLu788wz\nz2QnQEt069YNb29vALp37069evX47bffsr9/zz33MHToUIoVK2ZyeMbSISE3NzeefvppgoKCcHd3\np1evXuzevRuAmJgY7rnnHkaNGkXJkiUpX748LVq0yN5PQfv66quvGD16NL6+vpQrV47o6GiWL19O\nVlZW9jYTJ06kVKlSNGnShKZNm7Jnzx4ASpYsydGjR7l48SJly5alZcuWFj0nYTyS6IXFQkJCiIuL\nY+fOnQQGBtKmTRvi4+P57bff8Pf3x9PTk4sXL3Ljxg3uvffe7J+rXbt2ruGXgqSlpTFkyBB8fX3x\n8PAgJCSEK1eu5Epu1atXz/5/6dKlqVatWq7Pc95jMNeiRYto1qwZnp6eeHp6sm/fPi5dupT9/ZzD\nPLaS83mUKVMmO+7ExETq1q1r1WMmJSXlOfY3b97M9dfJ7V9oAGXLls3e7/z58zly5AgBAQG0aNGC\n2NhYq2IQxiGJXljs4Ycf5vDhw6xatYrQ0FACAgI4c+YMa9asyR62qVKlCiVKlMguaQQ4c+YMPj4+\nZu3jww8/5MiRIyQkJHDlyhXi4+MLvIq9PWRhLlPbnz59mhdeeIHZs2eTnJzM5cuXue+++3Lt09L9\nlCtXjrS0tOzPz58/b/bP1q5d2+R9BVDj/AW555578hz74sWL5/qlkh9/f3+WLl3K33//zZgxY+jW\nrRv//vuv2XEL45FELyxWtmxZHnzwQWbPnk1ISAgAjzzyCJ9++mn25+7u7nTv3p1x48aRmprK6dOn\nmTZtGr179zZrH6mpqZQpUwYPDw+Sk5NNjrfnTMCWDJnk9wvj2rVruLm5UaVKFbKysliwYAH79u0z\n+3FNadq0Kfv372fPnj2kp6fnGbcvKO6IiAiSkpKYPn06169f5+rVqyQkJADqr4BTp07l+/M9e/Zk\n2rRpnDp1itTU1Owx/bv9ggBYsmQJf//9NwAeHh64ubmZ9XPCuOTVE1YJCQnh5s2b2WPGISEhpKam\n0qpVq+xtZs6cSbly5ahbty7BwcH06tWL/v37m3y8nDcSAUaOHMm///5LlSpVeOSRR3jyySfzXE3n\n/PzOnzf1/fy2vf3/Ro0aMXr0aB5++GG8vb3Zt28fjz32WIH7uNvzqF+/Pm+++SZt2rShQYMGBAcH\nmx13hQoV2LhxIz/88AM1atSgfv362TfBn3nmGQAqV65MUFBQnjgGDBhAnz59aNWqFXXr1qVs2bLM\nnDnT5PG40/r167nvvvuoUKECo0aNYvny5ZQqVarA5y2MzU0WHhFCCOdWqCv6AQMGUL16dQIDA/Pd\nZvjw4dSrV4+mTZtaVQUhhBCicAqV6Pv378+6devy/f6aNWs4duwYR48e5bPPPuOll14qzO6EEEJY\noVCJPjg4GE9Pz3y/v3r1avr16wdAy5YtSUlJyVXeJYQQwv7s2uvm3LlzueqOfXx8OHv2bJ4SL0tL\n1oQQQijm3Ga1e9XNnUHkl9S1Wx99PT2z/5/z44XGjYn088v1tUg/P+JjYrLL5fT6mDhxos0ea9cu\njVGjNFq21ChbVuPBBzWGDdNYulRj506NuDiNb7/V+OwzjXff1Rg9WqNTJw0PD40ePTTWrtW4eVOf\n2B392Ev8rhN/fEwMkX5+TLwjn4wMDDSZf57NJy+NDw/X9XmYy65X9DVr1iQxMTH787Nnz1KzZs18\nt4/086N8xYpw+XKe76WcP8/cHDMUAd45fpwJM2fSKiLCdkHr5OBBePNN+PlnePllmDoVgoKgXDnz\nfj45GZYvV48xYAD07g3PPw+NGtk1bCEc0oYZM3jn+HGicnztnePHebZyZZPbV6hTh3FeXrxz/Hj2\n1yL9/Gj3yiv2DdRG7HpF37FjRxYtWgTA9u3bqVSpUr4z8yaEh9Nu+nSeffttxvn55fpepJ8f99xz\nj8mfc09Pt23QRezECejXD0JCVGI/dgwmTFCfm5vkAby81C+IhAT48UcoVgyeeAJefBFuNS0UQtxS\n/Pp1k1+v5O1tMv/0eestwqdPZ0J4OFEhIdn5ylEuMgt1Rd+zZ0/i4+O5ePEitWrVYtKkSdy4cQOA\nIUOG8NRTT7FmzRr8/f0pV66cyc6Ft719R/XOhJkzcU9PJ7N0adq98gobZsyAvXvz/FymAfp+3572\nb4kLFyAqCr7+GoYNg6NHwcPDNvEEBMCUKTB2LIweDYGB8PnnEBaWd1trYjcSiV9fjhr/zVsTwELv\n+Ho1Hx/CXnklT/65ndAdJbHfyRATptzc3O463rQlNpb1I0bk/dNp+nRA/SlW/Pp1bpYqRdvhww39\ngmzfDt26QffuEBkJVarYd3/r1sELL0C7dvDBB1Cxon33J4SRbImNzZMfgHzziZFzx53MyZ3gQIke\n1Au2Mcdv2rBb42N3vmDj/PwIN+gL9sUX6kp7/nzo0KHo9vvPP/D66yrpf/YZhIcX3b6FuM3Ly4vL\nJu7BiYJ5enpmL/aTk1MmelPGh4czecOGPF+fEB6eZzhITzduwKhRsHEjfP892Hg9arNt3AiDBqkr\n/MhIkMpWUZQK8153ZfkdN3OPp8OvGZvfTRUj3aT96y945hmoUEHdLLXVWLw1wsLU0FFYmLrKnzJF\nkr0Qzs7hu1fezKernhFu0gLs2gXNm0NwMKxerW+Sv61GDYiPh59+UpU6ORYdEkI4IYdP9G2HDzdZ\nDhVmgPrW/fvhySfh/fdh8mRV8mgUlSurMswDB6BvXzW0JIRwTg4/Rg+mb9K2iogwebe9qG7QnjwJ\nrVqpiU/PPVcku7RKWhp07QqlSqkJVwb5Q0g4KRmjt05hx+idItGbYqocs6iqcc6fh8ceUzdfhw61\n665sIiMDevVSE5K//96yiVpCWEISvXUKm+gNNJhgW7enOOf0zvHjbMyxyo49pKSo0sV+/RwjyQOU\nLAnLlqmx+169ZMxeiMI4efLkXbdJSkrKtZawvTltotejGictDdq3h8cfh/Hj7bYbuyheXNX2Jyer\nFgxCCMudOHGC7du333W7qlWr8t577xVBRIrTJvqirsbJyFCzXf384KOPHLNksWRJ+PZbdXW/dKne\n0Qihv6ysLPz9/c26SgeYO3cuPXv2vOt2xYsXJyIiIrsXmL05baIvymocTYPBg6FECXVVbKTqGktV\nrarG6UeMUDX/QriyYsWK8f777+Pj43PXbffs2WPWdrc1b96cTZs2FSY8szn8hKn83L7hml9zIlua\nN0/Vy//2mxoCcXSBgeoX1tNPq+dUQGdpIZxely5dzNouJiaGzp07W/TYVatW5dixY/j7+1sTmvk0\nAzBIGFbZvVvTqlTRtEOH9I7E9qKjNS0oSNOuXdM7EuEsjP5e3717t/bZZ59p3377rdaxY0ftxx9/\n1AIDA7Wff/5Z0zRNW7hwoValShVt06ZN2rJly7R+/fppp06d0jRN0zp16qRlZWVZtL+FCxdqy5cv\nv+t2+R03c4+nAw8yWG9LbCzjw8OJCg1lfHg4W2JjrXqcq1dVa4OPP4YGDWwcpAGMGaOe14ABanhK\niKLg5mabD2t8/vnntGvXjqeffpoOHTrQunVrmjVrxs2bNwHo27cvAQEBZGRk0KNHDx544AG++eYb\nANLS0vKsoLd69WpiY2MZO3YsX331FX369OHQoUPZ3/f09OTs2bPWBWsBJxhosIzJ+vpb/7dkWEfT\nYMgQCA1VJYnOyM1N9bEPDVXtjV9/Xe+IhCvQ86KiS5cuBAUFERwczKhRowA1Tp+Tu7s7DW91JfTw\n8ODUqVMAZGZm5truzJkzNGrUCH9/f958803Gjh2Lh4cHtWvXzt6mTJkyZGRk2PEZKS53RW+r+vp5\n82DfPrjVDt9plS4NK1eqNg5//KF3NELYl6+vLwcOHKBTp04MHjyY8+fPA3nXunZ3d8/+/+3vFb/j\nBl3t2rXx9/fnwoULVKhQgUqVKtG+fXvKli2bvc2VK1fw8vKy19PJ5nKJ3hb19Xv2wLhxanWoMmVs\nFZlx1a6tEn2fPpDP4RPCKcyZM4fy5cvTp08fRowYkZ3otTv+zLj9uZZjkW5vb29SU1Oztzl06BB7\n9uxhzZo1tGrVClA3bHNKSkqy/41YXDDRF7a+/upVtTKUs47L56dvX6hbVy1/KISzKlWqFJ9//jlf\nffUVqampnD9/nl9++YXZs2eTlpbG8uXLOXjwIFOmTGH37t0sW7aMtWvX8t///peQkBASctQkb9iw\ngZiYGDRNIz09nVWrVlGtWrVc+9u9ezePPvqo/Z+YuXeH7akow4iPidEi/fw0TQ0Fahpob/j5afEx\nMWb9fK9emjZokJ2DNKgLFzTN21vTbhUgCGExg6Qcu7h8+bI2btw4s7f/999/tVGjRpm1bX7Hzdzj\n6XI3YwtTX796taor37PH3lEaU7Vq8Omnqo/P7t1QvrzeEQlhHJUqVaJKlSpcvHiRKmYsBL18+XKG\nDBlSBJE5cfdKW/vnH2jcGBYtUr1sXFn//uom7Zw5ekciHI0jvNcLQ9M0Pv/8cwYPHlzgdomJiezc\nuZNOnTqZ9bjSpriIDBsG6emq3NDVXbkCTZuqRP/kk3pHIxyJI7zXjUgSvY2ZWqykhFcEXbuqFaM8\nPfWO0Bg2b4bevVXJZeXKekcjHIWR3uuOxOUXB7clU5OpIo8d5/sb8PHHEZLkc3j8cVV9NHIkLF6s\ndzRCiILIFX0O48PDmbxhQ56vR1QNJ+bCOodsPWxP165BQAAsWaKWTRTibozyXnc0ssKUDeU3maqJ\nX7okeRPKlVOtEYYNg1utQIQQBiSJPof8JlMV95AVs/PzzDOqh/0nn+gdiRAiP5LoczC5WEld+yxW\n4izc3GDWLHj7bbhwQe9ohBCmyBj9HbbExhL74Ux2/pzOfS1K0+UN+yxW4mxefx3+/hu+/FLvSISR\nGem97kikvNIOXnpJTQiaNk3vSBzH1avqxuzKlfDII3pHI4zKaO91RyGJ3sb271elg4cPS828pZYt\ng/feg99/hxxdXIXIZqT3uiORqhsbe+01GD9ekrw1evQADw+YO1fvSITQ38mTJwv8flJSEmlpaUUS\niyT6HNatgxMn1NCNsNztG7NRUWq8XghXdeLECbZv317gNlWrVuW9994rknhk6OaWmzdV/5boaOjY\nMe/3TbVGkJu0po0aBampahUuIXIywnu9KIwZM4apU6fedbv//ve/HDx4kL59+xa4nbRAsJHPP4fq\n1aFDh7zfs9U6s64iKgrq1VMJv1EjvaMRjsIWF1NGuCDbs2cPPj4+Zm3bvHlzZs6ceddEX2hmda23\nM73DSEnRtOrVNW3XLtPfH9e2ba6FSm5/jA8PL9pAHcgHH2hap056RyGMJr/3uqkFgSItWBDIVo9h\nC5MnT9b27dtn9vavvvqqdvTo0QK3ye+4mZs75YoeNVwTEQH332/6+7ZYZ9bVDB2qFk7/5RcptxR3\nt2HGjFx/MQO8c/w4E2bONPuK3BaPAfDJJ59w7do1ypYtS6lSpRg0aBBLly4lOTmZUqVKUaxYMQYO\nHMiePXtISEigcuXKLFy4kO+//x5QwzGRkZFm769p06bs2LHDrmvHunyiP3lSDdv88Uf+2xR2nVlX\nVLo0TJoEY8dCfDzSK0gUyBYXU7Z4jK1btxITE8OaNWvYtWsXn3zyCc2aNWPz5s3Mu3XTadSoUWzZ\nsoWvv/6a//u//6NWrVpcunQp+zHS0tJwy3HCr169Gnd3d7Zu3UpgYCDr1q1j3LhxNGzYEABPT0+O\nHDlidozWcPmqm7FjYcQIuOee/Lcx2RrBT1oj3E3fvnDpEqxZo3ckwuhscTFli8f4z3/+Q3BwMADN\nmjVj3rx5rFy5ksaNG2dv06hRI5YtW0aXLl0ICgqiW7duNMpxMyozMzP7/2fOnKFRo0ZERESwceNG\nIiIiePbZZ6ldu3b2NmXKlCEjI8PsGK3h0lf0CQmwbRssWFDwdoVZZ9aVubvDu+/CG29Au3YyiUrk\nr+3w4Yw7fjz3WhB+frSz4GLKFo+haRpZWVm5vnb9+nWu5/hrISMjgxs3buDr68uBAwdYs2YNgwcP\nZvPmzVSvXp3ixf+XVm8n9AsXLlChQgUqVapE+/btcz3+lStX8PLyMjtGa7h0on/zTTU5qmzZu2/b\nKiJCErsVOnZUs2WXLoU+ffSORhiVLS6mbPEYnTp1YvLkyYwbNw6AH374ga5duzJr1qzsbfbs2UPX\nrl2ZM2cOkydPpk+fPqSlpXH+/HmqV6+Ot7c3qamplC9fnkOHDnH9+nV27txJq1uLNsTExORK9klJ\nSQQEBJgdozVcto5+2za1FN7hw1CyZJHu2uVs3aqGcQ4dgnz+uhYuwhHq6GfMmMHFixfx8/OjYcOG\ntGzZki+++IK0tDSysrJwd3dn6NChjB8/nho1alCpUiXOnz/P6NGjAfjiiy/w9fWldevWzJgxg6tX\nr1KjRg0OHTrEww8/TM2aNWnRokX2/gYNGsSsWbMoXcAQk/S6sVLr1irRDxhQpLt1We3bQ1iYuh8i\nXJcjJPrCSklJ4YMPPmDy5Ml33TY9PZ3IyEg++uijAreTXjdW2LwZEhPVVaYoGtHRarz+n3/0jkQI\n+6pUqRJVqlTh4sWLd912+fLlDBkyxO4xuVyi1zSYMAEmToTiNrhDsSU2lvHh4USFhjI+PJwtsbGF\nf1AnFBgI4eHw4Yd6RyL0cPt94ipGjBjBqlWrCtwmMTERT09PGjRoYPd4XG7oZv16NTV/797CV4GY\nbI3g50f49Oly49aEU6fgwQfhyBGoXFnvaERRyfk+cQOnH7qxB92HbtatW0fDhg2pV6+eySY+cXFx\neHh40KxZM5o1a2bWuJW93L6aj4qyTalffjPxNs6cWfgHd0K+vtC1K3z8sd6RiKJk6n0iilahBi8y\nMzMZNmwYmzZtombNmjRv3pyOHTvmKRUKCQlh9erVhQrUFmJi4Pp16NbNNo8nrREs98Yb0Lw5vPqq\n9Px3Ffm9T0TRKdQVfUJCAv7+/vj6+lKiRAl69OiR3e8hJyP8qZaVperm33oLitnozoS0RrBcnTqq\ntn7GDL0jEUUlv/eJKDqFuqI/d+4ctWrVyv7cx8eH3377Ldc2bm5u/PLLLzRt2pSaNWvywQcf5Jou\nfFtUVFT2/0NDQwkNDS1MaHmsWqVuvprqNW8tW8zEc0WRkfDwwzBypFqRSjg3U+8TYZ24uDji4uIs\n/rlCJXo3MzpVPfDAAyQmJlK2bFnWrl1L586dTTbwyZnobS0zU1XZvP++bZtrSWsE6/j7w5NPqtWo\nbk1AFE4s5/uE9et1jsax3XkRPGnSJLN+rlBVN9u3bycqKop169YBEB0dTbFixRgzZky+P1OnTh12\n7NiRq7eDvatuVq6EadNUy1zpomgMhw9DcDAcPw4VKugdjSgqXl5eXL58We8wHI6npyfJycl5vl4k\nVTdBQUEcPXqUU6dOkZGRwYoVK+h4x9jIhQsXsgNJSEhA0zS7N/DJSdPURJ3x4yXJG0mDBtCmDXzy\nid6RiKKUnJyMpmnyYeGHqSRviUIN3RQvXpxZs2YRHh5OZmYmAwcOJCAggLlz5wIwZMgQvvnmG+bM\nmUPx4sUpW7Ysy5cvL1TAllq7ViX7p54q0t0KM4wbp1pRDBsG5crpHY0QzsupJ0xpGjz2GAwfDs8+\na/OHFzbQvTu0aAGvvaZ3JEI4HmlqBmzZAgMHqq6J0gvdmP74A9q2hRMnzGsXLYT4H2lqhhqbHztW\nkryRNWmi1pT97DO9IxHCeTntFf2OHdC5s6rq0KPf/JbYWDbMmEHx69e5WaoUbYcPl7LLfOzapdoY\nHz+u1poVjkvO+6Jlbu502hWmoqNh9Gj9knyeZme3/i8nfV7NmkHTprB4MQwerHc0wlpy3huXUw7d\nHDyoxuf1ShrS7MxyY8bABx+oyW3CMcl5b1xOmeinTlWVNnqV7EmzM8u1agWVKoGJVknCQch5b1xO\nl+hPn4YffoChQ/WLQZqdWc7NTV3VT52qymKF45Hz3ricLtF/8IEastGzBW7b4cMZ5+eX62uRfn6E\nSbOzAnXqBJcvq8XEheOR8964nKrq5sIFCAhQY/TVq9sgsELYEhvLxhzNzsKk2ZlZ5s2D774DWZHR\nMcl5X7RccsLUuHHqilD6pziu9HTVs37DBrXOrBAify6X6K9dU0vV/fqraoMrHFd0tPqrbNEivSMR\nwthcLtHPmgWbN8O339ooKKGblBSoWxd274batfWORgjjcqlEn5kJ9erBV1+plYuE43vtNfW6Tpum\ndyRCGJdL9bpZtQq8vSXJO5ORI2HhQihkG24hBE6Q6DVNlVRKm1vn4uOjyi3nzNE7EiEcn8MP3Wzb\nBv36qaXpHKFLpTR9Mt+BA2phkpMnoUwZvaMROcl5bAwu09Tsgw/g1VcdJ8lL0yfzNWoEzZvDkiXS\n7MxI5Dx2PA49dHP0qLqif/55vSMxjzR9styoUeqGrP5/d4rb5Dx2PA6d6KdNgyFDHGdlImn6ZLnH\nH1etptev1zsScZucx47HYRP933/DsmVqYWlHIU2fLOfm9r+remEMch47HodN9HPmQNeu+ve0sYQ0\nfbJOjx5qbdl9+/SORICcx47IIatu0tNVu4OfflI37ByJNH2yzuTJcOoUfP653pEIkPPYKJx6Zuy8\neWqBipgYOwYlDOXiRTX7+fBhqFZN72iEMAannRmrafDxx2rcVriOKlWge3eZQCWENRwu0W/apGrm\nW7fWOxJR1EaOVIleijuEsIzDJfqPP4YRI1Q1hnAtAQHwwAOwdKnekQjhWBxqjP7wYQgOVuvCypR4\n17Rxo5oJ/ccf8steCKcco58xQ02QkiTvutq0Uf9u2qRvHEI4Eoe5or98Gfz8VC31PfcUUWDCkL74\nAr75Btas0TsSIfTldOWVH3wAe/bA4sVFFFQRk26A5rs9j2LzZjVuL+xHzktjc6rulTdvwsyZzrtM\noHQDtEzp0vDii2ooT8ot7UfOS+fhEGP0330HtWpBUJDekdiHdAO03IsvwooVakhP2Iecl87DIRL9\nxx+rGmpnJd0ALeftDe3bS0sEe5Lz0nkYPtH//jskJkLnznpHYj/SDdA6w4fD7NlqaE/YnpyXzsPw\niX76dHjlFSjuEHcTrCPdAK0TFAQ1a8Lq1XpH4pzkvHQehq66+fNPaNwYTpwAT08dAitC0g3QOitX\nqqv6+Hi9I3FOcl4am1OUV06YAMnJ6o0shCk3bkDduvDDD3D//XpHI0TRcvhEn54O996rrtQaNtQp\nMOEQoqPV+sFffKF3JEIULYdP9AsXqqUC163TKSjhMC5dAn9/OHIEqlbVOxohio5D97rRNHUTdvhw\nvSMRjqByZejWDebO1TsSIYzJkFf027ZB//5w6BAUM+SvImE0e/dCu3Zw8iSULKl3NEIUDYe+op8x\nQ5VUSpIX5goMhAYNnLdNhhCFYbgr+rNnoUkTtRB0xYr6xiUcy/ffqxuz27frHYkQRcNhr+jnzIE+\nfSTJC8slFer7AAAYrklEQVS1bw9//QW//aZ3JEIYi6Gu6P/9V5VUbtsG9erpHZUxSJtYy3z0EezY\nAV99pXckjkXOM8fkkG2Kly2D5s0lyd8mbWItN2AAvP02JCVBjRp6R+MY5DxzfoYZutE0dRNWSir/\nR9rEWq5SJejZU0otLSHnmfMrdKJft24dDRs2pF69ekydOtXkNsOHD6devXo0bdqUXbt2mdxm61Y1\nGzYsrLAROQ9pE2udYcNUos/n8Ik7yHnm/AqV6DMzMxk2bBjr1q3jwIEDLFu2jIMHD+baZs2aNRw7\ndoyjR4/y2Wef8dJLL5l8LCmpzEvaxFqnUSO47z74+mu9I3EMcp45v0Kl1YSEBPz9/fH19aVEiRL0\n6NGD77//Ptc2q1evpl+/fgC0bNmSlJQULly4kOexNm+Gvn0LE43zkTax1hs+XM2u1r/UwPjkPHN+\nhboZe+7cOWrVqpX9uY+PD7/dUdtmapuzZ89SvXr1XNvVrx/Fhx+q/4eGhhIaGlqY0JzC7RthE3K0\niW0nbWLN8tRTalWy336Dhx7SOxpjk/PMMikpcOAAPPJI0e87Li6OuLg4i3+uUInezc3NrO3uLP8x\n9XOLF0fh71+YaJxTq4gIecNZwd1djdXPnCmJ3hxynpnviy9UCa8eif7Oi+BJkyaZ9XOFGrqpWbMm\niYmJ2Z8nJibi4+NT4DZnz56lZs2aeR5Lkrywtf79Ye1atYCNELaQmQmzZjledWChEn1QUBBHjx7l\n1KlTZGRksGLFCjp27Jhrm44dO7Jo0SIAtm/fTqVKlfIM2whhD5UqQY8eUmopbCc2VrXCbtlS70gs\nU6ihm+LFizNr1izCw8PJzMxk4MCBBAQEMPfWO2vIkCE89dRTrFmzBn9/f8qVK8eCBQtsErgQ5hg2\nDFq3hshIyKe4RAizOepcH0O1QBDCHtq2VRVdvXvrHYlwZPv3Q5s2cPq0cVphO2xTMyFsTUothS3M\nnAkvvmicJG8JuaIXTi8zE+rXV43OpAJHWOPyZbUI/cGD4O2tdzT/45BNzYT5pNug+W6XWk6fLole\nzhvrzJ+v2mAbKclbQhK9A5Jug5a73dXy3DkwUd3rEuS8sc7tkkpHbqkhY/QOSLoNWs7DA557Ti1s\n46rkvLHODz+oltfNm+sdifUk0Tsg6TZonVdegXnzVJdUVyTnjXUctaQyJ0n0Dki6DVqnQQN48EG1\nwI0rkvPGcvv2waFD0LWr3pEUjiR6ByTdBq3nyqWWct5YbuZMeOklxyypzEnKKx3UlthYNuboNhgm\n3QbNkpWl+tXPnQshIXpHU/TkvDFfcjL4+akreqN2bTE3d0qiFy7nk0/gxx/h22/1jkQY2XvvqaGb\nW626DEkSvRD5SE2Fe+9VrWZ9ffWORhjRjRvqav677+CBB/SOJn/SAkGIfJQvD88/D7Nn6x2JMKpV\nq9RFgJGTvCXkil64pJMnVV306dNQrpze0QijeeQReO01ePppvSMpmFzRC1GAOnXgscdg8WK9IxFG\nk5AASUnQqZPekdiOJHrhskaMUJNh5I9JkdP06Wpynbu73pHYjiR64bJCQ6F4cdi4Ue9IhFGcO6eW\nnxw4UO9IbEuamjkh6VBoHje3/02gattW72hsR15/633yiVqgxsND70hsSxK9k5EOhZbp1QvGjVOT\nYho21DuawpPX33ppaaoX0rZtekdiezJ042SkQ6FlypRRqwZ9/LHekdiGvP7W++orteh3vXp6R2J7\nkuidjHQotNzLL8OKFXDxot6RFJ68/tbRNPXLfuRIvSOxD0n0TkY6FFquenXo0kX1v3F08vpbZ9Mm\nKFYMWrfWOxL7kETvZKRDoXVGjVIzZfO5IHYY8vpb5/bVvJub3pHYh8yMdULSodA6YWHQpw/07at3\nJIUjr79lDh+G4GA1S7pMGb2jsYw0NRPCQmvWqAqcnTud98pO5DV0KHh6wuTJekdiOUn0QlgoKwsa\nN1a11I8/rnc0oihcvKiqbA4eBG9vvaOxnPS6EcJCxYqpcdpp0/SORBSVOXNU4zJHTPKWkCt6IXJI\nS1PtaX/+GerX1zsaYU/p6eq1/vFH9ZecI5IreiGsULYsvPCCaosgnNuSJWqxeEdN8paQK3oh7pCU\npN78x46Bl5fe0Qh7uH0/ZvZsx66dlyt6IaxUowZ06ACffaZ3JMJe1q5VpZSuctNdruhdiHQ1NN+e\nPfDUU3DiBOQz2VR38npa7/HHYfBgeO45vSMpHHNzp3SvdBHS1dAyTZvCfffB0qXQv7/e0eQlr6f1\nduyA48fhmWf0jqToyNCNi5Cuhpb7v/+D995T47lGI6+n9T78UK0uVqKE3pEUHUn0LkK6GlqudWu1\ncHhMjN6R5CWvp3XOnIH169WwjSuRRO8ipKuh5dzc1FX91Kl6R5KXvJ7WmT4dBgyAihX1jqRoSaJ3\nEdLV0Dpdu8L588ZbdUheT8ulpMCCBWr5SFcjVTcuRLoaWmfOHFWOt3q13pHkJq+nZd5/X1VTLVmi\ndyS2I03NhLCRf/+FOnXgp5+gUSO9oxHWuH4d6taF2Fi4/369o7EdmTAlhI2UKQPDhqkrQuGYFi5U\nCd6Zkrwl5IpeCDMkJ4O/P/zxB/j46B2NsMTNm9CgASxaBI8+qnc0tiVX9ELYkJcX9Osnzc4c0cqV\n6pezsyV5S8gVvRBmOnMGmjVTsyorVdI7GmGOrCw1y/n996FdO72jsT25ohfCxmrXhogI+PRTvSMR\n5oqJUTNgw8P1jkRfckUvpDmWBfbuhbZtVbOzolpIWl4f62gaPPQQvP46dOumdzT2IU3NhFmkOZZl\nAgOhZUv4/HMoirlJ8vpYb/NmuHIFunTROxL9ydCNi5PmWJabMEG1RSiKtjLy+lgvOhrGjgV3d70j\n0Z8kehcnzbEs9+CDqh57wQL770teH+skJMCRI9Crl96RGIPViT45OZmwsDDq169P27ZtSUlJMbmd\nr68vTZo0oVmzZrRo0cLqQIV9SHMs60yYoK4YMzLsux95fawTHQ2vveZarYgLYnWinzJlCmFhYRw5\ncoQnnniCKVOmmNzOzc2NuLg4du3aRUJCgtWBCvuQ5ljWadlStUNYuNC++5HXx3L798Ovv8LAgXpH\nYhxWV900bNiQ+Ph4qlevzvnz5wkNDeXQoUN5tqtTpw6///47lStXzj8IqbrRlTTHss4vv6ihgSNH\n7HvlKK+PZfr0Ub+E33hD70jsz+5NzTw9Pbl8+TIAmqbh5eWV/XlOdevWxcPDA3d3d4YMGcJgEx3/\n3dzcmDhxYvbnoaGhhIaGWhOWEEWqTRuV7I243KArOnQIgoPh2DHw8NA7GtuLi4sjLi4u+/NJkyYV\nPtGHhYVx/vz5PF9/55136NevX67E7uXlRXJycp5tk5KSqFGjBn///TdhYWHMnDmT4ODg3EHIFb1w\nUFu2qIUsDh2C4lKsrLuePVUJbGSk3pEUDZvU0W/cuDHf790esvH29iYpKYlq1aqZ3K5GjRoAVK1a\nlS5dupCQkJAn0QvhqFq1Un1Uli1TQwZCP/v2qVbS8+bpHYnxWH0ztmPHjiy8dSdq4cKFdO7cOc82\naWlpXL16FYBr166xYcMGAgMDrd2lEIb05psweTJkZuodiWubOFHNgi1fXu9IjMfqMfrk5GS6d+/O\nmTNn8PX1ZeXKlVSqVIk///yTwYMHExsby4kTJ3j66acBuHnzJr169eINE3dIZOhGODJNU1f2L7+s\nhg5E0du1S/UhOnYMypbVO5qiIytMiUKTHivm27gRRoxQvXCsnYkpx9t6HTpAWJjrrQcrvW5EoUiP\nFcu0aQOenrB0qXVj9XK8rffbb7B7N3z9td6RGJe0QBAmSY8Vy7i5wZQparw+n64FBZLjbb0334Rx\n40AmC+dPEr0wSXqsWC44GBo3tq5fvRxv6/z8s5qwNmCA3pEYmyR6YZL0WLFOdDS8+y78849lPyfH\n2zoTJqiPkiX1jsTYJNELk6THinUCA9VqRh9+aNnPyfG23E8/wdmz0Lev3pEYn1TdiHxJjxXrnDql\nWhkfOADVq5v/c3K8zadp8Nhj8NJL0Lu33tHoR8orhdDRyJFqApXcS7WP//wHoqJU/bwrLywiiV4I\nHf39NwQEqAUw6tbVOxrncv36/256t2mjdzT6Mjd3yhi9EHZQtaqavDNhgt6ROJ9Zs6BhQ0nylpAr\neiHsJDUV6tWDtWvV0oOi8C5eVEl+61b1F5Ork6EbYTcyVd98s2ZBbKxK9rfJ8bPeK6+oG7GzZukd\niTFICwRhFzJV3zIvvADTpsGmTWqoQY6f9Q4dguXL4eBBvSNxPDJGLywiU/UtU7IkfPSRuhLNyJDj\nVxivvw5jx0KVKnpH4ngk0QuLyFR9y3XsCHXqwPTpcvystWmTmpcwbJjekTgmSfTCIjJV33JubirJ\nT50KqZocP0tlZsLo0fDee5DP6SfuQhK9sIhM1bdOvXowZAgcQo6fpRYsUAt931rDSFhBqm6ExWSq\nvnWuXYNGjeD1F2O5EC/HzxxXrqgyytWrIShI72iMR8orhTCgb79Va5vu2gUlSugdjfG9/DLcuCEL\nfudHEr0QBqRpqrvlk0/CqFF6R2Ns27ZB9+6wb59avUvkJYleCIM6fFh1XvzjD6hRQ+9ojOn6dWjW\nDN56C7p10zsa45JEL4qczPjMraDjMXYsnDsHixfrHKRB3e5M+d13qmpJmCYzY0WRkhmfud3teIwf\nr24yxsdDSIheURrTgQMwe7ZK9JLkbUPKK4VNyIzP3O52PMqXh08+gf794epVPSI0pqwsGDwYJk0C\nHx+9o3EekuiFTciMz9zMOR4dOsDjj6vJQEKZO1f9++KL+sbhbCTRC5uQGbO5mXs8pk2DjRtVh0tX\nd/YsvPmmKqUsJpnJpuRwCpuQGbO5mXs8KlaEL79UXS4vXizCAA1G01Qfm6FD1aQyYVtSdSNsRmbM\n5mbJ8XjtNTh9GlaudM0bkIsXQ3S0ugEr/WzMJ+WVQjiQ9HR48EGIjIRevfSOpmgdPAitWsFPP0Fg\noN7ROBZJ9EI4mJ07oV079a+rVJykpUHLljByJAwcqHc0jkcSvTAMZ59IZcvnN3myqq1fv941bkgO\nGqRmwS5a5JpDVoUlE6aEITj7RCpbP7+xY+GHH1T/emfvhbN4Mfz8M/z+uyR5e3OBawahJ2efSGXr\n51e8uFoX9b33YMMGW0RoTAcPwquvqpvP5cvrHY3zk0Qv7MrZJ1LZ4/nVqQMrVkDv3qoBmrNJS1Nd\nKaOjoUkTvaNxDZLohV05+0Qqez2/Vq3g3XfVerOXLxfqoQxn+HBo2lRuvhYlSfTCrpx9IpU9n9+g\nQapv/bPPws2bhX44Q/j0UzUu/+mnMi5flKTqRtids0+ksufzu3kTIiJUp8uPP7bJQ+pm5UpVRrll\nC/j76x2Nc5DySuEQHKX0Us84U1JUrflrr6nOjo5o/Xro21fdYG7aVO9onIeUVwrDc5TSS73jrFRJ\nlVw+9hjUqwehoXbfpU39+qu6sfzdd5Lk9SJj9EI3jlJ6aYQ469eHZctUtcqPPxbZbgtt717o3FlN\niHr0Ub2jcV2S6IVuHKX00ihxPvEEfP019OyprvCN7sQJ1dLh44/VTWWhH0n0QjeOUnpppDhDQlTv\n+sGDYenSIt+92ZKSICwMxo9Xv5iEvuRmrNCNqbHvSD8/2k2fDqDLzU9TN12BfOPU617Cvn3qavnN\nN1UveyPZsQOefhpefhnGjNE7GucmVTfCIZgqTYS8iXWcnx/hdk6sJm+63tovYLgS0WPH1FXz0KGq\nIscIvvpKlVB++il07ap3NM7P7NypGYBBwhAGMa5tW01Tiw7l+hgfHu6U+y2MxERNa9BA015/XdOu\nX9cvjhs3NO3VVzXNz0/T9u7VLw5XY27ulDF6YTh63fw0yk1XS/j4qAlI+/dDixawe3fRx3DpkrrZ\nuncvJCTAffcVfQyiYFJHLwynoJuftpq4ZOpxjHTT1RLVqkFMjCphbNsWXnoJxo2DkiXtv+8dO+CZ\nZ6BbN9Wbp7hkFGOy818WZjFIGFbbvHmz3iFYzYixx8fEaJF+frmGT97w89NmT5yY5+u97rlHi4+J\nKfTjR+bz+G/4+Vn8+Jaw9fE/e1bT2rfXtCZNNG3HDps+dC6HDmlajx6a5um5WVu61H77sTcjnv+W\nMDd3Wj108/XXX9O4cWPc3d3ZuXNnvtutW7eOhg0bUq9ePaZOnWrt7gwtLi5O7xCsZsTYW0VEED59\nOhPCw4kKCWFCeDjtpk/nz19/zTNxyf/PP9k4cyZbYmMZHx5OVGgo48PD2RIbC2Dy6/lNgEravt3k\nfu1509XWx79mTVi9Gl5/XVXljBmjFh23ldOnYcAANUs3MBBefDHOocsnjXj+24PVf2gFBgayatUq\nhgwZku82mZmZDBs2jE2bNlGzZk2aN29Ox44dCQgIsHa3wkW0iojIk2B/ev99k9v+dfasyRYF+/77\nX84tWZLn62lly5p8HPf0dJP7dTRubqrlwBNPwDvvqEXHmzSBfv1UJYw1C30kJsLUqWp27ksvwdGj\nqjVDVJTNwxd2YPUVfcOGDalfv36B2yQkJODv74+vry8lSpSgR48efP/999buUri4/MbQU86fN3mF\nHj9rlsmv//nnnyYfx+hj8ZaqUQNmzYJz51RN+zffQK1a8PzzqsnYkSOQnAxZWbl/7uZN2LULPvlE\n/cLw81O/KEqWVCtDTZ6skrxwIIUdIwoNDdV25DMY+PXXX2uDBg3K/nzx4sXasGHD8mwHyId8yId8\nyIcVH+YocOgmLCyM8+fP5/n6u+++S4cOHQr6UUAV85tDk8lSQghhNwUm+o0bNxbqwWvWrEliYmL2\n54mJifj4+BTqMYUQQljGJhOm8rsiDwoK4ujRo5w6dYqMjAxWrFhBx44dbbFLIYQQZrI60a9atYpa\ntWqxfft2IiIiePJWH9I///yTiFtVC8WLF2fWrFmEh4fTqFEjnn32Wam4EUKIIqZ7U7N169YxcuRI\nMjMzGTRoEGMcqN3dgAEDiI2NpVq1auzdu1fvcCyWmJhI3759+euvv3Bzc+OFF15g+K1ujUaXnp5O\nSEgI169fJyMjg06dOhEdHa13WBbLzMwkKCgIHx8ffnCEJvM5+Pr6UrFiRdzd3SlRogQJCQl6h2SR\nlJQUBg0axP79+3Fzc+OLL77goYce0jsssxw+fJgePXpkf37ixAnefvvt/N+/ltfZ2M7Nmzc1Pz8/\n7eTJk1pGRobWtGlT7cCBA3qGZJEtW7ZoO3fu1O677z69Q7FKUlKStmvXLk3TNO3q1ata/fr1Her4\nX7t2TdM0Tbtx44bWsmVLbevWrTpHZLkPP/xQe+6557QOHTroHYrFfH19tUuXLukdhtX69u2rzZ8/\nX9M0dQ6lpKToHJF1MjMzNW9vb+3MmTP5bqNrUzNHr7MPDg7G09NT7zCs5u3tzf333w9A+fLlCQgI\nyLfG3IjK3pr4lJGRQWZmJl5eXjpHZJmzZ8+yZs0aBg0a5LCVZ44a95UrV9i6dSsDBgwA1DCzh4eH\nzlFZZ9OmTfj5+VGrVq18t9E10Z87dy5XcD4+Ppw7d07HiFzXqVOn2LVrFy1bttQ7FLNlZWVx//33\nU716dR5//HEaNWqkd0gWGTVqFO+//z7FijlmE1k3NzfatGlDUFAQ8+bN0zsci5w8eZKqVavSv39/\nHnjgAQYPHkxaWpreYVll+fLlPPfccwVuo+sZZm6dvbCv1NRUunXrxvTp0ylvzfx4nRQrVozdu3dz\n9uxZtmzZ4lB9S2JiYqhWrRrNmjVz2Kvibdu2sWvXLtauXcvs2bPZunWr3iGZ7ebNm+zcuZOXX36Z\nnTt3Uq5cOaZMmaJ3WBbLyMjghx9+4JlnnilwO10TvdTZ6+/GjRt07dqV3r1707lzZ73DsYqHhwcR\nERH8/vvveoditl9++YXVq1dTp04devbsyU8//UTfvn31DssiNWrUAKBq1ap06dLFoW7G+vj44OPj\nQ/PmzQHo1q1bgc0ZjWrt2rU8+OCDVK1atcDtdE30UmevL03TGDhwII0aNWLkyJF6h2ORixcvkpKS\nAsC///7Lxo0badasmc5Rme/dd98lMTGRkydPsnz5clq3bs2iRYv0DstsaWlpXL16FYBr166xYcMG\nAgMDdY7KfN7e3tSqVYsjR44Aapy7cePGOkdluWXLltHTjPahui4TkLPOPjMzk4EDBzpUnX3Pnj2J\nj4/n0qVL1KpVi7feeov+/fvrHZbZtm3bxpIlS2jSpEl2koyOjqZdu3Y6R3Z3SUlJ9OvXj6ysLLKy\nsujTpw9PPPGE3mFZzdGGMS9cuECXLl0ANQzSq1cv2rZtq3NUlpk5cya9evUiIyMDPz8/FixYoHdI\nFrl27RqbNm0y6/6I7nX0Qggh7Msxb/cLIYQwmyR6IYRwcpLohRDCyUmiF0IIJyeJXgghnJwkeiGE\ncHL/D9ce9mWkzIEYAAAAAElFTkSuQmCC\n" | |||
|
325 | } | |||
|
326 | ], | |||
|
327 | "prompt_number": 2 | |||
|
328 | }, | |||
|
329 | { | |||
|
330 | "cell_type": "markdown", | |||
|
331 | "metadata": {}, | |||
|
332 | "source": [ | |||
|
333 | "---" | |||
|
334 | ] | |||
|
335 | }, | |||
|
336 | { | |||
|
337 | "cell_type": "markdown", | |||
|
338 | "metadata": {}, | |||
|
339 | "source": [ | |||
|
340 | "The notebook, thanks to MathJax, has great LaTeX support, so that you can type inline math $(1,\\gamma,\\ldots, \\infty)$ as well as displayed equations:\n", | |||
|
341 | "\n", | |||
|
342 | "$$\n", | |||
|
343 | "e^{i \\pi}+1=0\n", | |||
|
344 | "$$\n", | |||
|
345 | "\n", | |||
|
346 | "**(the last equation is beatiful, isn't it?... Dami\u00e1n talking... he he!)**" | |||
|
347 | ] | |||
|
348 | }, | |||
|
349 | { | |||
|
350 | "cell_type": "markdown", | |||
|
351 | "metadata": {}, | |||
|
352 | "source": [ | |||
|
353 | "---" | |||
|
354 | ] | |||
|
355 | }, | |||
|
356 | { | |||
|
357 | "cell_type": "heading", | |||
|
358 | "level": 2, | |||
|
359 | "metadata": {}, | |||
|
360 | "source": [ | |||
|
361 | "You can easily include formatted text and code with markdown" | |||
|
362 | ] | |||
|
363 | }, | |||
|
364 | { | |||
|
365 | "cell_type": "markdown", | |||
|
366 | "metadata": {}, | |||
|
367 | "source": [ | |||
|
368 | "You can *italicize*, **boldface**\n", | |||
|
369 | "\n", | |||
|
370 | "* build \n", | |||
|
371 | "* lists\n", | |||
|
372 | "\n", | |||
|
373 | "and embed code meant for illustration instead of execution in Python:\n", | |||
|
374 | "\n", | |||
|
375 | " def f(x):\n", | |||
|
376 | " \"\"\"a docstring\"\"\"\n", | |||
|
377 | " return x**2" | |||
|
378 | ] | |||
|
379 | }, | |||
|
380 | { | |||
|
381 | "cell_type": "markdown", | |||
|
382 | "metadata": {}, | |||
|
383 | "source": [ | |||
|
384 | "---" | |||
|
385 | ] | |||
|
386 | }, | |||
|
387 | { | |||
|
388 | "cell_type": "markdown", | |||
|
389 | "metadata": {}, | |||
|
390 | "source": [ | |||
|
391 | "or other languages:\n", | |||
|
392 | "\n", | |||
|
393 | " if (i=0; i<n; i++) {\n", | |||
|
394 | " printf(\"hello %d\\n\", i);\n", | |||
|
395 | " x += 4;\n", | |||
|
396 | " }" | |||
|
397 | ] | |||
|
398 | }, | |||
|
399 | { | |||
|
400 | "cell_type": "markdown", | |||
|
401 | "metadata": {}, | |||
|
402 | "source": [ | |||
|
403 | "**And you also have highlight for your code, hehe! (Dami\u00e1n talking again...)**" | |||
|
404 | ] | |||
|
405 | }, | |||
|
406 | { | |||
|
407 | "cell_type": "markdown", | |||
|
408 | "metadata": {}, | |||
|
409 | "source": [ | |||
|
410 | "---" | |||
|
411 | ] | |||
|
412 | }, | |||
|
413 | { | |||
|
414 | "cell_type": "markdown", | |||
|
415 | "metadata": {}, | |||
|
416 | "source": [ | |||
|
417 | "And since the notebook can store displayed images in the file itself, you can show images which will be embedded in your post:" | |||
|
418 | ] | |||
|
419 | }, | |||
|
420 | { | |||
|
421 | "cell_type": "markdown", | |||
|
422 | "metadata": {}, | |||
|
423 | "source": [ | |||
|
424 | "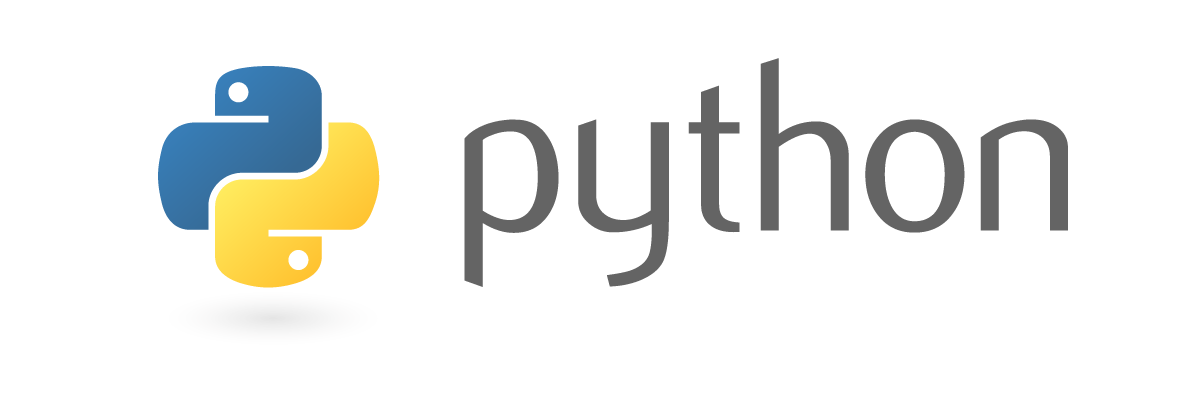" | |||
|
425 | ] | |||
|
426 | }, | |||
|
427 | { | |||
|
428 | "cell_type": "markdown", | |||
|
429 | "metadata": {}, | |||
|
430 | "source": [ | |||
|
431 | "---" | |||
|
432 | ] | |||
|
433 | }, | |||
|
434 | { | |||
|
435 | "cell_type": "heading", | |||
|
436 | "level": 1, | |||
|
437 | "metadata": {}, | |||
|
438 | "source": [ | |||
|
439 | "IPython rocks!" | |||
|
440 | ] | |||
|
441 | }, | |||
|
442 | { | |||
|
443 | "cell_type": "markdown", | |||
|
444 | "metadata": {}, | |||
|
445 | "source": [ | |||
|
446 | "Just my little contribution...\n", | |||
|
447 | "\n", | |||
|
448 | "I have a lot of work to do but this is an exciting beginning!\n", | |||
|
449 | "\n", | |||
|
450 | "You can check [vIPer](http://www.viperapp.oquanta.info)." | |||
|
451 | ] | |||
|
452 | }, | |||
|
453 | { | |||
|
454 | "cell_type": "markdown", | |||
|
455 | "metadata": {}, | |||
|
456 | "source": [ | |||
|
457 | "And you can find me at:\n", | |||
|
458 | "\n", | |||
|
459 | "* [@damian_avila](https://twitter.com/damian_avila)\n", | |||
|
460 | "* [OQUANTA](http://www.oquanta.info)\n", | |||
|
461 | "* [BLOG](http://www.damian.oquanta.info)" | |||
|
462 | ] | |||
|
463 | } | |||
|
464 | ], | |||
|
465 | "metadata": {} | |||
|
466 | } | |||
|
467 | ] | |||
|
468 | } No newline at end of file |
@@ -0,0 +1,173 b'' | |||||
|
1 | /* Default Print Stylesheet Template | |||
|
2 | by Rob Glazebrook of CSSnewbie.com | |||
|
3 | Last Updated: June 4, 2008 | |||
|
4 | ||||
|
5 | Feel free (nay, compelled) to edit, append, and | |||
|
6 | manipulate this file as you see fit. */ | |||
|
7 | ||||
|
8 | ||||
|
9 | /* SECTION 1: Set default width, margin, float, and | |||
|
10 | background. This prevents elements from extending | |||
|
11 | beyond the edge of the printed page, and prevents | |||
|
12 | unnecessary background images from printing */ | |||
|
13 | body { | |||
|
14 | background: #fff; | |||
|
15 | font-size: 13pt; | |||
|
16 | width: auto; | |||
|
17 | height: auto; | |||
|
18 | border: 0; | |||
|
19 | margin: 0 5%; | |||
|
20 | padding: 0; | |||
|
21 | float: none !important; | |||
|
22 | overflow: visible; | |||
|
23 | } | |||
|
24 | html { | |||
|
25 | background: #fff; | |||
|
26 | width: auto; | |||
|
27 | height: auto; | |||
|
28 | overflow: visible; | |||
|
29 | } | |||
|
30 | ||||
|
31 | /* SECTION 2: Remove any elements not needed in print. | |||
|
32 | This would include navigation, ads, sidebars, etc. */ | |||
|
33 | .nestedarrow, | |||
|
34 | .controls, | |||
|
35 | .reveal .progress, | |||
|
36 | .reveal.overview, | |||
|
37 | .fork-reveal, | |||
|
38 | .share-reveal, | |||
|
39 | .state-background { | |||
|
40 | display: none !important; | |||
|
41 | } | |||
|
42 | ||||
|
43 | /* SECTION 3: Set body font face, size, and color. | |||
|
44 | Consider using a serif font for readability. */ | |||
|
45 | body, p, td, li, div, a { | |||
|
46 | font-size: 16pt!important; | |||
|
47 | font-family: Georgia, "Times New Roman", Times, serif !important; | |||
|
48 | color: #000; | |||
|
49 | } | |||
|
50 | ||||
|
51 | /* SECTION 4: Set heading font face, sizes, and color. | |||
|
52 | Diffrentiate your headings from your body text. | |||
|
53 | Perhaps use a large sans-serif for distinction. */ | |||
|
54 | h1,h2,h3,h4,h5,h6 { | |||
|
55 | color: #000!important; | |||
|
56 | height: auto; | |||
|
57 | line-height: normal; | |||
|
58 | font-family: Georgia, "Times New Roman", Times, serif !important; | |||
|
59 | text-shadow: 0 0 0 #000 !important; | |||
|
60 | text-align: left; | |||
|
61 | letter-spacing: normal; | |||
|
62 | } | |||
|
63 | /* Need to reduce the size of the fonts for printing */ | |||
|
64 | h1 { font-size: 26pt !important; } | |||
|
65 | h2 { font-size: 22pt !important; } | |||
|
66 | h3 { font-size: 20pt !important; } | |||
|
67 | h4 { font-size: 20pt !important; font-variant: small-caps; } | |||
|
68 | h5 { font-size: 19pt !important; } | |||
|
69 | h6 { font-size: 18pt !important; font-style: italic; } | |||
|
70 | ||||
|
71 | /* SECTION 5: Make hyperlinks more usable. | |||
|
72 | Ensure links are underlined, and consider appending | |||
|
73 | the URL to the end of the link for usability. */ | |||
|
74 | a:link, | |||
|
75 | a:visited { | |||
|
76 | color: #000 !important; | |||
|
77 | font-weight: bold; | |||
|
78 | text-decoration: underline; | |||
|
79 | } | |||
|
80 | /* | |||
|
81 | .reveal a:link:after, | |||
|
82 | .reveal a:visited:after { | |||
|
83 | content: " (" attr(href) ") "; | |||
|
84 | color: #222 !important; | |||
|
85 | font-size: 90%; | |||
|
86 | } | |||
|
87 | */ | |||
|
88 | ||||
|
89 | ||||
|
90 | /* SECTION 6: more reveal.js specific additions by @skypanther */ | |||
|
91 | ul, ol, div, p { | |||
|
92 | visibility: visible; | |||
|
93 | position: static; | |||
|
94 | width: auto; | |||
|
95 | height: auto; | |||
|
96 | display: block; | |||
|
97 | overflow: visible; | |||
|
98 | margin: auto; | |||
|
99 | text-align: left !important; | |||
|
100 | } | |||
|
101 | .reveal .slides { | |||
|
102 | position: static; | |||
|
103 | width: auto; | |||
|
104 | height: auto; | |||
|
105 | ||||
|
106 | left: auto; | |||
|
107 | top: auto; | |||
|
108 | margin-left: auto; | |||
|
109 | margin-top: auto; | |||
|
110 | padding: auto; | |||
|
111 | ||||
|
112 | overflow: visible; | |||
|
113 | display: block; | |||
|
114 | ||||
|
115 | text-align: center; | |||
|
116 | -webkit-perspective: none; | |||
|
117 | -moz-perspective: none; | |||
|
118 | -ms-perspective: none; | |||
|
119 | perspective: none; | |||
|
120 | ||||
|
121 | -webkit-perspective-origin: 50% 50%; /* there isn't a none/auto value but 50-50 is the default */ | |||
|
122 | -moz-perspective-origin: 50% 50%; | |||
|
123 | -ms-perspective-origin: 50% 50%; | |||
|
124 | perspective-origin: 50% 50%; | |||
|
125 | } | |||
|
126 | .reveal .slides>section, | |||
|
127 | .reveal .slides>section>section { | |||
|
128 | ||||
|
129 | visibility: visible !important; | |||
|
130 | position: static !important; | |||
|
131 | width: 90% !important; | |||
|
132 | height: auto !important; | |||
|
133 | display: block !important; | |||
|
134 | overflow: visible !important; | |||
|
135 | ||||
|
136 | left: 0% !important; | |||
|
137 | top: 0% !important; | |||
|
138 | margin-left: 0px !important; | |||
|
139 | margin-top: 0px !important; | |||
|
140 | padding: 20px 0px !important; | |||
|
141 | ||||
|
142 | opacity: 1 !important; | |||
|
143 | ||||
|
144 | -webkit-transform-style: flat !important; | |||
|
145 | -moz-transform-style: flat !important; | |||
|
146 | -ms-transform-style: flat !important; | |||
|
147 | transform-style: flat !important; | |||
|
148 | ||||
|
149 | -webkit-transform: none !important; | |||
|
150 | -moz-transform: none !important; | |||
|
151 | -ms-transform: none !important; | |||
|
152 | transform: none !important; | |||
|
153 | } | |||
|
154 | .reveal section { | |||
|
155 | page-break-after: always !important; | |||
|
156 | display: block !important; | |||
|
157 | } | |||
|
158 | .reveal section.stack { | |||
|
159 | page-break-after: avoid !important; | |||
|
160 | } | |||
|
161 | .reveal section .fragment { | |||
|
162 | opacity: 1 !important; | |||
|
163 | } | |||
|
164 | .reveal section:last-of-type { | |||
|
165 | page-break-after: avoid !important; | |||
|
166 | } | |||
|
167 | .reveal section img { | |||
|
168 | display: block; | |||
|
169 | margin: 15px 0px; | |||
|
170 | background: rgba(255,255,255,1); | |||
|
171 | border: 1px solid #666; | |||
|
172 | box-shadow: none; | |||
|
173 | } No newline at end of file |
@@ -0,0 +1,159 b'' | |||||
|
1 | /* Default Print Stylesheet Template | |||
|
2 | by Rob Glazebrook of CSSnewbie.com | |||
|
3 | Last Updated: June 4, 2008 | |||
|
4 | ||||
|
5 | Feel free (nay, compelled) to edit, append, and | |||
|
6 | manipulate this file as you see fit. */ | |||
|
7 | ||||
|
8 | ||||
|
9 | /* SECTION 1: Set default width, margin, float, and | |||
|
10 | background. This prevents elements from extending | |||
|
11 | beyond the edge of the printed page, and prevents | |||
|
12 | unnecessary background images from printing */ | |||
|
13 | * { | |||
|
14 | -webkit-print-color-adjust: exact; | |||
|
15 | } | |||
|
16 | ||||
|
17 | body { | |||
|
18 | font-size: 18pt; | |||
|
19 | width: auto; | |||
|
20 | height: auto; | |||
|
21 | border: 0; | |||
|
22 | margin: 0 5%; | |||
|
23 | padding: 0; | |||
|
24 | float: none !important; | |||
|
25 | overflow: visible; | |||
|
26 | background-image: none; | |||
|
27 | } | |||
|
28 | ||||
|
29 | html { | |||
|
30 | width: auto; | |||
|
31 | height: auto; | |||
|
32 | overflow: visible; | |||
|
33 | } | |||
|
34 | ||||
|
35 | /* SECTION 2: Remove any elements not needed in print. | |||
|
36 | This would include navigation, ads, sidebars, etc. */ | |||
|
37 | .nestedarrow, | |||
|
38 | .controls, | |||
|
39 | .reveal .progress, | |||
|
40 | .reveal.overview, | |||
|
41 | .fork-reveal, | |||
|
42 | .share-reveal, | |||
|
43 | .state-background { | |||
|
44 | display: none !important; | |||
|
45 | } | |||
|
46 | ||||
|
47 | /* SECTION 3: Set body font face, size, and color. | |||
|
48 | Consider using a serif font for readability. */ | |||
|
49 | body, p, td, li, div { | |||
|
50 | font-size: 18pt; | |||
|
51 | } | |||
|
52 | ||||
|
53 | /* SECTION 4: Set heading font face, sizes, and color. | |||
|
54 | Diffrentiate your headings from your body text. | |||
|
55 | Perhaps use a large sans-serif for distinction. */ | |||
|
56 | h1,h2,h3,h4,h5,h6 { | |||
|
57 | text-shadow: 0 0 0 #000 !important; | |||
|
58 | } | |||
|
59 | ||||
|
60 | /* SECTION 5: Make hyperlinks more usable. | |||
|
61 | Ensure links are underlined, and consider appending | |||
|
62 | the URL to the end of the link for usability. */ | |||
|
63 | a:link, | |||
|
64 | a:visited { | |||
|
65 | font-weight: bold; | |||
|
66 | text-decoration: underline; | |||
|
67 | } | |||
|
68 | ||||
|
69 | ||||
|
70 | /* SECTION 6: more reveal.js specific additions by @skypanther */ | |||
|
71 | ul, ol, div, p { | |||
|
72 | visibility: visible; | |||
|
73 | position: static; | |||
|
74 | width: auto; | |||
|
75 | height: auto; | |||
|
76 | display: block; | |||
|
77 | overflow: visible; | |||
|
78 | margin: auto; | |||
|
79 | } | |||
|
80 | .reveal .slides { | |||
|
81 | position: static; | |||
|
82 | width: 100%; | |||
|
83 | height: auto; | |||
|
84 | ||||
|
85 | left: auto; | |||
|
86 | top: auto; | |||
|
87 | margin-left: auto; | |||
|
88 | margin-top: auto; | |||
|
89 | padding: auto; | |||
|
90 | ||||
|
91 | overflow: visible; | |||
|
92 | display: block; | |||
|
93 | ||||
|
94 | text-align: center; | |||
|
95 | -webkit-perspective: none; | |||
|
96 | -moz-perspective: none; | |||
|
97 | -ms-perspective: none; | |||
|
98 | perspective: none; | |||
|
99 | ||||
|
100 | -webkit-perspective-origin: 50% 50%; /* there isn't a none/auto value but 50-50 is the default */ | |||
|
101 | -moz-perspective-origin: 50% 50%; | |||
|
102 | -ms-perspective-origin: 50% 50%; | |||
|
103 | perspective-origin: 50% 50%; | |||
|
104 | } | |||
|
105 | .reveal .slides>section, .reveal .slides>section>section { | |||
|
106 | ||||
|
107 | visibility: visible !important; | |||
|
108 | position: static !important; | |||
|
109 | width: 100% !important; | |||
|
110 | height: auto !important; | |||
|
111 | min-height: initial !important; | |||
|
112 | display: block !important; | |||
|
113 | overflow: visible !important; | |||
|
114 | ||||
|
115 | left: 0% !important; | |||
|
116 | top: 0% !important; | |||
|
117 | margin-left: 0px !important; | |||
|
118 | margin-top: 50px !important; | |||
|
119 | padding: 20px 0px !important; | |||
|
120 | ||||
|
121 | opacity: 1 !important; | |||
|
122 | ||||
|
123 | -webkit-transform-style: flat !important; | |||
|
124 | -moz-transform-style: flat !important; | |||
|
125 | -ms-transform-style: flat !important; | |||
|
126 | transform-style: flat !important; | |||
|
127 | ||||
|
128 | -webkit-transform: none !important; | |||
|
129 | -moz-transform: none !important; | |||
|
130 | -ms-transform: none !important; | |||
|
131 | transform: none !important; | |||
|
132 | } | |||
|
133 | .reveal section { | |||
|
134 | page-break-after: always !important; | |||
|
135 | display: block !important; | |||
|
136 | } | |||
|
137 | .reveal section.stack { | |||
|
138 | margin: 0px !important; | |||
|
139 | padding: 0px !important; | |||
|
140 | page-break-after: avoid !important; | |||
|
141 | } | |||
|
142 | .reveal section .fragment { | |||
|
143 | opacity: 1 !important; | |||
|
144 | } | |||
|
145 | .reveal img { | |||
|
146 | box-shadow: none; | |||
|
147 | } | |||
|
148 | .reveal .roll { | |||
|
149 | overflow: visible; | |||
|
150 | line-height: 1em; | |||
|
151 | } | |||
|
152 | ||||
|
153 | .reveal small a { | |||
|
154 | font-size: 16pt !important; | |||
|
155 | } | |||
|
156 | ||||
|
157 | pre { | |||
|
158 | font-size: 9pt !important; | |||
|
159 | } |
This diff has been collapsed as it changes many lines, (1184 lines changed) Show them Hide them | |||||
@@ -0,0 +1,1184 b'' | |||||
|
1 | @charset "UTF-8"; | |||
|
2 | ||||
|
3 | /*! | |||
|
4 | * reveal.js | |||
|
5 | * http://lab.hakim.se/reveal-js | |||
|
6 | * MIT licensed | |||
|
7 | * | |||
|
8 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
9 | */ | |||
|
10 | ||||
|
11 | ||||
|
12 | /********************************************* | |||
|
13 | * RESET STYLES | |||
|
14 | *********************************************/ | |||
|
15 | ||||
|
16 | html, body, .reveal div, .reveal span, .reveal applet, .reveal object, .reveal iframe, | |||
|
17 | .reveal h1, .reveal h2, .reveal h3, .reveal h4, .reveal h5, .reveal h6, .reveal p, .reveal blockquote, .reveal pre, | |||
|
18 | .reveal a, .reveal abbr, .reveal acronym, .reveal address, .reveal big, .reveal cite, .reveal code, | |||
|
19 | .reveal del, .reveal dfn, .reveal em, .reveal img, .reveal ins, .reveal kbd, .reveal q, .reveal s, .reveal samp, | |||
|
20 | .reveal small, .reveal strike, .reveal strong, .reveal sub, .reveal sup, .reveal tt, .reveal var, | |||
|
21 | .reveal b, .reveal u, .reveal i, .reveal center, | |||
|
22 | .reveal dl, .reveal dt, .reveal dd, .reveal ol, .reveal ul, .reveal li, | |||
|
23 | .reveal fieldset, .reveal form, .reveal label, .reveal legend, | |||
|
24 | .reveal table, .reveal caption, .reveal tbody, .reveal tfoot, .reveal thead, .reveal tr, .reveal th, .reveal td, | |||
|
25 | .reveal article, .reveal aside, .reveal canvas, .reveal details, .reveal embed, | |||
|
26 | .reveal figure, .reveal figcaption, .reveal footer, .reveal header, .reveal hgroup, | |||
|
27 | .reveal menu, .reveal nav, .reveal output, .reveal ruby, .reveal section, .reveal summary, | |||
|
28 | .reveal time, .reveal mark, .reveal audio, video { | |||
|
29 | margin: 0; | |||
|
30 | padding: 0; | |||
|
31 | border: 0; | |||
|
32 | font-size: 100%; | |||
|
33 | font: inherit; | |||
|
34 | vertical-align: baseline; | |||
|
35 | } | |||
|
36 | ||||
|
37 | .reveal article, .reveal aside, .reveal details, .reveal figcaption, .reveal figure, | |||
|
38 | .reveal footer, .reveal header, .reveal hgroup, .reveal menu, .reveal nav, .reveal section { | |||
|
39 | display: block; | |||
|
40 | } | |||
|
41 | ||||
|
42 | ||||
|
43 | /********************************************* | |||
|
44 | * GLOBAL STYLES | |||
|
45 | *********************************************/ | |||
|
46 | ||||
|
47 | html, | |||
|
48 | body { | |||
|
49 | width: 100%; | |||
|
50 | height: 100%; | |||
|
51 | min-height: 600px; | |||
|
52 | overflow: hidden; | |||
|
53 | } | |||
|
54 | ||||
|
55 | body { | |||
|
56 | position: relative; | |||
|
57 | line-height: 1; | |||
|
58 | } | |||
|
59 | ||||
|
60 | ::selection { | |||
|
61 | background:#FF5E99; | |||
|
62 | color:#fff; | |||
|
63 | text-shadow: none; | |||
|
64 | } | |||
|
65 | ||||
|
66 | @media screen and (max-width: 900px) { | |||
|
67 | .reveal { | |||
|
68 | font-size: 30px; | |||
|
69 | } | |||
|
70 | } | |||
|
71 | ||||
|
72 | /********************************************* | |||
|
73 | * HEADERS | |||
|
74 | *********************************************/ | |||
|
75 | ||||
|
76 | .reveal h1 { font-size: 3.77em; } | |||
|
77 | .reveal h2 { font-size: 2.11em; } | |||
|
78 | .reveal h3 { font-size: 1.55em; } | |||
|
79 | .reveal h4 { font-size: 1em; } | |||
|
80 | ||||
|
81 | ||||
|
82 | /********************************************* | |||
|
83 | * VIEW FRAGMENTS | |||
|
84 | *********************************************/ | |||
|
85 | ||||
|
86 | .reveal .slides section .fragment { | |||
|
87 | opacity: 0; | |||
|
88 | ||||
|
89 | -webkit-transition: all .2s ease; | |||
|
90 | -moz-transition: all .2s ease; | |||
|
91 | -ms-transition: all .2s ease; | |||
|
92 | -o-transition: all .2s ease; | |||
|
93 | transition: all .2s ease; | |||
|
94 | } | |||
|
95 | .reveal .slides section .fragment.visible { | |||
|
96 | opacity: 1; | |||
|
97 | } | |||
|
98 | ||||
|
99 | .reveal .slides section .fragment.grow { | |||
|
100 | opacity: 1; | |||
|
101 | } | |||
|
102 | .reveal .slides section .fragment.grow.visible { | |||
|
103 | -webkit-transform: scale( 1.3 ); | |||
|
104 | -moz-transform: scale( 1.3 ); | |||
|
105 | -ms-transform: scale( 1.3 ); | |||
|
106 | -o-transform: scale( 1.3 ); | |||
|
107 | transform: scale( 1.3 ); | |||
|
108 | } | |||
|
109 | ||||
|
110 | .reveal .slides section .fragment.shrink { | |||
|
111 | opacity: 1; | |||
|
112 | } | |||
|
113 | .reveal .slides section .fragment.shrink.visible { | |||
|
114 | -webkit-transform: scale( 0.7 ); | |||
|
115 | -moz-transform: scale( 0.7 ); | |||
|
116 | -ms-transform: scale( 0.7 ); | |||
|
117 | -o-transform: scale( 0.7 ); | |||
|
118 | transform: scale( 0.7 ); | |||
|
119 | } | |||
|
120 | ||||
|
121 | .reveal .slides section .fragment.roll-in { | |||
|
122 | opacity: 0; | |||
|
123 | ||||
|
124 | -webkit-transform: rotateX( 90deg ); | |||
|
125 | -moz-transform: rotateX( 90deg ); | |||
|
126 | -ms-transform: rotateX( 90deg ); | |||
|
127 | -o-transform: rotateX( 90deg ); | |||
|
128 | transform: rotateX( 90deg ); | |||
|
129 | } | |||
|
130 | .reveal .slides section .fragment.roll-in.visible { | |||
|
131 | opacity: 1; | |||
|
132 | ||||
|
133 | -webkit-transform: rotateX( 0 ); | |||
|
134 | -moz-transform: rotateX( 0 ); | |||
|
135 | -ms-transform: rotateX( 0 ); | |||
|
136 | -o-transform: rotateX( 0 ); | |||
|
137 | transform: rotateX( 0 ); | |||
|
138 | } | |||
|
139 | ||||
|
140 | .reveal .slides section .fragment.fade-out { | |||
|
141 | opacity: 1; | |||
|
142 | } | |||
|
143 | .reveal .slides section .fragment.fade-out.visible { | |||
|
144 | opacity: 0; | |||
|
145 | } | |||
|
146 | ||||
|
147 | .reveal .slides section .fragment.highlight-red, | |||
|
148 | .reveal .slides section .fragment.highlight-green, | |||
|
149 | .reveal .slides section .fragment.highlight-blue { | |||
|
150 | opacity: 1; | |||
|
151 | } | |||
|
152 | .reveal .slides section .fragment.highlight-red.visible { | |||
|
153 | color: #ff2c2d | |||
|
154 | } | |||
|
155 | .reveal .slides section .fragment.highlight-green.visible { | |||
|
156 | color: #17ff2e; | |||
|
157 | } | |||
|
158 | .reveal .slides section .fragment.highlight-blue.visible { | |||
|
159 | color: #1b91ff; | |||
|
160 | } | |||
|
161 | ||||
|
162 | ||||
|
163 | /********************************************* | |||
|
164 | * DEFAULT ELEMENT STYLES | |||
|
165 | *********************************************/ | |||
|
166 | ||||
|
167 | .reveal .slides section { | |||
|
168 | line-height: 1.2em; | |||
|
169 | font-weight: normal; | |||
|
170 | } | |||
|
171 | ||||
|
172 | .reveal img { | |||
|
173 | /* preserve aspect ratio and scale image so it's bound within the section */ | |||
|
174 | max-width: 100%; | |||
|
175 | max-height: 100%; | |||
|
176 | } | |||
|
177 | ||||
|
178 | .reveal strong, | |||
|
179 | .reveal b { | |||
|
180 | font-weight: bold; | |||
|
181 | } | |||
|
182 | ||||
|
183 | .reveal em, | |||
|
184 | .reveal i { | |||
|
185 | font-style: italic; | |||
|
186 | } | |||
|
187 | ||||
|
188 | .reveal ol, | |||
|
189 | .reveal ul { | |||
|
190 | display: inline-block; | |||
|
191 | ||||
|
192 | text-align: left; | |||
|
193 | margin: 0 0 0 1em; | |||
|
194 | } | |||
|
195 | ||||
|
196 | .reveal ol { | |||
|
197 | list-style-type: decimal; | |||
|
198 | } | |||
|
199 | ||||
|
200 | .reveal ul { | |||
|
201 | list-style-type: disc; | |||
|
202 | } | |||
|
203 | ||||
|
204 | .reveal ul ul { | |||
|
205 | list-style-type: square; | |||
|
206 | } | |||
|
207 | ||||
|
208 | .reveal ul ul ul { | |||
|
209 | list-style-type: circle; | |||
|
210 | } | |||
|
211 | ||||
|
212 | .reveal ul ul, | |||
|
213 | .reveal ul ol, | |||
|
214 | .reveal ol ol, | |||
|
215 | .reveal ol ul { | |||
|
216 | display: block; | |||
|
217 | margin-left: 40px; | |||
|
218 | } | |||
|
219 | ||||
|
220 | .reveal p { | |||
|
221 | margin-bottom: 10px; | |||
|
222 | line-height: 1.2em; | |||
|
223 | } | |||
|
224 | ||||
|
225 | .reveal q, | |||
|
226 | .reveal blockquote { | |||
|
227 | quotes: none; | |||
|
228 | } | |||
|
229 | ||||
|
230 | .reveal blockquote { | |||
|
231 | display: block; | |||
|
232 | position: relative; | |||
|
233 | width: 70%; | |||
|
234 | margin: 5px auto; | |||
|
235 | padding: 5px; | |||
|
236 | ||||
|
237 | font-style: italic; | |||
|
238 | background: rgba(255, 255, 255, 0.05); | |||
|
239 | box-shadow: 0px 0px 2px rgba(0,0,0,0.2); | |||
|
240 | } | |||
|
241 | .reveal blockquote:before { | |||
|
242 | content: '\201C'; | |||
|
243 | } | |||
|
244 | .reveal blockquote:after { | |||
|
245 | content: '\201D'; | |||
|
246 | } | |||
|
247 | ||||
|
248 | .reveal q { | |||
|
249 | font-style: italic; | |||
|
250 | } | |||
|
251 | .reveal q:before { | |||
|
252 | content: '\201C'; | |||
|
253 | } | |||
|
254 | .reveal q:after { | |||
|
255 | content: '\201D'; | |||
|
256 | } | |||
|
257 | ||||
|
258 | .reveal pre { | |||
|
259 | display: block; | |||
|
260 | position: relative; | |||
|
261 | width: 90%; | |||
|
262 | margin: 15px auto; | |||
|
263 | ||||
|
264 | text-align: left; | |||
|
265 | font-size: 0.55em; | |||
|
266 | font-family: monospace; | |||
|
267 | line-height: 1.2em; | |||
|
268 | ||||
|
269 | word-wrap: break-word; | |||
|
270 | ||||
|
271 | box-shadow: 0px 0px 6px rgba(0,0,0,0.3); | |||
|
272 | } | |||
|
273 | .reveal pre code { | |||
|
274 | padding: 5px; | |||
|
275 | } | |||
|
276 | ||||
|
277 | .reveal code { | |||
|
278 | font-family: monospace; | |||
|
279 | overflow: auto; | |||
|
280 | max-height: 400px; | |||
|
281 | } | |||
|
282 | ||||
|
283 | .reveal table th, | |||
|
284 | .reveal table td { | |||
|
285 | text-align: left; | |||
|
286 | padding-right: .3em; | |||
|
287 | } | |||
|
288 | ||||
|
289 | .reveal table th { | |||
|
290 | text-shadow: rgb(255,255,255) 1px 1px 2px; | |||
|
291 | } | |||
|
292 | ||||
|
293 | .reveal sup { | |||
|
294 | vertical-align: super; | |||
|
295 | } | |||
|
296 | .reveal sub { | |||
|
297 | vertical-align: sub; | |||
|
298 | } | |||
|
299 | ||||
|
300 | .reveal small { | |||
|
301 | display: inline-block; | |||
|
302 | font-size: 0.6em; | |||
|
303 | line-height: 1.2em; | |||
|
304 | vertical-align: top; | |||
|
305 | } | |||
|
306 | ||||
|
307 | .reveal small * { | |||
|
308 | vertical-align: top; | |||
|
309 | } | |||
|
310 | ||||
|
311 | ||||
|
312 | /********************************************* | |||
|
313 | * CONTROLS | |||
|
314 | *********************************************/ | |||
|
315 | ||||
|
316 | .reveal .controls { | |||
|
317 | display: none; | |||
|
318 | position: fixed; | |||
|
319 | width: 110px; | |||
|
320 | height: 110px; | |||
|
321 | z-index: 30; | |||
|
322 | right: 10px; | |||
|
323 | bottom: 10px; | |||
|
324 | } | |||
|
325 | ||||
|
326 | .reveal .controls div { | |||
|
327 | position: absolute; | |||
|
328 | opacity: 0.1; | |||
|
329 | width: 0; | |||
|
330 | height: 0; | |||
|
331 | border: 12px solid transparent; | |||
|
332 | ||||
|
333 | -webkit-transition: opacity 0.2s ease; | |||
|
334 | -moz-transition: opacity 0.2s ease; | |||
|
335 | -ms-transition: opacity 0.2s ease; | |||
|
336 | -o-transition: opacity 0.2s ease; | |||
|
337 | transition: opacity 0.2s ease; | |||
|
338 | } | |||
|
339 | ||||
|
340 | .reveal .controls div.enabled { | |||
|
341 | opacity: 0.6; | |||
|
342 | cursor: pointer; | |||
|
343 | } | |||
|
344 | ||||
|
345 | .reveal .controls div.enabled:active { | |||
|
346 | margin-top: 1px; | |||
|
347 | } | |||
|
348 | ||||
|
349 | .reveal .controls div.left { | |||
|
350 | top: 42px; | |||
|
351 | ||||
|
352 | border-right-width: 22px; | |||
|
353 | border-right-color: #eee; | |||
|
354 | } | |||
|
355 | ||||
|
356 | .reveal .controls div.right { | |||
|
357 | left: 74px; | |||
|
358 | top: 42px; | |||
|
359 | ||||
|
360 | border-left-width: 22px; | |||
|
361 | border-left-color: #eee; | |||
|
362 | } | |||
|
363 | ||||
|
364 | .reveal .controls div.up { | |||
|
365 | left: 42px; | |||
|
366 | ||||
|
367 | border-bottom-width: 22px; | |||
|
368 | border-bottom-color: #eee; | |||
|
369 | } | |||
|
370 | ||||
|
371 | .reveal .controls div.down { | |||
|
372 | left: 42px; | |||
|
373 | top: 74px; | |||
|
374 | ||||
|
375 | border-top-width: 22px; | |||
|
376 | border-top-color: #eee; | |||
|
377 | } | |||
|
378 | ||||
|
379 | ||||
|
380 | /********************************************* | |||
|
381 | * PROGRESS BAR | |||
|
382 | *********************************************/ | |||
|
383 | ||||
|
384 | .reveal .progress { | |||
|
385 | position: fixed; | |||
|
386 | display: none; | |||
|
387 | height: 3px; | |||
|
388 | width: 100%; | |||
|
389 | bottom: 0; | |||
|
390 | left: 0; | |||
|
391 | z-index: 10; | |||
|
392 | } | |||
|
393 | .reveal .progress:after { | |||
|
394 | content: ''; | |||
|
395 | display: 'block'; | |||
|
396 | position: absolute; | |||
|
397 | height: 20px; | |||
|
398 | width: 100%; | |||
|
399 | top: -20px; | |||
|
400 | } | |||
|
401 | .reveal .progress span { | |||
|
402 | display: block; | |||
|
403 | height: 100%; | |||
|
404 | width: 0px; | |||
|
405 | ||||
|
406 | -webkit-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
407 | -moz-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
408 | -ms-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
409 | -o-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
410 | transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
411 | } | |||
|
412 | ||||
|
413 | ||||
|
414 | /********************************************* | |||
|
415 | * ROLLING LINKS | |||
|
416 | *********************************************/ | |||
|
417 | ||||
|
418 | .reveal .roll { | |||
|
419 | display: inline-block; | |||
|
420 | line-height: 1.2; | |||
|
421 | overflow: hidden; | |||
|
422 | ||||
|
423 | vertical-align: top; | |||
|
424 | ||||
|
425 | -webkit-perspective: 400px; | |||
|
426 | -moz-perspective: 400px; | |||
|
427 | -ms-perspective: 400px; | |||
|
428 | perspective: 400px; | |||
|
429 | ||||
|
430 | -webkit-perspective-origin: 50% 50%; | |||
|
431 | -moz-perspective-origin: 50% 50%; | |||
|
432 | -ms-perspective-origin: 50% 50%; | |||
|
433 | perspective-origin: 50% 50%; | |||
|
434 | } | |||
|
435 | .reveal .roll:hover { | |||
|
436 | background: none; | |||
|
437 | text-shadow: none; | |||
|
438 | } | |||
|
439 | .reveal .roll span { | |||
|
440 | display: block; | |||
|
441 | position: relative; | |||
|
442 | padding: 0 2px; | |||
|
443 | ||||
|
444 | pointer-events: none; | |||
|
445 | ||||
|
446 | -webkit-transition: all 400ms ease; | |||
|
447 | -moz-transition: all 400ms ease; | |||
|
448 | -ms-transition: all 400ms ease; | |||
|
449 | transition: all 400ms ease; | |||
|
450 | ||||
|
451 | -webkit-transform-origin: 50% 0%; | |||
|
452 | -moz-transform-origin: 50% 0%; | |||
|
453 | -ms-transform-origin: 50% 0%; | |||
|
454 | transform-origin: 50% 0%; | |||
|
455 | ||||
|
456 | -webkit-transform-style: preserve-3d; | |||
|
457 | -moz-transform-style: preserve-3d; | |||
|
458 | -ms-transform-style: preserve-3d; | |||
|
459 | transform-style: preserve-3d; | |||
|
460 | ||||
|
461 | -webkit-backface-visibility: hidden; | |||
|
462 | -moz-backface-visibility: hidden; | |||
|
463 | backface-visibility: hidden; | |||
|
464 | } | |||
|
465 | .reveal .roll:hover span { | |||
|
466 | background: rgba(0,0,0,0.5); | |||
|
467 | ||||
|
468 | -webkit-transform: translate3d( 0px, 0px, -45px ) rotateX( 90deg ); | |||
|
469 | -moz-transform: translate3d( 0px, 0px, -45px ) rotateX( 90deg ); | |||
|
470 | -ms-transform: translate3d( 0px, 0px, -45px ) rotateX( 90deg ); | |||
|
471 | transform: translate3d( 0px, 0px, -45px ) rotateX( 90deg ); | |||
|
472 | } | |||
|
473 | .reveal .roll span:after { | |||
|
474 | content: attr(data-title); | |||
|
475 | ||||
|
476 | display: block; | |||
|
477 | position: absolute; | |||
|
478 | left: 0; | |||
|
479 | top: 0; | |||
|
480 | padding: 0 2px; | |||
|
481 | ||||
|
482 | -webkit-transform-origin: 50% 0%; | |||
|
483 | -moz-transform-origin: 50% 0%; | |||
|
484 | -ms-transform-origin: 50% 0%; | |||
|
485 | transform-origin: 50% 0%; | |||
|
486 | ||||
|
487 | -webkit-transform: translate3d( 0px, 110%, 0px ) rotateX( -90deg ); | |||
|
488 | -moz-transform: translate3d( 0px, 110%, 0px ) rotateX( -90deg ); | |||
|
489 | -ms-transform: translate3d( 0px, 110%, 0px ) rotateX( -90deg ); | |||
|
490 | transform: translate3d( 0px, 110%, 0px ) rotateX( -90deg ); | |||
|
491 | } | |||
|
492 | ||||
|
493 | ||||
|
494 | /********************************************* | |||
|
495 | * SLIDES | |||
|
496 | *********************************************/ | |||
|
497 | ||||
|
498 | .reveal .slides { | |||
|
499 | position: absolute; | |||
|
500 | max-width: 900px; | |||
|
501 | width: 80%; | |||
|
502 | height: 60%; | |||
|
503 | left: 50%; | |||
|
504 | top: 50%; | |||
|
505 | margin-top: -320px; | |||
|
506 | padding: 20px 0px; | |||
|
507 | overflow: visible; | |||
|
508 | z-index: 1; | |||
|
509 | ||||
|
510 | text-align: center; | |||
|
511 | ||||
|
512 | -webkit-transition: -webkit-perspective .4s ease; | |||
|
513 | -moz-transition: -moz-perspective .4s ease; | |||
|
514 | -ms-transition: -ms-perspective .4s ease; | |||
|
515 | -o-transition: -o-perspective .4s ease; | |||
|
516 | transition: perspective .4s ease; | |||
|
517 | ||||
|
518 | -webkit-perspective: 600px; | |||
|
519 | -moz-perspective: 600px; | |||
|
520 | -ms-perspective: 600px; | |||
|
521 | perspective: 600px; | |||
|
522 | ||||
|
523 | -webkit-perspective-origin: 0% 25%; | |||
|
524 | -moz-perspective-origin: 0% 25%; | |||
|
525 | -ms-perspective-origin: 0% 25%; | |||
|
526 | perspective-origin: 0% 25%; | |||
|
527 | } | |||
|
528 | ||||
|
529 | .reveal .slides>section, | |||
|
530 | .reveal .slides>section>section { | |||
|
531 | display: none; | |||
|
532 | position: absolute; | |||
|
533 | width: 100%; | |||
|
534 | min-height: 600px; | |||
|
535 | ||||
|
536 | z-index: 10; | |||
|
537 | ||||
|
538 | -webkit-transform-style: preserve-3d; | |||
|
539 | -moz-transform-style: preserve-3d; | |||
|
540 | -ms-transform-style: preserve-3d; | |||
|
541 | transform-style: preserve-3d; | |||
|
542 | ||||
|
543 | -webkit-transition: all 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
544 | -moz-transition: all 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
545 | -ms-transition: all 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
546 | -o-transition: all 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
547 | transition: all 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
548 | } | |||
|
549 | ||||
|
550 | .reveal .slides>section.present { | |||
|
551 | display: block; | |||
|
552 | z-index: 11; | |||
|
553 | opacity: 1; | |||
|
554 | } | |||
|
555 | ||||
|
556 | .reveal .slides>section { | |||
|
557 | margin-left: -50%; | |||
|
558 | } | |||
|
559 | ||||
|
560 | ||||
|
561 | /********************************************* | |||
|
562 | * DEFAULT TRANSITION | |||
|
563 | *********************************************/ | |||
|
564 | ||||
|
565 | .reveal .slides>section.past { | |||
|
566 | display: block; | |||
|
567 | opacity: 0; | |||
|
568 | ||||
|
569 | -webkit-transform: translate3d(-100%, 0, 0) rotateY(-90deg) translate3d(-100%, 0, 0); | |||
|
570 | -moz-transform: translate3d(-100%, 0, 0) rotateY(-90deg) translate3d(-100%, 0, 0); | |||
|
571 | -ms-transform: translate3d(-100%, 0, 0) rotateY(-90deg) translate3d(-100%, 0, 0); | |||
|
572 | transform: translate3d(-100%, 0, 0) rotateY(-90deg) translate3d(-100%, 0, 0); | |||
|
573 | } | |||
|
574 | .reveal .slides>section.future { | |||
|
575 | display: block; | |||
|
576 | opacity: 0; | |||
|
577 | ||||
|
578 | -webkit-transform: translate3d(100%, 0, 0) rotateY(90deg) translate3d(100%, 0, 0); | |||
|
579 | -moz-transform: translate3d(100%, 0, 0) rotateY(90deg) translate3d(100%, 0, 0); | |||
|
580 | -ms-transform: translate3d(100%, 0, 0) rotateY(90deg) translate3d(100%, 0, 0); | |||
|
581 | transform: translate3d(100%, 0, 0) rotateY(90deg) translate3d(100%, 0, 0); | |||
|
582 | } | |||
|
583 | ||||
|
584 | .reveal .slides>section>section.past { | |||
|
585 | display: block; | |||
|
586 | opacity: 0; | |||
|
587 | ||||
|
588 | -webkit-transform: translate3d(0, -50%, 0) rotateX(70deg) translate3d(0, -50%, 0); | |||
|
589 | -moz-transform: translate3d(0, -50%, 0) rotateX(70deg) translate3d(0, -50%, 0); | |||
|
590 | -ms-transform: translate3d(0, -50%, 0) rotateX(70deg) translate3d(0, -50%, 0); | |||
|
591 | transform: translate3d(0, -50%, 0) rotateX(70deg) translate3d(0, -50%, 0); | |||
|
592 | } | |||
|
593 | .reveal .slides>section>section.future { | |||
|
594 | display: block; | |||
|
595 | opacity: 0; | |||
|
596 | ||||
|
597 | -webkit-transform: translate3d(0, 50%, 0) rotateX(-70deg) translate3d(0, 50%, 0); | |||
|
598 | -moz-transform: translate3d(0, 50%, 0) rotateX(-70deg) translate3d(0, 50%, 0); | |||
|
599 | -ms-transform: translate3d(0, 50%, 0) rotateX(-70deg) translate3d(0, 50%, 0); | |||
|
600 | transform: translate3d(0, 50%, 0) rotateX(-70deg) translate3d(0, 50%, 0); | |||
|
601 | } | |||
|
602 | ||||
|
603 | ||||
|
604 | /********************************************* | |||
|
605 | * CONCAVE TRANSITION | |||
|
606 | *********************************************/ | |||
|
607 | ||||
|
608 | .reveal.concave .slides>section.past { | |||
|
609 | -webkit-transform: translate3d(-100%, 0, 0) rotateY(90deg) translate3d(-100%, 0, 0); | |||
|
610 | -moz-transform: translate3d(-100%, 0, 0) rotateY(90deg) translate3d(-100%, 0, 0); | |||
|
611 | -ms-transform: translate3d(-100%, 0, 0) rotateY(90deg) translate3d(-100%, 0, 0); | |||
|
612 | transform: translate3d(-100%, 0, 0) rotateY(90deg) translate3d(-100%, 0, 0); | |||
|
613 | } | |||
|
614 | .reveal.concave .slides>section.future { | |||
|
615 | -webkit-transform: translate3d(100%, 0, 0) rotateY(-90deg) translate3d(100%, 0, 0); | |||
|
616 | -moz-transform: translate3d(100%, 0, 0) rotateY(-90deg) translate3d(100%, 0, 0); | |||
|
617 | -ms-transform: translate3d(100%, 0, 0) rotateY(-90deg) translate3d(100%, 0, 0); | |||
|
618 | transform: translate3d(100%, 0, 0) rotateY(-90deg) translate3d(100%, 0, 0); | |||
|
619 | } | |||
|
620 | ||||
|
621 | .reveal.concave .slides>section>section.past { | |||
|
622 | -webkit-transform: translate3d(0, -80%, 0) rotateX(-70deg) translate3d(0, -80%, 0); | |||
|
623 | -moz-transform: translate3d(0, -80%, 0) rotateX(-70deg) translate3d(0, -80%, 0); | |||
|
624 | -ms-transform: translate3d(0, -80%, 0) rotateX(-70deg) translate3d(0, -80%, 0); | |||
|
625 | transform: translate3d(0, -80%, 0) rotateX(-70deg) translate3d(0, -80%, 0); | |||
|
626 | } | |||
|
627 | .reveal.concave .slides>section>section.future { | |||
|
628 | -webkit-transform: translate3d(0, 80%, 0) rotateX(70deg) translate3d(0, 80%, 0); | |||
|
629 | -moz-transform: translate3d(0, 80%, 0) rotateX(70deg) translate3d(0, 80%, 0); | |||
|
630 | -ms-transform: translate3d(0, 80%, 0) rotateX(70deg) translate3d(0, 80%, 0); | |||
|
631 | transform: translate3d(0, 80%, 0) rotateX(70deg) translate3d(0, 80%, 0); | |||
|
632 | } | |||
|
633 | ||||
|
634 | ||||
|
635 | /********************************************* | |||
|
636 | * ZOOM TRANSITION | |||
|
637 | *********************************************/ | |||
|
638 | ||||
|
639 | .reveal.zoom .slides>section, | |||
|
640 | .reveal.zoom .slides>section>section { | |||
|
641 | -webkit-transition: all 600ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
642 | -moz-transition: all 600ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
643 | -ms-transition: all 600ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
644 | -o-transition: all 600ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
645 | transition: all 600ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
646 | } | |||
|
647 | ||||
|
648 | .reveal.zoom .slides>section.past { | |||
|
649 | opacity: 0; | |||
|
650 | visibility: hidden; | |||
|
651 | ||||
|
652 | -webkit-transform: scale(16); | |||
|
653 | -moz-transform: scale(16); | |||
|
654 | -ms-transform: scale(16); | |||
|
655 | -o-transform: scale(16); | |||
|
656 | transform: scale(16); | |||
|
657 | } | |||
|
658 | .reveal.zoom .slides>section.future { | |||
|
659 | opacity: 0; | |||
|
660 | visibility: hidden; | |||
|
661 | ||||
|
662 | -webkit-transform: scale(0.2); | |||
|
663 | -moz-transform: scale(0.2); | |||
|
664 | -ms-transform: scale(0.2); | |||
|
665 | -o-transform: scale(0.2); | |||
|
666 | transform: scale(0.2); | |||
|
667 | } | |||
|
668 | ||||
|
669 | .reveal.zoom .slides>section>section.past { | |||
|
670 | -webkit-transform: translate(0, -150%); | |||
|
671 | -moz-transform: translate(0, -150%); | |||
|
672 | -ms-transform: translate(0, -150%); | |||
|
673 | -o-transform: translate(0, -150%); | |||
|
674 | transform: translate(0, -150%); | |||
|
675 | } | |||
|
676 | .reveal.zoom .slides>section>section.future { | |||
|
677 | -webkit-transform: translate(0, 150%); | |||
|
678 | -moz-transform: translate(0, 150%); | |||
|
679 | -ms-transform: translate(0, 150%); | |||
|
680 | -o-transform: translate(0, 150%); | |||
|
681 | transform: translate(0, 150%); | |||
|
682 | } | |||
|
683 | ||||
|
684 | ||||
|
685 | /********************************************* | |||
|
686 | * LINEAR TRANSITION | |||
|
687 | *********************************************/ | |||
|
688 | ||||
|
689 | .reveal.linear .slides>section.past { | |||
|
690 | -webkit-transform: translate(-150%, 0); | |||
|
691 | -moz-transform: translate(-150%, 0); | |||
|
692 | -ms-transform: translate(-150%, 0); | |||
|
693 | -o-transform: translate(-150%, 0); | |||
|
694 | transform: translate(-150%, 0); | |||
|
695 | } | |||
|
696 | .reveal.linear .slides>section.future { | |||
|
697 | -webkit-transform: translate(150%, 0); | |||
|
698 | -moz-transform: translate(150%, 0); | |||
|
699 | -ms-transform: translate(150%, 0); | |||
|
700 | -o-transform: translate(150%, 0); | |||
|
701 | transform: translate(150%, 0); | |||
|
702 | } | |||
|
703 | ||||
|
704 | .reveal.linear .slides>section>section.past { | |||
|
705 | -webkit-transform: translate(0, -150%); | |||
|
706 | -moz-transform: translate(0, -150%); | |||
|
707 | -ms-transform: translate(0, -150%); | |||
|
708 | -o-transform: translate(0, -150%); | |||
|
709 | transform: translate(0, -150%); | |||
|
710 | } | |||
|
711 | .reveal.linear .slides>section>section.future { | |||
|
712 | -webkit-transform: translate(0, 150%); | |||
|
713 | -moz-transform: translate(0, 150%); | |||
|
714 | -ms-transform: translate(0, 150%); | |||
|
715 | -o-transform: translate(0, 150%); | |||
|
716 | transform: translate(0, 150%); | |||
|
717 | } | |||
|
718 | ||||
|
719 | ||||
|
720 | /********************************************* | |||
|
721 | * CUBE TRANSITION | |||
|
722 | *********************************************/ | |||
|
723 | ||||
|
724 | .reveal.cube .slides { | |||
|
725 | -webkit-perspective-origin: 0% 25%; | |||
|
726 | -moz-perspective-origin: 0% 25%; | |||
|
727 | -ms-perspective-origin: 0% 25%; | |||
|
728 | perspective-origin: 0% 25%; | |||
|
729 | ||||
|
730 | -webkit-perspective: 1300px; | |||
|
731 | -moz-perspective: 1300px; | |||
|
732 | -ms-perspective: 1300px; | |||
|
733 | perspective: 1300px; | |||
|
734 | } | |||
|
735 | ||||
|
736 | .reveal.cube .slides section { | |||
|
737 | padding: 30px; | |||
|
738 | ||||
|
739 | -webkit-backface-visibility: hidden; | |||
|
740 | -moz-backface-visibility: hidden; | |||
|
741 | -ms-backface-visibility: hidden; | |||
|
742 | backface-visibility: hidden; | |||
|
743 | ||||
|
744 | -webkit-box-sizing: border-box; | |||
|
745 | -moz-box-sizing: border-box; | |||
|
746 | box-sizing: border-box; | |||
|
747 | } | |||
|
748 | .reveal.cube .slides section:not(.stack):before { | |||
|
749 | content: ''; | |||
|
750 | position: absolute; | |||
|
751 | display: block; | |||
|
752 | width: 100%; | |||
|
753 | height: 100%; | |||
|
754 | left: 0; | |||
|
755 | top: 0; | |||
|
756 | background: rgba(0,0,0,0.1); | |||
|
757 | border-radius: 4px; | |||
|
758 | ||||
|
759 | -webkit-transform: translateZ( -20px ); | |||
|
760 | -moz-transform: translateZ( -20px ); | |||
|
761 | -ms-transform: translateZ( -20px ); | |||
|
762 | -o-transform: translateZ( -20px ); | |||
|
763 | transform: translateZ( -20px ); | |||
|
764 | } | |||
|
765 | .reveal.cube .slides section:not(.stack):after { | |||
|
766 | content: ''; | |||
|
767 | position: absolute; | |||
|
768 | display: block; | |||
|
769 | width: 90%; | |||
|
770 | height: 30px; | |||
|
771 | left: 5%; | |||
|
772 | bottom: 0; | |||
|
773 | background: none; | |||
|
774 | z-index: 1; | |||
|
775 | ||||
|
776 | border-radius: 4px; | |||
|
777 | box-shadow: 0px 95px 25px rgba(0,0,0,0.2); | |||
|
778 | ||||
|
779 | -webkit-transform: translateZ(-90px) rotateX( 65deg ); | |||
|
780 | -moz-transform: translateZ(-90px) rotateX( 65deg ); | |||
|
781 | -ms-transform: translateZ(-90px) rotateX( 65deg ); | |||
|
782 | -o-transform: translateZ(-90px) rotateX( 65deg ); | |||
|
783 | transform: translateZ(-90px) rotateX( 65deg ); | |||
|
784 | } | |||
|
785 | ||||
|
786 | .reveal.cube .slides>section.stack { | |||
|
787 | padding: 0; | |||
|
788 | background: none; | |||
|
789 | } | |||
|
790 | ||||
|
791 | .reveal.cube .slides>section.past { | |||
|
792 | -webkit-transform-origin: 100% 0%; | |||
|
793 | -moz-transform-origin: 100% 0%; | |||
|
794 | -ms-transform-origin: 100% 0%; | |||
|
795 | transform-origin: 100% 0%; | |||
|
796 | ||||
|
797 | -webkit-transform: translate3d(-100%, 0, 0) rotateY(-90deg); | |||
|
798 | -moz-transform: translate3d(-100%, 0, 0) rotateY(-90deg); | |||
|
799 | -ms-transform: translate3d(-100%, 0, 0) rotateY(-90deg); | |||
|
800 | transform: translate3d(-100%, 0, 0) rotateY(-90deg); | |||
|
801 | } | |||
|
802 | ||||
|
803 | .reveal.cube .slides>section.future { | |||
|
804 | -webkit-transform-origin: 0% 0%; | |||
|
805 | -moz-transform-origin: 0% 0%; | |||
|
806 | -ms-transform-origin: 0% 0%; | |||
|
807 | transform-origin: 0% 0%; | |||
|
808 | ||||
|
809 | -webkit-transform: translate3d(100%, 0, 0) rotateY(90deg); | |||
|
810 | -moz-transform: translate3d(100%, 0, 0) rotateY(90deg); | |||
|
811 | -ms-transform: translate3d(100%, 0, 0) rotateY(90deg); | |||
|
812 | transform: translate3d(100%, 0, 0) rotateY(90deg); | |||
|
813 | } | |||
|
814 | ||||
|
815 | .reveal.cube .slides>section>section.past { | |||
|
816 | -webkit-transform-origin: 0% 100%; | |||
|
817 | -moz-transform-origin: 0% 100%; | |||
|
818 | -ms-transform-origin: 0% 100%; | |||
|
819 | transform-origin: 0% 100%; | |||
|
820 | ||||
|
821 | -webkit-transform: translate3d(0, -100%, 0) rotateX(90deg); | |||
|
822 | -moz-transform: translate3d(0, -100%, 0) rotateX(90deg); | |||
|
823 | -ms-transform: translate3d(0, -100%, 0) rotateX(90deg); | |||
|
824 | transform: translate3d(0, -100%, 0) rotateX(90deg); | |||
|
825 | } | |||
|
826 | ||||
|
827 | .reveal.cube .slides>section>section.future { | |||
|
828 | -webkit-transform-origin: 0% 0%; | |||
|
829 | -moz-transform-origin: 0% 0%; | |||
|
830 | -ms-transform-origin: 0% 0%; | |||
|
831 | transform-origin: 0% 0%; | |||
|
832 | ||||
|
833 | -webkit-transform: translate3d(0, 100%, 0) rotateX(-90deg); | |||
|
834 | -moz-transform: translate3d(0, 100%, 0) rotateX(-90deg); | |||
|
835 | -ms-transform: translate3d(0, 100%, 0) rotateX(-90deg); | |||
|
836 | transform: translate3d(0, 100%, 0) rotateX(-90deg); | |||
|
837 | } | |||
|
838 | ||||
|
839 | ||||
|
840 | /********************************************* | |||
|
841 | * PAGE TRANSITION | |||
|
842 | *********************************************/ | |||
|
843 | ||||
|
844 | .reveal.page .slides { | |||
|
845 | -webkit-perspective-origin: 0% 50%; | |||
|
846 | -moz-perspective-origin: 0% 50%; | |||
|
847 | -ms-perspective-origin: 0% 50%; | |||
|
848 | perspective-origin: 0% 50%; | |||
|
849 | ||||
|
850 | -webkit-perspective: 3000px; | |||
|
851 | -moz-perspective: 3000px; | |||
|
852 | -ms-perspective: 3000px; | |||
|
853 | perspective: 3000px; | |||
|
854 | } | |||
|
855 | ||||
|
856 | .reveal.page .slides section { | |||
|
857 | padding: 30px; | |||
|
858 | ||||
|
859 | -webkit-box-sizing: border-box; | |||
|
860 | -moz-box-sizing: border-box; | |||
|
861 | box-sizing: border-box; | |||
|
862 | } | |||
|
863 | .reveal.page .slides section.past { | |||
|
864 | z-index: 12; | |||
|
865 | } | |||
|
866 | .reveal.page .slides section:not(.stack):before { | |||
|
867 | content: ''; | |||
|
868 | position: absolute; | |||
|
869 | display: block; | |||
|
870 | width: 100%; | |||
|
871 | height: 100%; | |||
|
872 | left: 0; | |||
|
873 | top: 0; | |||
|
874 | background: rgba(0,0,0,0.1); | |||
|
875 | ||||
|
876 | -webkit-transform: translateZ( -20px ); | |||
|
877 | -moz-transform: translateZ( -20px ); | |||
|
878 | -ms-transform: translateZ( -20px ); | |||
|
879 | -o-transform: translateZ( -20px ); | |||
|
880 | transform: translateZ( -20px ); | |||
|
881 | } | |||
|
882 | .reveal.page .slides section:not(.stack):after { | |||
|
883 | content: ''; | |||
|
884 | position: absolute; | |||
|
885 | display: block; | |||
|
886 | width: 90%; | |||
|
887 | height: 30px; | |||
|
888 | left: 5%; | |||
|
889 | bottom: 0; | |||
|
890 | background: none; | |||
|
891 | z-index: 1; | |||
|
892 | ||||
|
893 | border-radius: 4px; | |||
|
894 | box-shadow: 0px 95px 25px rgba(0,0,0,0.2); | |||
|
895 | ||||
|
896 | -webkit-transform: translateZ(-90px) rotateX( 65deg ); | |||
|
897 | } | |||
|
898 | ||||
|
899 | .reveal.page .slides>section.stack { | |||
|
900 | padding: 0; | |||
|
901 | background: none; | |||
|
902 | } | |||
|
903 | ||||
|
904 | .reveal.page .slides>section.past { | |||
|
905 | -webkit-transform-origin: 0% 0%; | |||
|
906 | -moz-transform-origin: 0% 0%; | |||
|
907 | -ms-transform-origin: 0% 0%; | |||
|
908 | transform-origin: 0% 0%; | |||
|
909 | ||||
|
910 | -webkit-transform: translate3d(-40%, 0, 0) rotateY(-80deg); | |||
|
911 | -moz-transform: translate3d(-40%, 0, 0) rotateY(-80deg); | |||
|
912 | -ms-transform: translate3d(-40%, 0, 0) rotateY(-80deg); | |||
|
913 | transform: translate3d(-40%, 0, 0) rotateY(-80deg); | |||
|
914 | } | |||
|
915 | ||||
|
916 | .reveal.page .slides>section.future { | |||
|
917 | -webkit-transform-origin: 100% 0%; | |||
|
918 | -moz-transform-origin: 100% 0%; | |||
|
919 | -ms-transform-origin: 100% 0%; | |||
|
920 | transform-origin: 100% 0%; | |||
|
921 | ||||
|
922 | -webkit-transform: translate3d(0, 0, 0); | |||
|
923 | -moz-transform: translate3d(0, 0, 0); | |||
|
924 | -ms-transform: translate3d(0, 0, 0); | |||
|
925 | transform: translate3d(0, 0, 0); | |||
|
926 | } | |||
|
927 | ||||
|
928 | .reveal.page .slides>section>section.past { | |||
|
929 | -webkit-transform-origin: 0% 0%; | |||
|
930 | -moz-transform-origin: 0% 0%; | |||
|
931 | -ms-transform-origin: 0% 0%; | |||
|
932 | transform-origin: 0% 0%; | |||
|
933 | ||||
|
934 | -webkit-transform: translate3d(0, -40%, 0) rotateX(80deg); | |||
|
935 | -moz-transform: translate3d(0, -40%, 0) rotateX(80deg); | |||
|
936 | -ms-transform: translate3d(0, -40%, 0) rotateX(80deg); | |||
|
937 | transform: translate3d(0, -40%, 0) rotateX(80deg); | |||
|
938 | } | |||
|
939 | ||||
|
940 | .reveal.page .slides>section>section.future { | |||
|
941 | -webkit-transform-origin: 0% 100%; | |||
|
942 | -moz-transform-origin: 0% 100%; | |||
|
943 | -ms-transform-origin: 0% 100%; | |||
|
944 | transform-origin: 0% 100%; | |||
|
945 | ||||
|
946 | -webkit-transform: translate3d(0, 0, 0); | |||
|
947 | -moz-transform: translate3d(0, 0, 0); | |||
|
948 | -ms-transform: translate3d(0, 0, 0); | |||
|
949 | transform: translate3d(0, 0, 0); | |||
|
950 | } | |||
|
951 | ||||
|
952 | ||||
|
953 | /********************************************* | |||
|
954 | * TILE-FLIP TRANSITION (CSS shader) | |||
|
955 | *********************************************/ | |||
|
956 | ||||
|
957 | .reveal.tileflip .slides section.present { | |||
|
958 | -webkit-transform: none; | |||
|
959 | -webkit-transition-duration: 800ms; | |||
|
960 | ||||
|
961 | -webkit-filter: custom( url(shaders/tile-flip.vs) mix(url(shaders/tile-flip.fs) multiply source-atop), 10 10 border-box detached, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), | |||
|
962 | amount 0, randomness 0, flipAxis 0 1 0, tileOutline 1 | |||
|
963 | ); | |||
|
964 | } | |||
|
965 | ||||
|
966 | .reveal.tileflip .slides section.past { | |||
|
967 | -webkit-transform: none; | |||
|
968 | -webkit-transition-duration: 800ms; | |||
|
969 | ||||
|
970 | -webkit-filter: custom( url(shaders/tile-flip.vs) mix(url(shaders/tile-flip.fs) multiply source-atop), 10 10 border-box detached, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), | |||
|
971 | amount 1, randomness 0, flipAxis 0 1 0, tileOutline 1 | |||
|
972 | ); | |||
|
973 | } | |||
|
974 | ||||
|
975 | .reveal.tileflip .slides section.future { | |||
|
976 | -webkit-transform: none; | |||
|
977 | -webkit-transition-duration: 800ms; | |||
|
978 | ||||
|
979 | -webkit-filter: custom( url(shaders/tile-flip.vs) mix(url(shaders/tile-flip.fs) multiply source-atop), 10 10 border-box detached, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), | |||
|
980 | amount 1, randomness 0, flipAxis 0 1 0, tileOutline 1 | |||
|
981 | ); | |||
|
982 | } | |||
|
983 | ||||
|
984 | .reveal.tileflip .slides>section>section.present { | |||
|
985 | -webkit-filter: custom( url(shaders/tile-flip.vs) mix(url(shaders/tile-flip.fs) multiply source-atop), 10 10 border-box detached, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), | |||
|
986 | amount 0, randomness 2, flipAxis 1 0 0, tileOutline 1 | |||
|
987 | ); | |||
|
988 | } | |||
|
989 | ||||
|
990 | .reveal.tileflip .slides>section>section.past { | |||
|
991 | -webkit-filter: custom( url(shaders/tile-flip.vs) mix(url(shaders/tile-flip.fs) multiply source-atop), 10 10 border-box detached, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), | |||
|
992 | amount 1, randomness 2, flipAxis 1 0 0, tileOutline 1 | |||
|
993 | ); | |||
|
994 | } | |||
|
995 | ||||
|
996 | .reveal.tileflip .slides>section>section.future { | |||
|
997 | -webkit-filter: custom( url(shaders/tile-flip.vs) mix(url(shaders/tile-flip.fs) multiply source-atop), 10 10 border-box detached, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), | |||
|
998 | amount 1, randomness 2, flipAxis 1 0 0, tileOutline 1 | |||
|
999 | ); | |||
|
1000 | } | |||
|
1001 | ||||
|
1002 | ||||
|
1003 | /********************************************* | |||
|
1004 | * NO TRANSITION | |||
|
1005 | *********************************************/ | |||
|
1006 | ||||
|
1007 | .reveal.none .slides section { | |||
|
1008 | -webkit-transform: none; | |||
|
1009 | -moz-transform: none; | |||
|
1010 | -ms-transform: none; | |||
|
1011 | -o-transform: none; | |||
|
1012 | transform: none; | |||
|
1013 | ||||
|
1014 | -webkit-transition: none; | |||
|
1015 | -moz-transition: none; | |||
|
1016 | -ms-transition: none; | |||
|
1017 | -o-transition: none; | |||
|
1018 | transition: none; | |||
|
1019 | } | |||
|
1020 | ||||
|
1021 | ||||
|
1022 | /********************************************* | |||
|
1023 | * OVERVIEW | |||
|
1024 | *********************************************/ | |||
|
1025 | ||||
|
1026 | .reveal.overview .slides { | |||
|
1027 | -webkit-perspective: 700px; | |||
|
1028 | -moz-perspective: 700px; | |||
|
1029 | -ms-perspective: 700px; | |||
|
1030 | perspective: 700px; | |||
|
1031 | } | |||
|
1032 | ||||
|
1033 | .reveal.overview .slides section { | |||
|
1034 | padding: 20px 0; | |||
|
1035 | max-height: 600px; | |||
|
1036 | overflow: hidden; | |||
|
1037 | opacity: 1!important; | |||
|
1038 | visibility: visible!important; | |||
|
1039 | cursor: pointer; | |||
|
1040 | background: rgba(0,0,0,0.1); | |||
|
1041 | } | |||
|
1042 | .reveal.overview .slides section .fragment { | |||
|
1043 | opacity: 1; | |||
|
1044 | } | |||
|
1045 | .reveal.overview .slides section:after, | |||
|
1046 | .reveal.overview .slides section:before { | |||
|
1047 | display: none !important; | |||
|
1048 | } | |||
|
1049 | .reveal.overview .slides section>section { | |||
|
1050 | opacity: 1; | |||
|
1051 | cursor: pointer; | |||
|
1052 | } | |||
|
1053 | .reveal.overview .slides section:hover { | |||
|
1054 | background: rgba(0,0,0,0.3); | |||
|
1055 | } | |||
|
1056 | ||||
|
1057 | .reveal.overview .slides section.present { | |||
|
1058 | background: rgba(0,0,0,0.3); | |||
|
1059 | } | |||
|
1060 | .reveal.overview .slides>section.stack { | |||
|
1061 | background: none; | |||
|
1062 | padding: 0; | |||
|
1063 | overflow: visible; | |||
|
1064 | } | |||
|
1065 | ||||
|
1066 | ||||
|
1067 | /********************************************* | |||
|
1068 | * PAUSED MODE | |||
|
1069 | *********************************************/ | |||
|
1070 | ||||
|
1071 | .reveal .pause-overlay { | |||
|
1072 | position: absolute; | |||
|
1073 | top: 0; | |||
|
1074 | left: 0; | |||
|
1075 | width: 100%; | |||
|
1076 | height: 100%; | |||
|
1077 | background: black; | |||
|
1078 | visibility: hidden; | |||
|
1079 | opacity: 0; | |||
|
1080 | z-index: 100; | |||
|
1081 | ||||
|
1082 | -webkit-transition: all 1s ease; | |||
|
1083 | -moz-transition: all 1s ease; | |||
|
1084 | -ms-transition: all 1s ease; | |||
|
1085 | -o-transition: all 1s ease; | |||
|
1086 | transition: all 1s ease; | |||
|
1087 | } | |||
|
1088 | .reveal.paused .pause-overlay { | |||
|
1089 | visibility: visible; | |||
|
1090 | opacity: 1; | |||
|
1091 | } | |||
|
1092 | ||||
|
1093 | ||||
|
1094 | /********************************************* | |||
|
1095 | * FALLBACK | |||
|
1096 | *********************************************/ | |||
|
1097 | ||||
|
1098 | .no-transforms { | |||
|
1099 | overflow-y: auto; | |||
|
1100 | } | |||
|
1101 | ||||
|
1102 | .no-transforms .slides section { | |||
|
1103 | display: block!important; | |||
|
1104 | opacity: 1!important; | |||
|
1105 | position: relative!important; | |||
|
1106 | height: auto; | |||
|
1107 | min-height: auto; | |||
|
1108 | margin-bottom: 100px; | |||
|
1109 | ||||
|
1110 | -webkit-transform: none; | |||
|
1111 | -moz-transform: none; | |||
|
1112 | -ms-transform: none; | |||
|
1113 | transform: none; | |||
|
1114 | } | |||
|
1115 | ||||
|
1116 | ||||
|
1117 | /********************************************* | |||
|
1118 | * BACKGROUND STATES | |||
|
1119 | *********************************************/ | |||
|
1120 | ||||
|
1121 | .reveal .state-background { | |||
|
1122 | position: absolute; | |||
|
1123 | width: 100%; | |||
|
1124 | height: 100%; | |||
|
1125 | background: rgba( 0, 0, 0, 0 ); | |||
|
1126 | ||||
|
1127 | -webkit-transition: background 800ms ease; | |||
|
1128 | -moz-transition: background 800ms ease; | |||
|
1129 | -ms-transition: background 800ms ease; | |||
|
1130 | -o-transition: background 800ms ease; | |||
|
1131 | transition: background 800ms ease; | |||
|
1132 | } | |||
|
1133 | .alert .reveal .state-background { | |||
|
1134 | background: rgba( 200, 50, 30, 0.6 ); | |||
|
1135 | } | |||
|
1136 | .soothe .reveal .state-background { | |||
|
1137 | background: rgba( 50, 200, 90, 0.4 ); | |||
|
1138 | } | |||
|
1139 | .blackout .reveal .state-background { | |||
|
1140 | background: rgba( 0, 0, 0, 0.6 ); | |||
|
1141 | } | |||
|
1142 | ||||
|
1143 | ||||
|
1144 | /********************************************* | |||
|
1145 | * SPEAKER NOTES | |||
|
1146 | *********************************************/ | |||
|
1147 | ||||
|
1148 | .reveal aside.notes { | |||
|
1149 | display: none; | |||
|
1150 | } | |||
|
1151 | ||||
|
1152 | ||||
|
1153 | /********************************************* | |||
|
1154 | * ZOOM PLUGIN | |||
|
1155 | *********************************************/ | |||
|
1156 | ||||
|
1157 | .zoomed .reveal *, | |||
|
1158 | .zoomed .reveal *:before, | |||
|
1159 | .zoomed .reveal *:after { | |||
|
1160 | -webkit-transform: none !important; | |||
|
1161 | -moz-transform: none !important; | |||
|
1162 | -ms-transform: none !important; | |||
|
1163 | transform: none !important; | |||
|
1164 | ||||
|
1165 | -webkit-backface-visibility: visible !important; | |||
|
1166 | -moz-backface-visibility: visible !important; | |||
|
1167 | -ms-backface-visibility: visible !important; | |||
|
1168 | backface-visibility: visible !important; | |||
|
1169 | } | |||
|
1170 | ||||
|
1171 | .zoomed .reveal .progress, | |||
|
1172 | .zoomed .reveal .controls { | |||
|
1173 | opacity: 0; | |||
|
1174 | } | |||
|
1175 | ||||
|
1176 | .zoomed .reveal .roll span { | |||
|
1177 | background: none; | |||
|
1178 | } | |||
|
1179 | ||||
|
1180 | .zoomed .reveal .roll span:after { | |||
|
1181 | visibility: hidden; | |||
|
1182 | } | |||
|
1183 | ||||
|
1184 |
@@ -0,0 +1,64 b'' | |||||
|
1 | /* | |||
|
2 | * Copyright (c) 2012 Adobe Systems Incorporated. All rights reserved. | |||
|
3 | * Copyright (c) 2012 Branislav Ulicny | |||
|
4 | * | |||
|
5 | * Licensed under the Apache License, Version 2.0 (the "License"); | |||
|
6 | * you may not use this file except in compliance with the License. | |||
|
7 | * You may obtain a copy of the License at | |||
|
8 | * | |||
|
9 | * http://www.apache.org/licenses/LICENSE-2.0 | |||
|
10 | * | |||
|
11 | * Unless required by applicable law or agreed to in writing, software | |||
|
12 | * distributed under the License is distributed on an "AS IS" BASIS, | |||
|
13 | * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | |||
|
14 | * See the License for the specific language governing permissions and | |||
|
15 | * limitations under the License. | |||
|
16 | */ | |||
|
17 | ||||
|
18 | precision mediump float; | |||
|
19 | ||||
|
20 | // Uniform values from CSS | |||
|
21 | ||||
|
22 | uniform float amount; | |||
|
23 | uniform float tileOutline; | |||
|
24 | ||||
|
25 | // Built-in uniforms | |||
|
26 | ||||
|
27 | uniform vec2 u_meshSize; | |||
|
28 | uniform vec2 u_textureSize; | |||
|
29 | ||||
|
30 | // Varyings passed in from vertex shader | |||
|
31 | ||||
|
32 | varying float v_depth; | |||
|
33 | varying vec2 v_uv; | |||
|
34 | ||||
|
35 | // Main | |||
|
36 | ||||
|
37 | void main() | |||
|
38 | { | |||
|
39 | // FIXME: Must swap x and y as a workaround for: | |||
|
40 | // https://bugs.webkit.org/show_bug.cgi?id=96285 | |||
|
41 | vec2 u_meshSize = u_meshSize.yx; | |||
|
42 | ||||
|
43 | vec4 c = vec4(1.0); | |||
|
44 | ||||
|
45 | // Fade out. | |||
|
46 | c.a = 1.0 - v_depth; | |||
|
47 | ||||
|
48 | // Show grid outline. | |||
|
49 | if (tileOutline >= 0.5) { | |||
|
50 | float cell_width = u_textureSize.x / u_meshSize.y; | |||
|
51 | float cell_height = u_textureSize.y / u_meshSize.x; | |||
|
52 | float dd = 1.0; | |||
|
53 | ||||
|
54 | if (mod(v_uv.x * u_textureSize.x + dd, cell_width) < 2.0 | |||
|
55 | || mod(v_uv.y * u_textureSize.y + dd, cell_height) < 2.0) { | |||
|
56 | if (amount > 0.0) | |||
|
57 | c.rgb = vec3(1.0 - sqrt(amount)); | |||
|
58 | } | |||
|
59 | } | |||
|
60 | css_ColorMatrix = mat4(c.r, 0.0, 0.0, 0.0, | |||
|
61 | 0.0, c.g, 0.0, 0.0, | |||
|
62 | 0.0, 0.0, c.b, 0.0, | |||
|
63 | 0.0, 0.0, 0.0, c.a); | |||
|
64 | } |
@@ -0,0 +1,141 b'' | |||||
|
1 | /* | |||
|
2 | * Copyright (c)2012 Adobe Systems Incorporated. All rights reserved. | |||
|
3 | * Copyright (c)2012 Branislav Ulicny | |||
|
4 | * | |||
|
5 | * Licensed under the Apache License, Version 2.0 (the "License"); | |||
|
6 | * you may not use this file except in compliance with the License. | |||
|
7 | * You may obtain a copy of the License at | |||
|
8 | * | |||
|
9 | * http://www.apache.org/licenses/LICENSE-2.0 | |||
|
10 | * | |||
|
11 | * Unless required by applicable law or agreed to in writing, software | |||
|
12 | * distributed under the License is distributed on an "AS IS" BASIS, | |||
|
13 | * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | |||
|
14 | * See the License for the specific language governing permissions and | |||
|
15 | * limitations under the License. | |||
|
16 | */ | |||
|
17 | ||||
|
18 | precision mediump float; | |||
|
19 | ||||
|
20 | // Built-in attributes | |||
|
21 | ||||
|
22 | attribute vec4 a_position; | |||
|
23 | attribute vec2 a_texCoord; | |||
|
24 | attribute vec3 a_triangleCoord; | |||
|
25 | ||||
|
26 | // Built-in uniforms | |||
|
27 | ||||
|
28 | uniform mat4 u_projectionMatrix; | |||
|
29 | uniform vec2 u_meshSize; | |||
|
30 | uniform vec2 u_textureSize; | |||
|
31 | ||||
|
32 | // Uniform passed in from CSS | |||
|
33 | ||||
|
34 | uniform mat4 transform; | |||
|
35 | uniform float amount; | |||
|
36 | uniform float randomness; | |||
|
37 | uniform vec3 flipAxis; | |||
|
38 | ||||
|
39 | // Varyings | |||
|
40 | ||||
|
41 | varying float v_depth; | |||
|
42 | varying vec2 v_uv; | |||
|
43 | ||||
|
44 | // Constants | |||
|
45 | ||||
|
46 | const float PI2 = 1.5707963267948966; | |||
|
47 | ||||
|
48 | // Create perspective matrix | |||
|
49 | ||||
|
50 | mat4 perspectiveMatrix(float p) | |||
|
51 | { | |||
|
52 | float perspective = - 1.0 / p; | |||
|
53 | return mat4( | |||
|
54 | 1.0, 0.0, 0.0, 0.0, | |||
|
55 | 0.0, 1.0, 0.0, 0.0, | |||
|
56 | 0.0, 0.0, 1.0, perspective, | |||
|
57 | 0.0, 0.0, 0.0, 1.0 | |||
|
58 | ); | |||
|
59 | } | |||
|
60 | ||||
|
61 | // Rotate vector | |||
|
62 | ||||
|
63 | vec3 rotateVectorByQuaternion(vec3 v, vec4 q) | |||
|
64 | { | |||
|
65 | vec3 dest = vec3(0.0); | |||
|
66 | ||||
|
67 | float x = v.x, y = v.y, z = v.z; | |||
|
68 | float qx = q.x, qy = q.y, qz = q.z, qw = q.w; | |||
|
69 | ||||
|
70 | // Calculate quaternion * vector. | |||
|
71 | ||||
|
72 | float ix = qw * x + qy * z - qz * y, | |||
|
73 | iy = qw * y + qz * x - qx * z, | |||
|
74 | iz = qw * z + qx * y - qy * x, | |||
|
75 | iw = -qx * x - qy * y - qz * z; | |||
|
76 | ||||
|
77 | // Calculate result * inverse quaternion. | |||
|
78 | ||||
|
79 | dest.x = ix * qw + iw * -qx + iy * -qz - iz * -qy; | |||
|
80 | dest.y = iy * qw + iw * -qy + iz * -qx - ix * -qz; | |||
|
81 | dest.z = iz * qw + iw * -qz + ix * -qy - iy * -qx; | |||
|
82 | ||||
|
83 | return dest; | |||
|
84 | } | |||
|
85 | ||||
|
86 | // Convert rotation. | |||
|
87 | ||||
|
88 | vec4 axisAngleToQuaternion(vec3 axis, float angle) | |||
|
89 | { | |||
|
90 | vec4 dest = vec4(0.0); | |||
|
91 | ||||
|
92 | float halfAngle = angle / 2.0; | |||
|
93 | float s = sin(halfAngle); | |||
|
94 | ||||
|
95 | dest.x = axis.x * s; | |||
|
96 | dest.y = axis.y * s; | |||
|
97 | dest.z = axis.z * s; | |||
|
98 | dest.w = cos(halfAngle); | |||
|
99 | ||||
|
100 | return dest; | |||
|
101 | } | |||
|
102 | ||||
|
103 | // Random function based on the tile coordinate. | |||
|
104 | // This will return the same value for all the vertices in the same tile (i.e. two triangles). | |||
|
105 | ||||
|
106 | float random(vec2 scale) | |||
|
107 | { | |||
|
108 | // Use the fragment position as a different seed per-pixel. | |||
|
109 | return fract(sin(dot(vec2(a_triangleCoord.x, a_triangleCoord.y), scale)) * 4000.0); | |||
|
110 | } | |||
|
111 | ||||
|
112 | // Main | |||
|
113 | ||||
|
114 | void main() | |||
|
115 | { | |||
|
116 | // FIXME: We must swap x and y as a workaround for: | |||
|
117 | // https://bugs.webkit.org/show_bug.cgi?id=96285 | |||
|
118 | vec2 u_meshSize = u_meshSize.yx; | |||
|
119 | ||||
|
120 | vec4 pos = a_position; | |||
|
121 | float aspect = u_textureSize.x / u_textureSize.y; | |||
|
122 | ||||
|
123 | float cx = a_triangleCoord.x / u_meshSize.y - 0.5 + 0.5 / u_meshSize.y; | |||
|
124 | float cy = a_triangleCoord.y / u_meshSize.x - 0.5 + 0.5 / u_meshSize.x; | |||
|
125 | ||||
|
126 | vec3 centroid = vec3(cx, cy, 0.0); | |||
|
127 | float r = random(vec2(10.0, 80.0)); | |||
|
128 | float rr = mix(0.0, PI2, amount * (1.0 + randomness * r)); | |||
|
129 | ||||
|
130 | vec4 rotation = vec4(flipAxis, rr); | |||
|
131 | vec4 qRotation = axisAngleToQuaternion(normalize(rotation.xyz), rotation.w); | |||
|
132 | ||||
|
133 | vec3 newPosition = rotateVectorByQuaternion((pos.xyz - centroid)* vec3(aspect, 1., 1.0), qRotation) * vec3(1.0 / aspect, 1.0, 1.0) + centroid; | |||
|
134 | pos.xyz = newPosition; | |||
|
135 | ||||
|
136 | gl_Position = u_projectionMatrix * transform * pos; | |||
|
137 | ||||
|
138 | // Pass varyings to the fragment shader. | |||
|
139 | v_depth = abs(rr)/ PI2; | |||
|
140 | v_uv = a_texCoord; | |||
|
141 | } |
@@ -0,0 +1,5 b'' | |||||
|
1 | Themes are written using Sass to keep things modular and reduce the need for repeated selectors across files. Find out how to install Sass here http://sass-lang.com/, once Sass is installed run the follwing command to start monitoring the source files for changes. | |||
|
2 | ||||
|
3 | ``` | |||
|
4 | sass --watch css/theme/source/:css/theme --style expanded | |||
|
5 | ``` No newline at end of file |
@@ -0,0 +1,163 b'' | |||||
|
1 | @import url(http://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic); | |||
|
2 | /** | |||
|
3 | * Beige theme for reveal.js. | |||
|
4 | * | |||
|
5 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
6 | */ | |||
|
7 | @font-face { | |||
|
8 | font-family: 'League Gothic'; | |||
|
9 | src: url("../../lib/font/league_gothic-webfont.eot"); | |||
|
10 | src: url("../../lib/font/league_gothic-webfont.eot?#iefix") format("embedded-opentype"), url("../../lib/font/league_gothic-webfont.woff") format("woff"), url("../../lib/font/league_gothic-webfont.ttf") format("truetype"), url("../../lib/font/league_gothic-webfont.svg#LeagueGothicRegular") format("svg"); | |||
|
11 | font-weight: normal; | |||
|
12 | font-style: normal; | |||
|
13 | } | |||
|
14 | ||||
|
15 | /********************************************* | |||
|
16 | * GLOBAL STYLES | |||
|
17 | *********************************************/ | |||
|
18 | body { | |||
|
19 | background: #f7f2d3; | |||
|
20 | background: -moz-radial-gradient(center, circle cover, white 0%, #f7f2d3 100%); | |||
|
21 | background: -webkit-gradient(radial, center center, 0px, center center, 100%, color-stop(0%, white), color-stop(100%, #f7f2d3)); | |||
|
22 | background: -webkit-radial-gradient(center, circle cover, white 0%, #f7f2d3 100%); | |||
|
23 | background: -o-radial-gradient(center, circle cover, white 0%, #f7f2d3 100%); | |||
|
24 | background: -ms-radial-gradient(center, circle cover, white 0%, #f7f2d3 100%); | |||
|
25 | background: radial-gradient(center, circle cover, white 0%, #f7f2d3 100%); | |||
|
26 | background-color: #f7f3de; | |||
|
27 | } | |||
|
28 | ||||
|
29 | .reveal { | |||
|
30 | font-family: "Lato", Times, "Times New Roman", serif; | |||
|
31 | font-size: 36px; | |||
|
32 | font-weight: 200; | |||
|
33 | letter-spacing: -0.02em; | |||
|
34 | color: #333333; | |||
|
35 | } | |||
|
36 | ||||
|
37 | ::selection { | |||
|
38 | color: white; | |||
|
39 | background: rgba(79, 64, 28, 0.99); | |||
|
40 | text-shadow: none; | |||
|
41 | } | |||
|
42 | ||||
|
43 | /********************************************* | |||
|
44 | * HEADERS | |||
|
45 | *********************************************/ | |||
|
46 | .reveal h1, | |||
|
47 | .reveal h2, | |||
|
48 | .reveal h3, | |||
|
49 | .reveal h4, | |||
|
50 | .reveal h5, | |||
|
51 | .reveal h6 { | |||
|
52 | margin: 0 0 20px 0; | |||
|
53 | color: #333333; | |||
|
54 | font-family: "League Gothic", Impact, sans-serif; | |||
|
55 | line-height: 0.9em; | |||
|
56 | letter-spacing: 0.02em; | |||
|
57 | text-transform: uppercase; | |||
|
58 | text-shadow: none; | |||
|
59 | } | |||
|
60 | ||||
|
61 | .reveal h1 { | |||
|
62 | text-shadow: 0 1px 0 #cccccc, 0 2px 0 #c9c9c9, 0 3px 0 #bbbbbb, 0 4px 0 #b9b9b9, 0 5px 0 #aaaaaa, 0 6px 1px rgba(0, 0, 0, 0.1), 0 0 5px rgba(0, 0, 0, 0.1), 0 1px 3px rgba(0, 0, 0, 0.3), 0 3px 5px rgba(0, 0, 0, 0.2), 0 5px 10px rgba(0, 0, 0, 0.25), 0 20px 20px rgba(0, 0, 0, 0.15); | |||
|
63 | } | |||
|
64 | ||||
|
65 | /********************************************* | |||
|
66 | * LINKS | |||
|
67 | *********************************************/ | |||
|
68 | .reveal a:not(.image) { | |||
|
69 | color: #8b743d; | |||
|
70 | text-decoration: none; | |||
|
71 | -webkit-transition: color .15s ease; | |||
|
72 | -moz-transition: color .15s ease; | |||
|
73 | -ms-transition: color .15s ease; | |||
|
74 | -o-transition: color .15s ease; | |||
|
75 | transition: color .15s ease; | |||
|
76 | } | |||
|
77 | ||||
|
78 | .reveal a:not(.image):hover { | |||
|
79 | color: #c0a86e; | |||
|
80 | text-shadow: none; | |||
|
81 | border: none; | |||
|
82 | } | |||
|
83 | ||||
|
84 | .reveal .roll span:after { | |||
|
85 | color: #fff; | |||
|
86 | background: #564826; | |||
|
87 | } | |||
|
88 | ||||
|
89 | /********************************************* | |||
|
90 | * IMAGES | |||
|
91 | *********************************************/ | |||
|
92 | .reveal section img { | |||
|
93 | margin: 15px; | |||
|
94 | background: rgba(255, 255, 255, 0.12); | |||
|
95 | border: 4px solid #333333; | |||
|
96 | box-shadow: 0 0 10px rgba(0, 0, 0, 0.15); | |||
|
97 | -webkit-transition: all .2s linear; | |||
|
98 | -moz-transition: all .2s linear; | |||
|
99 | -ms-transition: all .2s linear; | |||
|
100 | -o-transition: all .2s linear; | |||
|
101 | transition: all .2s linear; | |||
|
102 | } | |||
|
103 | ||||
|
104 | .reveal a:hover img { | |||
|
105 | background: rgba(255, 255, 255, 0.2); | |||
|
106 | border-color: #8b743d; | |||
|
107 | box-shadow: 0 0 20px rgba(0, 0, 0, 0.55); | |||
|
108 | } | |||
|
109 | ||||
|
110 | /********************************************* | |||
|
111 | * NAVIGATION CONTROLS | |||
|
112 | *********************************************/ | |||
|
113 | .reveal .controls div.left, | |||
|
114 | .reveal .controls div.left.enabled { | |||
|
115 | border-right-color: #8b743d; | |||
|
116 | } | |||
|
117 | ||||
|
118 | .reveal .controls div.right, | |||
|
119 | .reveal .controls div.right.enabled { | |||
|
120 | border-left-color: #8b743d; | |||
|
121 | } | |||
|
122 | ||||
|
123 | .reveal .controls div.up, | |||
|
124 | .reveal .controls div.up.enabled { | |||
|
125 | border-bottom-color: #8b743d; | |||
|
126 | } | |||
|
127 | ||||
|
128 | .reveal .controls div.down, | |||
|
129 | .reveal .controls div.down.enabled { | |||
|
130 | border-top-color: #8b743d; | |||
|
131 | } | |||
|
132 | ||||
|
133 | .reveal .controls div.left.enabled:hover { | |||
|
134 | border-right-color: #c0a86e; | |||
|
135 | } | |||
|
136 | ||||
|
137 | .reveal .controls div.right.enabled:hover { | |||
|
138 | border-left-color: #c0a86e; | |||
|
139 | } | |||
|
140 | ||||
|
141 | .reveal .controls div.up.enabled:hover { | |||
|
142 | border-bottom-color: #c0a86e; | |||
|
143 | } | |||
|
144 | ||||
|
145 | .reveal .controls div.down.enabled:hover { | |||
|
146 | border-top-color: #c0a86e; | |||
|
147 | } | |||
|
148 | ||||
|
149 | /********************************************* | |||
|
150 | * PROGRESS BAR | |||
|
151 | *********************************************/ | |||
|
152 | .reveal .progress { | |||
|
153 | background: rgba(0, 0, 0, 0.2); | |||
|
154 | } | |||
|
155 | ||||
|
156 | .reveal .progress span { | |||
|
157 | background: #8b743d; | |||
|
158 | -webkit-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
159 | -moz-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
160 | -ms-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
161 | -o-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
162 | transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
163 | } |
@@ -0,0 +1,163 b'' | |||||
|
1 | @import url(http://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic); | |||
|
2 | /** | |||
|
3 | * Default theme for reveal.js. | |||
|
4 | * | |||
|
5 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
6 | */ | |||
|
7 | @font-face { | |||
|
8 | font-family: 'League Gothic'; | |||
|
9 | src: url("../../lib/font/league_gothic-webfont.eot"); | |||
|
10 | src: url("../../lib/font/league_gothic-webfont.eot?#iefix") format("embedded-opentype"), url("../../lib/font/league_gothic-webfont.woff") format("woff"), url("../../lib/font/league_gothic-webfont.ttf") format("truetype"), url("../../lib/font/league_gothic-webfont.svg#LeagueGothicRegular") format("svg"); | |||
|
11 | font-weight: normal; | |||
|
12 | font-style: normal; | |||
|
13 | } | |||
|
14 | ||||
|
15 | /********************************************* | |||
|
16 | * GLOBAL STYLES | |||
|
17 | *********************************************/ | |||
|
18 | body { | |||
|
19 | background: #1c1e20; | |||
|
20 | background: -moz-radial-gradient(center, circle cover, #555a5f 0%, #1c1e20 100%); | |||
|
21 | background: -webkit-gradient(radial, center center, 0px, center center, 100%, color-stop(0%, #555a5f), color-stop(100%, #1c1e20)); | |||
|
22 | background: -webkit-radial-gradient(center, circle cover, #555a5f 0%, #1c1e20 100%); | |||
|
23 | background: -o-radial-gradient(center, circle cover, #555a5f 0%, #1c1e20 100%); | |||
|
24 | background: -ms-radial-gradient(center, circle cover, #555a5f 0%, #1c1e20 100%); | |||
|
25 | background: radial-gradient(center, circle cover, #555a5f 0%, #1c1e20 100%); | |||
|
26 | background-color: #2b2b2b; | |||
|
27 | } | |||
|
28 | ||||
|
29 | .reveal { | |||
|
30 | font-family: "Lato", Times, "Times New Roman", serif; | |||
|
31 | font-size: 36px; | |||
|
32 | font-weight: 200; | |||
|
33 | letter-spacing: -0.02em; | |||
|
34 | color: #eeeeee; | |||
|
35 | } | |||
|
36 | ||||
|
37 | ::selection { | |||
|
38 | color: white; | |||
|
39 | background: #ff5e99; | |||
|
40 | text-shadow: none; | |||
|
41 | } | |||
|
42 | ||||
|
43 | /********************************************* | |||
|
44 | * HEADERS | |||
|
45 | *********************************************/ | |||
|
46 | .reveal h1, | |||
|
47 | .reveal h2, | |||
|
48 | .reveal h3, | |||
|
49 | .reveal h4, | |||
|
50 | .reveal h5, | |||
|
51 | .reveal h6 { | |||
|
52 | margin: 0 0 20px 0; | |||
|
53 | color: #eeeeee; | |||
|
54 | font-family: "League Gothic", Impact, sans-serif; | |||
|
55 | line-height: 0.9em; | |||
|
56 | letter-spacing: 0.02em; | |||
|
57 | text-transform: uppercase; | |||
|
58 | text-shadow: 0px 0px 6px rgba(0, 0, 0, 0.2); | |||
|
59 | } | |||
|
60 | ||||
|
61 | .reveal h1 { | |||
|
62 | text-shadow: 0 1px 0 #cccccc, 0 2px 0 #c9c9c9, 0 3px 0 #bbbbbb, 0 4px 0 #b9b9b9, 0 5px 0 #aaaaaa, 0 6px 1px rgba(0, 0, 0, 0.1), 0 0 5px rgba(0, 0, 0, 0.1), 0 1px 3px rgba(0, 0, 0, 0.3), 0 3px 5px rgba(0, 0, 0, 0.2), 0 5px 10px rgba(0, 0, 0, 0.25), 0 20px 20px rgba(0, 0, 0, 0.15); | |||
|
63 | } | |||
|
64 | ||||
|
65 | /********************************************* | |||
|
66 | * LINKS | |||
|
67 | *********************************************/ | |||
|
68 | .reveal a:not(.image) { | |||
|
69 | color: #13daec; | |||
|
70 | text-decoration: none; | |||
|
71 | -webkit-transition: color .15s ease; | |||
|
72 | -moz-transition: color .15s ease; | |||
|
73 | -ms-transition: color .15s ease; | |||
|
74 | -o-transition: color .15s ease; | |||
|
75 | transition: color .15s ease; | |||
|
76 | } | |||
|
77 | ||||
|
78 | .reveal a:not(.image):hover { | |||
|
79 | color: #71e9f4; | |||
|
80 | text-shadow: none; | |||
|
81 | border: none; | |||
|
82 | } | |||
|
83 | ||||
|
84 | .reveal .roll span:after { | |||
|
85 | color: #fff; | |||
|
86 | background: #0d99a5; | |||
|
87 | } | |||
|
88 | ||||
|
89 | /********************************************* | |||
|
90 | * IMAGES | |||
|
91 | *********************************************/ | |||
|
92 | .reveal section img { | |||
|
93 | margin: 15px; | |||
|
94 | background: rgba(255, 255, 255, 0.12); | |||
|
95 | border: 4px solid #eeeeee; | |||
|
96 | box-shadow: 0 0 10px rgba(0, 0, 0, 0.15); | |||
|
97 | -webkit-transition: all .2s linear; | |||
|
98 | -moz-transition: all .2s linear; | |||
|
99 | -ms-transition: all .2s linear; | |||
|
100 | -o-transition: all .2s linear; | |||
|
101 | transition: all .2s linear; | |||
|
102 | } | |||
|
103 | ||||
|
104 | .reveal a:hover img { | |||
|
105 | background: rgba(255, 255, 255, 0.2); | |||
|
106 | border-color: #13daec; | |||
|
107 | box-shadow: 0 0 20px rgba(0, 0, 0, 0.55); | |||
|
108 | } | |||
|
109 | ||||
|
110 | /********************************************* | |||
|
111 | * NAVIGATION CONTROLS | |||
|
112 | *********************************************/ | |||
|
113 | .reveal .controls div.left, | |||
|
114 | .reveal .controls div.left.enabled { | |||
|
115 | border-right-color: #13daec; | |||
|
116 | } | |||
|
117 | ||||
|
118 | .reveal .controls div.right, | |||
|
119 | .reveal .controls div.right.enabled { | |||
|
120 | border-left-color: #13daec; | |||
|
121 | } | |||
|
122 | ||||
|
123 | .reveal .controls div.up, | |||
|
124 | .reveal .controls div.up.enabled { | |||
|
125 | border-bottom-color: #13daec; | |||
|
126 | } | |||
|
127 | ||||
|
128 | .reveal .controls div.down, | |||
|
129 | .reveal .controls div.down.enabled { | |||
|
130 | border-top-color: #13daec; | |||
|
131 | } | |||
|
132 | ||||
|
133 | .reveal .controls div.left.enabled:hover { | |||
|
134 | border-right-color: #71e9f4; | |||
|
135 | } | |||
|
136 | ||||
|
137 | .reveal .controls div.right.enabled:hover { | |||
|
138 | border-left-color: #71e9f4; | |||
|
139 | } | |||
|
140 | ||||
|
141 | .reveal .controls div.up.enabled:hover { | |||
|
142 | border-bottom-color: #71e9f4; | |||
|
143 | } | |||
|
144 | ||||
|
145 | .reveal .controls div.down.enabled:hover { | |||
|
146 | border-top-color: #71e9f4; | |||
|
147 | } | |||
|
148 | ||||
|
149 | /********************************************* | |||
|
150 | * PROGRESS BAR | |||
|
151 | *********************************************/ | |||
|
152 | .reveal .progress { | |||
|
153 | background: rgba(0, 0, 0, 0.2); | |||
|
154 | } | |||
|
155 | ||||
|
156 | .reveal .progress span { | |||
|
157 | background: #13daec; | |||
|
158 | -webkit-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
159 | -moz-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
160 | -ms-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
161 | -o-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
162 | transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
163 | } |
@@ -0,0 +1,150 b'' | |||||
|
1 | /** | |||
|
2 | * A simple theme for reveal.js presentations, similar | |||
|
3 | * to the default theme. The accent color is darkblue. | |||
|
4 | * | |||
|
5 | * This theme is Copyright (C) 2012 Owen Versteeg, https://github.com/StereotypicalApps. It is MIT licensed. | |||
|
6 | * reveal.js is Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se; so is the theme - beige.css - that this is based off of. | |||
|
7 | */ | |||
|
8 | /********************************************* | |||
|
9 | * GLOBAL STYLES | |||
|
10 | *********************************************/ | |||
|
11 | body { | |||
|
12 | background: #f0f1eb; | |||
|
13 | background-color: #f0f1eb; | |||
|
14 | } | |||
|
15 | ||||
|
16 | .reveal { | |||
|
17 | font-family: "Palatino Linotype", "Book Antiqua", Palatino, FreeSerif, serif; | |||
|
18 | font-size: 36px; | |||
|
19 | font-weight: 200; | |||
|
20 | letter-spacing: -0.02em; | |||
|
21 | color: black; | |||
|
22 | } | |||
|
23 | ||||
|
24 | ::selection { | |||
|
25 | color: white; | |||
|
26 | background: #26351c; | |||
|
27 | text-shadow: none; | |||
|
28 | } | |||
|
29 | ||||
|
30 | /********************************************* | |||
|
31 | * HEADERS | |||
|
32 | *********************************************/ | |||
|
33 | .reveal h1, | |||
|
34 | .reveal h2, | |||
|
35 | .reveal h3, | |||
|
36 | .reveal h4, | |||
|
37 | .reveal h5, | |||
|
38 | .reveal h6 { | |||
|
39 | margin: 0 0 20px 0; | |||
|
40 | color: #383d3d; | |||
|
41 | font-family: "Palatino Linotype", "Book Antiqua", Palatino, FreeSerif, serif; | |||
|
42 | line-height: 0.9em; | |||
|
43 | letter-spacing: 0.02em; | |||
|
44 | text-transform: none; | |||
|
45 | text-shadow: none; | |||
|
46 | } | |||
|
47 | ||||
|
48 | .reveal h1 { | |||
|
49 | text-shadow: 0px 0px 6px rgba(0, 0, 0, 0.2); | |||
|
50 | } | |||
|
51 | ||||
|
52 | /********************************************* | |||
|
53 | * LINKS | |||
|
54 | *********************************************/ | |||
|
55 | .reveal a:not(.image) { | |||
|
56 | color: #51483d; | |||
|
57 | text-decoration: none; | |||
|
58 | -webkit-transition: color .15s ease; | |||
|
59 | -moz-transition: color .15s ease; | |||
|
60 | -ms-transition: color .15s ease; | |||
|
61 | -o-transition: color .15s ease; | |||
|
62 | transition: color .15s ease; | |||
|
63 | } | |||
|
64 | ||||
|
65 | .reveal a:not(.image):hover { | |||
|
66 | color: #8b7c69; | |||
|
67 | text-shadow: none; | |||
|
68 | border: none; | |||
|
69 | } | |||
|
70 | ||||
|
71 | .reveal .roll span:after { | |||
|
72 | color: #fff; | |||
|
73 | background: #25211c; | |||
|
74 | } | |||
|
75 | ||||
|
76 | /********************************************* | |||
|
77 | * IMAGES | |||
|
78 | *********************************************/ | |||
|
79 | .reveal section img { | |||
|
80 | margin: 15px; | |||
|
81 | background: rgba(255, 255, 255, 0.12); | |||
|
82 | border: 4px solid black; | |||
|
83 | box-shadow: 0 0 10px rgba(0, 0, 0, 0.15); | |||
|
84 | -webkit-transition: all .2s linear; | |||
|
85 | -moz-transition: all .2s linear; | |||
|
86 | -ms-transition: all .2s linear; | |||
|
87 | -o-transition: all .2s linear; | |||
|
88 | transition: all .2s linear; | |||
|
89 | } | |||
|
90 | ||||
|
91 | .reveal a:hover img { | |||
|
92 | background: rgba(255, 255, 255, 0.2); | |||
|
93 | border-color: #51483d; | |||
|
94 | box-shadow: 0 0 20px rgba(0, 0, 0, 0.55); | |||
|
95 | } | |||
|
96 | ||||
|
97 | /********************************************* | |||
|
98 | * NAVIGATION CONTROLS | |||
|
99 | *********************************************/ | |||
|
100 | .reveal .controls div.left, | |||
|
101 | .reveal .controls div.left.enabled { | |||
|
102 | border-right-color: #51483d; | |||
|
103 | } | |||
|
104 | ||||
|
105 | .reveal .controls div.right, | |||
|
106 | .reveal .controls div.right.enabled { | |||
|
107 | border-left-color: #51483d; | |||
|
108 | } | |||
|
109 | ||||
|
110 | .reveal .controls div.up, | |||
|
111 | .reveal .controls div.up.enabled { | |||
|
112 | border-bottom-color: #51483d; | |||
|
113 | } | |||
|
114 | ||||
|
115 | .reveal .controls div.down, | |||
|
116 | .reveal .controls div.down.enabled { | |||
|
117 | border-top-color: #51483d; | |||
|
118 | } | |||
|
119 | ||||
|
120 | .reveal .controls div.left.enabled:hover { | |||
|
121 | border-right-color: #8b7c69; | |||
|
122 | } | |||
|
123 | ||||
|
124 | .reveal .controls div.right.enabled:hover { | |||
|
125 | border-left-color: #8b7c69; | |||
|
126 | } | |||
|
127 | ||||
|
128 | .reveal .controls div.up.enabled:hover { | |||
|
129 | border-bottom-color: #8b7c69; | |||
|
130 | } | |||
|
131 | ||||
|
132 | .reveal .controls div.down.enabled:hover { | |||
|
133 | border-top-color: #8b7c69; | |||
|
134 | } | |||
|
135 | ||||
|
136 | /********************************************* | |||
|
137 | * PROGRESS BAR | |||
|
138 | *********************************************/ | |||
|
139 | .reveal .progress { | |||
|
140 | background: rgba(0, 0, 0, 0.2); | |||
|
141 | } | |||
|
142 | ||||
|
143 | .reveal .progress span { | |||
|
144 | background: #51483d; | |||
|
145 | -webkit-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
146 | -moz-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
147 | -ms-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
148 | -o-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
149 | transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
150 | } |
@@ -0,0 +1,152 b'' | |||||
|
1 | @import url(http://fonts.googleapis.com/css?family=News+Cycle:400,700); | |||
|
2 | @import url(http://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic); | |||
|
3 | /** | |||
|
4 | * A simple theme for reveal.js presentations, similar | |||
|
5 | * to the default theme. The accent color is darkblue. | |||
|
6 | * | |||
|
7 | * This theme is Copyright (C) 2012 Owen Versteeg, https://github.com/StereotypicalApps. It is MIT licensed. | |||
|
8 | * reveal.js is Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
9 | */ | |||
|
10 | /********************************************* | |||
|
11 | * GLOBAL STYLES | |||
|
12 | *********************************************/ | |||
|
13 | body { | |||
|
14 | background: white; | |||
|
15 | background-color: white; | |||
|
16 | } | |||
|
17 | ||||
|
18 | .reveal { | |||
|
19 | font-family: "Lato", Times, "Times New Roman", serif; | |||
|
20 | font-size: 36px; | |||
|
21 | font-weight: 200; | |||
|
22 | letter-spacing: -0.02em; | |||
|
23 | color: black; | |||
|
24 | } | |||
|
25 | ||||
|
26 | ::selection { | |||
|
27 | color: white; | |||
|
28 | background: rgba(0, 0, 0, 0.99); | |||
|
29 | text-shadow: none; | |||
|
30 | } | |||
|
31 | ||||
|
32 | /********************************************* | |||
|
33 | * HEADERS | |||
|
34 | *********************************************/ | |||
|
35 | .reveal h1, | |||
|
36 | .reveal h2, | |||
|
37 | .reveal h3, | |||
|
38 | .reveal h4, | |||
|
39 | .reveal h5, | |||
|
40 | .reveal h6 { | |||
|
41 | margin: 0 0 20px 0; | |||
|
42 | color: black; | |||
|
43 | font-family: "News Cycle", Impact, sans-serif; | |||
|
44 | line-height: 0.9em; | |||
|
45 | letter-spacing: 0.02em; | |||
|
46 | text-transform: none; | |||
|
47 | text-shadow: none; | |||
|
48 | } | |||
|
49 | ||||
|
50 | .reveal h1 { | |||
|
51 | text-shadow: 0px 0px 6px rgba(0, 0, 0, 0.2); | |||
|
52 | } | |||
|
53 | ||||
|
54 | /********************************************* | |||
|
55 | * LINKS | |||
|
56 | *********************************************/ | |||
|
57 | .reveal a:not(.image) { | |||
|
58 | color: darkblue; | |||
|
59 | text-decoration: none; | |||
|
60 | -webkit-transition: color .15s ease; | |||
|
61 | -moz-transition: color .15s ease; | |||
|
62 | -ms-transition: color .15s ease; | |||
|
63 | -o-transition: color .15s ease; | |||
|
64 | transition: color .15s ease; | |||
|
65 | } | |||
|
66 | ||||
|
67 | .reveal a:not(.image):hover { | |||
|
68 | color: #0000f1; | |||
|
69 | text-shadow: none; | |||
|
70 | border: none; | |||
|
71 | } | |||
|
72 | ||||
|
73 | .reveal .roll span:after { | |||
|
74 | color: #fff; | |||
|
75 | background: #00003f; | |||
|
76 | } | |||
|
77 | ||||
|
78 | /********************************************* | |||
|
79 | * IMAGES | |||
|
80 | *********************************************/ | |||
|
81 | .reveal section img { | |||
|
82 | margin: 15px; | |||
|
83 | background: rgba(255, 255, 255, 0.12); | |||
|
84 | border: 4px solid black; | |||
|
85 | box-shadow: 0 0 10px rgba(0, 0, 0, 0.15); | |||
|
86 | -webkit-transition: all .2s linear; | |||
|
87 | -moz-transition: all .2s linear; | |||
|
88 | -ms-transition: all .2s linear; | |||
|
89 | -o-transition: all .2s linear; | |||
|
90 | transition: all .2s linear; | |||
|
91 | } | |||
|
92 | ||||
|
93 | .reveal a:hover img { | |||
|
94 | background: rgba(255, 255, 255, 0.2); | |||
|
95 | border-color: darkblue; | |||
|
96 | box-shadow: 0 0 20px rgba(0, 0, 0, 0.55); | |||
|
97 | } | |||
|
98 | ||||
|
99 | /********************************************* | |||
|
100 | * NAVIGATION CONTROLS | |||
|
101 | *********************************************/ | |||
|
102 | .reveal .controls div.left, | |||
|
103 | .reveal .controls div.left.enabled { | |||
|
104 | border-right-color: darkblue; | |||
|
105 | } | |||
|
106 | ||||
|
107 | .reveal .controls div.right, | |||
|
108 | .reveal .controls div.right.enabled { | |||
|
109 | border-left-color: darkblue; | |||
|
110 | } | |||
|
111 | ||||
|
112 | .reveal .controls div.up, | |||
|
113 | .reveal .controls div.up.enabled { | |||
|
114 | border-bottom-color: darkblue; | |||
|
115 | } | |||
|
116 | ||||
|
117 | .reveal .controls div.down, | |||
|
118 | .reveal .controls div.down.enabled { | |||
|
119 | border-top-color: darkblue; | |||
|
120 | } | |||
|
121 | ||||
|
122 | .reveal .controls div.left.enabled:hover { | |||
|
123 | border-right-color: #0000f1; | |||
|
124 | } | |||
|
125 | ||||
|
126 | .reveal .controls div.right.enabled:hover { | |||
|
127 | border-left-color: #0000f1; | |||
|
128 | } | |||
|
129 | ||||
|
130 | .reveal .controls div.up.enabled:hover { | |||
|
131 | border-bottom-color: #0000f1; | |||
|
132 | } | |||
|
133 | ||||
|
134 | .reveal .controls div.down.enabled:hover { | |||
|
135 | border-top-color: #0000f1; | |||
|
136 | } | |||
|
137 | ||||
|
138 | /********************************************* | |||
|
139 | * PROGRESS BAR | |||
|
140 | *********************************************/ | |||
|
141 | .reveal .progress { | |||
|
142 | background: rgba(0, 0, 0, 0.2); | |||
|
143 | } | |||
|
144 | ||||
|
145 | .reveal .progress span { | |||
|
146 | background: darkblue; | |||
|
147 | -webkit-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
148 | -moz-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
149 | -ms-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
150 | -o-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
151 | transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
152 | } |
@@ -0,0 +1,156 b'' | |||||
|
1 | @import url(http://fonts.googleapis.com/css?family=Quicksand:400,700,400italic,700italic); | |||
|
2 | @import url(http://fonts.googleapis.com/css?family=Open+Sans:400italic,700italic,400,700); | |||
|
3 | /** | |||
|
4 | * Sky theme for reveal.js. | |||
|
5 | * | |||
|
6 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
7 | */ | |||
|
8 | /********************************************* | |||
|
9 | * GLOBAL STYLES | |||
|
10 | *********************************************/ | |||
|
11 | body { | |||
|
12 | background: #add9e4; | |||
|
13 | background: -moz-radial-gradient(center, circle cover, #f7fbfc 0%, #add9e4 100%); | |||
|
14 | background: -webkit-gradient(radial, center center, 0px, center center, 100%, color-stop(0%, #f7fbfc), color-stop(100%, #add9e4)); | |||
|
15 | background: -webkit-radial-gradient(center, circle cover, #f7fbfc 0%, #add9e4 100%); | |||
|
16 | background: -o-radial-gradient(center, circle cover, #f7fbfc 0%, #add9e4 100%); | |||
|
17 | background: -ms-radial-gradient(center, circle cover, #f7fbfc 0%, #add9e4 100%); | |||
|
18 | background: radial-gradient(center, circle cover, #f7fbfc 0%, #add9e4 100%); | |||
|
19 | background-color: #f7fbfc; | |||
|
20 | } | |||
|
21 | ||||
|
22 | .reveal { | |||
|
23 | font-family: "Open Sans", sans-serif; | |||
|
24 | font-size: 36px; | |||
|
25 | font-weight: 200; | |||
|
26 | letter-spacing: -0.02em; | |||
|
27 | color: #333333; | |||
|
28 | } | |||
|
29 | ||||
|
30 | ::selection { | |||
|
31 | color: white; | |||
|
32 | background: #134674; | |||
|
33 | text-shadow: none; | |||
|
34 | } | |||
|
35 | ||||
|
36 | /********************************************* | |||
|
37 | * HEADERS | |||
|
38 | *********************************************/ | |||
|
39 | .reveal h1, | |||
|
40 | .reveal h2, | |||
|
41 | .reveal h3, | |||
|
42 | .reveal h4, | |||
|
43 | .reveal h5, | |||
|
44 | .reveal h6 { | |||
|
45 | margin: 0 0 20px 0; | |||
|
46 | color: #333333; | |||
|
47 | font-family: "Quicksand", sans-serif; | |||
|
48 | line-height: 0.9em; | |||
|
49 | letter-spacing: -0.08em; | |||
|
50 | text-transform: uppercase; | |||
|
51 | text-shadow: none; | |||
|
52 | } | |||
|
53 | ||||
|
54 | .reveal h1 { | |||
|
55 | text-shadow: 0px 0px 6px rgba(0, 0, 0, 0.2); | |||
|
56 | } | |||
|
57 | ||||
|
58 | /********************************************* | |||
|
59 | * LINKS | |||
|
60 | *********************************************/ | |||
|
61 | .reveal a:not(.image) { | |||
|
62 | color: #3b759e; | |||
|
63 | text-decoration: none; | |||
|
64 | -webkit-transition: color .15s ease; | |||
|
65 | -moz-transition: color .15s ease; | |||
|
66 | -ms-transition: color .15s ease; | |||
|
67 | -o-transition: color .15s ease; | |||
|
68 | transition: color .15s ease; | |||
|
69 | } | |||
|
70 | ||||
|
71 | .reveal a:not(.image):hover { | |||
|
72 | color: #74a7cb; | |||
|
73 | text-shadow: none; | |||
|
74 | border: none; | |||
|
75 | } | |||
|
76 | ||||
|
77 | .reveal .roll span:after { | |||
|
78 | color: #fff; | |||
|
79 | background: #264c66; | |||
|
80 | } | |||
|
81 | ||||
|
82 | /********************************************* | |||
|
83 | * IMAGES | |||
|
84 | *********************************************/ | |||
|
85 | .reveal section img { | |||
|
86 | margin: 15px; | |||
|
87 | background: rgba(255, 255, 255, 0.12); | |||
|
88 | border: 4px solid #333333; | |||
|
89 | box-shadow: 0 0 10px rgba(0, 0, 0, 0.15); | |||
|
90 | -webkit-transition: all .2s linear; | |||
|
91 | -moz-transition: all .2s linear; | |||
|
92 | -ms-transition: all .2s linear; | |||
|
93 | -o-transition: all .2s linear; | |||
|
94 | transition: all .2s linear; | |||
|
95 | } | |||
|
96 | ||||
|
97 | .reveal a:hover img { | |||
|
98 | background: rgba(255, 255, 255, 0.2); | |||
|
99 | border-color: #3b759e; | |||
|
100 | box-shadow: 0 0 20px rgba(0, 0, 0, 0.55); | |||
|
101 | } | |||
|
102 | ||||
|
103 | /********************************************* | |||
|
104 | * NAVIGATION CONTROLS | |||
|
105 | *********************************************/ | |||
|
106 | .reveal .controls div.left, | |||
|
107 | .reveal .controls div.left.enabled { | |||
|
108 | border-right-color: #3b759e; | |||
|
109 | } | |||
|
110 | ||||
|
111 | .reveal .controls div.right, | |||
|
112 | .reveal .controls div.right.enabled { | |||
|
113 | border-left-color: #3b759e; | |||
|
114 | } | |||
|
115 | ||||
|
116 | .reveal .controls div.up, | |||
|
117 | .reveal .controls div.up.enabled { | |||
|
118 | border-bottom-color: #3b759e; | |||
|
119 | } | |||
|
120 | ||||
|
121 | .reveal .controls div.down, | |||
|
122 | .reveal .controls div.down.enabled { | |||
|
123 | border-top-color: #3b759e; | |||
|
124 | } | |||
|
125 | ||||
|
126 | .reveal .controls div.left.enabled:hover { | |||
|
127 | border-right-color: #74a7cb; | |||
|
128 | } | |||
|
129 | ||||
|
130 | .reveal .controls div.right.enabled:hover { | |||
|
131 | border-left-color: #74a7cb; | |||
|
132 | } | |||
|
133 | ||||
|
134 | .reveal .controls div.up.enabled:hover { | |||
|
135 | border-bottom-color: #74a7cb; | |||
|
136 | } | |||
|
137 | ||||
|
138 | .reveal .controls div.down.enabled:hover { | |||
|
139 | border-top-color: #74a7cb; | |||
|
140 | } | |||
|
141 | ||||
|
142 | /********************************************* | |||
|
143 | * PROGRESS BAR | |||
|
144 | *********************************************/ | |||
|
145 | .reveal .progress { | |||
|
146 | background: rgba(0, 0, 0, 0.2); | |||
|
147 | } | |||
|
148 | ||||
|
149 | .reveal .progress span { | |||
|
150 | background: #3b759e; | |||
|
151 | -webkit-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
152 | -moz-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
153 | -ms-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
154 | -o-transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
155 | transition: width 800ms cubic-bezier(0.26, 0.86, 0.44, 0.985); | |||
|
156 | } |
@@ -0,0 +1,50 b'' | |||||
|
1 | /** | |||
|
2 | * Beige theme for reveal.js. | |||
|
3 | * | |||
|
4 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
5 | */ | |||
|
6 | ||||
|
7 | ||||
|
8 | // Default mixins and settings ----------------- | |||
|
9 | @import "../template/mixins"; | |||
|
10 | @import "../template/settings"; | |||
|
11 | // --------------------------------------------- | |||
|
12 | ||||
|
13 | ||||
|
14 | ||||
|
15 | // Include theme-specific fonts | |||
|
16 | @font-face { | |||
|
17 | font-family: 'League Gothic'; | |||
|
18 | src: url('../../lib/font/league_gothic-webfont.eot'); | |||
|
19 | src: url('../../lib/font/league_gothic-webfont.eot?#iefix') format('embedded-opentype'), | |||
|
20 | url('../../lib/font/league_gothic-webfont.woff') format('woff'), | |||
|
21 | url('../../lib/font/league_gothic-webfont.ttf') format('truetype'), | |||
|
22 | url('../../lib/font/league_gothic-webfont.svg#LeagueGothicRegular') format('svg'); | |||
|
23 | ||||
|
24 | font-weight: normal; | |||
|
25 | font-style: normal; | |||
|
26 | } | |||
|
27 | ||||
|
28 | @import url(http://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic); | |||
|
29 | ||||
|
30 | ||||
|
31 | // Override theme settings (see ../template/settings.scss) | |||
|
32 | $mainColor: #333; | |||
|
33 | $headingColor: #333; | |||
|
34 | $headingTextShadow: none; | |||
|
35 | $backgroundColor: #f7f3de; | |||
|
36 | $linkColor: #8b743d; | |||
|
37 | $linkColorHover: lighten( $linkColor, 20% ); | |||
|
38 | $selectionBackgroundColor: rgba(79, 64, 28, 0.99); | |||
|
39 | $heading1TextShadow: 0 1px 0 #ccc, 0 2px 0 #c9c9c9, 0 3px 0 #bbb, 0 4px 0 #b9b9b9, 0 5px 0 #aaa, 0 6px 1px rgba(0,0,0,.1), 0 0 5px rgba(0,0,0,.1), 0 1px 3px rgba(0,0,0,.3), 0 3px 5px rgba(0,0,0,.2), 0 5px 10px rgba(0,0,0,.25), 0 20px 20px rgba(0,0,0,.15); | |||
|
40 | ||||
|
41 | // Background generator | |||
|
42 | @mixin bodyBackground() { | |||
|
43 | @include radial-gradient( rgba(247,242,211,1), rgba(255,255,255,1) ); | |||
|
44 | } | |||
|
45 | ||||
|
46 | ||||
|
47 | ||||
|
48 | // Theme template ------------------------------ | |||
|
49 | @import "../template/theme"; | |||
|
50 | // --------------------------------------------- No newline at end of file |
@@ -0,0 +1,42 b'' | |||||
|
1 | /** | |||
|
2 | * Default theme for reveal.js. | |||
|
3 | * | |||
|
4 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
5 | */ | |||
|
6 | ||||
|
7 | ||||
|
8 | // Default mixins and settings ----------------- | |||
|
9 | @import "../template/mixins"; | |||
|
10 | @import "../template/settings"; | |||
|
11 | // --------------------------------------------- | |||
|
12 | ||||
|
13 | ||||
|
14 | ||||
|
15 | // Include theme-specific fonts | |||
|
16 | @font-face { | |||
|
17 | font-family: 'League Gothic'; | |||
|
18 | src: url('../../lib/font/league_gothic-webfont.eot'); | |||
|
19 | src: url('../../lib/font/league_gothic-webfont.eot?#iefix') format('embedded-opentype'), | |||
|
20 | url('../../lib/font/league_gothic-webfont.woff') format('woff'), | |||
|
21 | url('../../lib/font/league_gothic-webfont.ttf') format('truetype'), | |||
|
22 | url('../../lib/font/league_gothic-webfont.svg#LeagueGothicRegular') format('svg'); | |||
|
23 | ||||
|
24 | font-weight: normal; | |||
|
25 | font-style: normal; | |||
|
26 | } | |||
|
27 | ||||
|
28 | @import url(http://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic); | |||
|
29 | ||||
|
30 | // Override theme settings (see ../template/settings.scss) | |||
|
31 | $heading1TextShadow: 0 1px 0 #ccc, 0 2px 0 #c9c9c9, 0 3px 0 #bbb, 0 4px 0 #b9b9b9, 0 5px 0 #aaa, 0 6px 1px rgba(0,0,0,.1), 0 0 5px rgba(0,0,0,.1), 0 1px 3px rgba(0,0,0,.3), 0 3px 5px rgba(0,0,0,.2), 0 5px 10px rgba(0,0,0,.25), 0 20px 20px rgba(0,0,0,.15); | |||
|
32 | ||||
|
33 | // Background generator | |||
|
34 | @mixin bodyBackground() { | |||
|
35 | @include radial-gradient( rgba(28,30,32,1), rgba(85,90,95,1) ); | |||
|
36 | } | |||
|
37 | ||||
|
38 | ||||
|
39 | ||||
|
40 | // Theme template ------------------------------ | |||
|
41 | @import "../template/theme"; | |||
|
42 | // --------------------------------------------- No newline at end of file |
@@ -0,0 +1,33 b'' | |||||
|
1 | /** | |||
|
2 | * A simple theme for reveal.js presentations, similar | |||
|
3 | * to the default theme. The accent color is darkblue. | |||
|
4 | * | |||
|
5 | * This theme is Copyright (C) 2012 Owen Versteeg, https://github.com/StereotypicalApps. It is MIT licensed. | |||
|
6 | * reveal.js is Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se; so is the theme - beige.css - that this is based off of. | |||
|
7 | */ | |||
|
8 | ||||
|
9 | ||||
|
10 | // Default mixins and settings ----------------- | |||
|
11 | @import "../template/mixins"; | |||
|
12 | @import "../template/settings"; | |||
|
13 | // --------------------------------------------- | |||
|
14 | ||||
|
15 | ||||
|
16 | ||||
|
17 | // Override theme settings (see ../template/settings.scss) | |||
|
18 | $mainFont: 'Palatino Linotype', 'Book Antiqua', Palatino, FreeSerif, serif; | |||
|
19 | $mainColor: #000; | |||
|
20 | $headingFont: 'Palatino Linotype', 'Book Antiqua', Palatino, FreeSerif, serif; | |||
|
21 | $headingColor: #383D3D; | |||
|
22 | $headingTextShadow: none; | |||
|
23 | $headingTextTransform: none; | |||
|
24 | $backgroundColor: #F0F1EB; | |||
|
25 | $linkColor: #51483D; | |||
|
26 | $linkColorHover: lighten( $linkColor, 20% ); | |||
|
27 | $selectionBackgroundColor: #26351C; | |||
|
28 | ||||
|
29 | ||||
|
30 | ||||
|
31 | // Theme template ------------------------------ | |||
|
32 | @import "../template/theme"; | |||
|
33 | // --------------------------------------------- No newline at end of file |
@@ -0,0 +1,38 b'' | |||||
|
1 | /** | |||
|
2 | * A simple theme for reveal.js presentations, similar | |||
|
3 | * to the default theme. The accent color is darkblue. | |||
|
4 | * | |||
|
5 | * This theme is Copyright (C) 2012 Owen Versteeg, https://github.com/StereotypicalApps. It is MIT licensed. | |||
|
6 | * reveal.js is Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
7 | */ | |||
|
8 | ||||
|
9 | ||||
|
10 | // Default mixins and settings ----------------- | |||
|
11 | @import "../template/mixins"; | |||
|
12 | @import "../template/settings"; | |||
|
13 | // --------------------------------------------- | |||
|
14 | ||||
|
15 | ||||
|
16 | ||||
|
17 | // Include theme-specific fonts | |||
|
18 | @import url(http://fonts.googleapis.com/css?family=News+Cycle:400,700); | |||
|
19 | @import url(http://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic); | |||
|
20 | ||||
|
21 | ||||
|
22 | // Override theme settings (see ../template/settings.scss) | |||
|
23 | $mainFont: 'Lato', Times, 'Times New Roman', serif; | |||
|
24 | $mainColor: #000; | |||
|
25 | $headingFont: 'News Cycle', Impact, sans-serif; | |||
|
26 | $headingColor: #000; | |||
|
27 | $headingTextShadow: none; | |||
|
28 | $headingTextTransform: none; | |||
|
29 | $backgroundColor: #fff; | |||
|
30 | $linkColor: #00008B; | |||
|
31 | $linkColorHover: lighten( $linkColor, 20% ); | |||
|
32 | $selectionBackgroundColor: rgba(0, 0, 0, 0.99); | |||
|
33 | ||||
|
34 | ||||
|
35 | ||||
|
36 | // Theme template ------------------------------ | |||
|
37 | @import "../template/theme"; | |||
|
38 | // --------------------------------------------- No newline at end of file |
@@ -0,0 +1,41 b'' | |||||
|
1 | /** | |||
|
2 | * Sky theme for reveal.js. | |||
|
3 | * | |||
|
4 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
5 | */ | |||
|
6 | ||||
|
7 | ||||
|
8 | // Default mixins and settings ----------------- | |||
|
9 | @import "../template/mixins"; | |||
|
10 | @import "../template/settings"; | |||
|
11 | // --------------------------------------------- | |||
|
12 | ||||
|
13 | ||||
|
14 | ||||
|
15 | // Include theme-specific fonts | |||
|
16 | @import url(http://fonts.googleapis.com/css?family=Quicksand:400,700,400italic,700italic); | |||
|
17 | @import url(http://fonts.googleapis.com/css?family=Open+Sans:400italic,700italic,400,700); | |||
|
18 | ||||
|
19 | ||||
|
20 | // Override theme settings (see ../template/settings.scss) | |||
|
21 | $mainFont: 'Open Sans', sans-serif; | |||
|
22 | $mainColor: #333; | |||
|
23 | $headingFont: 'Quicksand', sans-serif; | |||
|
24 | $headingColor: #333; | |||
|
25 | $headingLetterSpacing: -0.08em; | |||
|
26 | $headingTextShadow: none; | |||
|
27 | $backgroundColor: #f7fbfc; | |||
|
28 | $linkColor: #3b759e; | |||
|
29 | $linkColorHover: lighten( $linkColor, 20% ); | |||
|
30 | $selectionBackgroundColor: #134674; | |||
|
31 | ||||
|
32 | // Background generator | |||
|
33 | @mixin bodyBackground() { | |||
|
34 | @include radial-gradient( #add9e4, #f7fbfc ); | |||
|
35 | } | |||
|
36 | ||||
|
37 | ||||
|
38 | ||||
|
39 | // Theme template ------------------------------ | |||
|
40 | @import "../template/theme"; | |||
|
41 | // --------------------------------------------- No newline at end of file |
@@ -0,0 +1,29 b'' | |||||
|
1 | @mixin vertical-gradient( $top, $bottom ) { | |||
|
2 | background: $top; | |||
|
3 | background: -moz-linear-gradient( top, $top 0%, $bottom 100% ); | |||
|
4 | background: -webkit-gradient( linear, left top, left bottom, color-stop(0%,$top), color-stop(100%,$bottom) ); | |||
|
5 | background: -webkit-linear-gradient( top, $top 0%, $bottom 100% ); | |||
|
6 | background: -o-linear-gradient( top, $top 0%, $bottom 100% ); | |||
|
7 | background: -ms-linear-gradient( top, $top 0%, $bottom 100% ); | |||
|
8 | background: linear-gradient( top, $top 0%, $bottom 100% ); | |||
|
9 | } | |||
|
10 | ||||
|
11 | @mixin horizontal-gradient( $top, $bottom ) { | |||
|
12 | background: $top; | |||
|
13 | background: -moz-linear-gradient( left, $top 0%, $bottom 100% ); | |||
|
14 | background: -webkit-gradient( linear, left top, right top, color-stop(0%,$top), color-stop(100%,$bottom) ); | |||
|
15 | background: -webkit-linear-gradient( left, $top 0%, $bottom 100% ); | |||
|
16 | background: -o-linear-gradient( left, $top 0%, $bottom 100% ); | |||
|
17 | background: -ms-linear-gradient( left, $top 0%, $bottom 100% ); | |||
|
18 | background: linear-gradient( left, $top 0%, $bottom 100% ); | |||
|
19 | } | |||
|
20 | ||||
|
21 | @mixin radial-gradient( $outer, $inner, $type: circle ) { | |||
|
22 | background: $outer; | |||
|
23 | background: -moz-radial-gradient( center, $type cover, $inner 0%, $outer 100% ); | |||
|
24 | background: -webkit-gradient( radial, center center, 0px, center center, 100%, color-stop(0%,$inner), color-stop(100%,$outer) ); | |||
|
25 | background: -webkit-radial-gradient( center, $type cover, $inner 0%, $outer 100% ); | |||
|
26 | background: -o-radial-gradient( center, $type cover, $inner 0%, $outer 100% ); | |||
|
27 | background: -ms-radial-gradient( center, $type cover, $inner 0%, $outer 100% ); | |||
|
28 | background: radial-gradient( center, $type cover, $inner 0%, $outer 100% ); | |||
|
29 | } No newline at end of file |
@@ -0,0 +1,33 b'' | |||||
|
1 | // Base settings for all themes that can optionally be | |||
|
2 | // overridden by the super-theme | |||
|
3 | ||||
|
4 | // Background of the presentation | |||
|
5 | $backgroundColor: #2b2b2b; | |||
|
6 | ||||
|
7 | // Primary/body text | |||
|
8 | $mainFont: 'Lato', Times, 'Times New Roman', serif; | |||
|
9 | $mainFontSize: 36px; | |||
|
10 | $mainColor: #eee; | |||
|
11 | ||||
|
12 | // Headings | |||
|
13 | $headingFont: 'League Gothic', Impact, sans-serif; | |||
|
14 | $headingColor: #eee; | |||
|
15 | $headingLineHeight: 0.9em; | |||
|
16 | $headingLetterSpacing: 0.02em; | |||
|
17 | $headingTextTransform: uppercase; | |||
|
18 | $headingTextShadow: 0px 0px 6px rgba(0,0,0,0.2); | |||
|
19 | $heading1TextShadow: $headingTextShadow; | |||
|
20 | ||||
|
21 | // Links and actions | |||
|
22 | $linkColor: #13DAEC; | |||
|
23 | $linkColorHover: lighten( $linkColor, 20% ); | |||
|
24 | ||||
|
25 | // Text selection | |||
|
26 | $selectionBackgroundColor: #FF5E99; | |||
|
27 | $selectionColor: #fff; | |||
|
28 | ||||
|
29 | // Generates the presentation background, can be overridden | |||
|
30 | // to return a background image or gradient | |||
|
31 | @mixin bodyBackground() { | |||
|
32 | background: $backgroundColor; | |||
|
33 | } No newline at end of file |
@@ -0,0 +1,163 b'' | |||||
|
1 | // Base theme template for reveal.js | |||
|
2 | ||||
|
3 | /********************************************* | |||
|
4 | * GLOBAL STYLES | |||
|
5 | *********************************************/ | |||
|
6 | ||||
|
7 | body { | |||
|
8 | @include bodyBackground(); | |||
|
9 | background-color: $backgroundColor; | |||
|
10 | } | |||
|
11 | ||||
|
12 | .reveal { | |||
|
13 | font-family: $mainFont; | |||
|
14 | font-size: $mainFontSize; | |||
|
15 | font-weight: 200; | |||
|
16 | letter-spacing: -0.02em; | |||
|
17 | color: $mainColor; | |||
|
18 | } | |||
|
19 | ||||
|
20 | ::selection { | |||
|
21 | color: $selectionColor; | |||
|
22 | background: $selectionBackgroundColor; | |||
|
23 | text-shadow: none; | |||
|
24 | } | |||
|
25 | ||||
|
26 | /********************************************* | |||
|
27 | * HEADERS | |||
|
28 | *********************************************/ | |||
|
29 | ||||
|
30 | .reveal h1, | |||
|
31 | .reveal h2, | |||
|
32 | .reveal h3, | |||
|
33 | .reveal h4, | |||
|
34 | .reveal h5, | |||
|
35 | .reveal h6 { | |||
|
36 | margin: 0 0 20px 0; | |||
|
37 | color: $headingColor; | |||
|
38 | ||||
|
39 | font-family: $headingFont; | |||
|
40 | line-height: $headingLineHeight; | |||
|
41 | letter-spacing: $headingLetterSpacing; | |||
|
42 | ||||
|
43 | text-transform: $headingTextTransform; | |||
|
44 | text-shadow: $headingTextShadow; | |||
|
45 | } | |||
|
46 | ||||
|
47 | .reveal h1 { | |||
|
48 | text-shadow: $heading1TextShadow; | |||
|
49 | } | |||
|
50 | ||||
|
51 | ||||
|
52 | /********************************************* | |||
|
53 | * LINKS | |||
|
54 | *********************************************/ | |||
|
55 | ||||
|
56 | .reveal a:not(.image) { | |||
|
57 | color: $linkColor; | |||
|
58 | text-decoration: none; | |||
|
59 | ||||
|
60 | -webkit-transition: color .15s ease; | |||
|
61 | -moz-transition: color .15s ease; | |||
|
62 | -ms-transition: color .15s ease; | |||
|
63 | -o-transition: color .15s ease; | |||
|
64 | transition: color .15s ease; | |||
|
65 | } | |||
|
66 | .reveal a:not(.image):hover { | |||
|
67 | color: $linkColorHover; | |||
|
68 | ||||
|
69 | text-shadow: none; | |||
|
70 | border: none; | |||
|
71 | } | |||
|
72 | ||||
|
73 | .reveal .roll span:after { | |||
|
74 | color: #fff; | |||
|
75 | background: darken( $linkColor, 15% ); | |||
|
76 | } | |||
|
77 | ||||
|
78 | ||||
|
79 | /********************************************* | |||
|
80 | * IMAGES | |||
|
81 | *********************************************/ | |||
|
82 | ||||
|
83 | .reveal section img { | |||
|
84 | margin: 15px; | |||
|
85 | background: rgba(255,255,255,0.12); | |||
|
86 | border: 4px solid $mainColor; | |||
|
87 | ||||
|
88 | box-shadow: 0 0 10px rgba(0, 0, 0, 0.15); | |||
|
89 | ||||
|
90 | -webkit-transition: all .2s linear; | |||
|
91 | -moz-transition: all .2s linear; | |||
|
92 | -ms-transition: all .2s linear; | |||
|
93 | -o-transition: all .2s linear; | |||
|
94 | transition: all .2s linear; | |||
|
95 | } | |||
|
96 | ||||
|
97 | .reveal a:hover img { | |||
|
98 | background: rgba(255,255,255,0.2); | |||
|
99 | border-color: $linkColor; | |||
|
100 | ||||
|
101 | box-shadow: 0 0 20px rgba(0, 0, 0, 0.55); | |||
|
102 | } | |||
|
103 | ||||
|
104 | ||||
|
105 | /********************************************* | |||
|
106 | * NAVIGATION CONTROLS | |||
|
107 | *********************************************/ | |||
|
108 | ||||
|
109 | .reveal .controls div.left, | |||
|
110 | .reveal .controls div.left.enabled { | |||
|
111 | border-right-color: $linkColor; | |||
|
112 | } | |||
|
113 | ||||
|
114 | .reveal .controls div.right, | |||
|
115 | .reveal .controls div.right.enabled { | |||
|
116 | border-left-color: $linkColor; | |||
|
117 | } | |||
|
118 | ||||
|
119 | .reveal .controls div.up, | |||
|
120 | .reveal .controls div.up.enabled { | |||
|
121 | border-bottom-color: $linkColor; | |||
|
122 | } | |||
|
123 | ||||
|
124 | .reveal .controls div.down, | |||
|
125 | .reveal .controls div.down.enabled { | |||
|
126 | border-top-color: $linkColor; | |||
|
127 | } | |||
|
128 | ||||
|
129 | .reveal .controls div.left.enabled:hover { | |||
|
130 | border-right-color: $linkColorHover; | |||
|
131 | } | |||
|
132 | ||||
|
133 | .reveal .controls div.right.enabled:hover { | |||
|
134 | border-left-color: $linkColorHover; | |||
|
135 | } | |||
|
136 | ||||
|
137 | .reveal .controls div.up.enabled:hover { | |||
|
138 | border-bottom-color: $linkColorHover; | |||
|
139 | } | |||
|
140 | ||||
|
141 | .reveal .controls div.down.enabled:hover { | |||
|
142 | border-top-color: $linkColorHover; | |||
|
143 | } | |||
|
144 | ||||
|
145 | ||||
|
146 | /********************************************* | |||
|
147 | * PROGRESS BAR | |||
|
148 | *********************************************/ | |||
|
149 | ||||
|
150 | .reveal .progress { | |||
|
151 | background: rgba(0,0,0,0.2); | |||
|
152 | } | |||
|
153 | .reveal .progress span { | |||
|
154 | background: $linkColor; | |||
|
155 | ||||
|
156 | -webkit-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
157 | -moz-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
158 | -ms-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
159 | -o-transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
160 | transition: width 800ms cubic-bezier(0.260, 0.860, 0.440, 0.985); | |||
|
161 | } | |||
|
162 | ||||
|
163 |
This diff has been collapsed as it changes many lines, (1385 lines changed) Show them Hide them | |||||
@@ -0,0 +1,1385 b'' | |||||
|
1 | /*! | |||
|
2 | * reveal.js 2.1 r37 | |||
|
3 | * http://lab.hakim.se/reveal-js | |||
|
4 | * MIT licensed | |||
|
5 | * | |||
|
6 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
7 | */ | |||
|
8 | var Reveal = (function(){ | |||
|
9 | ||||
|
10 | 'use strict'; | |||
|
11 | ||||
|
12 | var HORIZONTAL_SLIDES_SELECTOR = '.reveal .slides>section', | |||
|
13 | VERTICAL_SLIDES_SELECTOR = '.reveal .slides>section.present>section', | |||
|
14 | ||||
|
15 | // Configurations defaults, can be overridden at initialization time | |||
|
16 | config = { | |||
|
17 | // Display controls in the bottom right corner | |||
|
18 | controls: true, | |||
|
19 | ||||
|
20 | // Display a presentation progress bar | |||
|
21 | progress: true, | |||
|
22 | ||||
|
23 | // Push each slide change to the browser history | |||
|
24 | history: false, | |||
|
25 | ||||
|
26 | // Enable keyboard shortcuts for navigation | |||
|
27 | keyboard: true, | |||
|
28 | ||||
|
29 | // Enable the slide overview mode | |||
|
30 | overview: true, | |||
|
31 | ||||
|
32 | // Loop the presentation | |||
|
33 | loop: false, | |||
|
34 | ||||
|
35 | // Number of milliseconds between automatically proceeding to the | |||
|
36 | // next slide, disabled when set to 0, this value can be overwritten | |||
|
37 | // by using a data-autoslide attribute on your slides | |||
|
38 | autoSlide: 0, | |||
|
39 | ||||
|
40 | // Enable slide navigation via mouse wheel | |||
|
41 | mouseWheel: true, | |||
|
42 | ||||
|
43 | // Apply a 3D roll to links on hover | |||
|
44 | rollingLinks: true, | |||
|
45 | ||||
|
46 | // Transition style (see /css/theme) | |||
|
47 | theme: null, | |||
|
48 | ||||
|
49 | // Transition style | |||
|
50 | transition: 'default', // default/cube/page/concave/zoom/linear/none | |||
|
51 | ||||
|
52 | // Script dependencies to load | |||
|
53 | dependencies: [] | |||
|
54 | }, | |||
|
55 | ||||
|
56 | // Stores if the next slide should be shown automatically | |||
|
57 | // after n milliseconds | |||
|
58 | autoSlide = config.autoSlide, | |||
|
59 | ||||
|
60 | // The horizontal and verical index of the currently active slide | |||
|
61 | indexh = 0, | |||
|
62 | indexv = 0, | |||
|
63 | ||||
|
64 | // The previous and current slide HTML elements | |||
|
65 | previousSlide, | |||
|
66 | currentSlide, | |||
|
67 | ||||
|
68 | // Slides may hold a data-state attribute which we pick up and apply | |||
|
69 | // as a class to the body. This list contains the combined state of | |||
|
70 | // all current slides. | |||
|
71 | state = [], | |||
|
72 | ||||
|
73 | // Cached references to DOM elements | |||
|
74 | dom = {}, | |||
|
75 | ||||
|
76 | // Detect support for CSS 3D transforms | |||
|
77 | supports3DTransforms = 'WebkitPerspective' in document.body.style || | |||
|
78 | 'MozPerspective' in document.body.style || | |||
|
79 | 'msPerspective' in document.body.style || | |||
|
80 | 'OPerspective' in document.body.style || | |||
|
81 | 'perspective' in document.body.style, | |||
|
82 | ||||
|
83 | supports2DTransforms = 'WebkitTransform' in document.body.style || | |||
|
84 | 'MozTransform' in document.body.style || | |||
|
85 | 'msTransform' in document.body.style || | |||
|
86 | 'OTransform' in document.body.style || | |||
|
87 | 'transform' in document.body.style, | |||
|
88 | ||||
|
89 | // Throttles mouse wheel navigation | |||
|
90 | mouseWheelTimeout = 0, | |||
|
91 | ||||
|
92 | // An interval used to automatically move on to the next slide | |||
|
93 | autoSlideTimeout = 0, | |||
|
94 | ||||
|
95 | // Delays updates to the URL due to a Chrome thumbnailer bug | |||
|
96 | writeURLTimeout = 0, | |||
|
97 | ||||
|
98 | // Holds information about the currently ongoing touch input | |||
|
99 | touch = { | |||
|
100 | startX: 0, | |||
|
101 | startY: 0, | |||
|
102 | startSpan: 0, | |||
|
103 | startCount: 0, | |||
|
104 | handled: false, | |||
|
105 | threshold: 80 | |||
|
106 | }; | |||
|
107 | ||||
|
108 | /** | |||
|
109 | * Starts up the presentation if the client is capable. | |||
|
110 | */ | |||
|
111 | function initialize( options ) { | |||
|
112 | if( ( !supports2DTransforms && !supports3DTransforms ) ) { | |||
|
113 | document.body.setAttribute( 'class', 'no-transforms' ); | |||
|
114 | ||||
|
115 | // If the browser doesn't support core features we won't be | |||
|
116 | // using JavaScript to control the presentation | |||
|
117 | return; | |||
|
118 | } | |||
|
119 | ||||
|
120 | // Copy options over to our config object | |||
|
121 | extend( config, options ); | |||
|
122 | ||||
|
123 | // Hide the address bar in mobile browsers | |||
|
124 | hideAddressBar(); | |||
|
125 | ||||
|
126 | // Loads the dependencies and continues to #start() once done | |||
|
127 | load(); | |||
|
128 | ||||
|
129 | } | |||
|
130 | ||||
|
131 | /** | |||
|
132 | * Finds and stores references to DOM elements which are | |||
|
133 | * required by the presentation. If a required element is | |||
|
134 | * not found, it is created. | |||
|
135 | */ | |||
|
136 | function setupDOM() { | |||
|
137 | // Cache references to key DOM elements | |||
|
138 | dom.theme = document.querySelector( '#theme' ); | |||
|
139 | dom.wrapper = document.querySelector( '.reveal' ); | |||
|
140 | ||||
|
141 | // Progress bar | |||
|
142 | if( !dom.wrapper.querySelector( '.progress' ) && config.progress ) { | |||
|
143 | var progressElement = document.createElement( 'div' ); | |||
|
144 | progressElement.classList.add( 'progress' ); | |||
|
145 | progressElement.innerHTML = '<span></span>'; | |||
|
146 | dom.wrapper.appendChild( progressElement ); | |||
|
147 | } | |||
|
148 | ||||
|
149 | // Arrow controls | |||
|
150 | if( !dom.wrapper.querySelector( '.controls' ) && config.controls ) { | |||
|
151 | var controlsElement = document.createElement( 'aside' ); | |||
|
152 | controlsElement.classList.add( 'controls' ); | |||
|
153 | controlsElement.innerHTML = '<div class="left"></div>' + | |||
|
154 | '<div class="right"></div>' + | |||
|
155 | '<div class="up"></div>' + | |||
|
156 | '<div class="down"></div>'; | |||
|
157 | dom.wrapper.appendChild( controlsElement ); | |||
|
158 | } | |||
|
159 | ||||
|
160 | // Presentation background element | |||
|
161 | if( !dom.wrapper.querySelector( '.state-background' ) ) { | |||
|
162 | var backgroundElement = document.createElement( 'div' ); | |||
|
163 | backgroundElement.classList.add( 'state-background' ); | |||
|
164 | dom.wrapper.appendChild( backgroundElement ); | |||
|
165 | } | |||
|
166 | ||||
|
167 | // Overlay graphic which is displayed during the paused mode | |||
|
168 | if( !dom.wrapper.querySelector( '.pause-overlay' ) ) { | |||
|
169 | var pausedElement = document.createElement( 'div' ); | |||
|
170 | pausedElement.classList.add( 'pause-overlay' ); | |||
|
171 | dom.wrapper.appendChild( pausedElement ); | |||
|
172 | } | |||
|
173 | ||||
|
174 | // Cache references to elements | |||
|
175 | dom.progress = document.querySelector( '.reveal .progress' ); | |||
|
176 | dom.progressbar = document.querySelector( '.reveal .progress span' ); | |||
|
177 | ||||
|
178 | if ( config.controls ) { | |||
|
179 | dom.controls = document.querySelector( '.reveal .controls' ); | |||
|
180 | dom.controlsLeft = document.querySelector( '.reveal .controls .left' ); | |||
|
181 | dom.controlsRight = document.querySelector( '.reveal .controls .right' ); | |||
|
182 | dom.controlsUp = document.querySelector( '.reveal .controls .up' ); | |||
|
183 | dom.controlsDown = document.querySelector( '.reveal .controls .down' ); | |||
|
184 | } | |||
|
185 | } | |||
|
186 | ||||
|
187 | /** | |||
|
188 | * Hides the address bar if we're on a mobile device. | |||
|
189 | */ | |||
|
190 | function hideAddressBar() { | |||
|
191 | if( navigator.userAgent.match( /(iphone|ipod|android)/i ) ) { | |||
|
192 | // Give the page some scrollable overflow | |||
|
193 | document.documentElement.style.overflow = 'scroll'; | |||
|
194 | document.body.style.height = '120%'; | |||
|
195 | ||||
|
196 | // Events that should trigger the address bar to hide | |||
|
197 | window.addEventListener( 'load', removeAddressBar, false ); | |||
|
198 | window.addEventListener( 'orientationchange', removeAddressBar, false ); | |||
|
199 | } | |||
|
200 | } | |||
|
201 | ||||
|
202 | /** | |||
|
203 | * Loads the dependencies of reveal.js. Dependencies are | |||
|
204 | * defined via the configuration option 'dependencies' | |||
|
205 | * and will be loaded prior to starting/binding reveal.js. | |||
|
206 | * Some dependencies may have an 'async' flag, if so they | |||
|
207 | * will load after reveal.js has been started up. | |||
|
208 | */ | |||
|
209 | function load() { | |||
|
210 | var scripts = [], | |||
|
211 | scriptsAsync = []; | |||
|
212 | ||||
|
213 | for( var i = 0, len = config.dependencies.length; i < len; i++ ) { | |||
|
214 | var s = config.dependencies[i]; | |||
|
215 | ||||
|
216 | // Load if there's no condition or the condition is truthy | |||
|
217 | if( !s.condition || s.condition() ) { | |||
|
218 | if( s.async ) { | |||
|
219 | scriptsAsync.push( s.src ); | |||
|
220 | } | |||
|
221 | else { | |||
|
222 | scripts.push( s.src ); | |||
|
223 | } | |||
|
224 | ||||
|
225 | // Extension may contain callback functions | |||
|
226 | if( typeof s.callback === 'function' ) { | |||
|
227 | head.ready( s.src.match( /([\w\d_\-]*)\.?[^\\\/]*$/i )[0], s.callback ); | |||
|
228 | } | |||
|
229 | } | |||
|
230 | } | |||
|
231 | ||||
|
232 | // Called once synchronous scritps finish loading | |||
|
233 | function proceed() { | |||
|
234 | if( scriptsAsync.length ) { | |||
|
235 | // Load asynchronous scripts | |||
|
236 | head.js.apply( null, scriptsAsync ); | |||
|
237 | } | |||
|
238 | ||||
|
239 | start(); | |||
|
240 | } | |||
|
241 | ||||
|
242 | if( scripts.length ) { | |||
|
243 | head.ready( proceed ); | |||
|
244 | ||||
|
245 | // Load synchronous scripts | |||
|
246 | head.js.apply( null, scripts ); | |||
|
247 | } | |||
|
248 | else { | |||
|
249 | proceed(); | |||
|
250 | } | |||
|
251 | } | |||
|
252 | ||||
|
253 | /** | |||
|
254 | * Starts up reveal.js by binding input events and navigating | |||
|
255 | * to the current URL deeplink if there is one. | |||
|
256 | */ | |||
|
257 | function start() { | |||
|
258 | // Make sure we've got all the DOM elements we need | |||
|
259 | setupDOM(); | |||
|
260 | ||||
|
261 | // Subscribe to input | |||
|
262 | addEventListeners(); | |||
|
263 | ||||
|
264 | // Updates the presentation to match the current configuration values | |||
|
265 | configure(); | |||
|
266 | ||||
|
267 | // Read the initial hash | |||
|
268 | readURL(); | |||
|
269 | ||||
|
270 | // Start auto-sliding if it's enabled | |||
|
271 | cueAutoSlide(); | |||
|
272 | ||||
|
273 | // Notify listeners that the presentation is ready but use a 1ms | |||
|
274 | // timeout to ensure it's not fired synchronously after #initialize() | |||
|
275 | setTimeout( function() { | |||
|
276 | dispatchEvent( 'ready', { | |||
|
277 | 'indexh': indexh, | |||
|
278 | 'indexv': indexv, | |||
|
279 | 'currentSlide': currentSlide | |||
|
280 | } ); | |||
|
281 | }, 1 ); | |||
|
282 | } | |||
|
283 | ||||
|
284 | /** | |||
|
285 | * Applies the configuration settings from the config object. | |||
|
286 | */ | |||
|
287 | function configure() { | |||
|
288 | if( supports3DTransforms === false ) { | |||
|
289 | config.transition = 'linear'; | |||
|
290 | } | |||
|
291 | ||||
|
292 | if( config.controls && dom.controls ) { | |||
|
293 | dom.controls.style.display = 'block'; | |||
|
294 | } | |||
|
295 | ||||
|
296 | if( config.progress && dom.progress ) { | |||
|
297 | dom.progress.style.display = 'block'; | |||
|
298 | } | |||
|
299 | ||||
|
300 | if( config.transition !== 'default' ) { | |||
|
301 | dom.wrapper.classList.add( config.transition ); | |||
|
302 | } | |||
|
303 | ||||
|
304 | if( config.mouseWheel ) { | |||
|
305 | document.addEventListener( 'DOMMouseScroll', onDocumentMouseScroll, false ); // FF | |||
|
306 | document.addEventListener( 'mousewheel', onDocumentMouseScroll, false ); | |||
|
307 | } | |||
|
308 | ||||
|
309 | // 3D links | |||
|
310 | if( config.rollingLinks ) { | |||
|
311 | linkify(); | |||
|
312 | } | |||
|
313 | ||||
|
314 | // Load the theme in the config, if it's not already loaded | |||
|
315 | if( config.theme && dom.theme ) { | |||
|
316 | var themeURL = dom.theme.getAttribute( 'href' ); | |||
|
317 | var themeFinder = /[^\/]*?(?=\.css)/; | |||
|
318 | var themeName = themeURL.match(themeFinder)[0]; | |||
|
319 | ||||
|
320 | if( config.theme !== themeName ) { | |||
|
321 | themeURL = themeURL.replace(themeFinder, config.theme); | |||
|
322 | dom.theme.setAttribute( 'href', themeURL ); | |||
|
323 | } | |||
|
324 | } | |||
|
325 | } | |||
|
326 | ||||
|
327 | /** | |||
|
328 | * Binds all event listeners. | |||
|
329 | */ | |||
|
330 | function addEventListeners() { | |||
|
331 | document.addEventListener( 'touchstart', onDocumentTouchStart, false ); | |||
|
332 | document.addEventListener( 'touchmove', onDocumentTouchMove, false ); | |||
|
333 | document.addEventListener( 'touchend', onDocumentTouchEnd, false ); | |||
|
334 | window.addEventListener( 'hashchange', onWindowHashChange, false ); | |||
|
335 | ||||
|
336 | if( config.keyboard ) { | |||
|
337 | document.addEventListener( 'keydown', onDocumentKeyDown, false ); | |||
|
338 | } | |||
|
339 | ||||
|
340 | if ( config.progress && dom.progress ) { | |||
|
341 | dom.progress.addEventListener( 'click', preventAndForward( onProgressClick ), false ); | |||
|
342 | } | |||
|
343 | ||||
|
344 | if ( config.controls && dom.controls ) { | |||
|
345 | dom.controlsLeft.addEventListener( 'click', preventAndForward( navigateLeft ), false ); | |||
|
346 | dom.controlsRight.addEventListener( 'click', preventAndForward( navigateRight ), false ); | |||
|
347 | dom.controlsUp.addEventListener( 'click', preventAndForward( navigateUp ), false ); | |||
|
348 | dom.controlsDown.addEventListener( 'click', preventAndForward( navigateDown ), false ); | |||
|
349 | } | |||
|
350 | } | |||
|
351 | ||||
|
352 | /** | |||
|
353 | * Unbinds all event listeners. | |||
|
354 | */ | |||
|
355 | function removeEventListeners() { | |||
|
356 | document.removeEventListener( 'keydown', onDocumentKeyDown, false ); | |||
|
357 | document.removeEventListener( 'touchstart', onDocumentTouchStart, false ); | |||
|
358 | document.removeEventListener( 'touchmove', onDocumentTouchMove, false ); | |||
|
359 | document.removeEventListener( 'touchend', onDocumentTouchEnd, false ); | |||
|
360 | window.removeEventListener( 'hashchange', onWindowHashChange, false ); | |||
|
361 | ||||
|
362 | if ( config.progress && dom.progress ) { | |||
|
363 | dom.progress.removeEventListener( 'click', preventAndForward( onProgressClick ), false ); | |||
|
364 | } | |||
|
365 | ||||
|
366 | if ( config.controls && dom.controls ) { | |||
|
367 | dom.controlsLeft.removeEventListener( 'click', preventAndForward( navigateLeft ), false ); | |||
|
368 | dom.controlsRight.removeEventListener( 'click', preventAndForward( navigateRight ), false ); | |||
|
369 | dom.controlsUp.removeEventListener( 'click', preventAndForward( navigateUp ), false ); | |||
|
370 | dom.controlsDown.removeEventListener( 'click', preventAndForward( navigateDown ), false ); | |||
|
371 | } | |||
|
372 | } | |||
|
373 | ||||
|
374 | /** | |||
|
375 | * Extend object a with the properties of object b. | |||
|
376 | * If there's a conflict, object b takes precedence. | |||
|
377 | */ | |||
|
378 | function extend( a, b ) { | |||
|
379 | for( var i in b ) { | |||
|
380 | a[ i ] = b[ i ]; | |||
|
381 | } | |||
|
382 | } | |||
|
383 | ||||
|
384 | /** | |||
|
385 | * Measures the distance in pixels between point a | |||
|
386 | * and point b. | |||
|
387 | * | |||
|
388 | * @param {Object} a point with x/y properties | |||
|
389 | * @param {Object} b point with x/y properties | |||
|
390 | */ | |||
|
391 | function distanceBetween( a, b ) { | |||
|
392 | var dx = a.x - b.x, | |||
|
393 | dy = a.y - b.y; | |||
|
394 | ||||
|
395 | return Math.sqrt( dx*dx + dy*dy ); | |||
|
396 | } | |||
|
397 | ||||
|
398 | /** | |||
|
399 | * Prevents an events defaults behavior calls the | |||
|
400 | * specified delegate. | |||
|
401 | * | |||
|
402 | * @param {Function} delegate The method to call | |||
|
403 | * after the wrapper has been executed | |||
|
404 | */ | |||
|
405 | function preventAndForward( delegate ) { | |||
|
406 | return function( event ) { | |||
|
407 | event.preventDefault(); | |||
|
408 | delegate.call( null, event ); | |||
|
409 | }; | |||
|
410 | } | |||
|
411 | ||||
|
412 | /** | |||
|
413 | * Causes the address bar to hide on mobile devices, | |||
|
414 | * more vertical space ftw. | |||
|
415 | */ | |||
|
416 | function removeAddressBar() { | |||
|
417 | setTimeout( function() { | |||
|
418 | window.scrollTo( 0, 1 ); | |||
|
419 | }, 0 ); | |||
|
420 | } | |||
|
421 | ||||
|
422 | /** | |||
|
423 | * Dispatches an event of the specified type from the | |||
|
424 | * reveal DOM element. | |||
|
425 | */ | |||
|
426 | function dispatchEvent( type, properties ) { | |||
|
427 | var event = document.createEvent( "HTMLEvents", 1, 2 ); | |||
|
428 | event.initEvent( type, true, true ); | |||
|
429 | extend( event, properties ); | |||
|
430 | dom.wrapper.dispatchEvent( event ); | |||
|
431 | } | |||
|
432 | ||||
|
433 | /** | |||
|
434 | * Wrap all links in 3D goodness. | |||
|
435 | */ | |||
|
436 | function linkify() { | |||
|
437 | if( supports3DTransforms && !( 'msPerspective' in document.body.style ) ) { | |||
|
438 | var nodes = document.querySelectorAll( '.reveal .slides section a:not(.image)' ); | |||
|
439 | ||||
|
440 | for( var i = 0, len = nodes.length; i < len; i++ ) { | |||
|
441 | var node = nodes[i]; | |||
|
442 | ||||
|
443 | if( node.textContent && !node.querySelector( 'img' ) && ( !node.className || !node.classList.contains( node, 'roll' ) ) ) { | |||
|
444 | node.classList.add( 'roll' ); | |||
|
445 | node.innerHTML = '<span data-title="'+ node.text +'">' + node.innerHTML + '</span>'; | |||
|
446 | } | |||
|
447 | } | |||
|
448 | } | |||
|
449 | } | |||
|
450 | ||||
|
451 | /** | |||
|
452 | * Displays the overview of slides (quick nav) by | |||
|
453 | * scaling down and arranging all slide elements. | |||
|
454 | * | |||
|
455 | * Experimental feature, might be dropped if perf | |||
|
456 | * can't be improved. | |||
|
457 | */ | |||
|
458 | function activateOverview() { | |||
|
459 | ||||
|
460 | // Only proceed if enabled in config | |||
|
461 | if( config.overview ) { | |||
|
462 | ||||
|
463 | dom.wrapper.classList.add( 'overview' ); | |||
|
464 | ||||
|
465 | var horizontalSlides = document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR ); | |||
|
466 | ||||
|
467 | for( var i = 0, len1 = horizontalSlides.length; i < len1; i++ ) { | |||
|
468 | var hslide = horizontalSlides[i], | |||
|
469 | htransform = 'translateZ(-2500px) translate(' + ( ( i - indexh ) * 105 ) + '%, 0%)'; | |||
|
470 | ||||
|
471 | hslide.setAttribute( 'data-index-h', i ); | |||
|
472 | hslide.style.display = 'block'; | |||
|
473 | hslide.style.WebkitTransform = htransform; | |||
|
474 | hslide.style.MozTransform = htransform; | |||
|
475 | hslide.style.msTransform = htransform; | |||
|
476 | hslide.style.OTransform = htransform; | |||
|
477 | hslide.style.transform = htransform; | |||
|
478 | ||||
|
479 | if( !hslide.classList.contains( 'stack' ) ) { | |||
|
480 | // Navigate to this slide on click | |||
|
481 | hslide.addEventListener( 'click', onOverviewSlideClicked, true ); | |||
|
482 | } | |||
|
483 | ||||
|
484 | var verticalSlides = hslide.querySelectorAll( 'section' ); | |||
|
485 | ||||
|
486 | for( var j = 0, len2 = verticalSlides.length; j < len2; j++ ) { | |||
|
487 | var vslide = verticalSlides[j], | |||
|
488 | vtransform = 'translate(0%, ' + ( ( j - ( i === indexh ? indexv : 0 ) ) * 105 ) + '%)'; | |||
|
489 | ||||
|
490 | vslide.setAttribute( 'data-index-h', i ); | |||
|
491 | vslide.setAttribute( 'data-index-v', j ); | |||
|
492 | vslide.style.display = 'block'; | |||
|
493 | vslide.style.WebkitTransform = vtransform; | |||
|
494 | vslide.style.MozTransform = vtransform; | |||
|
495 | vslide.style.msTransform = vtransform; | |||
|
496 | vslide.style.OTransform = vtransform; | |||
|
497 | vslide.style.transform = vtransform; | |||
|
498 | ||||
|
499 | // Navigate to this slide on click | |||
|
500 | vslide.addEventListener( 'click', onOverviewSlideClicked, true ); | |||
|
501 | } | |||
|
502 | ||||
|
503 | } | |||
|
504 | ||||
|
505 | } | |||
|
506 | ||||
|
507 | } | |||
|
508 | ||||
|
509 | /** | |||
|
510 | * Exits the slide overview and enters the currently | |||
|
511 | * active slide. | |||
|
512 | */ | |||
|
513 | function deactivateOverview() { | |||
|
514 | ||||
|
515 | // Only proceed if enabled in config | |||
|
516 | if( config.overview ) { | |||
|
517 | ||||
|
518 | dom.wrapper.classList.remove( 'overview' ); | |||
|
519 | ||||
|
520 | // Select all slides | |||
|
521 | var slides = Array.prototype.slice.call( document.querySelectorAll( '.reveal .slides section' ) ); | |||
|
522 | ||||
|
523 | for( var i = 0, len = slides.length; i < len; i++ ) { | |||
|
524 | var element = slides[i]; | |||
|
525 | ||||
|
526 | // Resets all transforms to use the external styles | |||
|
527 | element.style.WebkitTransform = ''; | |||
|
528 | element.style.MozTransform = ''; | |||
|
529 | element.style.msTransform = ''; | |||
|
530 | element.style.OTransform = ''; | |||
|
531 | element.style.transform = ''; | |||
|
532 | ||||
|
533 | element.removeEventListener( 'click', onOverviewSlideClicked ); | |||
|
534 | } | |||
|
535 | ||||
|
536 | slide(); | |||
|
537 | ||||
|
538 | } | |||
|
539 | } | |||
|
540 | ||||
|
541 | /** | |||
|
542 | * Toggles the slide overview mode on and off. | |||
|
543 | * | |||
|
544 | * @param {Boolean} override Optional flag which overrides the | |||
|
545 | * toggle logic and forcibly sets the desired state. True means | |||
|
546 | * overview is open, false means it's closed. | |||
|
547 | */ | |||
|
548 | function toggleOverview( override ) { | |||
|
549 | if( typeof override === 'boolean' ) { | |||
|
550 | override ? activateOverview() : deactivateOverview(); | |||
|
551 | } | |||
|
552 | else { | |||
|
553 | isOverviewActive() ? deactivateOverview() : activateOverview(); | |||
|
554 | } | |||
|
555 | } | |||
|
556 | ||||
|
557 | /** | |||
|
558 | * Checks if the overview is currently active. | |||
|
559 | * | |||
|
560 | * @return {Boolean} true if the overview is active, | |||
|
561 | * false otherwise | |||
|
562 | */ | |||
|
563 | function isOverviewActive() { | |||
|
564 | return dom.wrapper.classList.contains( 'overview' ); | |||
|
565 | } | |||
|
566 | ||||
|
567 | /** | |||
|
568 | * Handling the fullscreen functionality via the fullscreen API | |||
|
569 | * | |||
|
570 | * @see http://fullscreen.spec.whatwg.org/ | |||
|
571 | * @see https://developer.mozilla.org/en-US/docs/DOM/Using_fullscreen_mode | |||
|
572 | */ | |||
|
573 | function enterFullscreen() { | |||
|
574 | var element = document.body; | |||
|
575 | ||||
|
576 | // Check which implementation is available | |||
|
577 | var requestMethod = element.requestFullScreen || | |||
|
578 | element.webkitRequestFullScreen || | |||
|
579 | element.mozRequestFullScreen || | |||
|
580 | element.msRequestFullScreen; | |||
|
581 | ||||
|
582 | if( requestMethod ) { | |||
|
583 | requestMethod.apply( element ); | |||
|
584 | } | |||
|
585 | } | |||
|
586 | ||||
|
587 | /** | |||
|
588 | * Enters the paused mode which fades everything on screen to | |||
|
589 | * black. | |||
|
590 | */ | |||
|
591 | function pause() { | |||
|
592 | dom.wrapper.classList.add( 'paused' ); | |||
|
593 | } | |||
|
594 | ||||
|
595 | /** | |||
|
596 | * Exits from the paused mode. | |||
|
597 | */ | |||
|
598 | function resume() { | |||
|
599 | dom.wrapper.classList.remove( 'paused' ); | |||
|
600 | } | |||
|
601 | ||||
|
602 | /** | |||
|
603 | * Toggles the paused mode on and off. | |||
|
604 | */ | |||
|
605 | function togglePause() { | |||
|
606 | if( isPaused() ) { | |||
|
607 | resume(); | |||
|
608 | } | |||
|
609 | else { | |||
|
610 | pause(); | |||
|
611 | } | |||
|
612 | } | |||
|
613 | ||||
|
614 | /** | |||
|
615 | * Checks if we are currently in the paused mode. | |||
|
616 | */ | |||
|
617 | function isPaused() { | |||
|
618 | return dom.wrapper.classList.contains( 'paused' ); | |||
|
619 | } | |||
|
620 | ||||
|
621 | /** | |||
|
622 | * Steps from the current point in the presentation to the | |||
|
623 | * slide which matches the specified horizontal and vertical | |||
|
624 | * indices. | |||
|
625 | * | |||
|
626 | * @param {int} h Horizontal index of the target slide | |||
|
627 | * @param {int} v Vertical index of the target slide | |||
|
628 | */ | |||
|
629 | function slide( h, v ) { | |||
|
630 | // Remember where we were at before | |||
|
631 | previousSlide = currentSlide; | |||
|
632 | ||||
|
633 | // Remember the state before this slide | |||
|
634 | var stateBefore = state.concat(); | |||
|
635 | ||||
|
636 | // Reset the state array | |||
|
637 | state.length = 0; | |||
|
638 | ||||
|
639 | var indexhBefore = indexh, | |||
|
640 | indexvBefore = indexv; | |||
|
641 | ||||
|
642 | // Activate and transition to the new slide | |||
|
643 | indexh = updateSlides( HORIZONTAL_SLIDES_SELECTOR, h === undefined ? indexh : h ); | |||
|
644 | indexv = updateSlides( VERTICAL_SLIDES_SELECTOR, v === undefined ? indexv : v ); | |||
|
645 | ||||
|
646 | // Apply the new state | |||
|
647 | stateLoop: for( var i = 0, len = state.length; i < len; i++ ) { | |||
|
648 | // Check if this state existed on the previous slide. If it | |||
|
649 | // did, we will avoid adding it repeatedly. | |||
|
650 | for( var j = 0; j < stateBefore.length; j++ ) { | |||
|
651 | if( stateBefore[j] === state[i] ) { | |||
|
652 | stateBefore.splice( j, 1 ); | |||
|
653 | continue stateLoop; | |||
|
654 | } | |||
|
655 | } | |||
|
656 | ||||
|
657 | document.documentElement.classList.add( state[i] ); | |||
|
658 | ||||
|
659 | // Dispatch custom event matching the state's name | |||
|
660 | dispatchEvent( state[i] ); | |||
|
661 | } | |||
|
662 | ||||
|
663 | // Clean up the remaints of the previous state | |||
|
664 | while( stateBefore.length ) { | |||
|
665 | document.documentElement.classList.remove( stateBefore.pop() ); | |||
|
666 | } | |||
|
667 | ||||
|
668 | // Update progress if enabled | |||
|
669 | if( config.progress && dom.progress ) { | |||
|
670 | dom.progressbar.style.width = ( indexh / ( document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR ).length - 1 ) ) * window.innerWidth + 'px'; | |||
|
671 | } | |||
|
672 | ||||
|
673 | // If the overview is active, re-activate it to update positions | |||
|
674 | if( isOverviewActive() ) { | |||
|
675 | activateOverview(); | |||
|
676 | } | |||
|
677 | ||||
|
678 | updateControls(); | |||
|
679 | ||||
|
680 | // Update the URL hash after a delay since updating it mid-transition | |||
|
681 | // is likely to cause visual lag | |||
|
682 | clearTimeout( writeURLTimeout ); | |||
|
683 | writeURLTimeout = setTimeout( writeURL, 1500 ); | |||
|
684 | ||||
|
685 | // Query all horizontal slides in the deck | |||
|
686 | var horizontalSlides = document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR ); | |||
|
687 | ||||
|
688 | // Find the current horizontal slide and any possible vertical slides | |||
|
689 | // within it | |||
|
690 | var currentHorizontalSlide = horizontalSlides[ indexh ], | |||
|
691 | currentVerticalSlides = currentHorizontalSlide.querySelectorAll( 'section' ); | |||
|
692 | ||||
|
693 | // Store references to the previous and current slides | |||
|
694 | currentSlide = currentVerticalSlides[ indexv ] || currentHorizontalSlide; | |||
|
695 | ||||
|
696 | // Dispatch an event if the slide changed | |||
|
697 | if( indexh !== indexhBefore || indexv !== indexvBefore ) { | |||
|
698 | dispatchEvent( 'slidechanged', { | |||
|
699 | 'indexh': indexh, | |||
|
700 | 'indexv': indexv, | |||
|
701 | 'previousSlide': previousSlide, | |||
|
702 | 'currentSlide': currentSlide | |||
|
703 | } ); | |||
|
704 | } | |||
|
705 | else { | |||
|
706 | // Ensure that the previous slide is never the same as the current | |||
|
707 | previousSlide = null; | |||
|
708 | } | |||
|
709 | ||||
|
710 | // Solves an edge case where the previous slide maintains the | |||
|
711 | // 'present' class when navigating between adjacent vertical | |||
|
712 | // stacks | |||
|
713 | if( previousSlide ) { | |||
|
714 | previousSlide.classList.remove( 'present' ); | |||
|
715 | } | |||
|
716 | } | |||
|
717 | ||||
|
718 | /** | |||
|
719 | * Updates one dimension of slides by showing the slide | |||
|
720 | * with the specified index. | |||
|
721 | * | |||
|
722 | * @param {String} selector A CSS selector that will fetch | |||
|
723 | * the group of slides we are working with | |||
|
724 | * @param {Number} index The index of the slide that should be | |||
|
725 | * shown | |||
|
726 | * | |||
|
727 | * @return {Number} The index of the slide that is now shown, | |||
|
728 | * might differ from the passed in index if it was out of | |||
|
729 | * bounds. | |||
|
730 | */ | |||
|
731 | function updateSlides( selector, index ) { | |||
|
732 | // Select all slides and convert the NodeList result to | |||
|
733 | // an array | |||
|
734 | var slides = Array.prototype.slice.call( document.querySelectorAll( selector ) ), | |||
|
735 | slidesLength = slides.length; | |||
|
736 | ||||
|
737 | if( slidesLength ) { | |||
|
738 | ||||
|
739 | // Should the index loop? | |||
|
740 | if( config.loop ) { | |||
|
741 | index %= slidesLength; | |||
|
742 | ||||
|
743 | if( index < 0 ) { | |||
|
744 | index = slidesLength + index; | |||
|
745 | } | |||
|
746 | } | |||
|
747 | ||||
|
748 | // Enforce max and minimum index bounds | |||
|
749 | index = Math.max( Math.min( index, slidesLength - 1 ), 0 ); | |||
|
750 | ||||
|
751 | for( var i = 0; i < slidesLength; i++ ) { | |||
|
752 | var element = slides[i]; | |||
|
753 | ||||
|
754 | // Optimization; hide all slides that are three or more steps | |||
|
755 | // away from the present slide | |||
|
756 | if( isOverviewActive() === false ) { | |||
|
757 | // The distance loops so that it measures 1 between the first | |||
|
758 | // and last slides | |||
|
759 | var distance = Math.abs( ( index - i ) % ( slidesLength - 3 ) ) || 0; | |||
|
760 | ||||
|
761 | element.style.display = distance > 3 ? 'none' : 'block'; | |||
|
762 | } | |||
|
763 | ||||
|
764 | slides[i].classList.remove( 'past' ); | |||
|
765 | slides[i].classList.remove( 'present' ); | |||
|
766 | slides[i].classList.remove( 'future' ); | |||
|
767 | ||||
|
768 | if( i < index ) { | |||
|
769 | // Any element previous to index is given the 'past' class | |||
|
770 | slides[i].classList.add( 'past' ); | |||
|
771 | } | |||
|
772 | else if( i > index ) { | |||
|
773 | // Any element subsequent to index is given the 'future' class | |||
|
774 | slides[i].classList.add( 'future' ); | |||
|
775 | } | |||
|
776 | ||||
|
777 | // If this element contains vertical slides | |||
|
778 | if( element.querySelector( 'section' ) ) { | |||
|
779 | slides[i].classList.add( 'stack' ); | |||
|
780 | } | |||
|
781 | } | |||
|
782 | ||||
|
783 | // Mark the current slide as present | |||
|
784 | slides[index].classList.add( 'present' ); | |||
|
785 | ||||
|
786 | // If this slide has a state associated with it, add it | |||
|
787 | // onto the current state of the deck | |||
|
788 | var slideState = slides[index].getAttribute( 'data-state' ); | |||
|
789 | if( slideState ) { | |||
|
790 | state = state.concat( slideState.split( ' ' ) ); | |||
|
791 | } | |||
|
792 | ||||
|
793 | // If this slide has a data-autoslide attribtue associated use this as | |||
|
794 | // autoSlide value otherwise use the global configured time | |||
|
795 | var slideAutoSlide = slides[index].getAttribute( 'data-autoslide' ); | |||
|
796 | if( slideAutoSlide ) { | |||
|
797 | autoSlide = parseInt( slideAutoSlide ); | |||
|
798 | } else { | |||
|
799 | autoSlide = config.autoSlide | |||
|
800 | } | |||
|
801 | ||||
|
802 | } | |||
|
803 | else { | |||
|
804 | // Since there are no slides we can't be anywhere beyond the | |||
|
805 | // zeroth index | |||
|
806 | index = 0; | |||
|
807 | } | |||
|
808 | ||||
|
809 | return index; | |||
|
810 | ||||
|
811 | } | |||
|
812 | ||||
|
813 | /** | |||
|
814 | * Updates the state and link pointers of the controls. | |||
|
815 | */ | |||
|
816 | function updateControls() { | |||
|
817 | if ( config.controls && dom.controls ) { | |||
|
818 | ||||
|
819 | var routes = availableRoutes(); | |||
|
820 | ||||
|
821 | // Remove the 'enabled' class from all directions | |||
|
822 | [ dom.controlsLeft, dom.controlsRight, dom.controlsUp, dom.controlsDown ].forEach( function( node ) { | |||
|
823 | node.classList.remove( 'enabled' ); | |||
|
824 | } ); | |||
|
825 | ||||
|
826 | // Add the 'enabled' class to the available routes | |||
|
827 | if( routes.left ) dom.controlsLeft.classList.add( 'enabled' ); | |||
|
828 | if( routes.right ) dom.controlsRight.classList.add( 'enabled' ); | |||
|
829 | if( routes.up ) dom.controlsUp.classList.add( 'enabled' ); | |||
|
830 | if( routes.down ) dom.controlsDown.classList.add( 'enabled' ); | |||
|
831 | ||||
|
832 | } | |||
|
833 | } | |||
|
834 | ||||
|
835 | /** | |||
|
836 | * Determine what available routes there are for navigation. | |||
|
837 | * | |||
|
838 | * @return {Object} containing four booleans: left/right/up/down | |||
|
839 | */ | |||
|
840 | function availableRoutes() { | |||
|
841 | var horizontalSlides = document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR ), | |||
|
842 | verticalSlides = document.querySelectorAll( VERTICAL_SLIDES_SELECTOR ); | |||
|
843 | ||||
|
844 | return { | |||
|
845 | left: indexh > 0, | |||
|
846 | right: indexh < horizontalSlides.length - 1, | |||
|
847 | up: indexv > 0, | |||
|
848 | down: indexv < verticalSlides.length - 1 | |||
|
849 | }; | |||
|
850 | } | |||
|
851 | ||||
|
852 | /** | |||
|
853 | * Reads the current URL (hash) and navigates accordingly. | |||
|
854 | */ | |||
|
855 | function readURL() { | |||
|
856 | var hash = window.location.hash; | |||
|
857 | ||||
|
858 | // Attempt to parse the hash as either an index or name | |||
|
859 | var bits = hash.slice( 2 ).split( '/' ), | |||
|
860 | name = hash.replace( /#|\//gi, '' ); | |||
|
861 | ||||
|
862 | // If the first bit is invalid and there is a name we can | |||
|
863 | // assume that this is a named link | |||
|
864 | if( isNaN( parseInt( bits[0], 10 ) ) && name.length ) { | |||
|
865 | // Find the slide with the specified name | |||
|
866 | var element = document.querySelector( '#' + name ); | |||
|
867 | ||||
|
868 | if( element ) { | |||
|
869 | // Find the position of the named slide and navigate to it | |||
|
870 | var indices = Reveal.getIndices( element ); | |||
|
871 | slide( indices.h, indices.v ); | |||
|
872 | } | |||
|
873 | // If the slide doesn't exist, navigate to the current slide | |||
|
874 | else { | |||
|
875 | slide( indexh, indexv ); | |||
|
876 | } | |||
|
877 | } | |||
|
878 | else { | |||
|
879 | // Read the index components of the hash | |||
|
880 | var h = parseInt( bits[0], 10 ) || 0, | |||
|
881 | v = parseInt( bits[1], 10 ) || 0; | |||
|
882 | ||||
|
883 | slide( h, v ); | |||
|
884 | } | |||
|
885 | } | |||
|
886 | ||||
|
887 | /** | |||
|
888 | * Updates the page URL (hash) to reflect the current | |||
|
889 | * state. | |||
|
890 | */ | |||
|
891 | function writeURL() { | |||
|
892 | if( config.history ) { | |||
|
893 | var url = '/'; | |||
|
894 | ||||
|
895 | // Only include the minimum possible number of components in | |||
|
896 | // the URL | |||
|
897 | if( indexh > 0 || indexv > 0 ) url += indexh; | |||
|
898 | if( indexv > 0 ) url += '/' + indexv; | |||
|
899 | ||||
|
900 | window.location.hash = url; | |||
|
901 | } | |||
|
902 | } | |||
|
903 | ||||
|
904 | /** | |||
|
905 | * Retrieves the h/v location of the current, or specified, | |||
|
906 | * slide. | |||
|
907 | * | |||
|
908 | * @param {HTMLElement} slide If specified, the returned | |||
|
909 | * index will be for this slide rather than the currently | |||
|
910 | * active one | |||
|
911 | * | |||
|
912 | * @return {Object} { h: <int>, v: <int> } | |||
|
913 | */ | |||
|
914 | function getIndices( slide ) { | |||
|
915 | // By default, return the current indices | |||
|
916 | var h = indexh, | |||
|
917 | v = indexv; | |||
|
918 | ||||
|
919 | // If a slide is specified, return the indices of that slide | |||
|
920 | if( slide ) { | |||
|
921 | var isVertical = !!slide.parentNode.nodeName.match( /section/gi ); | |||
|
922 | var slideh = isVertical ? slide.parentNode : slide; | |||
|
923 | ||||
|
924 | // Select all horizontal slides | |||
|
925 | var horizontalSlides = Array.prototype.slice.call( document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR ) ); | |||
|
926 | ||||
|
927 | // Now that we know which the horizontal slide is, get its index | |||
|
928 | h = Math.max( horizontalSlides.indexOf( slideh ), 0 ); | |||
|
929 | ||||
|
930 | // If this is a vertical slide, grab the vertical index | |||
|
931 | if( isVertical ) { | |||
|
932 | v = Math.max( Array.prototype.slice.call( slide.parentNode.children ).indexOf( slide ), 0 ); | |||
|
933 | } | |||
|
934 | } | |||
|
935 | ||||
|
936 | return { h: h, v: v }; | |||
|
937 | } | |||
|
938 | ||||
|
939 | /** | |||
|
940 | * Navigate to the next slide fragment. | |||
|
941 | * | |||
|
942 | * @return {Boolean} true if there was a next fragment, | |||
|
943 | * false otherwise | |||
|
944 | */ | |||
|
945 | function nextFragment() { | |||
|
946 | // Vertical slides: | |||
|
947 | if( document.querySelector( VERTICAL_SLIDES_SELECTOR + '.present' ) ) { | |||
|
948 | var verticalFragments = document.querySelectorAll( VERTICAL_SLIDES_SELECTOR + '.present .fragment:not(.visible)' ); | |||
|
949 | if( verticalFragments.length ) { | |||
|
950 | verticalFragments[0].classList.add( 'visible' ); | |||
|
951 | ||||
|
952 | // Notify subscribers of the change | |||
|
953 | dispatchEvent( 'fragmentshown', { fragment: verticalFragments[0] } ); | |||
|
954 | return true; | |||
|
955 | } | |||
|
956 | } | |||
|
957 | // Horizontal slides: | |||
|
958 | else { | |||
|
959 | var horizontalFragments = document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR + '.present .fragment:not(.visible)' ); | |||
|
960 | if( horizontalFragments.length ) { | |||
|
961 | horizontalFragments[0].classList.add( 'visible' ); | |||
|
962 | ||||
|
963 | // Notify subscribers of the change | |||
|
964 | dispatchEvent( 'fragmentshown', { fragment: horizontalFragments[0] } ); | |||
|
965 | return true; | |||
|
966 | } | |||
|
967 | } | |||
|
968 | ||||
|
969 | return false; | |||
|
970 | } | |||
|
971 | ||||
|
972 | /** | |||
|
973 | * Navigate to the previous slide fragment. | |||
|
974 | * | |||
|
975 | * @return {Boolean} true if there was a previous fragment, | |||
|
976 | * false otherwise | |||
|
977 | */ | |||
|
978 | function previousFragment() { | |||
|
979 | // Vertical slides: | |||
|
980 | if( document.querySelector( VERTICAL_SLIDES_SELECTOR + '.present' ) ) { | |||
|
981 | var verticalFragments = document.querySelectorAll( VERTICAL_SLIDES_SELECTOR + '.present .fragment.visible' ); | |||
|
982 | if( verticalFragments.length ) { | |||
|
983 | verticalFragments[ verticalFragments.length - 1 ].classList.remove( 'visible' ); | |||
|
984 | ||||
|
985 | // Notify subscribers of the change | |||
|
986 | dispatchEvent( 'fragmenthidden', { fragment: verticalFragments[ verticalFragments.length - 1 ] } ); | |||
|
987 | return true; | |||
|
988 | } | |||
|
989 | } | |||
|
990 | // Horizontal slides: | |||
|
991 | else { | |||
|
992 | var horizontalFragments = document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR + '.present .fragment.visible' ); | |||
|
993 | if( horizontalFragments.length ) { | |||
|
994 | horizontalFragments[ horizontalFragments.length - 1 ].classList.remove( 'visible' ); | |||
|
995 | ||||
|
996 | // Notify subscribers of the change | |||
|
997 | dispatchEvent( 'fragmenthidden', { fragment: horizontalFragments[ horizontalFragments.length - 1 ] } ); | |||
|
998 | return true; | |||
|
999 | } | |||
|
1000 | } | |||
|
1001 | ||||
|
1002 | return false; | |||
|
1003 | } | |||
|
1004 | ||||
|
1005 | /** | |||
|
1006 | * Cues a new automated slide if enabled in the config. | |||
|
1007 | */ | |||
|
1008 | function cueAutoSlide() { | |||
|
1009 | clearTimeout( autoSlideTimeout ); | |||
|
1010 | ||||
|
1011 | // Cue the next auto-slide if enabled | |||
|
1012 | if( autoSlide ) { | |||
|
1013 | autoSlideTimeout = setTimeout( navigateNext, autoSlide ); | |||
|
1014 | } | |||
|
1015 | } | |||
|
1016 | ||||
|
1017 | function navigateLeft() { | |||
|
1018 | // Prioritize hiding fragments | |||
|
1019 | if( availableRoutes().left && ( isOverviewActive() || previousFragment() === false ) ) { | |||
|
1020 | slide( indexh - 1, 0 ); | |||
|
1021 | } | |||
|
1022 | } | |||
|
1023 | ||||
|
1024 | function navigateRight() { | |||
|
1025 | // Prioritize revealing fragments | |||
|
1026 | if( availableRoutes().right && ( isOverviewActive() || nextFragment() === false ) ) { | |||
|
1027 | slide( indexh + 1, 0 ); | |||
|
1028 | } | |||
|
1029 | } | |||
|
1030 | ||||
|
1031 | function navigateUp() { | |||
|
1032 | // Prioritize hiding fragments | |||
|
1033 | if( availableRoutes().up && ( isOverviewActive() || previousFragment() === false ) ) { | |||
|
1034 | slide( indexh, indexv - 1 ); | |||
|
1035 | } | |||
|
1036 | } | |||
|
1037 | ||||
|
1038 | function navigateDown() { | |||
|
1039 | // Prioritize revealing fragments | |||
|
1040 | if( availableRoutes().down && ( isOverviewActive() || nextFragment() === false ) ) { | |||
|
1041 | slide( indexh, indexv + 1 ); | |||
|
1042 | } | |||
|
1043 | } | |||
|
1044 | ||||
|
1045 | /** | |||
|
1046 | * Navigates backwards, prioritized in the following order: | |||
|
1047 | * 1) Previous fragment | |||
|
1048 | * 2) Previous vertical slide | |||
|
1049 | * 3) Previous horizontal slide | |||
|
1050 | */ | |||
|
1051 | function navigatePrev() { | |||
|
1052 | // Prioritize revealing fragments | |||
|
1053 | if( previousFragment() === false ) { | |||
|
1054 | if( availableRoutes().up ) { | |||
|
1055 | navigateUp(); | |||
|
1056 | } | |||
|
1057 | else { | |||
|
1058 | // Fetch the previous horizontal slide, if there is one | |||
|
1059 | var previousSlide = document.querySelector( '.reveal .slides>section.past:nth-child(' + indexh + ')' ); | |||
|
1060 | ||||
|
1061 | if( previousSlide ) { | |||
|
1062 | indexv = ( previousSlide.querySelectorAll( 'section' ).length + 1 ) || 0; | |||
|
1063 | indexh --; | |||
|
1064 | slide(); | |||
|
1065 | } | |||
|
1066 | } | |||
|
1067 | } | |||
|
1068 | } | |||
|
1069 | ||||
|
1070 | /** | |||
|
1071 | * Same as #navigatePrev() but navigates forwards. | |||
|
1072 | */ | |||
|
1073 | function navigateNext() { | |||
|
1074 | // Prioritize revealing fragments | |||
|
1075 | if( nextFragment() === false ) { | |||
|
1076 | availableRoutes().down ? navigateDown() : navigateRight(); | |||
|
1077 | } | |||
|
1078 | ||||
|
1079 | // If auto-sliding is enabled we need to cue up | |||
|
1080 | // another timeout | |||
|
1081 | cueAutoSlide(); | |||
|
1082 | } | |||
|
1083 | ||||
|
1084 | ||||
|
1085 | // --------------------------------------------------------------------// | |||
|
1086 | // ----------------------------- EVENTS -------------------------------// | |||
|
1087 | // --------------------------------------------------------------------// | |||
|
1088 | ||||
|
1089 | ||||
|
1090 | /** | |||
|
1091 | * Handler for the document level 'keydown' event. | |||
|
1092 | * | |||
|
1093 | * @param {Object} event | |||
|
1094 | */ | |||
|
1095 | function onDocumentKeyDown( event ) { | |||
|
1096 | // Check if there's a focused element that could be using | |||
|
1097 | // the keyboard | |||
|
1098 | var activeElement = document.activeElement; | |||
|
1099 | var hasFocus = !!( document.activeElement && ( document.activeElement.type || document.activeElement.href || document.activeElement.contentEditable !== 'inherit' ) ); | |||
|
1100 | ||||
|
1101 | // Disregard the event if there's a focused element or a | |||
|
1102 | // keyboard modifier key is present | |||
|
1103 | if ( hasFocus || event.shiftKey || event.altKey || event.ctrlKey || event.metaKey ) return; | |||
|
1104 | ||||
|
1105 | var triggered = true; | |||
|
1106 | ||||
|
1107 | switch( event.keyCode ) { | |||
|
1108 | // p, page up | |||
|
1109 | case 80: case 33: navigatePrev(); break; | |||
|
1110 | // n, page down | |||
|
1111 | case 78: case 34: navigateNext(); break; | |||
|
1112 | // h, left | |||
|
1113 | case 72: case 37: navigateLeft(); break; | |||
|
1114 | // l, right | |||
|
1115 | case 76: case 39: navigateRight(); break; | |||
|
1116 | // k, up | |||
|
1117 | case 75: case 38: navigateUp(); break; | |||
|
1118 | // j, down | |||
|
1119 | case 74: case 40: navigateDown(); break; | |||
|
1120 | // home | |||
|
1121 | case 36: slide( 0 ); break; | |||
|
1122 | // end | |||
|
1123 | case 35: slide( Number.MAX_VALUE ); break; | |||
|
1124 | // space | |||
|
1125 | case 32: isOverviewActive() ? deactivateOverview() : navigateNext(); break; | |||
|
1126 | // return | |||
|
1127 | case 13: isOverviewActive() ? deactivateOverview() : triggered = false; break; | |||
|
1128 | // b, period | |||
|
1129 | case 66: case 190: togglePause(); break; | |||
|
1130 | // f | |||
|
1131 | case 70: enterFullscreen(); break; | |||
|
1132 | default: | |||
|
1133 | triggered = false; | |||
|
1134 | } | |||
|
1135 | ||||
|
1136 | // If the input resulted in a triggered action we should prevent | |||
|
1137 | // the browsers default behavior | |||
|
1138 | if( triggered ) { | |||
|
1139 | event.preventDefault(); | |||
|
1140 | } | |||
|
1141 | else if ( event.keyCode === 27 && supports3DTransforms ) { | |||
|
1142 | toggleOverview(); | |||
|
1143 | ||||
|
1144 | event.preventDefault(); | |||
|
1145 | } | |||
|
1146 | ||||
|
1147 | // If auto-sliding is enabled we need to cue up | |||
|
1148 | // another timeout | |||
|
1149 | cueAutoSlide(); | |||
|
1150 | ||||
|
1151 | } | |||
|
1152 | ||||
|
1153 | /** | |||
|
1154 | * Handler for the document level 'touchstart' event, | |||
|
1155 | * enables support for swipe and pinch gestures. | |||
|
1156 | */ | |||
|
1157 | function onDocumentTouchStart( event ) { | |||
|
1158 | touch.startX = event.touches[0].clientX; | |||
|
1159 | touch.startY = event.touches[0].clientY; | |||
|
1160 | touch.startCount = event.touches.length; | |||
|
1161 | ||||
|
1162 | // If there's two touches we need to memorize the distance | |||
|
1163 | // between those two points to detect pinching | |||
|
1164 | if( event.touches.length === 2 && config.overview ) { | |||
|
1165 | touch.startSpan = distanceBetween( { | |||
|
1166 | x: event.touches[1].clientX, | |||
|
1167 | y: event.touches[1].clientY | |||
|
1168 | }, { | |||
|
1169 | x: touch.startX, | |||
|
1170 | y: touch.startY | |||
|
1171 | } ); | |||
|
1172 | } | |||
|
1173 | } | |||
|
1174 | ||||
|
1175 | /** | |||
|
1176 | * Handler for the document level 'touchmove' event. | |||
|
1177 | */ | |||
|
1178 | function onDocumentTouchMove( event ) { | |||
|
1179 | // Each touch should only trigger one action | |||
|
1180 | if( !touch.handled ) { | |||
|
1181 | var currentX = event.touches[0].clientX; | |||
|
1182 | var currentY = event.touches[0].clientY; | |||
|
1183 | ||||
|
1184 | // If the touch started off with two points and still has | |||
|
1185 | // two active touches; test for the pinch gesture | |||
|
1186 | if( event.touches.length === 2 && touch.startCount === 2 && config.overview ) { | |||
|
1187 | ||||
|
1188 | // The current distance in pixels between the two touch points | |||
|
1189 | var currentSpan = distanceBetween( { | |||
|
1190 | x: event.touches[1].clientX, | |||
|
1191 | y: event.touches[1].clientY | |||
|
1192 | }, { | |||
|
1193 | x: touch.startX, | |||
|
1194 | y: touch.startY | |||
|
1195 | } ); | |||
|
1196 | ||||
|
1197 | // If the span is larger than the desire amount we've got | |||
|
1198 | // ourselves a pinch | |||
|
1199 | if( Math.abs( touch.startSpan - currentSpan ) > touch.threshold ) { | |||
|
1200 | touch.handled = true; | |||
|
1201 | ||||
|
1202 | if( currentSpan < touch.startSpan ) { | |||
|
1203 | activateOverview(); | |||
|
1204 | } | |||
|
1205 | else { | |||
|
1206 | deactivateOverview(); | |||
|
1207 | } | |||
|
1208 | } | |||
|
1209 | ||||
|
1210 | event.preventDefault(); | |||
|
1211 | ||||
|
1212 | } | |||
|
1213 | // There was only one touch point, look for a swipe | |||
|
1214 | else if( event.touches.length === 1 && touch.startCount !== 2 ) { | |||
|
1215 | ||||
|
1216 | var deltaX = currentX - touch.startX, | |||
|
1217 | deltaY = currentY - touch.startY; | |||
|
1218 | ||||
|
1219 | if( deltaX > touch.threshold && Math.abs( deltaX ) > Math.abs( deltaY ) ) { | |||
|
1220 | touch.handled = true; | |||
|
1221 | navigateLeft(); | |||
|
1222 | } | |||
|
1223 | else if( deltaX < -touch.threshold && Math.abs( deltaX ) > Math.abs( deltaY ) ) { | |||
|
1224 | touch.handled = true; | |||
|
1225 | navigateRight(); | |||
|
1226 | } | |||
|
1227 | else if( deltaY > touch.threshold ) { | |||
|
1228 | touch.handled = true; | |||
|
1229 | navigateUp(); | |||
|
1230 | } | |||
|
1231 | else if( deltaY < -touch.threshold ) { | |||
|
1232 | touch.handled = true; | |||
|
1233 | navigateDown(); | |||
|
1234 | } | |||
|
1235 | ||||
|
1236 | event.preventDefault(); | |||
|
1237 | ||||
|
1238 | } | |||
|
1239 | } | |||
|
1240 | // There's a bug with swiping on some Android devices unless | |||
|
1241 | // the default action is always prevented | |||
|
1242 | else if( navigator.userAgent.match( /android/gi ) ) { | |||
|
1243 | event.preventDefault(); | |||
|
1244 | } | |||
|
1245 | } | |||
|
1246 | ||||
|
1247 | /** | |||
|
1248 | * Handler for the document level 'touchend' event. | |||
|
1249 | */ | |||
|
1250 | function onDocumentTouchEnd( event ) { | |||
|
1251 | touch.handled = false; | |||
|
1252 | } | |||
|
1253 | ||||
|
1254 | /** | |||
|
1255 | * Handles mouse wheel scrolling, throttled to avoid skipping | |||
|
1256 | * multiple slides. | |||
|
1257 | */ | |||
|
1258 | function onDocumentMouseScroll( event ){ | |||
|
1259 | clearTimeout( mouseWheelTimeout ); | |||
|
1260 | ||||
|
1261 | mouseWheelTimeout = setTimeout( function() { | |||
|
1262 | var delta = event.detail || -event.wheelDelta; | |||
|
1263 | if( delta > 0 ) { | |||
|
1264 | navigateNext(); | |||
|
1265 | } | |||
|
1266 | else { | |||
|
1267 | navigatePrev(); | |||
|
1268 | } | |||
|
1269 | }, 100 ); | |||
|
1270 | } | |||
|
1271 | ||||
|
1272 | /** | |||
|
1273 | * Clicking on the progress bar results in a navigation to the | |||
|
1274 | * closest approximate horizontal slide using this equation: | |||
|
1275 | * | |||
|
1276 | * ( clickX / presentationWidth ) * numberOfSlides | |||
|
1277 | */ | |||
|
1278 | function onProgressClick( event ) { | |||
|
1279 | var slidesTotal = Array.prototype.slice.call( document.querySelectorAll( HORIZONTAL_SLIDES_SELECTOR ) ).length; | |||
|
1280 | var slideIndex = Math.floor( ( event.clientX / dom.wrapper.offsetWidth ) * slidesTotal ); | |||
|
1281 | ||||
|
1282 | slide( slideIndex ); | |||
|
1283 | } | |||
|
1284 | ||||
|
1285 | /** | |||
|
1286 | * Handler for the window level 'hashchange' event. | |||
|
1287 | * | |||
|
1288 | * @param {Object} event | |||
|
1289 | */ | |||
|
1290 | function onWindowHashChange( event ) { | |||
|
1291 | readURL(); | |||
|
1292 | } | |||
|
1293 | ||||
|
1294 | /** | |||
|
1295 | * Invoked when a slide is and we're in the overview. | |||
|
1296 | */ | |||
|
1297 | function onOverviewSlideClicked( event ) { | |||
|
1298 | // TODO There's a bug here where the event listeners are not | |||
|
1299 | // removed after deactivating the overview. | |||
|
1300 | if( isOverviewActive() ) { | |||
|
1301 | event.preventDefault(); | |||
|
1302 | ||||
|
1303 | deactivateOverview(); | |||
|
1304 | ||||
|
1305 | indexh = this.getAttribute( 'data-index-h' ); | |||
|
1306 | indexv = this.getAttribute( 'data-index-v' ); | |||
|
1307 | ||||
|
1308 | slide(); | |||
|
1309 | } | |||
|
1310 | } | |||
|
1311 | ||||
|
1312 | ||||
|
1313 | // --------------------------------------------------------------------// | |||
|
1314 | // ------------------------------- API --------------------------------// | |||
|
1315 | // --------------------------------------------------------------------// | |||
|
1316 | ||||
|
1317 | ||||
|
1318 | return { | |||
|
1319 | initialize: initialize, | |||
|
1320 | ||||
|
1321 | // Navigation methods | |||
|
1322 | slide: slide, | |||
|
1323 | left: navigateLeft, | |||
|
1324 | right: navigateRight, | |||
|
1325 | up: navigateUp, | |||
|
1326 | down: navigateDown, | |||
|
1327 | prev: navigatePrev, | |||
|
1328 | next: navigateNext, | |||
|
1329 | prevFragment: previousFragment, | |||
|
1330 | nextFragment: nextFragment, | |||
|
1331 | ||||
|
1332 | // Deprecated aliases | |||
|
1333 | navigateTo: slide, | |||
|
1334 | navigateLeft: navigateLeft, | |||
|
1335 | navigateRight: navigateRight, | |||
|
1336 | navigateUp: navigateUp, | |||
|
1337 | navigateDown: navigateDown, | |||
|
1338 | navigatePrev: navigatePrev, | |||
|
1339 | navigateNext: navigateNext, | |||
|
1340 | ||||
|
1341 | // Toggles the overview mode on/off | |||
|
1342 | toggleOverview: toggleOverview, | |||
|
1343 | ||||
|
1344 | // Adds or removes all internal event listeners (such as keyboard) | |||
|
1345 | addEventListeners: addEventListeners, | |||
|
1346 | removeEventListeners: removeEventListeners, | |||
|
1347 | ||||
|
1348 | // Returns the indices of the current, or specified, slide | |||
|
1349 | getIndices: getIndices, | |||
|
1350 | ||||
|
1351 | // Returns the previous slide element, may be null | |||
|
1352 | getPreviousSlide: function() { | |||
|
1353 | return previousSlide; | |||
|
1354 | }, | |||
|
1355 | ||||
|
1356 | // Returns the current slide element | |||
|
1357 | getCurrentSlide: function() { | |||
|
1358 | return currentSlide; | |||
|
1359 | }, | |||
|
1360 | ||||
|
1361 | // Helper method, retrieves query string as a key/value hash | |||
|
1362 | getQueryHash: function() { | |||
|
1363 | var query = {}; | |||
|
1364 | ||||
|
1365 | location.search.replace( /[A-Z0-9]+?=(\w*)/gi, function(a) { | |||
|
1366 | query[ a.split( '=' ).shift() ] = a.split( '=' ).pop(); | |||
|
1367 | } ); | |||
|
1368 | ||||
|
1369 | return query; | |||
|
1370 | }, | |||
|
1371 | ||||
|
1372 | // Forward event binding to the reveal DOM element | |||
|
1373 | addEventListener: function( type, listener, useCapture ) { | |||
|
1374 | if( 'addEventListener' in window ) { | |||
|
1375 | ( dom.wrapper || document.querySelector( '.reveal' ) ).addEventListener( type, listener, useCapture ); | |||
|
1376 | } | |||
|
1377 | }, | |||
|
1378 | removeEventListener: function( type, listener, useCapture ) { | |||
|
1379 | if( 'addEventListener' in window ) { | |||
|
1380 | ( dom.wrapper || document.querySelector( '.reveal' ) ).removeEventListener( type, listener, useCapture ); | |||
|
1381 | } | |||
|
1382 | } | |||
|
1383 | }; | |||
|
1384 | ||||
|
1385 | })(); No newline at end of file |
@@ -0,0 +1,83 b'' | |||||
|
1 | /*! | |||
|
2 | * reveal.js 2.1 r37 | |||
|
3 | * http://lab.hakim.se/reveal-js | |||
|
4 | * MIT licensed | |||
|
5 | * | |||
|
6 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
7 | */ | |||
|
8 | var Reveal=(function(){var l=".reveal .slides>section",b=".reveal .slides>section.present>section",R={controls:true,progress:true,history:false,keyboard:true,overview:true,loop:false,autoSlide:0,mouseWheel:true,rollingLinks:true,theme:null,transition:"default",dependencies:[]},Y=R.autoSlide,m=0,e=0,y,G,ak=[],f={},T="WebkitPerspective" in document.body.style||"MozPerspective" in document.body.style||"msPerspective" in document.body.style||"OPerspective" in document.body.style||"perspective" in document.body.style,n="WebkitTransform" in document.body.style||"MozTransform" in document.body.style||"msTransform" in document.body.style||"OTransform" in document.body.style||"transform" in document.body.style,z=0,k=0,D=0,ac={startX:0,startY:0,startSpan:0,startCount:0,handled:false,threshold:80}; | |||
|
9 | function i(al){if((!n&&!T)){document.body.setAttribute("class","no-transforms");return;}t(R,al);d();V();}function P(){f.theme=document.querySelector("#theme"); | |||
|
10 | f.wrapper=document.querySelector(".reveal");if(!f.wrapper.querySelector(".progress")&&R.progress){var ao=document.createElement("div");ao.classList.add("progress"); | |||
|
11 | ao.innerHTML="<span></span>";f.wrapper.appendChild(ao);}if(!f.wrapper.querySelector(".controls")&&R.controls){var an=document.createElement("aside");an.classList.add("controls"); | |||
|
12 | an.innerHTML='<div class="left"></div><div class="right"></div><div class="up"></div><div class="down"></div>';f.wrapper.appendChild(an);}if(!f.wrapper.querySelector(".state-background")){var am=document.createElement("div"); | |||
|
13 | am.classList.add("state-background");f.wrapper.appendChild(am);}if(!f.wrapper.querySelector(".pause-overlay")){var al=document.createElement("div");al.classList.add("pause-overlay"); | |||
|
14 | f.wrapper.appendChild(al);}f.progress=document.querySelector(".reveal .progress");f.progressbar=document.querySelector(".reveal .progress span");if(R.controls){f.controls=document.querySelector(".reveal .controls"); | |||
|
15 | f.controlsLeft=document.querySelector(".reveal .controls .left");f.controlsRight=document.querySelector(".reveal .controls .right");f.controlsUp=document.querySelector(".reveal .controls .up"); | |||
|
16 | f.controlsDown=document.querySelector(".reveal .controls .down");}}function d(){if(navigator.userAgent.match(/(iphone|ipod|android)/i)){document.documentElement.style.overflow="scroll"; | |||
|
17 | document.body.style.height="120%";window.addEventListener("load",ad,false);window.addEventListener("orientationchange",ad,false);}}function V(){var am=[],aq=[]; | |||
|
18 | for(var an=0,al=R.dependencies.length;an<al;an++){var ao=R.dependencies[an];if(!ao.condition||ao.condition()){if(ao.async){aq.push(ao.src);}else{am.push(ao.src); | |||
|
19 | }if(typeof ao.callback==="function"){head.ready(ao.src.match(/([\w\d_\-]*)\.?[^\\\/]*$/i)[0],ao.callback);}}}function ap(){if(aq.length){head.js.apply(null,aq); | |||
|
20 | }H();}if(am.length){head.ready(ap);head.js.apply(null,am);}else{ap();}}function H(){P();E();K();J();O();setTimeout(function(){r("ready",{indexh:m,indexv:e,currentSlide:G}); | |||
|
21 | },1);}function K(){if(T===false){R.transition="linear";}if(R.controls&&f.controls){f.controls.style.display="block";}if(R.progress&&f.progress){f.progress.style.display="block"; | |||
|
22 | }if(R.transition!=="default"){f.wrapper.classList.add(R.transition);}if(R.mouseWheel){document.addEventListener("DOMMouseScroll",o,false);document.addEventListener("mousewheel",o,false); | |||
|
23 | }if(R.rollingLinks){N();}if(R.theme&&f.theme){var an=f.theme.getAttribute("href");var al=/[^\/]*?(?=\.css)/;var am=an.match(al)[0];if(R.theme!==am){an=an.replace(al,R.theme); | |||
|
24 | f.theme.setAttribute("href",an);}}}function E(){document.addEventListener("touchstart",A,false);document.addEventListener("touchmove",af,false);document.addEventListener("touchend",W,false); | |||
|
25 | window.addEventListener("hashchange",w,false);if(R.keyboard){document.addEventListener("keydown",ah,false);}if(R.progress&&f.progress){f.progress.addEventListener("click",q(ai),false); | |||
|
26 | }if(R.controls&&f.controls){f.controlsLeft.addEventListener("click",q(B),false);f.controlsRight.addEventListener("click",q(j),false);f.controlsUp.addEventListener("click",q(u),false); | |||
|
27 | f.controlsDown.addEventListener("click",q(F),false);}}function U(){document.removeEventListener("keydown",ah,false);document.removeEventListener("touchstart",A,false); | |||
|
28 | document.removeEventListener("touchmove",af,false);document.removeEventListener("touchend",W,false);window.removeEventListener("hashchange",w,false);if(R.progress&&f.progress){f.progress.removeEventListener("click",q(ai),false); | |||
|
29 | }if(R.controls&&f.controls){f.controlsLeft.removeEventListener("click",q(B),false);f.controlsRight.removeEventListener("click",q(j),false);f.controlsUp.removeEventListener("click",q(u),false); | |||
|
30 | f.controlsDown.removeEventListener("click",q(F),false);}}function t(am,al){for(var an in al){am[an]=al[an];}}function S(an,al){var ao=an.x-al.x,am=an.y-al.y; | |||
|
31 | return Math.sqrt(ao*ao+am*am);}function q(al){return function(am){am.preventDefault();al.call(null,am);};}function ad(){setTimeout(function(){window.scrollTo(0,1); | |||
|
32 | },0);}function r(am,al){var an=document.createEvent("HTMLEvents",1,2);an.initEvent(am,true,true);t(an,al);f.wrapper.dispatchEvent(an);}function N(){if(T&&!("msPerspective" in document.body.style)){var am=document.querySelectorAll(".reveal .slides section a:not(.image)"); | |||
|
33 | for(var an=0,al=am.length;an<al;an++){var ao=am[an];if(ao.textContent&&!ao.querySelector("img")&&(!ao.className||!ao.classList.contains(ao,"roll"))){ao.classList.add("roll"); | |||
|
34 | ao.innerHTML='<span data-title="'+ao.text+'">'+ao.innerHTML+"</span>";}}}}function I(){if(R.overview){f.wrapper.classList.add("overview");var al=document.querySelectorAll(l); | |||
|
35 | for(var aq=0,ao=al.length;aq<ao;aq++){var an=al[aq],av="translateZ(-2500px) translate("+((aq-m)*105)+"%, 0%)";an.setAttribute("data-index-h",aq);an.style.display="block"; | |||
|
36 | an.style.WebkitTransform=av;an.style.MozTransform=av;an.style.msTransform=av;an.style.OTransform=av;an.style.transform=av;if(!an.classList.contains("stack")){an.addEventListener("click",C,true); | |||
|
37 | }var au=an.querySelectorAll("section");for(var ap=0,am=au.length;ap<am;ap++){var at=au[ap],ar="translate(0%, "+((ap-(aq===m?e:0))*105)+"%)";at.setAttribute("data-index-h",aq); | |||
|
38 | at.setAttribute("data-index-v",ap);at.style.display="block";at.style.WebkitTransform=ar;at.style.MozTransform=ar;at.style.msTransform=ar;at.style.OTransform=ar; | |||
|
39 | at.style.transform=ar;at.addEventListener("click",C,true);}}}}function ae(){if(R.overview){f.wrapper.classList.remove("overview");var ao=Array.prototype.slice.call(document.querySelectorAll(".reveal .slides section")); | |||
|
40 | for(var an=0,al=ao.length;an<al;an++){var am=ao[an];am.style.WebkitTransform="";am.style.MozTransform="";am.style.msTransform="";am.style.OTransform=""; | |||
|
41 | am.style.transform="";am.removeEventListener("click",C);}a();}}function X(al){if(typeof al==="boolean"){al?I():ae();}else{L()?ae():I();}}function L(){return f.wrapper.classList.contains("overview"); | |||
|
42 | }function ab(){var al=document.body;var am=al.requestFullScreen||al.webkitRequestFullScreen||al.mozRequestFullScreen||al.msRequestFullScreen;if(am){am.apply(al); | |||
|
43 | }}function c(){f.wrapper.classList.add("paused");}function p(){f.wrapper.classList.remove("paused");}function aa(){if(ag()){p();}else{c();}}function ag(){return f.wrapper.classList.contains("paused"); | |||
|
44 | }function a(ar,aw){y=G;var ao=ak.concat();ak.length=0;var av=m,am=e;m=aj(l,ar===undefined?m:ar);e=aj(b,aw===undefined?e:aw);stateLoop:for(var ap=0,at=ak.length; | |||
|
45 | ap<at;ap++){for(var an=0;an<ao.length;an++){if(ao[an]===ak[ap]){ao.splice(an,1);continue stateLoop;}}document.documentElement.classList.add(ak[ap]);r(ak[ap]); | |||
|
46 | }while(ao.length){document.documentElement.classList.remove(ao.pop());}if(R.progress&&f.progress){f.progressbar.style.width=(m/(document.querySelectorAll(l).length-1))*window.innerWidth+"px"; | |||
|
47 | }if(L()){I();}s();clearTimeout(D);D=setTimeout(h,1500);var al=document.querySelectorAll(l);var au=al[m],aq=au.querySelectorAll("section");G=aq[e]||au;if(m!==av||e!==am){r("slidechanged",{indexh:m,indexv:e,previousSlide:y,currentSlide:G}); | |||
|
48 | }else{y=null;}if(y){y.classList.remove("present");}}function aj(ao,au){var am=Array.prototype.slice.call(document.querySelectorAll(ao)),at=am.length;if(at){if(R.loop){au%=at; | |||
|
49 | if(au<0){au=at+au;}}au=Math.max(Math.min(au,at-1),0);for(var aq=0;aq<at;aq++){var ar=am[aq];if(L()===false){var al=Math.abs((au-aq)%(at-3))||0;ar.style.display=al>3?"none":"block"; | |||
|
50 | }am[aq].classList.remove("past");am[aq].classList.remove("present");am[aq].classList.remove("future");if(aq<au){am[aq].classList.add("past");}else{if(aq>au){am[aq].classList.add("future"); | |||
|
51 | }}if(ar.querySelector("section")){am[aq].classList.add("stack");}}am[au].classList.add("present");var an=am[au].getAttribute("data-state");if(an){ak=ak.concat(an.split(" ")); | |||
|
52 | }var ap=am[au].getAttribute("data-autoslide");if(ap){Y=parseInt(ap);}else{Y=R.autoSlide;}}else{au=0;}return au;}function s(){if(R.controls&&f.controls){var al=g(); | |||
|
53 | [f.controlsLeft,f.controlsRight,f.controlsUp,f.controlsDown].forEach(function(am){am.classList.remove("enabled");});if(al.left){f.controlsLeft.classList.add("enabled"); | |||
|
54 | }if(al.right){f.controlsRight.classList.add("enabled");}if(al.up){f.controlsUp.classList.add("enabled");}if(al.down){f.controlsDown.classList.add("enabled"); | |||
|
55 | }}}function g(){var al=document.querySelectorAll(l),am=document.querySelectorAll(b);return{left:m>0,right:m<al.length-1,up:e>0,down:e<am.length-1};}function J(){var aq=window.location.hash; | |||
|
56 | var ap=aq.slice(2).split("/"),am=aq.replace(/#|\//gi,"");if(isNaN(parseInt(ap[0],10))&&am.length){var an=document.querySelector("#"+am);if(an){var ar=Reveal.getIndices(an); | |||
|
57 | a(ar.h,ar.v);}else{a(m,e);}}else{var ao=parseInt(ap[0],10)||0,al=parseInt(ap[1],10)||0;a(ao,al);}}function h(){if(R.history){var al="/";if(m>0||e>0){al+=m; | |||
|
58 | }if(e>0){al+="/"+e;}window.location.hash=al;}}function M(al){var ap=m,an=e;if(al){var aq=!!al.parentNode.nodeName.match(/section/gi);var ao=aq?al.parentNode:al; | |||
|
59 | var am=Array.prototype.slice.call(document.querySelectorAll(l));ap=Math.max(am.indexOf(ao),0);if(aq){an=Math.max(Array.prototype.slice.call(al.parentNode.children).indexOf(al),0); | |||
|
60 | }}return{h:ap,v:an};}function v(){if(document.querySelector(b+".present")){var am=document.querySelectorAll(b+".present .fragment:not(.visible)");if(am.length){am[0].classList.add("visible"); | |||
|
61 | r("fragmentshown",{fragment:am[0]});return true;}}else{var al=document.querySelectorAll(l+".present .fragment:not(.visible)");if(al.length){al[0].classList.add("visible"); | |||
|
62 | r("fragmentshown",{fragment:al[0]});return true;}}return false;}function Q(){if(document.querySelector(b+".present")){var am=document.querySelectorAll(b+".present .fragment.visible"); | |||
|
63 | if(am.length){am[am.length-1].classList.remove("visible");r("fragmenthidden",{fragment:am[am.length-1]});return true;}}else{var al=document.querySelectorAll(l+".present .fragment.visible"); | |||
|
64 | if(al.length){al[al.length-1].classList.remove("visible");r("fragmenthidden",{fragment:al[al.length-1]});return true;}}return false;}function O(){clearTimeout(k); | |||
|
65 | if(Y){k=setTimeout(x,Y);}}function B(){if(g().left&&(L()||Q()===false)){a(m-1,0);}}function j(){if(g().right&&(L()||v()===false)){a(m+1,0);}}function u(){if(g().up&&(L()||Q()===false)){a(m,e-1); | |||
|
66 | }}function F(){if(g().down&&(L()||v()===false)){a(m,e+1);}}function Z(){if(Q()===false){if(g().up){u();}else{var al=document.querySelector(".reveal .slides>section.past:nth-child("+m+")"); | |||
|
67 | if(al){e=(al.querySelectorAll("section").length+1)||0;m--;a();}}}}function x(){if(v()===false){g().down?F():j();}O();}function ah(an){var am=document.activeElement; | |||
|
68 | var ao=!!(document.activeElement&&(document.activeElement.type||document.activeElement.href||document.activeElement.contentEditable!=="inherit"));if(ao||an.shiftKey||an.altKey||an.ctrlKey||an.metaKey){return; | |||
|
69 | }var al=true;switch(an.keyCode){case 80:case 33:Z();break;case 78:case 34:x();break;case 72:case 37:B();break;case 76:case 39:j();break;case 75:case 38:u(); | |||
|
70 | break;case 74:case 40:F();break;case 36:a(0);break;case 35:a(Number.MAX_VALUE);break;case 32:L()?ae():x();break;case 13:L()?ae():al=false;break;case 66:case 190:aa(); | |||
|
71 | break;case 70:ab();break;default:al=false;}if(al){an.preventDefault();}else{if(an.keyCode===27&&T){X();an.preventDefault();}}O();}function A(al){ac.startX=al.touches[0].clientX; | |||
|
72 | ac.startY=al.touches[0].clientY;ac.startCount=al.touches.length;if(al.touches.length===2&&R.overview){ac.startSpan=S({x:al.touches[1].clientX,y:al.touches[1].clientY},{x:ac.startX,y:ac.startY}); | |||
|
73 | }}function af(aq){if(!ac.handled){var ao=aq.touches[0].clientX;var an=aq.touches[0].clientY;if(aq.touches.length===2&&ac.startCount===2&&R.overview){var ap=S({x:aq.touches[1].clientX,y:aq.touches[1].clientY},{x:ac.startX,y:ac.startY}); | |||
|
74 | if(Math.abs(ac.startSpan-ap)>ac.threshold){ac.handled=true;if(ap<ac.startSpan){I();}else{ae();}}aq.preventDefault();}else{if(aq.touches.length===1&&ac.startCount!==2){var am=ao-ac.startX,al=an-ac.startY; | |||
|
75 | if(am>ac.threshold&&Math.abs(am)>Math.abs(al)){ac.handled=true;B();}else{if(am<-ac.threshold&&Math.abs(am)>Math.abs(al)){ac.handled=true;j();}else{if(al>ac.threshold){ac.handled=true; | |||
|
76 | u();}else{if(al<-ac.threshold){ac.handled=true;F();}}}}aq.preventDefault();}}}else{if(navigator.userAgent.match(/android/gi)){aq.preventDefault();}}}function W(al){ac.handled=false; | |||
|
77 | }function o(al){clearTimeout(z);z=setTimeout(function(){var am=al.detail||-al.wheelDelta;if(am>0){x();}else{Z();}},100);}function ai(am){var al=Array.prototype.slice.call(document.querySelectorAll(l)).length; | |||
|
78 | var an=Math.floor((am.clientX/f.wrapper.offsetWidth)*al);a(an);}function w(al){J();}function C(al){if(L()){al.preventDefault();ae();m=this.getAttribute("data-index-h"); | |||
|
79 | e=this.getAttribute("data-index-v");a();}}return{initialize:i,slide:a,left:B,right:j,up:u,down:F,prev:Z,next:x,prevFragment:Q,nextFragment:v,navigateTo:a,navigateLeft:B,navigateRight:j,navigateUp:u,navigateDown:F,navigatePrev:Z,navigateNext:x,toggleOverview:X,addEventListeners:E,removeEventListeners:U,getIndices:M,getPreviousSlide:function(){return y; | |||
|
80 | },getCurrentSlide:function(){return G;},getQueryHash:function(){var al={};location.search.replace(/[A-Z0-9]+?=(\w*)/gi,function(am){al[am.split("=").shift()]=am.split("=").pop(); | |||
|
81 | });return al;},addEventListener:function(am,an,al){if("addEventListener" in window){(f.wrapper||document.querySelector(".reveal")).addEventListener(am,an,al); | |||
|
82 | }},removeEventListener:function(am,an,al){if("addEventListener" in window){(f.wrapper||document.querySelector(".reveal")).removeEventListener(am,an,al); | |||
|
83 | }}};})(); No newline at end of file |
@@ -0,0 +1,115 b'' | |||||
|
1 | /* | |||
|
2 | ||||
|
3 | Zenburn style from voldmar.ru (c) Vladimir Epifanov <voldmar@voldmar.ru> | |||
|
4 | based on dark.css by Ivan Sagalaev | |||
|
5 | ||||
|
6 | */ | |||
|
7 | ||||
|
8 | pre code { | |||
|
9 | display: block; padding: 0.5em; | |||
|
10 | background: #3F3F3F; | |||
|
11 | color: #DCDCDC; | |||
|
12 | } | |||
|
13 | ||||
|
14 | pre .keyword, | |||
|
15 | pre .tag, | |||
|
16 | pre .django .tag, | |||
|
17 | pre .django .keyword, | |||
|
18 | pre .css .class, | |||
|
19 | pre .css .id, | |||
|
20 | pre .lisp .title { | |||
|
21 | color: #E3CEAB; | |||
|
22 | } | |||
|
23 | ||||
|
24 | pre .django .template_tag, | |||
|
25 | pre .django .variable, | |||
|
26 | pre .django .filter .argument { | |||
|
27 | color: #DCDCDC; | |||
|
28 | } | |||
|
29 | ||||
|
30 | pre .number, | |||
|
31 | pre .date { | |||
|
32 | color: #8CD0D3; | |||
|
33 | } | |||
|
34 | ||||
|
35 | pre .dos .envvar, | |||
|
36 | pre .dos .stream, | |||
|
37 | pre .variable, | |||
|
38 | pre .apache .sqbracket { | |||
|
39 | color: #EFDCBC; | |||
|
40 | } | |||
|
41 | ||||
|
42 | pre .dos .flow, | |||
|
43 | pre .diff .change, | |||
|
44 | pre .python .exception, | |||
|
45 | pre .python .built_in, | |||
|
46 | pre .literal, | |||
|
47 | pre .tex .special { | |||
|
48 | color: #EFEFAF; | |||
|
49 | } | |||
|
50 | ||||
|
51 | pre .diff .chunk, | |||
|
52 | pre .ruby .subst { | |||
|
53 | color: #8F8F8F; | |||
|
54 | } | |||
|
55 | ||||
|
56 | pre .dos .keyword, | |||
|
57 | pre .python .decorator, | |||
|
58 | pre .class .title, | |||
|
59 | pre .haskell .label, | |||
|
60 | pre .function .title, | |||
|
61 | pre .ini .title, | |||
|
62 | pre .diff .header, | |||
|
63 | pre .ruby .class .parent, | |||
|
64 | pre .apache .tag, | |||
|
65 | pre .nginx .built_in, | |||
|
66 | pre .tex .command, | |||
|
67 | pre .input_number { | |||
|
68 | color: #efef8f; | |||
|
69 | } | |||
|
70 | ||||
|
71 | pre .dos .winutils, | |||
|
72 | pre .ruby .symbol, | |||
|
73 | pre .ruby .symbol .string, | |||
|
74 | pre .ruby .symbol .keyword, | |||
|
75 | pre .ruby .symbol .keymethods, | |||
|
76 | pre .ruby .string, | |||
|
77 | pre .ruby .instancevar { | |||
|
78 | color: #DCA3A3; | |||
|
79 | } | |||
|
80 | ||||
|
81 | pre .diff .deletion, | |||
|
82 | pre .string, | |||
|
83 | pre .tag .value, | |||
|
84 | pre .preprocessor, | |||
|
85 | pre .built_in, | |||
|
86 | pre .sql .aggregate, | |||
|
87 | pre .javadoc, | |||
|
88 | pre .smalltalk .class, | |||
|
89 | pre .smalltalk .localvars, | |||
|
90 | pre .smalltalk .array, | |||
|
91 | pre .css .rules .value, | |||
|
92 | pre .attr_selector, | |||
|
93 | pre .pseudo, | |||
|
94 | pre .apache .cbracket, | |||
|
95 | pre .tex .formula { | |||
|
96 | color: #CC9393; | |||
|
97 | } | |||
|
98 | ||||
|
99 | pre .shebang, | |||
|
100 | pre .diff .addition, | |||
|
101 | pre .comment, | |||
|
102 | pre .java .annotation, | |||
|
103 | pre .template_comment, | |||
|
104 | pre .pi, | |||
|
105 | pre .doctype { | |||
|
106 | color: #7F9F7F; | |||
|
107 | } | |||
|
108 | ||||
|
109 | pre .xml .css, | |||
|
110 | pre .xml .javascript, | |||
|
111 | pre .xml .vbscript, | |||
|
112 | pre .tex .formula { | |||
|
113 | opacity: 0.5; | |||
|
114 | } | |||
|
115 |
1 | NO CONTENT: new file 100755, binary diff hidden |
|
NO CONTENT: new file 100755, binary diff hidden |
@@ -0,0 +1,230 b'' | |||||
|
1 | <?xml version="1.0" standalone="no"?> | |||
|
2 | <!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd" > | |||
|
3 | <svg xmlns="http://www.w3.org/2000/svg"> | |||
|
4 | <metadata></metadata> | |||
|
5 | <defs> | |||
|
6 | <font id="LeagueGothicRegular" horiz-adv-x="724" > | |||
|
7 | <font-face units-per-em="2048" ascent="1505" descent="-543" /> | |||
|
8 | <missing-glyph horiz-adv-x="315" /> | |||
|
9 | <glyph horiz-adv-x="0" /> | |||
|
10 | <glyph horiz-adv-x="2048" /> | |||
|
11 | <glyph unicode="
" horiz-adv-x="682" /> | |||
|
12 | <glyph unicode=" " horiz-adv-x="315" /> | |||
|
13 | <glyph unicode="	" horiz-adv-x="315" /> | |||
|
14 | <glyph unicode=" " horiz-adv-x="315" /> | |||
|
15 | <glyph unicode="!" horiz-adv-x="387" d="M74 1505h239l-55 -1099h-129zM86 0v227h215v-227h-215z" /> | |||
|
16 | <glyph unicode=""" horiz-adv-x="329" d="M57 1505h215l-30 -551h-154z" /> | |||
|
17 | <glyph unicode="#" horiz-adv-x="1232" d="M49 438l27 195h198l37 258h-196l26 194h197l57 420h197l-57 -420h260l57 420h197l-58 -420h193l-27 -194h-192l-37 -258h190l-26 -195h-191l-59 -438h-197l60 438h-261l-59 -438h-197l60 438h-199zM471 633h260l37 258h-260z" /> | |||
|
18 | <glyph unicode="$" horiz-adv-x="692" d="M37 358l192 13q12 -186 129 -187q88 0 93 185q0 74 -61 175q-21 36 -34 53l-40 55q-28 38 -65.5 90t-70.5 101.5t-70.5 141.5t-37.5 170q4 293 215 342v131h123v-125q201 -23 235 -282l-192 -25q-14 129 -93 125q-80 -2 -84 -162q0 -102 94 -227l41 -59q30 -42 37 -52 t33 -48l37 -52q41 -57 68 -109l26 -55q43 -94 43 -186q-4 -338 -245 -369v-217h-123v221q-236 41 -250 352z" /> | |||
|
19 | <glyph unicode="%" horiz-adv-x="1001" d="M55 911v437q0 110 82 156q33 18 90.5 18t97.5 -44t44 -87l4 -43v-437q0 -107 -81 -157q-32 -19 -77 -19q-129 0 -156 135zM158 0l553 1505h131l-547 -1505h-137zM178 911q-4 -55 37 -55q16 0 25.5 14.5t9.5 26.5v451q2 55 -35 55q-18 0 -27.5 -13.5t-9.5 -27.5v-451z M631 158v436q0 108 81 156q33 20 79 20q125 0 153 -135l4 -41v-436q0 -110 -80 -156q-32 -18 -90.5 -18t-98.5 43t-44 88zM754 158q-4 -57 37 -58q37 0 34 58v436q2 55 -34 55q-18 0 -27.5 -13t-9.5 -28v-450z" /> | |||
|
20 | <glyph unicode="&" horiz-adv-x="854" d="M49 304q0 126 44 225.5t126 222.5q-106 225 -106 442v18q0 94 47 180q70 130 223 130q203 0 252 -215q14 -61 12 -113q0 -162 -205 -434q76 -174 148 -285q33 96 47 211l176 -33q-16 -213 -92 -358q55 -63 92 -76v-235q-23 0 -86 37.5t-123 101.5q-123 -139 -252 -139 t-216 97t-87 223zM263 325.5q1 -65.5 28.5 -107.5t78.5 -42t117 86q-88 139 -174 295q-18 -30 -34.5 -98t-15.5 -133.5zM305 1194q0 -111 55 -246q101 156 101 252q-2 2 0 15.5t-2 36t-11 42.5q-19 52 -61.5 52t-62 -38t-19.5 -75v-39z" /> | |||
|
21 | <glyph unicode="'" horiz-adv-x="309" d="M45 1012l72 266h-72v227h215v-227l-113 -266h-102z" /> | |||
|
22 | <glyph unicode="(" horiz-adv-x="561" d="M66 645q0 143 29.5 292.5t73.5 261.5q92 235 159 343l30 47l162 -84q-38 -53 -86.5 -148t-82.5 -189.5t-61.5 -238t-27.5 -284.5t26.5 -282.5t64.5 -240.5q80 -207 141 -296l26 -39l-162 -84q-41 61 -96 173t-94 217.5t-70.5 257t-31.5 294.5z" /> | |||
|
23 | <glyph unicode=")" horiz-adv-x="561" d="M41 -213q36 50 85.5 147t83.5 190t61.5 236.5t27.5 284.5t-26.5 282.5t-64.5 240.5q-78 205 -140 298l-27 39l162 84q41 -61 96 -173.5t94 -217t71 -257.5t32 -296t-30 -292.5t-74 -260.5q-92 -233 -159 -342l-30 -47z" /> | |||
|
24 | <glyph unicode="*" horiz-adv-x="677" d="M74 1251l43 148l164 -70l-19 176h154l-19 -176l164 70l43 -148l-172 -34l115 -138l-131 -80l-78 152l-76 -152l-131 80l115 138z" /> | |||
|
25 | <glyph unicode="+" horiz-adv-x="1060" d="M74 649v172h370v346h172v-346h371v-172h-371v-346h-172v346h-370z" /> | |||
|
26 | <glyph unicode="," horiz-adv-x="309" d="M45 0v227h215v-227l-113 -266h-102l72 266h-72z" /> | |||
|
27 | <glyph unicode="-" horiz-adv-x="444" d="M74 455v194h297v-194h-297z" /> | |||
|
28 | <glyph unicode="." horiz-adv-x="321" d="M53 0v227h215v-227h-215z" /> | |||
|
29 | <glyph unicode="/" horiz-adv-x="720" d="M8 -147l543 1652h162l-537 -1652h-168z" /> | |||
|
30 | <glyph unicode="0" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5l2 26v887q0 46 -19.5 78.5 t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
31 | <glyph unicode="1" horiz-adv-x="475" d="M25 1180v141q129 25 205 130q16 21 30 54h133v-1505h-221v1180h-147z" /> | |||
|
32 | <glyph unicode="2" horiz-adv-x="731" d="M55 0v219l39 62q25 39 88.5 152.5t112.5 220t91 241.5t44 238q0 184 -73.5 184t-73.5 -184v-105h-222v105q0 389 295 389t295 -375q0 -336 -346 -928h350v-219h-600z" /> | |||
|
33 | <glyph unicode="3" horiz-adv-x="686" d="M45 1071q0 249 63 343q29 42 84.5 75t134.5 33t136 -31t84.5 -71t44.5 -92q22 -71 22 -130q0 -291 -108 -399q127 -100 127 -414q0 -68 -19.5 -145.5t-47 -128t-85 -89t-136.5 -38.5t-135 31.5t-86 75.5t-48 113q-23 91 -23 230h217q2 -150 17.5 -203t59.5 -53t56.5 50.5 t12.5 104.5t1 102t0 63q-6 82 -14 95l-18 33q-12 22 -29 29q-55 22 -108 25h-19v184q133 7 156 73q12 34 12 91v105q0 146 -29 177q-16 17 -40 17q-41 0 -52.5 -49t-13.5 -207h-217z" /> | |||
|
34 | <glyph unicode="4" horiz-adv-x="684" d="M25 328v194l323 983h221v-983h103v-194h-103v-328h-202v328h-342zM213 522h154v516h-13z" /> | |||
|
35 | <glyph unicode="5" horiz-adv-x="704" d="M74 438h221v-59q0 -115 14.5 -159t52 -44t53 45t15.5 156v336q0 111 -70 110q-33 0 -59.5 -40t-26.5 -70h-186v792h535v-219h-344v-313q74 55 127 51q78 0 133 -40t77 -100q35 -98 35 -171v-336q0 -393 -289 -393q-78 0 -133 29.5t-84.5 71.5t-46.5 109q-24 98 -24 244z " /> | |||
|
36 | <glyph unicode="6" horiz-adv-x="700" d="M66 309v856q0 356 288.5 356.5t288.5 -356.5v-94h-221q0 162 -11.5 210t-53.5 48t-56 -37t-14 -127v-268q59 37 124.5 37t119 -36t75.5 -93q37 -92 37 -189v-307q0 -90 -42 -187q-26 -61 -89 -99.5t-157.5 -38.5t-158 38.5t-88.5 99.5q-42 98 -42 187zM287 244 q0 -20 17.5 -44t49 -24t50 24.5t18.5 43.5v450q0 18 -18.5 43t-49 25t-48 -20.5t-19.5 -41.5v-456z" /> | |||
|
37 | <glyph unicode="7" horiz-adv-x="589" d="M8 1286v219h557v-221l-221 -1284h-229l225 1286h-332z" /> | |||
|
38 | <glyph unicode="8" horiz-adv-x="696" d="M53 322v176q0 188 115 297q-102 102 -102 276v127q0 213 147 293q57 31 135 31t135.5 -31t84 -71t42.5 -93q21 -66 21 -129v-127q0 -174 -103 -276q115 -109 115 -297v-176q0 -222 -153 -306q-60 -32 -142 -32t-141.5 32.5t-88 73.5t-44.5 96q-21 69 -21 136zM269 422 q1 -139 16.5 -187.5t57.5 -48.5t59.5 30t21.5 71t4 158t-13.5 174t-66.5 57t-66.5 -57.5t-12.5 -196.5zM284 1116q-1 -123 11 -173t53 -50t53.5 50t12.5 170t-12.5 167t-51.5 47t-52 -44t-14 -167z" /> | |||
|
39 | <glyph unicode="9" horiz-adv-x="700" d="M57 340v94h222q0 -162 11 -210t53 -48t56.5 37t14.5 127v283q-59 -37 -125 -37t-119 35.5t-76 92.5q-37 96 -37 189v293q0 87 43 188q25 60 88.5 99t157.5 39t157.5 -39t88.5 -99q43 -101 43 -188v-856q0 -356 -289 -356t-289 356zM279 825q0 -18 18 -42.5t49 -24.5 t48.5 20.5t19.5 40.5v443q0 20 -17.5 43.5t-49.5 23.5t-50 -24.5t-18 -42.5v-437z" /> | |||
|
40 | <glyph unicode=":" horiz-adv-x="362" d="M74 0v227h215v-227h-215zM74 893v227h215v-227h-215z" /> | |||
|
41 | <glyph unicode=";" horiz-adv-x="362" d="M74 0v227h215v-227l-113 -266h-102l71 266h-71zM74 893v227h215v-227h-215z" /> | |||
|
42 | <glyph unicode="<" horiz-adv-x="1058" d="M74 649v160l911 475v-199l-698 -356l698 -356v-199z" /> | |||
|
43 | <glyph unicode="=" horiz-adv-x="1058" d="M74 477v172h911v-172h-911zM74 864v172h911v-172h-911z" /> | |||
|
44 | <glyph unicode=">" horiz-adv-x="1058" d="M74 174v199l698 356l-698 356v199l911 -475v-160z" /> | |||
|
45 | <glyph unicode="?" horiz-adv-x="645" d="M25 1260q24 67 78 131q105 128 235 122q82 -2 138 -33.5t82 -81.5q46 -88 46 -170.5t-80 -219.5l-57 -96q-18 -32 -42 -106.5t-24 -143.5v-256h-190v256q0 102 24.5 195t48 140t65.5 118t50 105t-9 67.5t-60 34.5t-78 -48t-49 -98zM199 0h215v227h-215v-227z" /> | |||
|
46 | <glyph unicode="@" horiz-adv-x="872" d="M66 303v889q0 97 73 200q39 56 117 93t184.5 37t184 -37t116.5 -93q74 -105 74 -200v-793h-164l-20 56q-14 -28 -46 -48t-67 -20q-145 0 -145 172v485q0 170 145 170q71 0 113 -67v45q0 51 -45 104.5t-145.5 53.5t-145.5 -53.5t-45 -104.5v-889q0 -53 44 -103t153.5 -50 t160.5 63l152 -86q-109 -143 -320 -143q-106 0 -184 35.5t-117 90.5q-73 102 -73 193zM535 573q0 -53 48 -53t48 53v455q0 53 -48 53t-48 -53v-455z" /> | |||
|
47 | <glyph unicode="A" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM307 541h152l-64 475l-6 39h-12z" /> | |||
|
48 | <glyph unicode="B" horiz-adv-x="745" d="M82 0v1505h194q205 0 304.5 -91t99.5 -308q0 -106 -29.5 -175t-107.5 -136q14 -5 47 -38.5t54 -71.5q52 -97 52 -259q0 -414 -342 -426h-272zM303 219q74 0 109 31q55 56 55 211t-63 195q-42 26 -93 26h-8v-463zM303 885q87 0 119 39q45 55 45 138t-14.5 124t-30.5 60.5 t-45 28.5q-35 11 -74 11v-401z" /> | |||
|
49 | <glyph unicode="C" horiz-adv-x="708" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-207h-206v207q-2 0 0 11.5t-3.5 27.5t-12.5 33q-17 39 -68 39q-70 -10 -78 -111v-887q0 -43 21.5 -76.5t59.5 -33.5t59.5 27.5t21.5 56.5v233h206v-207q0 -42 -17 -106t-45 -107 t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175z" /> | |||
|
50 | <glyph unicode="D" horiz-adv-x="761" d="M82 0v1505h174q270 0 346 -113q31 -46 50.5 -95.5t28.5 -139.5t12 -177t3 -228.5t-3 -228.5t-12 -176t-28.5 -138t-50.5 -95t-80 -68q-106 -46 -266 -46h-174zM303 221q117 0 140.5 78t23.5 399v111q0 322 -23.5 398.5t-140.5 76.5v-1063z" /> | |||
|
51 | <glyph unicode="E" horiz-adv-x="628" d="M82 0v1505h506v-227h-285v-395h205v-242h-205v-414h285v-227h-506z" /> | |||
|
52 | <glyph unicode="F" horiz-adv-x="616" d="M82 0v1505h526v-227h-305v-395h205v-228h-205v-655h-221z" /> | |||
|
53 | <glyph unicode="G" horiz-adv-x="737" d="M67 271.5q0 26.5 1 37.5v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-231h-221v231q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-905q0 -46 19.5 -78.5t54 -32.5t53 28t18.5 54l2 29v272h-88v187h309v-750h-131l-26 72 q-70 -88 -172 -88q-203 0 -250 213q-11 48 -11 74.5z" /> | |||
|
54 | <glyph unicode="H" horiz-adv-x="778" d="M82 0v1505h221v-622h172v622h221v-1505h-221v655h-172v-655h-221z" /> | |||
|
55 | <glyph unicode="I" horiz-adv-x="385" d="M82 0v1505h221v-1505h-221z" /> | |||
|
56 | <glyph unicode="J" horiz-adv-x="423" d="M12 -14v217q4 0 12.5 -1t29 2t35.5 12t28.5 34.5t13.5 62.5v1192h221v-1226q0 -137 -74 -216q-74 -78 -223 -78h-4q-19 0 -39 1z" /> | |||
|
57 | <glyph unicode="K" horiz-adv-x="768" d="M82 0v1505h221v-526h8l195 526h215l-203 -495l230 -1010h-216l-153 655l-6 31h-6l-64 -154v-532h-221z" /> | |||
|
58 | <glyph unicode="L" horiz-adv-x="604" d="M82 0v1505h221v-1300h293v-205h-514z" /> | |||
|
59 | <glyph unicode="M" horiz-adv-x="991" d="M82 0v1505h270l131 -688l11 -80h4l10 80l131 688h270v-1505h-204v1010h-13l-149 -1010h-94l-142 946l-8 64h-12v-1010h-205z" /> | |||
|
60 | <glyph unicode="N" horiz-adv-x="808" d="M82 0v1505h197l215 -784l18 -70h12v854h203v-1505h-197l-215 784l-18 70h-12v-854h-203z" /> | |||
|
61 | <glyph unicode="O" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5l2 26v887q0 46 -19.5 78.5 t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
62 | <glyph unicode="P" horiz-adv-x="720" d="M82 0v1505h221q166 0 277.5 -105.5t111.5 -345t-111.5 -346t-277.5 -106.5v-602h-221zM303 827q102 0 134 45.5t32 175.5t-33 181t-133 51v-453z" /> | |||
|
63 | <glyph unicode="Q" horiz-adv-x="729" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -94 -45 -182q33 -43 88 -53v-189q-160 0 -227 117q-55 -18 -125 -18t-130 33.5t-88 81.5q-55 94 -60 175zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5l2 26v887 q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
64 | <glyph unicode="R" horiz-adv-x="739" d="M82 0v1505h221q377 0 377 -434q0 -258 -123 -342l141 -729h-221l-115 635h-59v-635h-221zM303 840q117 0 149 98q15 49 15 125t-15.5 125t-45.5 68q-44 30 -103 30v-446z" /> | |||
|
65 | <glyph unicode="S" horiz-adv-x="702" d="M37 422l217 20q0 -256 104 -256q90 0 91 166q0 59 -32 117.5t-45 79.5l-54 79q-40 58 -77 113t-73.5 117t-68 148.5t-31.5 162.5q0 139 71.5 245t216.5 108h10q88 0 152 -36t94 -100q54 -120 54 -264l-217 -20q0 217 -89 217q-75 -2 -75 -146q0 -59 23 -105 q32 -66 58 -104l197 -296q31 -49 67 -139.5t36 -166.5q0 -378 -306 -378h-2q-229 0 -290 188q-31 99 -31 250z" /> | |||
|
66 | <glyph unicode="T" horiz-adv-x="647" d="M4 1278v227h639v-227h-209v-1278h-221v1278h-209z" /> | |||
|
67 | <glyph unicode="U" horiz-adv-x="749" d="M80 309v1196h221v-1196q0 -46 19.5 -78t54.5 -32t53 27.5t18 56.5l3 26v1196h221v-1196q0 -42 -17.5 -106t-45 -107t-88 -77.5t-144.5 -34.5t-144.5 33.5t-88.5 81.5q-55 97 -60 175z" /> | |||
|
68 | <glyph unicode="V" horiz-adv-x="716" d="M18 1505h215l111 -827l8 -64h13l118 891h215l-229 -1505h-221z" /> | |||
|
69 | <glyph unicode="W" horiz-adv-x="1036" d="M25 1505h204l88 -782l5 -49h16l100 831h160l100 -831h17l92 831h205l-203 -1505h-172l-115 801h-8l-115 -801h-172z" /> | |||
|
70 | <glyph unicode="X" horiz-adv-x="737" d="M16 0l244 791l-240 714h218l120 -381l7 -18h8l127 399h217l-240 -714l244 -791h-217l-127 449l-4 18h-8l-132 -467h-217z" /> | |||
|
71 | <glyph unicode="Y" horiz-adv-x="700" d="M14 1505h217l111 -481l6 -14h4l6 14l111 481h217l-225 -864v-641h-221v641z" /> | |||
|
72 | <glyph unicode="Z" horiz-adv-x="626" d="M20 0v238l347 1048h-297v219h536v-219l-352 -1067h352v-219h-586z" /> | |||
|
73 | <glyph unicode="[" horiz-adv-x="538" d="M82 -213v1718h399v-196h-202v-1325h202v-197h-399z" /> | |||
|
74 | <glyph unicode="\" horiz-adv-x="792" d="M8 1692h162l614 -1872h-168z" /> | |||
|
75 | <glyph unicode="]" horiz-adv-x="538" d="M57 -16h203v1325h-203v196h400v-1718h-400v197z" /> | |||
|
76 | <glyph unicode="^" horiz-adv-x="1101" d="M53 809l381 696h234l381 -696h-199l-299 543l-299 -543h-199z" /> | |||
|
77 | <glyph unicode="_" horiz-adv-x="1210" d="M74 -154h1063v-172h-1063v172z" /> | |||
|
78 | <glyph unicode="`" horiz-adv-x="1024" d="M293 1489h215l106 -184h-159z" /> | |||
|
79 | <glyph unicode="a" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM252 291 q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250z" /> | |||
|
80 | <glyph unicode="b" horiz-adv-x="686" d="M82 0v1505h207v-458q88 90 165 90t117.5 -69t40.5 -150v-715q0 -82 -41 -150.5t-118 -68.5q-33 0 -74 22.5t-66 44.5l-24 23v-74h-207zM289 246q0 -29 19.5 -48.5t42 -19.5t39 19.5t16.5 48.5v628q0 29 -16.5 48.5t-39 19.5t-42 -21.5t-19.5 -46.5v-628z" /> | |||
|
81 | <glyph unicode="c" horiz-adv-x="645" d="M66 315v490q0 332 264 332q137 0 201.5 -71t64.5 -251v-88h-207v135q0 51 -12 70.5t-47 19.5q-58 0 -58 -90v-604q0 -90 58 -90q35 0 47 19.5t12 70.5v156h207v-109q0 -180 -64.5 -250.5t-201.5 -70.5q-264 0 -264 331z" /> | |||
|
82 | <glyph unicode="d" horiz-adv-x="686" d="M74 203v715q0 82 41 150.5t118 68.5q33 0 74 -22.5t66 -45.5l24 -22v458h207v-1505h-207v74q-88 -90 -165 -90t-117.5 68.5t-40.5 150.5zM281 246q0 -29 16 -48.5t38.5 -19.5t42 19.5t19.5 48.5v628q0 25 -19.5 46.5t-42 21.5t-38.5 -19.5t-16 -48.5v-628z" /> | |||
|
83 | <glyph unicode="e" horiz-adv-x="659" d="M66 279v563q0 36 16 94.5t42 97.5t81 71t129 32q199 0 252 -197q14 -51 14 -92v-326h-342v-256q0 -59 39 -88q16 -12 37 -12q70 10 74 113v122h192v-129q0 -37 -16.5 -93t-41 -95t-79.5 -69.5t-130 -30.5t-130.5 30.5t-80.5 73.5q-49 87 -54 160zM258 684h150v158 q0 48 -19.5 81t-53.5 33t-53.5 -28.5t-21.5 -57.5l-2 -28v-158z" /> | |||
|
84 | <glyph unicode="f" horiz-adv-x="475" d="M20 934v186h105v31q0 190 51 270q23 35 71 63.5t115 28.5l97 -14v-178q-27 8 -62 8q-65 0 -65 -175v-5v-29h104v-186h-104v-934h-207v934h-105z" /> | |||
|
85 | <glyph unicode="g" horiz-adv-x="700" d="M12 -184q0 94 162 170q-125 35 -125 149q0 45 40 93t89 75q-51 35 -80.5 95.5t-34.5 105.5l-4 43v305q0 35 16.5 91t41 94t79 69t126.5 31q135 0 206 -103q102 102 170 103v-185q-72 0 -120 -24l10 -70v-317q0 -37 -17.5 -90.5t-42 -90t-79 -66.5t-104.5 -30t-62 2 q-29 -25 -29 -46t11 -33.5t42 -20.5t45.5 -10t65.5 -10.5t95 -21.5t89 -41q96 -60 96 -205t-103 -212q-100 -65 -250 -65h-9q-156 2 -240 50t-84 165zM213 -150q0 -77 132 -77h3q59 0 108.5 19t49.5 54t-20.5 52.5t-90.5 29.5l-106 21q-76 -43 -76 -99zM262 509 q0 -17 15.5 -45t44.5 -28q63 6 63 101v307q-2 0 0 10q1 3 1 7q0 8 -3 19q-4 15 -9 30q-11 36 -46 36t-50.5 -25.5t-15.5 -52.5v-359z" /> | |||
|
86 | <glyph unicode="h" horiz-adv-x="690" d="M82 0v1505h207v-479l32 32q79 79 145.5 79t106 -69t39.5 -150v-918h-206v887q-1 49 -50 49q-41 0 -67 -53v-883h-207z" /> | |||
|
87 | <glyph unicode="i" horiz-adv-x="370" d="M82 0v1120h207v-1120h-207zM82 1298v207h207v-207h-207z" /> | |||
|
88 | <glyph unicode="j" horiz-adv-x="364" d="M-45 -182q29 -8 57 -8q64 0 64 142v1168h207v-1149q0 -186 -51 -266q-23 -35 -71 -62.5t-115 -27.5t-91 12v191zM76 1298v207h207v-207h-207z" /> | |||
|
89 | <glyph unicode="k" horiz-adv-x="641" d="M82 0v1505h207v-714h10l113 329h186l-149 -364l188 -756h-199l-102 453l-4 16h-10l-33 -82v-387h-207z" /> | |||
|
90 | <glyph unicode="l" horiz-adv-x="370" d="M82 0v1505h207v-1505h-207z" /> | |||
|
91 | <glyph unicode="m" horiz-adv-x="1021" d="M82 0v1120h207v-94q2 0 33 30q80 81 139 81q100 0 139 -125q125 125 194.5 125t109.5 -69t40 -150v-918h-194v887q-1 49 -56 49q-41 0 -78 -53v-883h-194v887q0 49 -55 49q-41 0 -78 -53v-883h-207z" /> | |||
|
92 | <glyph unicode="n" horiz-adv-x="690" d="M82 0v1120h207v-94l32 32q79 79 145.5 79t106 -69t39.5 -150v-918h-206v887q-1 49 -50 49q-41 0 -67 -53v-883h-207z" /> | |||
|
93 | <glyph unicode="o" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM257 259q0 -17 9 -44q18 -49 62 -49q70 10 71 113v563l1 19q0 19 -10 45q-18 50 -62 50 q-68 -10 -70 -114v-563q1 -1 1 -4z" /> | |||
|
94 | <glyph unicode="p" horiz-adv-x="686" d="M82 -385v1505h207v-73q88 90 165 90t117.5 -69t40.5 -150v-715q0 -82 -41 -150.5t-118 -68.5q-33 0 -74 22.5t-66 44.5l-24 23v-459h-207zM289 246q0 -25 19.5 -46.5t42 -21.5t39 19.5t16.5 48.5v628q0 29 -16.5 48.5t-39 19.5t-42 -19.5t-19.5 -48.5v-628z" /> | |||
|
95 | <glyph unicode="q" horiz-adv-x="686" d="M74 203v715q0 82 41 150.5t118 68.5q33 0 74 -22.5t66 -45.5l24 -22v73h207v-1505h-207v459q-88 -90 -165 -90t-117.5 68.5t-40.5 150.5zM281 246q0 -29 16 -48.5t38.5 -19.5t42 21.5t19.5 46.5v628q0 29 -19.5 48.5t-42 19.5t-38.5 -19.5t-16 -48.5v-628z" /> | |||
|
96 | <glyph unicode="r" horiz-adv-x="503" d="M82 0v1120h207v-125q8 41 58.5 91.5t148.5 50.5v-230q-34 11 -77 11t-86.5 -39t-43.5 -101v-778h-207z" /> | |||
|
97 | <glyph unicode="s" horiz-adv-x="630" d="M37 326h192q0 -170 97 -170q71 0 71 131q0 78 -129 202q-68 66 -98.5 99t-64 101.5t-33.5 134t12 114.5t39 95q59 100 201 104h11q161 0 211 -105q42 -86 42 -198h-193q0 131 -67 131q-63 -2 -64 -131q0 -33 23.5 -73t45 -62.5t66.5 -65.5q190 -182 191 -342 q0 -123 -64.5 -215t-199.5 -92q-197 0 -260 170q-29 76 -29 172z" /> | |||
|
98 | <glyph unicode="t" horiz-adv-x="501" d="M20 934v186h105v277h207v-277h141v-186h-141v-557q0 -184 65 -184l76 8v-203q-45 -14 -112 -14t-114.5 28.5t-70 64.5t-34.5 96q-17 79 -17 187v574h-105z" /> | |||
|
99 | <glyph unicode="u" horiz-adv-x="690" d="M78 203v917h207v-887q0 -49 49 -49q41 0 67 54v882h207v-1120h-207v94l-31 -32q-78 -78 -145.5 -78t-107 68.5t-39.5 150.5z" /> | |||
|
100 | <glyph unicode="v" horiz-adv-x="602" d="M16 1120h201l68 -649l8 -72h16l76 721h201l-183 -1120h-204z" /> | |||
|
101 | <glyph unicode="w" horiz-adv-x="905" d="M20 1120h189l65 -585l9 -64h12l96 649h123l86 -585l10 -64h13l73 649h189l-166 -1120h-172l-80 535l-10 63h-8l-91 -598h-172z" /> | |||
|
102 | <glyph unicode="x" horiz-adv-x="618" d="M16 0l193 578l-176 542h194l74 -262l6 -31h4l6 31l74 262h195l-176 -542l192 -578h-201l-84 283l-6 30h-4l-6 -30l-84 -283h-201z" /> | |||
|
103 | <glyph unicode="y" horiz-adv-x="634" d="M25 1120h202l82 -688l4 -57h9l4 57l82 688h202l-198 -1204q-16 -127 -94 -222t-193 -95l-92 4v184q16 -4 49 -4q61 6 97 61.5t36 122.5z" /> | |||
|
104 | <glyph unicode="z" horiz-adv-x="532" d="M12 0v168l285 764h-240v188h459v-168l-285 -764h285v-188h-504z" /> | |||
|
105 | <glyph unicode="{" horiz-adv-x="688" d="M61 453v163q72 0 102 49.5t30 90.5v397q0 223 96 298t342 71v-172q-135 2 -188.5 -38t-53.5 -159v-397q0 -143 -127 -221q127 -82 127 -222v-397q0 -119 53.5 -159t188.5 -38v-172q-246 -4 -342 71t-96 298v397q0 57 -41 97.5t-91 42.5z" /> | |||
|
106 | <glyph unicode="|" horiz-adv-x="356" d="M82 -512v2204h192v-2204h-192z" /> | |||
|
107 | <glyph unicode="}" horiz-adv-x="688" d="M57 -281q135 -2 188.5 38t53.5 159v397q0 139 127 222q-127 78 -127 221v397q0 119 -53 159t-189 38v172q246 4 342.5 -71t96.5 -298v-397q0 -63 41 -101.5t90 -38.5v-163q-72 -4 -101.5 -52.5t-29.5 -87.5v-397q0 -223 -96.5 -298t-342.5 -71v172z" /> | |||
|
108 | <glyph unicode="~" horiz-adv-x="1280" d="M113 1352q35 106 115 200q34 41 94.5 74t121 33t116.5 -18.5t82 -33t83 -51.5q106 -72 174 -71q109 0 178 153l13 29l135 -57q-63 -189 -206 -276q-56 -34 -120 -34q-121 0 -272 101q-115 74 -178.5 74t-113.5 -45.5t-69 -90.5l-18 -45z" /> | |||
|
109 | <glyph unicode="¡" horiz-adv-x="387" d="M74 -385l55 1100h129l55 -1100h-239zM86 893v227h215v-227h-215z" /> | |||
|
110 | <glyph unicode="¢" horiz-adv-x="636" d="M66 508v489q0 297 208 328v242h123v-244q98 -16 144.5 -88t46.5 -227v-88h-189v135q0 90 -72.5 90t-72.5 -90v-604q0 -90 72 -91q74 0 73 91v155h189v-108q0 -156 -46 -228.5t-145 -89.5v-303h-123v301q-209 31 -208 330z" /> | |||
|
111 | <glyph unicode="£" horiz-adv-x="817" d="M4 63q8 20 23.5 53.5t70 91.5t117.5 68q37 111 37 189t-31 184h-188v137h147l-6 21q-78 254 -78 333t15.5 140t48.5 116q72 122 231 126q190 4 267 -126q65 -108 65 -276h-213q0 201 -115 197q-47 -2 -68.5 -51t-21.5 -139.5t70 -315.5l6 -25h211v-137h-174 q25 -100 24.5 -189t-57.5 -204q16 -8 44 -24q59 -35 89 -35q74 4 82 190l188 -22q-12 -182 -81.5 -281.5t-169.5 -99.5q-51 0 -143.5 51t-127.5 51t-63.5 -25.5t-40.5 -52.5l-12 -24z" /> | |||
|
112 | <glyph unicode="¥" horiz-adv-x="720" d="M25 1505h217l110 -481l6 -14h4l7 14l110 481h217l-196 -753h147v-138h-176v-137h176v-137h-176v-340h-221v340h-176v137h176v137h-176v138h147z" /> | |||
|
113 | <glyph unicode="¨" horiz-adv-x="1024" d="M272 1305v200h191v-200h-191zM561 1305v200h191v-200h-191z" /> | |||
|
114 | <glyph unicode="©" horiz-adv-x="1644" d="M53 751.5q0 317.5 225.5 544t543 226.5t543.5 -226.5t226 -544t-226 -542.5t-543.5 -225t-543 225t-225.5 542.5zM172 751.5q0 -266.5 191.5 -458t457.5 -191.5t459 191.5t193 459t-191.5 459t-459 191.5t-459 -192.5t-191.5 -459zM627 487v531q0 122 97 174q40 22 95 22 q147 0 182 -147l7 -49v-125h-138v142q0 11 -12 28.5t-37 17.5q-47 -2 -49 -63v-531q0 -63 49 -63q53 2 49 63v125h138v-125q0 -68 -40 -127q-18 -26 -57 -47.5t-108.5 -21.5t-117.5 49t-54 98z" /> | |||
|
115 | <glyph unicode="ª" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM252 291 q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250z" /> | |||
|
116 | <glyph unicode="­" horiz-adv-x="444" d="M74 455v194h297v-194h-297z" /> | |||
|
117 | <glyph unicode="®" horiz-adv-x="1644" d="M53 751.5q0 317.5 225.5 544t543 226.5t543.5 -226.5t226 -544t-226 -542.5t-543.5 -225t-543 225t-225.5 542.5zM172 751.5q0 -266.5 191.5 -458t457.5 -191.5t459 191.5t193 459t-191.5 459t-459 191.5t-459 -192.5t-191.5 -459zM625 313v879h196q231 0 232 -258 q0 -76 -16.5 -125t-71.5 -96l106 -400h-151l-95 365h-55v-365h-145zM770 805h45q43 0 65.5 21.5t27.5 45t5 61.5t-5 62.5t-27.5 46t-65.5 21.5h-45v-258z" /> | |||
|
118 | <glyph unicode="¯" horiz-adv-x="1024" d="M313 1315v162h398v-162h-398z" /> | |||
|
119 | <glyph unicode="²" horiz-adv-x="731" d="M55 0v219l39 62q25 39 88.5 152.5t112.5 220t91 241.5t44 238q0 184 -73.5 184t-73.5 -184v-105h-222v105q0 389 295 389t295 -375q0 -336 -346 -928h350v-219h-600z" /> | |||
|
120 | <glyph unicode="³" horiz-adv-x="686" d="M45 1071q0 249 63 343q29 42 84.5 75t134.5 33t136 -31t84.5 -71t44.5 -92q22 -71 22 -130q0 -291 -108 -399q127 -100 127 -414q0 -68 -19.5 -145.5t-47 -128t-85 -89t-136.5 -38.5t-135 31.5t-86 75.5t-48 113q-23 91 -23 230h217q2 -150 17.5 -203t59.5 -53t56.5 50.5 t12.5 104.5t1 102t0 63q-6 82 -14 95l-18 33q-12 22 -29 29q-55 22 -108 25h-19v184q133 7 156 73q12 34 12 91v105q0 146 -29 177q-16 17 -40 17q-41 0 -52.5 -49t-13.5 -207h-217z" /> | |||
|
121 | <glyph unicode="´" horiz-adv-x="1024" d="M410 1305l106 184h215l-162 -184h-159z" /> | |||
|
122 | <glyph unicode="·" horiz-adv-x="215" d="M0 649v228h215v-228h-215z" /> | |||
|
123 | <glyph unicode="¸" horiz-adv-x="1024" d="M426 -111h172v-141l-45 -133h-104l40 133h-63v141z" /> | |||
|
124 | <glyph unicode="¹" horiz-adv-x="475" d="M25 1180v141q129 25 205 130q16 21 30 54h133v-1505h-221v1180h-147z" /> | |||
|
125 | <glyph unicode="º" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM257 259q0 -17 9 -44q18 -49 62 -49q70 10 71 113v563l1 19q0 19 -10 45q-18 50 -62 50 q-68 -10 -70 -114v-563q1 -1 1 -4z" /> | |||
|
126 | <glyph unicode="¿" horiz-adv-x="645" d="M41 -106q0 82 80 219l57 95q18 32 42 106.5t24 144.5v256h190v-256q0 -102 -24.5 -195.5t-48 -140.5t-65.5 -118t-50 -104.5t9 -67.5t60 -35t78 48.5t49 98.5l179 -84q-24 -66 -78 -132q-104 -126 -236 -122q-163 4 -220 115q-46 90 -46 172zM231 893v227h215v-227h-215z " /> | |||
|
127 | <glyph unicode="À" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM141 1823h215l107 -185h-160zM307 541h152l-64 475l-6 39h-12z" /> | |||
|
128 | <glyph unicode="Á" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM293 1638l106 185h215l-161 -185h-160zM307 541h152l-64 475l-6 39h-12z" /> | |||
|
129 | <glyph unicode="Â" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM133 1638l141 185h220l141 -185h-189l-63 72l-61 -72h-189zM307 541h152l-64 475l-6 39h-12z" /> | |||
|
130 | <glyph unicode="Ã" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM184 1632v152q49 39 95.5 39t104.5 -18.5t100.5 -19.5t97.5 32v-152q-51 -39 -95.5 -39t-102.5 19.5t-98 19.5t-102 -33zM307 541h152l-64 475l-6 39h-12z" /> | |||
|
131 | <glyph unicode="Ä" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM143 1638v201h191v-201h-191zM307 541h152l-64 475l-6 39h-12zM432 1638v201h191v-201h-191z" /> | |||
|
132 | <glyph unicode="Å" horiz-adv-x="765" d="M20 0l228 1505h270l227 -1505h-215l-41 307h-213l-40 -307h-216zM231 1761.5q0 61.5 45.5 102.5t109 41t107.5 -41t44 -102.5t-44 -102.5t-107.5 -41t-109 41t-45.5 102.5zM307 541h152l-64 475l-6 39h-12zM309 1761.5q0 -28.5 23.5 -50t52.5 -21.5t52.5 21.5t23.5 50 t-23.5 50t-52.5 21.5t-52.5 -21.5t-23.5 -50z" /> | |||
|
133 | <glyph unicode="Æ" horiz-adv-x="1099" d="M16 0l420 1505h623v-227h-285v-395h205v-242h-205v-414h285v-227h-506v307h-227l-90 -307h-220zM393 541h160v514h-10z" /> | |||
|
134 | <glyph unicode="Ç" horiz-adv-x="708" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-207h-206v207q-2 0 0 11.5t-3.5 27.5t-12.5 33q-17 39 -68 39q-70 -10 -78 -111v-887q0 -43 21.5 -76.5t59.5 -33.5t59.5 27.5t21.5 56.5v233h206v-207q0 -42 -17 -106t-45 -107 t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM268 -111v-141h64l-41 -133h104l45 133v141h-172z" /> | |||
|
135 | <glyph unicode="È" horiz-adv-x="628" d="M82 0v1505h506v-227h-285v-395h205v-242h-205v-414h285v-227h-506zM111 1823h215l106 -185h-160z" /> | |||
|
136 | <glyph unicode="É" horiz-adv-x="628" d="M82 0v1505h506v-227h-285v-395h205v-242h-205v-414h285v-227h-506zM236 1638l106 185h215l-162 -185h-159z" /> | |||
|
137 | <glyph unicode="Ê" horiz-adv-x="628" d="M82 0v1505h506v-227h-285v-395h205v-242h-205v-414h285v-227h-506zM84 1638l141 185h219l142 -185h-189l-63 72l-62 -72h-188z" /> | |||
|
138 | <glyph unicode="Ë" horiz-adv-x="628" d="M82 0v1505h506v-227h-285v-395h205v-242h-205v-414h285v-227h-506zM94 1638v201h191v-201h-191zM383 1638v201h190v-201h-190z" /> | |||
|
139 | <glyph unicode="Ì" horiz-adv-x="401" d="M-6 1823h215l106 -185h-159zM98 0v1505h221v-1505h-221z" /> | |||
|
140 | <glyph unicode="Í" horiz-adv-x="401" d="M82 0v1505h221v-1505h-221zM86 1638l107 185h215l-162 -185h-160z" /> | |||
|
141 | <glyph unicode="Î" horiz-adv-x="370" d="M-66 1638l142 185h219l141 -185h-188l-64 72l-61 -72h-189zM74 0v1505h221v-1505h-221z" /> | |||
|
142 | <glyph unicode="Ï" horiz-adv-x="372" d="M-53 1638v201h190v-201h-190zM76 0v1505h221v-1505h-221zM236 1638v201h190v-201h-190z" /> | |||
|
143 | <glyph unicode="Ð" horiz-adv-x="761" d="M20 655v228h62v622h174q270 0 346 -113q31 -46 50.5 -95.5t28.5 -139.5t12 -177t3 -228.5t-3 -228.5t-12 -176t-28.5 -138t-50.5 -95t-80 -68q-106 -46 -266 -46h-174v655h-62zM303 221q117 0 141.5 81t22.5 452q2 371 -22.5 450.5t-141.5 79.5v-401h84v-228h-84v-434z " /> | |||
|
144 | <glyph unicode="Ñ" horiz-adv-x="808" d="M82 0v1505h197l215 -784l18 -70h12v854h203v-1505h-197l-215 784l-18 70h-12v-854h-203zM207 1632v152q49 39 95 39t104.5 -18.5t102.5 -19.5t95 32v-152q-51 -39 -95 -39t-102.5 19.5t-100 19.5t-99.5 -33z" /> | |||
|
145 | <glyph unicode="Ò" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM121 1823h215l106 -185h-159zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5 l2 26v887q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
146 | <glyph unicode="Ó" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM285 1638l106 185h215l-162 -185h-159zM289 309q0 -46 19.5 -78t54 -32t53 27.5 t18.5 56.5l2 26v887q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
147 | <glyph unicode="Ô" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM113 1638l141 185h219l141 -185h-188l-64 72l-61 -72h-188zM289 309q0 -46 19.5 -78 t54 -32t53 27.5t18.5 56.5l2 26v887q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
148 | <glyph unicode="Õ" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM164 1632v152q49 39 95 39t104.5 -18.5t102.5 -19.5t95 32v-152q-51 -39 -95 -39 t-102.5 19.5t-100 19.5t-99.5 -33zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5l2 26v887q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887z" /> | |||
|
149 | <glyph unicode="Ö" d="M68 309v887q0 42 17 106t45 107t88.5 78t144 35t144 -34t88.5 -81q55 -93 60 -178l2 -33v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-144 -34.5t-144 33.5t-88.5 81.5q-55 94 -60 175zM123 1638v201h190v-201h-190zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5 l2 26v887q0 46 -19.5 78.5t-54 32.5t-53 -28t-18.5 -54l-2 -29v-887zM412 1638v201h190v-201h-190z" /> | |||
|
150 | <glyph unicode="Ø" d="M59 -20l47 157q-36 74 -36 148l-2 24v887q0 42 17 106t45 107t88.5 78t148 35t153.5 -43l15 47h122l-45 -150q43 -84 43 -155l2 -25v-887q0 -42 -17 -106t-45 -107t-88.5 -77.5t-150.5 -34.5t-153 43l-15 -47h-129zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5 l2 26v488zM289 727l147 479q-8 100 -74 101q-35 0 -53 -28t-18 -54l-2 -29v-469z" /> | |||
|
151 | <glyph unicode="Ù" horiz-adv-x="749" d="M80 309q0 -42 17.5 -106t45 -107t88 -77.5t144.5 -34.5t144.5 33.5t88.5 81.5q55 97 60 175l2 35v1196h-221v-1196q0 -44 -19.5 -77t-54.5 -33t-53.5 27.5t-18.5 56.5l-2 26v1196h-221v-1196zM145 1823h215l107 -185h-160z" /> | |||
|
152 | <glyph unicode="Ú" horiz-adv-x="749" d="M80 309q0 -42 17.5 -106t45 -107t88 -77.5t144.5 -34.5t144.5 33.5t88.5 81.5q55 97 60 175l2 35v1196h-221v-1196q0 -44 -19.5 -77t-54.5 -33t-53.5 27.5t-18.5 56.5l-2 26v1196h-221v-1196zM307 1638l107 185h215l-162 -185h-160z" /> | |||
|
153 | <glyph unicode="Û" horiz-adv-x="749" d="M80 309q0 -42 17.5 -106t45 -107t88 -77.5t144.5 -34.5t144.5 33.5t88.5 81.5q55 97 60 175l2 35v1196h-221v-1196q0 -44 -19.5 -77t-54.5 -33t-53.5 27.5t-18.5 56.5l-2 26v1196h-221v-1196zM125 1638l141 185h219l142 -185h-189l-63 72l-62 -72h-188z" /> | |||
|
154 | <glyph unicode="Ü" horiz-adv-x="749" d="M80 309v1196h221v-1196q0 -46 19.5 -78t54.5 -32t53 27.5t18 56.5l3 26v1196h221v-1196q0 -42 -17.5 -106t-45 -107t-88 -77.5t-144.5 -34.5t-144.5 33.5t-88.5 81.5q-55 97 -60 175zM135 1638v201h191v-201h-191zM424 1638v201h190v-201h-190z" /> | |||
|
155 | <glyph unicode="Ý" horiz-adv-x="704" d="M16 1505l226 -864v-641h221v641l225 864h-217l-111 -481l-6 -14h-4l-6 14l-111 481h-217zM254 1638l106 185h215l-161 -185h-160z" /> | |||
|
156 | <glyph unicode="Þ" d="M82 0v1505h219v-241h2q166 0 277.5 -105.5t111.5 -345.5t-111.5 -346.5t-277.5 -106.5v-360h-221zM303 586q102 0 134 45t32 175t-33 181t-133 51v-452z" /> | |||
|
157 | <glyph unicode="ß" horiz-adv-x="733" d="M66 0v1235q0 123 70.5 205t206.5 82t204.5 -81t68.5 -197t-88 -181q152 -88 152 -488q0 -362 -87 -475q-46 -59 -102.5 -79.5t-144.5 -20.5v193q45 0 70 25q57 57 57 357q0 316 -57 377q-25 27 -70 27v141q35 0 60.5 33t25.5 84q0 100 -86 100q-74 0 -74 -102v-1235h-206 z" /> | |||
|
158 | <glyph unicode="à" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM102 1489h215 l107 -184h-160zM252 291q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250z" /> | |||
|
159 | <glyph unicode="á" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM252 291 q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250zM264 1305l107 184h215l-162 -184h-160z" /> | |||
|
160 | <glyph unicode="â" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM90 1305 l141 184h220l141 -184h-189l-63 71l-61 -71h-189zM252 291q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250z" /> | |||
|
161 | <glyph unicode="ã" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM143 1305v151 q49 39 95.5 39t104.5 -18.5t97 -19.5t101 32v-152q-51 -39 -95.5 -39t-102.5 19.5t-99 19.5t-101 -32zM252 291q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250z" /> | |||
|
162 | <glyph unicode="ä" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM102 1305v200 h191v-200h-191zM252 291q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250zM391 1305v200h191v-200h-191z" /> | |||
|
163 | <glyph unicode="å" horiz-adv-x="681" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t190 88t184.5 -74t75 -180v-688q0 -109 14 -195h-202q-18 20 -19 90h-14q-20 -37 -65.5 -71.5t-102.5 -34.5t-110.5 60t-53.5 191zM188 1421.5 q0 61.5 45.5 102.5t109 41t107.5 -41t44 -102.5t-44 -102.5t-107.5 -41t-109 41t-45.5 102.5zM252 291q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250zM266 1421.5q0 -28.5 23.5 -50t52.5 -21.5t52.5 21.5t23.5 50t-23.5 50t-52.5 21.5t-52.5 -21.5 t-23.5 -50z" /> | |||
|
164 | <glyph unicode="æ" horiz-adv-x="989" d="M49 235q0 131 34 212t83 124t98 73t88 50.5t43 36.5v123q0 102 -57 102q-41 0 -50 -42t-9 -84v-39h-207v47q0 123 80.5 211t197.5 88q84 0 152 -52q66 51 162 52q199 0 251 -197q14 -51 15 -92v-326h-342v-256q0 -60 38 -88q17 -12 38 -12q70 10 73 113v122h193v-129 q0 -37 -16.5 -93t-41 -95t-80 -69.5t-130.5 -30.5q-158 0 -226 131q-102 -131 -221 -131q-59 0 -112.5 60t-53.5 191zM252 291q0 -104 57 -105q35 0 60.5 19.5t25.5 48.5v287q-143 -62 -143 -250zM588 684h149v158q0 48 -19.5 81t-53 33t-53 -28.5t-21.5 -57.5l-2 -28v-158z " /> | |||
|
165 | <glyph unicode="ç" horiz-adv-x="645" d="M66 315v490q0 332 264 332q137 0 201.5 -71t64.5 -251v-88h-207v135q0 51 -12 70.5t-47 19.5q-58 0 -58 -90v-604q0 -90 58 -90q35 0 47 19.5t12 70.5v156h207v-109q0 -180 -64.5 -250.5t-201.5 -70.5q-264 0 -264 331zM238 -111v-141h63l-41 -133h105l45 133v141h-172z " /> | |||
|
166 | <glyph unicode="è" horiz-adv-x="659" d="M66 279v563q0 36 16 94.5t42 97.5t81 71t129 32q199 0 252 -197q14 -51 14 -92v-326h-342v-256q0 -59 39 -88q16 -12 37 -12q70 10 74 113v122h192v-129q0 -37 -16.5 -93t-41 -95t-79.5 -69.5t-130 -30.5t-130.5 30.5t-80.5 73.5q-49 87 -54 160zM102 1489h215l107 -184 h-160zM258 684h150v158q0 48 -19.5 81t-53.5 33t-53.5 -28.5t-21.5 -57.5l-2 -28v-158z" /> | |||
|
167 | <glyph unicode="é" horiz-adv-x="659" d="M66 279v563q0 36 16 94.5t42 97.5t81 71t129 32q199 0 252 -197q14 -51 14 -92v-326h-342v-256q0 -59 39 -88q16 -12 37 -12q70 10 74 113v122h192v-129q0 -37 -16.5 -93t-41 -95t-79.5 -69.5t-130 -30.5t-130.5 30.5t-80.5 73.5q-49 87 -54 160zM258 684h150v158 q0 48 -19.5 81t-53.5 33t-53.5 -28.5t-21.5 -57.5l-2 -28v-158zM264 1305l107 184h215l-162 -184h-160z" /> | |||
|
168 | <glyph unicode="ê" horiz-adv-x="659" d="M66 279v563q0 36 16 94.5t42 97.5t81 71t129 32q199 0 252 -197q14 -51 14 -92v-326h-342v-256q0 -59 39 -88q16 -12 37 -12q70 10 74 113v122h192v-129q0 -37 -16.5 -93t-41 -95t-79.5 -69.5t-130 -30.5t-130.5 30.5t-80.5 73.5q-49 87 -54 160zM80 1305l141 184h219 l142 -184h-189l-63 71l-62 -71h-188zM258 684h150v158q0 48 -19.5 81t-53.5 33t-53.5 -28.5t-21.5 -57.5l-2 -28v-158z" /> | |||
|
169 | <glyph unicode="ë" horiz-adv-x="659" d="M66 279v563q0 36 16 94.5t42 97.5t81 71t129 32q199 0 252 -197q14 -51 14 -92v-326h-342v-256q0 -59 39 -88q16 -12 37 -12q70 10 74 113v122h192v-129q0 -37 -16.5 -93t-41 -95t-79.5 -69.5t-130 -30.5t-130.5 30.5t-80.5 73.5q-49 87 -54 160zM90 1305v200h191v-200 h-191zM258 684h150v158q0 48 -19.5 81t-53.5 33t-53.5 -28.5t-21.5 -57.5l-2 -28v-158zM379 1305v200h190v-200h-190z" /> | |||
|
170 | <glyph unicode="ì" horiz-adv-x="370" d="M-33 1489h215l107 -184h-160zM82 0h207v1120h-207v-1120z" /> | |||
|
171 | <glyph unicode="í" horiz-adv-x="370" d="M82 0h207v1120h-207v-1120zM82 1305l106 184h215l-161 -184h-160z" /> | |||
|
172 | <glyph unicode="î" horiz-adv-x="370" d="M-66 1305l142 184h219l141 -184h-188l-64 71l-61 -71h-189zM82 0h207v1120h-207v-1120z" /> | |||
|
173 | <glyph unicode="ï" horiz-adv-x="372" d="M-53 1305v200h190v-200h-190zM82 0v1120h207v-1120h-207zM236 1305v200h190v-200h-190z" /> | |||
|
174 | <glyph unicode="ð" horiz-adv-x="673" d="M76 279v579q0 279 172 279q63 0 155 -78q-12 109 -51 203l-82 -72l-55 63l100 88l-45 66l109 100q25 -27 53 -61l94 82l56 -66l-101 -88q125 -201 125 -446v-656q0 -102 -56 -188q-26 -39 -80 -69.5t-129 -30.5t-130 30.5t-80 73.5q-53 91 -53 160zM270 267.5 q-2 -11.5 2 -29t10 -34.5q16 -38 58 -38q70 10 72 113v563q-2 0 0 11t-2 28.5t-10 34.5q-16 40 -60 40q-68 -10 -70 -114v-563q2 0 0 -11.5z" /> | |||
|
175 | <glyph unicode="ñ" horiz-adv-x="690" d="M82 0v1120h207v-94l32 32q79 79 145.5 79t106 -69t39.5 -150v-918h-206v887q-1 49 -50 49q-41 0 -67 -53v-883h-207zM147 1305v151q49 39 95.5 39t105 -18.5t97 -19.5t100.5 32v-152q-51 -39 -95.5 -39t-102.5 19.5t-99 19.5t-101 -32z" /> | |||
|
176 | <glyph unicode="ò" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM98 1489h215l107 -184h-160zM258 267.5q-2 -11.5 2 -29t10 -34.5q14 -38 58 -38 q70 10 71 113v563q-2 0 0 11t-2 28.5t-10 34.5q-15 40 -59 40q-68 -10 -70 -114v-563q2 0 0 -11.5z" /> | |||
|
177 | <glyph unicode="ó" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM258 267.5q-2 -11.5 2 -29t10 -34.5q14 -38 58 -38q70 10 71 113v563q-2 0 0 11t-2 28.5 t-10 34.5q-15 40 -59 40q-68 -10 -70 -114v-563q2 0 0 -11.5zM260 1305l107 184h215l-162 -184h-160z" /> | |||
|
178 | <glyph unicode="ô" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM78 1305l141 184h219l142 -184h-189l-63 71l-62 -71h-188zM258 267.5q-2 -11.5 2 -29 t10 -34.5q14 -38 58 -38q70 10 71 113v563q-2 0 0 11t-2 28.5t-10 34.5q-15 40 -59 40q-68 -10 -70 -114v-563q2 0 0 -11.5z" /> | |||
|
179 | <glyph unicode="õ" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM131 1305v151q49 39 95.5 39t104.5 -18.5t98.5 -19.5t98.5 32v-152q-51 -39 -95 -39 t-102 19.5t-101 19.5t-99 -32zM258 267.5q-2 -11.5 2 -29t10 -34.5q14 -38 58 -38q70 10 71 113v563q-2 0 0 11t-2 28.5t-10 34.5q-15 40 -59 40q-68 -10 -70 -114v-563q2 0 0 -11.5z" /> | |||
|
180 | <glyph unicode="ö" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t129.5 32q199 0 252 -197q14 -51 14 -92v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-129 -30.5t-130 30.5t-80.5 73.5q-52 92 -52 160zM90 1305v200h191v-200h-191zM258 267.5q-2 -11.5 2 -29t10 -34.5q14 -38 58 -38 q70 10 71 113v563q-2 0 0 11t-2 28.5t-10 34.5q-15 40 -59 40q-68 -10 -70 -114v-563q2 0 0 -11.5zM379 1305v200h190v-200h-190z" /> | |||
|
181 | <glyph unicode="ø" horiz-adv-x="657" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t118 32t117.5 -19l21 80h75l-30 -121q88 -84 94 -229v-576q0 -102 -56 -188q-26 -39 -80.5 -69.5t-120.5 -30.5t-112 16l-20 -78h-80l31 121q-41 39 -64.5 97.5t-25.5 97.5zM258 436l125 486q-18 35 -55 34q-68 -10 -70 -114 v-406zM274 197q17 -31 54 -31q70 10 71 113v403z" /> | |||
|
182 | <glyph unicode="ù" horiz-adv-x="690" d="M78 203v917h207v-887q0 -49 49 -49q41 0 67 54v882h207v-1120h-207v94l-31 -32q-78 -78 -145.5 -78t-107 68.5t-39.5 150.5zM113 1489h215l106 -184h-160z" /> | |||
|
183 | <glyph unicode="ú" horiz-adv-x="690" d="M78 203v917h207v-887q0 -49 49 -49q41 0 67 54v882h207v-1120h-207v94l-31 -32q-78 -78 -145.5 -78t-107 68.5t-39.5 150.5zM274 1305l107 184h215l-162 -184h-160z" /> | |||
|
184 | <glyph unicode="û" horiz-adv-x="690" d="M78 203v917h207v-887q0 -49 49 -49q41 0 67 54v882h207v-1120h-207v94l-31 -32q-78 -78 -145.5 -78t-107 68.5t-39.5 150.5zM94 1305l142 184h219l141 -184h-188l-64 71l-61 -71h-189z" /> | |||
|
185 | <glyph unicode="ü" horiz-adv-x="690" d="M78 203v917h207v-887q0 -49 49 -49q41 0 67 54v882h207v-1120h-207v94l-31 -32q-78 -78 -145.5 -78t-107 68.5t-39.5 150.5zM106 1305v200h191v-200h-191zM395 1305v200h191v-200h-191z" /> | |||
|
186 | <glyph unicode="ý" horiz-adv-x="634" d="M25 1120l190 -1153q0 -68 -36 -123t-97 -61l-49 4v-184q70 -4 92 -4q115 0 192.5 95t94.5 222l198 1204h-202l-82 -688l-4 -57h-9l-4 57l-82 688h-202zM231 1305l107 184h215l-162 -184h-160z" /> | |||
|
187 | <glyph unicode="þ" horiz-adv-x="686" d="M82 -385v1890h207v-458q88 90 165 90t117.5 -69t40.5 -150v-715q0 -82 -41 -150.5t-118 -68.5q-33 0 -74 22.5t-66 44.5l-24 23v-459h-207zM289 246q0 -25 19.5 -46.5t42 -21.5t39 19.5t16.5 48.5v628q0 29 -16.5 48.5t-39 19.5t-42 -19.5t-19.5 -48.5v-628z" /> | |||
|
188 | <glyph unicode="ÿ" horiz-adv-x="634" d="M25 1120h202l82 -688l4 -57h9l4 57l82 688h202l-198 -1204q-16 -127 -94 -222t-193 -95l-92 4v184q16 -4 49 -4q61 6 97 61.5t36 122.5zM78 1305v200h190v-200h-190zM367 1305v200h190v-200h-190z" /> | |||
|
189 | <glyph unicode="Œ" horiz-adv-x="983" d="M68 309v887q0 41 17 101.5t45 100.5t88.5 73.5t143.5 33.5h580v-227h-285v-395h205v-242h-205v-414h285v-227h-580q-84 0 -144 31.5t-88 78.5q-55 91 -60 169zM289 309q0 -46 19.5 -78t54 -32t53 27.5t18.5 56.5l2 26v901q-6 96 -74 97q-35 0 -53 -28t-18 -54l-2 -29 v-887z" /> | |||
|
190 | <glyph unicode="œ" horiz-adv-x="995" d="M63 279v563q0 40 15.5 96.5t40 95.5t80 71t145.5 32t156 -60q66 59 170 60q199 0 252 -197q14 -51 14 -92v-326h-342v-250q0 -46 22.5 -76t53.5 -30q70 10 73 113v122h193v-129q0 -37 -16.5 -93t-41 -95t-80 -69.5t-146 -30.5t-154.5 57q-68 -57 -156 -57t-143.5 30.5 t-80.5 73.5q-52 92 -52 160zM258 267.5q-2 -11.5 2 -29t10 -34.5q14 -38 58 -38q70 10 71 113v563q-2 0 0 11t-2 28.5t-10 34.5q-15 40 -59 40q-68 -10 -70 -114v-563q2 0 0 -11.5zM594 684h149v158q0 48 -19 81t-58 33t-55.5 -37.5t-16.5 -70.5v-164z" /> | |||
|
191 | <glyph unicode="Ÿ" horiz-adv-x="704" d="M16 1505h217l111 -481l6 -14h4l6 14l111 481h217l-225 -864v-641h-221v641zM113 1638v201h190v-201h-190zM401 1638v201h191v-201h-191z" /> | |||
|
192 | <glyph unicode="ˆ" horiz-adv-x="1021" d="M260 1305l141 184h220l141 -184h-189l-63 71l-61 -71h-189z" /> | |||
|
193 | <glyph unicode="˜" horiz-adv-x="1024" d="M313 1305v151q49 39 95.5 39t104.5 -18.5t97 -19.5t101 32v-152q-51 -39 -95.5 -39t-102.5 19.5t-99 19.5t-101 -32z" /> | |||
|
194 | <glyph unicode=" " horiz-adv-x="952" /> | |||
|
195 | <glyph unicode=" " horiz-adv-x="1905" /> | |||
|
196 | <glyph unicode=" " horiz-adv-x="952" /> | |||
|
197 | <glyph unicode=" " horiz-adv-x="1905" /> | |||
|
198 | <glyph unicode=" " horiz-adv-x="635" /> | |||
|
199 | <glyph unicode=" " horiz-adv-x="476" /> | |||
|
200 | <glyph unicode=" " horiz-adv-x="317" /> | |||
|
201 | <glyph unicode=" " horiz-adv-x="317" /> | |||
|
202 | <glyph unicode=" " horiz-adv-x="238" /> | |||
|
203 | <glyph unicode=" " horiz-adv-x="381" /> | |||
|
204 | <glyph unicode=" " horiz-adv-x="105" /> | |||
|
205 | <glyph unicode="‐" horiz-adv-x="444" d="M74 455v194h297v-194h-297z" /> | |||
|
206 | <glyph unicode="‑" horiz-adv-x="444" d="M74 455v194h297v-194h-297z" /> | |||
|
207 | <glyph unicode="‒" horiz-adv-x="444" d="M74 455v194h297v-194h-297z" /> | |||
|
208 | <glyph unicode="–" horiz-adv-x="806" d="M74 649v195h659v-195h-659z" /> | |||
|
209 | <glyph unicode="—" horiz-adv-x="972" d="M74 649v195h825v-195h-825z" /> | |||
|
210 | <glyph unicode="‘" horiz-adv-x="309" d="M49 1012v227l113 266h102l-71 -266h71v-227h-215z" /> | |||
|
211 | <glyph unicode="’" horiz-adv-x="309" d="M45 1012l72 266h-72v227h215v-227l-113 -266h-102z" /> | |||
|
212 | <glyph unicode="‚" horiz-adv-x="309" d="M45 0v227h215v-227l-113 -266h-102l72 266h-72z" /> | |||
|
213 | <glyph unicode="“" horiz-adv-x="624" d="M53 1012v227l113 266h102l-71 -266h71v-227h-215zM356 1012v227l113 266h102l-71 -266h71v-227h-215z" /> | |||
|
214 | <glyph unicode="”" horiz-adv-x="624" d="M53 1012l72 266h-72v227h215v-227l-112 -266h-103zM356 1012l72 266h-72v227h215v-227l-112 -266h-103z" /> | |||
|
215 | <glyph unicode="„" horiz-adv-x="624" d="M53 0v227h215v-227l-112 -266h-103l72 266h-72zM356 0v227h215v-227l-112 -266h-103l72 266h-72z" /> | |||
|
216 | <glyph unicode="•" horiz-adv-x="663" d="M82 815q0 104 72.5 177t177 73t177.5 -72.5t73 -177t-73 -177.5t-177 -73t-177 73t-73 177z" /> | |||
|
217 | <glyph unicode="…" horiz-adv-x="964" d="M53 0v227h215v-227h-215zM375 0v227h215v-227h-215zM696 0v227h215v-227h-215z" /> | |||
|
218 | <glyph unicode=" " horiz-adv-x="381" /> | |||
|
219 | <glyph unicode="‹" horiz-adv-x="1058" d="M74 649v160l911 475v-199l-698 -356l698 -356v-199z" /> | |||
|
220 | <glyph unicode="›" horiz-adv-x="1058" d="M74 174v199l698 356l-698 356v199l911 -475v-160z" /> | |||
|
221 | <glyph unicode=" " horiz-adv-x="476" /> | |||
|
222 | <glyph unicode="€" horiz-adv-x="813" d="M53 547v137h107v137h-107v137h107v238q0 42 17.5 106t45 107t88 78t144.5 35t144 -34t88 -81q53 -90 61 -178l2 -33v-84h-207v84q-2 0 0 11.5t-3 27.5t-12 33q-18 39 -69 39q-70 -10 -78 -111v-238h233v-137h-233v-137h233v-137h-233v-238q0 -43 21.5 -76.5t59.5 -33.5 t58.5 27.5t20.5 56.5l2 26v84h207v-84q0 -38 -17.5 -104t-45.5 -109t-88 -77.5t-144 -34.5t-144.5 33.5t-88.5 81.5q-55 97 -60 175l-2 35v238h-107z" /> | |||
|
223 | <glyph unicode="™" horiz-adv-x="937" d="M74 1401v104h321v-104h-104v-580h-113v580h-104zM440 821v684h138l67 -319h6l68 319h137v-684h-104v449l-78 -449h-51l-80 449v-449h-103z" /> | |||
|
224 | <glyph unicode="" horiz-adv-x="1120" d="M0 0v1120h1120v-1120h-1120z" /> | |||
|
225 | <glyph unicode="fi" horiz-adv-x="772" d="M20 934v186h105v31q0 172 31 231q16 31 42 67q53 71 181 71q59 0 127 -13l20 -2v-184q-41 12 -91 12t-69.5 -18.5t-25.5 -58.5q-8 -52 -8 -107v-29h358v-1120h-207v934h-151v-934h-207v934h-105z" /> | |||
|
226 | <glyph unicode="fl" horiz-adv-x="772" d="M20 934v186h105v31q0 172 31 231q16 31 42 67q53 71 181 71q59 0 127 -13l20 -2h164v-1505h-207v1329q-37 4 -67.5 4t-50 -18.5t-25.5 -58.5q-8 -52 -8 -107v-29h104v-186h-104v-934h-207v934h-105z" /> | |||
|
227 | <glyph unicode="ffi" horiz-adv-x="1320" d="M20 934v186h105v31q0 190 51 270q23 35 71 63.5t115 28.5l97 -14v-178q-27 8 -62 8q-66 0 -65 -180v-29h104v-186h-104v-934h-207v934h-105zM495 934v186h105v31q0 190 51 270q23 35 71 63.5t115 28.5l97 -14v-178q-27 8 -62 8q-66 0 -65 -180v-29h104v-186h-104v-934 h-207v934h-105zM1032 0v1120h207v-1120h-207zM1032 1298v207h207v-207h-207z" /> | |||
|
228 | <glyph unicode="ffl" horiz-adv-x="1320" d="M20 934v186h105v31q0 190 51 270q23 35 71 63.5t115 28.5l97 -14v-178q-27 8 -62 8q-66 0 -65 -180v-29h104v-186h-104v-934h-207v934h-105zM495 934v186h105v31q0 190 51 270q23 35 71 63.5t115 28.5l97 -14v-178q-27 8 -62 8q-66 0 -65 -180v-29h104v-186h-104v-934 h-207v934h-105zM1032 0v1505h207v-1505h-207z" /> | |||
|
229 | </font> | |||
|
230 | </defs></svg> No newline at end of file |
1 | NO CONTENT: new file 100644, binary diff hidden |
|
NO CONTENT: new file 100644, binary diff hidden |
1 | NO CONTENT: new file 100644, binary diff hidden |
|
NO CONTENT: new file 100644, binary diff hidden |
@@ -0,0 +1,2 b'' | |||||
|
1 | SIL Open Font License (OFL) | |||
|
2 | http://scripts.sil.org/cms/scripts/page.php?site_id=nrsi&id=OFL |
@@ -0,0 +1,2 b'' | |||||
|
1 | /*! @source http://purl.eligrey.com/github/classList.js/blob/master/classList.js*/ | |||
|
2 | if(typeof document!=="undefined"&&!("classList" in document.createElement("a"))){(function(j){var a="classList",f="prototype",m=(j.HTMLElement||j.Element)[f],b=Object,k=String[f].trim||function(){return this.replace(/^\s+|\s+$/g,"")},c=Array[f].indexOf||function(q){var p=0,o=this.length;for(;p<o;p++){if(p in this&&this[p]===q){return p}}return -1},n=function(o,p){this.name=o;this.code=DOMException[o];this.message=p},g=function(p,o){if(o===""){throw new n("SYNTAX_ERR","An invalid or illegal string was specified")}if(/\s/.test(o)){throw new n("INVALID_CHARACTER_ERR","String contains an invalid character")}return c.call(p,o)},d=function(s){var r=k.call(s.className),q=r?r.split(/\s+/):[],p=0,o=q.length;for(;p<o;p++){this.push(q[p])}this._updateClassName=function(){s.className=this.toString()}},e=d[f]=[],i=function(){return new d(this)};n[f]=Error[f];e.item=function(o){return this[o]||null};e.contains=function(o){o+="";return g(this,o)!==-1};e.add=function(o){o+="";if(g(this,o)===-1){this.push(o);this._updateClassName()}};e.remove=function(p){p+="";var o=g(this,p);if(o!==-1){this.splice(o,1);this._updateClassName()}};e.toggle=function(o){o+="";if(g(this,o)===-1){this.add(o)}else{this.remove(o)}};e.toString=function(){return this.join(" ")};if(b.defineProperty){var l={get:i,enumerable:true,configurable:true};try{b.defineProperty(m,a,l)}catch(h){if(h.number===-2146823252){l.enumerable=false;b.defineProperty(m,a,l)}}}else{if(b[f].__defineGetter__){m.__defineGetter__(a,i)}}}(self))}; No newline at end of file |
@@ -0,0 +1,8 b'' | |||||
|
1 | /** | |||
|
2 | Head JS The only script in your <HEAD> | |||
|
3 | Copyright Tero Piirainen (tipiirai) | |||
|
4 | License MIT / http://bit.ly/mit-license | |||
|
5 | Version 0.96 | |||
|
6 | ||||
|
7 | http://headjs.com | |||
|
8 | */(function(a){function z(){d||(d=!0,s(e,function(a){p(a)}))}function y(c,d){var e=a.createElement("script");e.type="text/"+(c.type||"javascript"),e.src=c.src||c,e.async=!1,e.onreadystatechange=e.onload=function(){var a=e.readyState;!d.done&&(!a||/loaded|complete/.test(a))&&(d.done=!0,d())},(a.body||b).appendChild(e)}function x(a,b){if(a.state==o)return b&&b();if(a.state==n)return k.ready(a.name,b);if(a.state==m)return a.onpreload.push(function(){x(a,b)});a.state=n,y(a.url,function(){a.state=o,b&&b(),s(g[a.name],function(a){p(a)}),u()&&d&&s(g.ALL,function(a){p(a)})})}function w(a,b){a.state===undefined&&(a.state=m,a.onpreload=[],y({src:a.url,type:"cache"},function(){v(a)}))}function v(a){a.state=l,s(a.onpreload,function(a){a.call()})}function u(a){a=a||h;var b;for(var c in a){if(a.hasOwnProperty(c)&&a[c].state!=o)return!1;b=!0}return b}function t(a){return Object.prototype.toString.call(a)=="[object Function]"}function s(a,b){if(!!a){typeof a=="object"&&(a=[].slice.call(a));for(var c=0;c<a.length;c++)b.call(a,a[c],c)}}function r(a){var b;if(typeof a=="object")for(var c in a)a[c]&&(b={name:c,url:a[c]});else b={name:q(a),url:a};var d=h[b.name];if(d&&d.url===b.url)return d;h[b.name]=b;return b}function q(a){var b=a.split("/"),c=b[b.length-1],d=c.indexOf("?");return d!=-1?c.substring(0,d):c}function p(a){a._done||(a(),a._done=1)}var b=a.documentElement,c,d,e=[],f=[],g={},h={},i=a.createElement("script").async===!0||"MozAppearance"in a.documentElement.style||window.opera,j=window.head_conf&&head_conf.head||"head",k=window[j]=window[j]||function(){k.ready.apply(null,arguments)},l=1,m=2,n=3,o=4;i?k.js=function(){var a=arguments,b=a[a.length-1],c={};t(b)||(b=null),s(a,function(d,e){d!=b&&(d=r(d),c[d.name]=d,x(d,b&&e==a.length-2?function(){u(c)&&p(b)}:null))});return k}:k.js=function(){var a=arguments,b=[].slice.call(a,1),d=b[0];if(!c){f.push(function(){k.js.apply(null,a)});return k}d?(s(b,function(a){t(a)||w(r(a))}),x(r(a[0]),t(d)?d:function(){k.js.apply(null,b)})):x(r(a[0]));return k},k.ready=function(b,c){if(b==a){d?p(c):e.push(c);return k}t(b)&&(c=b,b="ALL");if(typeof b!="string"||!t(c))return k;var f=h[b];if(f&&f.state==o||b=="ALL"&&u()&&d){p(c);return k}var i=g[b];i?i.push(c):i=g[b]=[c];return k},k.ready(a,function(){u()&&s(g.ALL,function(a){p(a)}),k.feature&&k.feature("domloaded",!0)});if(window.addEventListener)a.addEventListener("DOMContentLoaded",z,!1),window.addEventListener("load",z,!1);else if(window.attachEvent){a.attachEvent("onreadystatechange",function(){a.readyState==="complete"&&z()});var A=1;try{A=window.frameElement}catch(B){}!A&&b.doScroll&&function(){try{b.doScroll("left"),z()}catch(a){setTimeout(arguments.callee,1);return}}(),window.attachEvent("onload",z)}!a.readyState&&a.addEventListener&&(a.readyState="loading",a.addEventListener("DOMContentLoaded",handler=function(){a.removeEventListener("DOMContentLoaded",handler,!1),a.readyState="complete"},!1)),setTimeout(function(){c=!0,s(f,function(a){a()})},300)})(document) No newline at end of file |
@@ -0,0 +1,7 b'' | |||||
|
1 | document.createElement('header'); | |||
|
2 | document.createElement('nav'); | |||
|
3 | document.createElement('section'); | |||
|
4 | document.createElement('article'); | |||
|
5 | document.createElement('aside'); | |||
|
6 | document.createElement('footer'); | |||
|
7 | document.createElement('hgroup'); No newline at end of file |
@@ -0,0 +1,14 b'' | |||||
|
1 | // START CUSTOM REVEAL.JS INTEGRATION | |||
|
2 | [].slice.call( document.querySelectorAll( 'pre code' ) ).forEach( function( element ) { | |||
|
3 | element.addEventListener( 'focusout', function( event ) { | |||
|
4 | hljs.highlightBlock( event.currentTarget ); | |||
|
5 | }, false ); | |||
|
6 | } ); | |||
|
7 | // END CUSTOM REVEAL.JS INTEGRATION | |||
|
8 | ||||
|
9 | ||||
|
10 | /* | |||
|
11 | Syntax highlighting with language autodetection. | |||
|
12 | http://softwaremaniacs.org/soft/highlight/ | |||
|
13 | */ | |||
|
14 | var hljs=new function(){function m(p){return p.replace(/</gm,"<")}function c(r,q,p){return RegExp(q,"m"+(r.cI?"i":"")+(p?"g":""))}function j(r){for(var p=0;p<r.childNodes.length;p++){var q=r.childNodes[p];if(q.nodeName=="CODE"){return q}if(!(q.nodeType==3&&q.nodeValue.match(/\s+/))){break}}}function g(t,s){var r="";for(var q=0;q<t.childNodes.length;q++){if(t.childNodes[q].nodeType==3){var p=t.childNodes[q].nodeValue;if(s){p=p.replace(/\n/g,"")}r+=p}else{if(t.childNodes[q].nodeName=="BR"){r+="\n"}else{r+=g(t.childNodes[q])}}}if(/MSIE [678]/.test(navigator.userAgent)){r=r.replace(/\r/g,"\n")}return r}function a(s){var q=s.className.split(/\s+/);q=q.concat(s.parentNode.className.split(/\s+/));for(var p=0;p<q.length;p++){var r=q[p].replace(/^language-/,"");if(d[r]||r=="no-highlight"){return r}}}function b(p){var q=[];(function(s,t){for(var r=0;r<s.childNodes.length;r++){if(s.childNodes[r].nodeType==3){t+=s.childNodes[r].nodeValue.length}else{if(s.childNodes[r].nodeName=="BR"){t+=1}else{q.push({event:"start",offset:t,node:s.childNodes[r]});t=arguments.callee(s.childNodes[r],t);q.push({event:"stop",offset:t,node:s.childNodes[r]})}}}return t})(p,0);return q}function l(y,z,x){var r=0;var w="";var t=[];function u(){if(y.length&&z.length){if(y[0].offset!=z[0].offset){return(y[0].offset<z[0].offset)?y:z}else{return z[0].event=="start"?y:z}}else{return y.length?y:z}}function s(C){var D="<"+C.nodeName.toLowerCase();for(var A=0;A<C.attributes.length;A++){var B=C.attributes[A];D+=" "+B.nodeName.toLowerCase();if(B.nodeValue!=undefined&&B.nodeValue!=false&&B.nodeValue!=null){D+='="'+m(B.nodeValue)+'"'}}return D+">"}while(y.length||z.length){var v=u().splice(0,1)[0];w+=m(x.substr(r,v.offset-r));r=v.offset;if(v.event=="start"){w+=s(v.node);t.push(v.node)}else{if(v.event=="stop"){var q=t.length;do{q--;var p=t[q];w+=("</"+p.nodeName.toLowerCase()+">")}while(p!=v.node);t.splice(q,1);while(q<t.length){w+=s(t[q]);q++}}}}w+=x.substr(r);return w}function i(){function p(u,t,v){if(u.compiled){return}if(!v){u.bR=c(t,u.b?u.b:"\\B|\\b");if(!u.e&&!u.eW){u.e="\\B|\\b"}if(u.e){u.eR=c(t,u.e)}}if(u.i){u.iR=c(t,u.i)}if(u.r==undefined){u.r=1}if(u.k){u.lR=c(t,u.l||hljs.IR,true)}for(var s in u.k){if(!u.k.hasOwnProperty(s)){continue}if(u.k[s] instanceof Object){u.kG=u.k}else{u.kG={keyword:u.k}}break}if(!u.c){u.c=[]}u.compiled=true;for(var r=0;r<u.c.length;r++){p(u.c[r],t,false)}if(u.starts){p(u.starts,t,false)}}for(var q in d){if(!d.hasOwnProperty(q)){continue}p(d[q].dM,d[q],true)}}function e(J,D){if(!i.called){i();i.called=true}function z(r,M){for(var L=0;L<M.c.length;L++){if(M.c[L].bR.test(r)){return M.c[L]}}}function w(L,r){if(C[L].e&&C[L].eR.test(r)){return 1}if(C[L].eW){var M=w(L-1,r);return M?M+1:0}return 0}function x(r,L){return L.iR&&L.iR.test(r)}function A(O,N){var M=[];for(var L=0;L<O.c.length;L++){M.push(O.c[L].b)}var r=C.length-1;do{if(C[r].e){M.push(C[r].e)}r--}while(C[r+1].eW);if(O.i){M.push(O.i)}return c(N,"("+M.join("|")+")",true)}function s(M,L){var N=C[C.length-1];if(!N.t){N.t=A(N,H)}N.t.lastIndex=L;var r=N.t.exec(M);if(r){return[M.substr(L,r.index-L),r[0],false]}else{return[M.substr(L),"",true]}}function p(O,r){var L=H.cI?r[0].toLowerCase():r[0];for(var N in O.kG){if(!O.kG.hasOwnProperty(N)){continue}var M=O.kG[N].hasOwnProperty(L);if(M){return[N,M]}}return false}function F(M,O){if(!O.k){return m(M)}var N="";var P=0;O.lR.lastIndex=0;var L=O.lR.exec(M);while(L){N+=m(M.substr(P,L.index-P));var r=p(O,L);if(r){t+=r[1];N+='<span class="'+r[0]+'">'+m(L[0])+"</span>"}else{N+=m(L[0])}P=O.lR.lastIndex;L=O.lR.exec(M)}N+=m(M.substr(P,M.length-P));return N}function K(r,M){if(M.sL&&d[M.sL]){var L=e(M.sL,r);t+=L.keyword_count;return L.value}else{return F(r,M)}}function I(M,r){var L=M.cN?'<span class="'+M.cN+'">':"";if(M.rB){q+=L;M.buffer=""}else{if(M.eB){q+=m(r)+L;M.buffer=""}else{q+=L;M.buffer=r}}C.push(M);B+=M.r}function E(O,L,Q){var R=C[C.length-1];if(Q){q+=K(R.buffer+O,R);return false}var M=z(L,R);if(M){q+=K(R.buffer+O,R);I(M,L);return M.rB}var r=w(C.length-1,L);if(r){var N=R.cN?"</span>":"";if(R.rE){q+=K(R.buffer+O,R)+N}else{if(R.eE){q+=K(R.buffer+O,R)+N+m(L)}else{q+=K(R.buffer+O+L,R)+N}}while(r>1){N=C[C.length-2].cN?"</span>":"";q+=N;r--;C.length--}var P=C[C.length-1];C.length--;C[C.length-1].buffer="";if(P.starts){I(P.starts,"")}return R.rE}if(x(L,R)){throw"Illegal"}}var H=d[J];var C=[H.dM];var B=0;var t=0;var q="";try{var v=0;H.dM.buffer="";do{var y=s(D,v);var u=E(y[0],y[1],y[2]);v+=y[0].length;if(!u){v+=y[1].length}}while(!y[2]);if(C.length>1){throw"Illegal"}return{r:B,keyword_count:t,value:q}}catch(G){if(G=="Illegal"){return{r:0,keyword_count:0,value:m(D)}}else{throw G}}}function f(t){var r={keyword_count:0,r:0,value:m(t)};var q=r;for(var p in d){if(!d.hasOwnProperty(p)){continue}var s=e(p,t);s.language=p;if(s.keyword_count+s.r>q.keyword_count+q.r){q=s}if(s.keyword_count+s.r>r.keyword_count+r.r){q=r;r=s}}if(q.language){r.second_best=q}return r}function h(r,q,p){if(q){r=r.replace(/^((<[^>]+>|\t)+)/gm,function(t,w,v,u){return w.replace(/\t/g,q)})}if(p){r=r.replace(/\n/g,"<br>")}return r}function o(u,x,q){var y=g(u,q);var s=a(u);if(s=="no-highlight"){return}if(s){var w=e(s,y)}else{var w=f(y);s=w.language}var p=b(u);if(p.length){var r=document.createElement("pre");r.innerHTML=w.value;w.value=l(p,b(r),y)}w.value=h(w.value,x,q);var t=u.className;if(!t.match("(\\s|^)(language-)?"+s+"(\\s|$)")){t=t?(t+" "+s):s}if(/MSIE [678]/.test(navigator.userAgent)&&u.tagName=="CODE"&&u.parentNode.tagName=="PRE"){var r=u.parentNode;var v=document.createElement("div");v.innerHTML="<pre><code>"+w.value+"</code></pre>";u=v.firstChild.firstChild;v.firstChild.cN=r.cN;r.parentNode.replaceChild(v.firstChild,r)}else{u.innerHTML=w.value}u.className=t;u.result={language:s,kw:w.keyword_count,re:w.r};if(w.second_best){u.second_best={language:w.second_best.language,kw:w.second_best.keyword_count,re:w.second_best.r}}}function k(){if(k.called){return}k.called=true;var r=document.getElementsByTagName("pre");for(var p=0;p<r.length;p++){var q=j(r[p]);if(q){o(q,hljs.tabReplace)}}}function n(){if(window.addEventListener){window.addEventListener("DOMContentLoaded",k,false);window.addEventListener("load",k,false)}else{if(window.attachEvent){window.attachEvent("onload",k)}else{window.onload=k}}}var d={};this.LANGUAGES=d;this.highlight=e;this.highlightAuto=f;this.fixMarkup=h;this.highlightBlock=o;this.initHighlighting=k;this.initHighlightingOnLoad=n;this.IR="[a-zA-Z][a-zA-Z0-9_]*";this.UIR="[a-zA-Z_][a-zA-Z0-9_]*";this.NR="\\b\\d+(\\.\\d+)?";this.CNR="\\b(0x[A-Za-z0-9]+|\\d+(\\.\\d+)?)";this.RSR="!|!=|!==|%|%=|&|&&|&=|\\*|\\*=|\\+|\\+=|,|\\.|-|-=|/|/=|:|;|<|<<|<<=|<=|=|==|===|>|>=|>>|>>=|>>>|>>>=|\\?|\\[|\\{|\\(|\\^|\\^=|\\||\\|=|\\|\\||~";this.BE={b:"\\\\.",r:0};this.ASM={cN:"string",b:"'",e:"'",i:"\\n",c:[this.BE],r:0};this.QSM={cN:"string",b:'"',e:'"',i:"\\n",c:[this.BE],r:0};this.CLCM={cN:"comment",b:"//",e:"$"};this.CBLCLM={cN:"comment",b:"/\\*",e:"\\*/"};this.HCM={cN:"comment",b:"#",e:"$"};this.NM={cN:"number",b:this.NR,r:0};this.CNM={cN:"number",b:this.CNR,r:0};this.inherit=function(p,s){var r={};for(var q in p){r[q]=p[q]}if(s){for(var q in s){r[q]=s[q]}}return r}}();hljs.LANGUAGES.cs={dM:{k:{"abstract":1,as:1,base:1,bool:1,"break":1,"byte":1,"case":1,"catch":1,"char":1,checked:1,"class":1,"const":1,"continue":1,decimal:1,"default":1,delegate:1,"do":1,"do":1,"double":1,"else":1,"enum":1,event:1,explicit:1,extern:1,"false":1,"finally":1,fixed:1,"float":1,"for":1,foreach:1,"goto":1,"if":1,implicit:1,"in":1,"int":1,"interface":1,internal:1,is:1,lock:1,"long":1,namespace:1,"new":1,"null":1,object:1,operator:1,out:1,override:1,params:1,"private":1,"protected":1,"public":1,readonly:1,ref:1,"return":1,sbyte:1,sealed:1,"short":1,sizeof:1,stackalloc:1,"static":1,string:1,struct:1,"switch":1,"this":1,"throw":1,"true":1,"try":1,"typeof":1,uint:1,ulong:1,unchecked:1,unsafe:1,ushort:1,using:1,virtual:1,"volatile":1,"void":1,"while":1,ascending:1,descending:1,from:1,get:1,group:1,into:1,join:1,let:1,orderby:1,partial:1,select:1,set:1,value:1,"var":1,where:1,yield:1},c:[{cN:"comment",b:"///",e:"$",rB:true,c:[{cN:"xmlDocTag",b:"///|<!--|-->"},{cN:"xmlDocTag",b:"</?",e:">"}]},hljs.CLCM,hljs.CBLCLM,{cN:"string",b:'@"',e:'"',c:[{b:'""'}]},hljs.ASM,hljs.QSM,hljs.CNM]}};hljs.LANGUAGES.ruby=function(){var g="[a-zA-Z_][a-zA-Z0-9_]*(\\!|\\?)?";var a="[a-zA-Z_]\\w*[!?=]?|[-+~]\\@|<<|>>|=~|===?|<=>|[<>]=?|\\*\\*|[-/+%^&*~`|]|\\[\\]=?";var n={keyword:{and:1,"false":1,then:1,defined:1,module:1,"in":1,"return":1,redo:1,"if":1,BEGIN:1,retry:1,end:1,"for":1,"true":1,self:1,when:1,next:1,until:1,"do":1,begin:1,unless:1,END:1,rescue:1,nil:1,"else":1,"break":1,undef:1,not:1,"super":1,"class":1,"case":1,require:1,yield:1,alias:1,"while":1,ensure:1,elsif:1,or:1,def:1},keymethods:{__id__:1,__send__:1,abort:1,abs:1,"all?":1,allocate:1,ancestors:1,"any?":1,arity:1,assoc:1,at:1,at_exit:1,autoload:1,"autoload?":1,"between?":1,binding:1,binmode:1,"block_given?":1,call:1,callcc:1,caller:1,capitalize:1,"capitalize!":1,casecmp:1,"catch":1,ceil:1,center:1,chomp:1,"chomp!":1,chop:1,"chop!":1,chr:1,"class":1,class_eval:1,"class_variable_defined?":1,class_variables:1,clear:1,clone:1,close:1,close_read:1,close_write:1,"closed?":1,coerce:1,collect:1,"collect!":1,compact:1,"compact!":1,concat:1,"const_defined?":1,const_get:1,const_missing:1,const_set:1,constants:1,count:1,crypt:1,"default":1,default_proc:1,"delete":1,"delete!":1,delete_at:1,delete_if:1,detect:1,display:1,div:1,divmod:1,downcase:1,"downcase!":1,downto:1,dump:1,dup:1,each:1,each_byte:1,each_index:1,each_key:1,each_line:1,each_pair:1,each_value:1,each_with_index:1,"empty?":1,entries:1,eof:1,"eof?":1,"eql?":1,"equal?":1,"eval":1,exec:1,exit:1,"exit!":1,extend:1,fail:1,fcntl:1,fetch:1,fileno:1,fill:1,find:1,find_all:1,first:1,flatten:1,"flatten!":1,floor:1,flush:1,for_fd:1,foreach:1,fork:1,format:1,freeze:1,"frozen?":1,fsync:1,getc:1,gets:1,global_variables:1,grep:1,gsub:1,"gsub!":1,"has_key?":1,"has_value?":1,hash:1,hex:1,id:1,include:1,"include?":1,included_modules:1,index:1,indexes:1,indices:1,induced_from:1,inject:1,insert:1,inspect:1,instance_eval:1,instance_method:1,instance_methods:1,"instance_of?":1,"instance_variable_defined?":1,instance_variable_get:1,instance_variable_set:1,instance_variables:1,"integer?":1,intern:1,invert:1,ioctl:1,"is_a?":1,isatty:1,"iterator?":1,join:1,"key?":1,keys:1,"kind_of?":1,lambda:1,last:1,length:1,lineno:1,ljust:1,load:1,local_variables:1,loop:1,lstrip:1,"lstrip!":1,map:1,"map!":1,match:1,max:1,"member?":1,merge:1,"merge!":1,method:1,"method_defined?":1,method_missing:1,methods:1,min:1,module_eval:1,modulo:1,name:1,nesting:1,"new":1,next:1,"next!":1,"nil?":1,nitems:1,"nonzero?":1,object_id:1,oct:1,open:1,pack:1,partition:1,pid:1,pipe:1,pop:1,popen:1,pos:1,prec:1,prec_f:1,prec_i:1,print:1,printf:1,private_class_method:1,private_instance_methods:1,"private_method_defined?":1,private_methods:1,proc:1,protected_instance_methods:1,"protected_method_defined?":1,protected_methods:1,public_class_method:1,public_instance_methods:1,"public_method_defined?":1,public_methods:1,push:1,putc:1,puts:1,quo:1,raise:1,rand:1,rassoc:1,read:1,read_nonblock:1,readchar:1,readline:1,readlines:1,readpartial:1,rehash:1,reject:1,"reject!":1,remainder:1,reopen:1,replace:1,require:1,"respond_to?":1,reverse:1,"reverse!":1,reverse_each:1,rewind:1,rindex:1,rjust:1,round:1,rstrip:1,"rstrip!":1,scan:1,seek:1,select:1,send:1,set_trace_func:1,shift:1,singleton_method_added:1,singleton_methods:1,size:1,sleep:1,slice:1,"slice!":1,sort:1,"sort!":1,sort_by:1,split:1,sprintf:1,squeeze:1,"squeeze!":1,srand:1,stat:1,step:1,store:1,strip:1,"strip!":1,sub:1,"sub!":1,succ:1,"succ!":1,sum:1,superclass:1,swapcase:1,"swapcase!":1,sync:1,syscall:1,sysopen:1,sysread:1,sysseek:1,system:1,syswrite:1,taint:1,"tainted?":1,tell:1,test:1,"throw":1,times:1,to_a:1,to_ary:1,to_f:1,to_hash:1,to_i:1,to_int:1,to_io:1,to_proc:1,to_s:1,to_str:1,to_sym:1,tr:1,"tr!":1,tr_s:1,"tr_s!":1,trace_var:1,transpose:1,trap:1,truncate:1,"tty?":1,type:1,ungetc:1,uniq:1,"uniq!":1,unpack:1,unshift:1,untaint:1,untrace_var:1,upcase:1,"upcase!":1,update:1,upto:1,"value?":1,values:1,values_at:1,warn:1,write:1,write_nonblock:1,"zero?":1,zip:1}};var h={cN:"yardoctag",b:"@[A-Za-z]+"};var d={cN:"comment",b:"#",e:"$",c:[h]};var c={cN:"comment",b:"^\\=begin",e:"^\\=end",c:[h],r:10};var b={cN:"comment",b:"^__END__",e:"\\n$"};var u={cN:"subst",b:"#\\{",e:"}",l:g,k:n};var p=[hljs.BE,u];var s={cN:"string",b:"'",e:"'",c:p,r:0};var r={cN:"string",b:'"',e:'"',c:p,r:0};var q={cN:"string",b:"%[qw]?\\(",e:"\\)",c:p,r:10};var o={cN:"string",b:"%[qw]?\\[",e:"\\]",c:p,r:10};var m={cN:"string",b:"%[qw]?{",e:"}",c:p,r:10};var l={cN:"string",b:"%[qw]?<",e:">",c:p,r:10};var k={cN:"string",b:"%[qw]?/",e:"/",c:p,r:10};var j={cN:"string",b:"%[qw]?%",e:"%",c:p,r:10};var i={cN:"string",b:"%[qw]?-",e:"-",c:p,r:10};var t={cN:"string",b:"%[qw]?\\|",e:"\\|",c:p,r:10};var e={cN:"function",b:"\\bdef\\s+",e:" |$|;",l:g,k:n,c:[{cN:"title",b:a,l:g,k:n},{cN:"params",b:"\\(",e:"\\)",l:g,k:n},d,c,b]};var f={cN:"identifier",b:g,l:g,k:n,r:0};var v=[d,c,b,s,r,q,o,m,l,k,j,i,t,{cN:"class",b:"\\b(class|module)\\b",e:"$|;",k:{"class":1,module:1},c:[{cN:"title",b:"[A-Za-z_]\\w*(::\\w+)*(\\?|\\!)?",r:0},{cN:"inheritance",b:"<\\s*",c:[{cN:"parent",b:"("+hljs.IR+"::)?"+hljs.IR}]},d,c,b]},e,{cN:"constant",b:"(::)?([A-Z]\\w*(::)?)+",r:0},{cN:"symbol",b:":",c:[s,r,q,o,m,l,k,j,i,t,f],r:0},{cN:"number",b:"(\\b0[0-7_]+)|(\\b0x[0-9a-fA-F_]+)|(\\b[1-9][0-9_]*(\\.[0-9_]+)?)|[0_]\\b",r:0},{cN:"number",b:"\\?\\w"},{cN:"variable",b:"(\\$\\W)|((\\$|\\@\\@?)(\\w+))"},f,{b:"("+hljs.RSR+")\\s*",c:[d,c,b,{cN:"regexp",b:"/",e:"/[a-z]*",i:"\\n",c:[hljs.BE]}],r:0}];u.c=v;e.c[1].c=v;return{dM:{l:g,k:n,c:v}}}();hljs.LANGUAGES.javascript={dM:{k:{keyword:{"in":1,"if":1,"for":1,"while":1,"finally":1,"var":1,"new":1,"function":1,"do":1,"return":1,"void":1,"else":1,"break":1,"catch":1,"instanceof":1,"with":1,"throw":1,"case":1,"default":1,"try":1,"this":1,"switch":1,"continue":1,"typeof":1,"delete":1},literal:{"true":1,"false":1,"null":1}},c:[hljs.ASM,hljs.QSM,hljs.CLCM,hljs.CBLCLM,hljs.CNM,{b:"("+hljs.RSR+"|case|return|throw)\\s*",k:{"return":1,"throw":1,"case":1},c:[hljs.CLCM,hljs.CBLCLM,{cN:"regexp",b:"/",e:"/[gim]*",c:[{b:"\\\\/"}]}],r:0},{cN:"function",b:"\\bfunction\\b",e:"{",k:{"function":1},c:[{cN:"title",b:"[A-Za-z$_][0-9A-Za-z$_]*"},{cN:"params",b:"\\(",e:"\\)",c:[hljs.ASM,hljs.QSM,hljs.CLCM,hljs.CBLCLM]}]}]}};hljs.LANGUAGES.css=function(){var a={cN:"function",b:hljs.IR+"\\(",e:"\\)",c:[{eW:true,eE:true,c:[hljs.NM,hljs.ASM,hljs.QSM]}]};return{cI:true,dM:{i:"[=/|']",c:[hljs.CBLCLM,{cN:"id",b:"\\#[A-Za-z0-9_-]+"},{cN:"class",b:"\\.[A-Za-z0-9_-]+",r:0},{cN:"attr_selector",b:"\\[",e:"\\]",i:"$"},{cN:"pseudo",b:":(:)?[a-zA-Z0-9\\_\\-\\+\\(\\)\\\"\\']+"},{cN:"at_rule",b:"@(font-face|page)",l:"[a-z-]+",k:{"font-face":1,page:1}},{cN:"at_rule",b:"@",e:"[{;]",eE:true,k:{"import":1,page:1,media:1,charset:1},c:[a,hljs.ASM,hljs.QSM,hljs.NM]},{cN:"tag",b:hljs.IR,r:0},{cN:"rules",b:"{",e:"}",i:"[^\\s]",r:0,c:[hljs.CBLCLM,{cN:"rule",b:"[^\\s]",rB:true,e:";",eW:true,c:[{cN:"attribute",b:"[A-Z\\_\\.\\-]+",e:":",eE:true,i:"[^\\s]",starts:{cN:"value",eW:true,eE:true,c:[a,hljs.NM,hljs.QSM,hljs.ASM,hljs.CBLCLM,{cN:"hexcolor",b:"\\#[0-9A-F]+"},{cN:"important",b:"!important"}]}}]}]}]}}}();hljs.LANGUAGES.xml=function(){var b="[A-Za-z0-9\\._:-]+";var a={eW:true,c:[{cN:"attribute",b:b,r:0},{b:'="',rB:true,e:'"',c:[{cN:"value",b:'"',eW:true}]},{b:"='",rB:true,e:"'",c:[{cN:"value",b:"'",eW:true}]},{b:"=",c:[{cN:"value",b:"[^\\s/>]+"}]}]};return{cI:true,dM:{c:[{cN:"pi",b:"<\\?",e:"\\?>",r:10},{cN:"doctype",b:"<!DOCTYPE",e:">",r:10,c:[{b:"\\[",e:"\\]"}]},{cN:"comment",b:"<!--",e:"-->",r:10},{cN:"cdata",b:"<\\!\\[CDATA\\[",e:"\\]\\]>",r:10},{cN:"tag",b:"<style",e:">",k:{title:{style:1}},c:[a],starts:{cN:"css",e:"</style>",rE:true,sL:"css"}},{cN:"tag",b:"<script",e:">",k:{title:{script:1}},c:[a],starts:{cN:"javascript",e:"<\/script>",rE:true,sL:"javascript"}},{cN:"vbscript",b:"<%",e:"%>",sL:"vbscript"},{cN:"tag",b:"</?",e:"/?>",c:[{cN:"title",b:"[^ />]+"},a]}]}}}();hljs.LANGUAGES.java={dM:{k:{"false":1,"synchronized":1,"int":1,"abstract":1,"float":1,"private":1,"char":1,"interface":1,"boolean":1,"static":1,"null":1,"if":1,"const":1,"for":1,"true":1,"while":1,"long":1,"throw":1,strictfp:1,"finally":1,"protected":1,"extends":1,"import":1,"native":1,"final":1,"implements":1,"return":1,"void":1,"enum":1,"else":1,"break":1,"transient":1,"new":1,"catch":1,"instanceof":1,"byte":1,"super":1,"class":1,"volatile":1,"case":1,assert:1,"short":1,"package":1,"default":1,"double":1,"public":1,"try":1,"this":1,"switch":1,"continue":1,"throws":1},c:[{cN:"javadoc",b:"/\\*\\*",e:"\\*/",c:[{cN:"javadoctag",b:"@[A-Za-z]+"}],r:10},hljs.CLCM,hljs.CBLCLM,hljs.ASM,hljs.QSM,{cN:"class",b:"(class |interface )",e:"{",k:{"class":1,"interface":1},i:":",c:[{b:"(implements|extends)",k:{"extends":1,"implements":1},r:10},{cN:"title",b:hljs.UIR}]},hljs.CNM,{cN:"annotation",b:"@[A-Za-z]+"}]}};hljs.LANGUAGES.php={cI:true,dM:{k:{and:1,include_once:1,list:1,"abstract":1,global:1,"private":1,echo:1,"interface":1,as:1,"static":1,endswitch:1,array:1,"null":1,"if":1,endwhile:1,or:1,"const":1,"for":1,endforeach:1,self:1,"var":1,"while":1,isset:1,"public":1,"protected":1,exit:1,foreach:1,"throw":1,elseif:1,"extends":1,include:1,__FILE__:1,empty:1,require_once:1,"function":1,"do":1,xor:1,"return":1,"implements":1,parent:1,clone:1,use:1,__CLASS__:1,__LINE__:1,"else":1,"break":1,print:1,"eval":1,"new":1,"catch":1,__METHOD__:1,"class":1,"case":1,exception:1,php_user_filter:1,"default":1,die:1,require:1,__FUNCTION__:1,enddeclare:1,"final":1,"try":1,"this":1,"switch":1,"continue":1,endfor:1,endif:1,declare:1,unset:1,"true":1,"false":1,namespace:1},c:[hljs.CLCM,hljs.HCM,{cN:"comment",b:"/\\*",e:"\\*/",c:[{cN:"phpdoc",b:"\\s@[A-Za-z]+",r:10}]},hljs.CNM,hljs.inherit(hljs.ASM,{i:null}),hljs.inherit(hljs.QSM,{i:null}),{cN:"variable",b:"\\$[a-zA-Z_\x7f-\xff][a-zA-Z0-9_\x7f-\xff]*"},{cN:"preprocessor",b:"<\\?php",r:10},{cN:"preprocessor",b:"\\?>"}]}};hljs.LANGUAGES.python=function(){var c={cN:"string",b:"(u|b)?r?'''",e:"'''",r:10};var b={cN:"string",b:'(u|b)?r?"""',e:'"""',r:10};var a={cN:"string",b:"(u|r|ur|b|br)'",e:"'",c:[hljs.BE],r:10};var f={cN:"string",b:'(u|r|ur|b|br)"',e:'"',c:[hljs.BE],r:10};var d={cN:"title",b:hljs.UIR};var e={cN:"params",b:"\\(",e:"\\)",c:[c,b,a,f,hljs.ASM,hljs.QSM]};return{dM:{k:{keyword:{and:1,elif:1,is:1,global:1,as:1,"in":1,"if":1,from:1,raise:1,"for":1,except:1,"finally":1,print:1,"import":1,pass:1,"return":1,exec:1,"else":1,"break":1,not:1,"with":1,"class":1,assert:1,yield:1,"try":1,"while":1,"continue":1,del:1,or:1,def:1,lambda:1,nonlocal:10},built_in:{None:1,True:1,False:1,Ellipsis:1,NotImplemented:1}},i:"(</|->|\\?)",c:[hljs.HCM,c,b,a,f,hljs.ASM,hljs.QSM,{cN:"function",b:"\\bdef ",e:":",i:"$",k:{def:1},c:[d,e],r:10},{cN:"class",b:"\\bclass ",e:":",i:"[${]",k:{"class":1},c:[d,e],r:10},hljs.CNM,{cN:"decorator",b:"@",e:"$"}]}}}();hljs.LANGUAGES.perl=function(){var c={getpwent:1,getservent:1,quotemeta:1,msgrcv:1,scalar:1,kill:1,dbmclose:1,undef:1,lc:1,ma:1,syswrite:1,tr:1,send:1,umask:1,sysopen:1,shmwrite:1,vec:1,qx:1,utime:1,local:1,oct:1,semctl:1,localtime:1,readpipe:1,"do":1,"return":1,format:1,read:1,sprintf:1,dbmopen:1,pop:1,getpgrp:1,not:1,getpwnam:1,rewinddir:1,qq:1,fileno:1,qw:1,endprotoent:1,wait:1,sethostent:1,bless:1,s:1,opendir:1,"continue":1,each:1,sleep:1,endgrent:1,shutdown:1,dump:1,chomp:1,connect:1,getsockname:1,die:1,socketpair:1,close:1,flock:1,exists:1,index:1,shmget:1,sub:1,"for":1,endpwent:1,redo:1,lstat:1,msgctl:1,setpgrp:1,abs:1,exit:1,select:1,print:1,ref:1,gethostbyaddr:1,unshift:1,fcntl:1,syscall:1,"goto":1,getnetbyaddr:1,join:1,gmtime:1,symlink:1,semget:1,splice:1,x:1,getpeername:1,recv:1,log:1,setsockopt:1,cos:1,last:1,reverse:1,gethostbyname:1,getgrnam:1,study:1,formline:1,endhostent:1,times:1,chop:1,length:1,gethostent:1,getnetent:1,pack:1,getprotoent:1,getservbyname:1,rand:1,mkdir:1,pos:1,chmod:1,y:1,substr:1,endnetent:1,printf:1,next:1,open:1,msgsnd:1,readdir:1,use:1,unlink:1,getsockopt:1,getpriority:1,rindex:1,wantarray:1,hex:1,system:1,getservbyport:1,endservent:1,"int":1,chr:1,untie:1,rmdir:1,prototype:1,tell:1,listen:1,fork:1,shmread:1,ucfirst:1,setprotoent:1,"else":1,sysseek:1,link:1,getgrgid:1,shmctl:1,waitpid:1,unpack:1,getnetbyname:1,reset:1,chdir:1,grep:1,split:1,require:1,caller:1,lcfirst:1,until:1,warn:1,"while":1,values:1,shift:1,telldir:1,getpwuid:1,my:1,getprotobynumber:1,"delete":1,and:1,sort:1,uc:1,defined:1,srand:1,accept:1,"package":1,seekdir:1,getprotobyname:1,semop:1,our:1,rename:1,seek:1,"if":1,q:1,chroot:1,sysread:1,setpwent:1,no:1,crypt:1,getc:1,chown:1,sqrt:1,write:1,setnetent:1,setpriority:1,foreach:1,tie:1,sin:1,msgget:1,map:1,stat:1,getlogin:1,unless:1,elsif:1,truncate:1,exec:1,keys:1,glob:1,tied:1,closedir:1,ioctl:1,socket:1,readlink:1,"eval":1,xor:1,readline:1,binmode:1,setservent:1,eof:1,ord:1,bind:1,alarm:1,pipe:1,atan2:1,getgrent:1,exp:1,time:1,push:1,setgrent:1,gt:1,lt:1,or:1,ne:1,m:1};var d={cN:"subst",b:"[$@]\\{",e:"}",k:c,r:10};var b={cN:"variable",b:"\\$\\d"};var a={cN:"variable",b:"[\\$\\%\\@\\*](\\^\\w\\b|#\\w+(\\:\\:\\w+)*|[^\\s\\w{]|{\\w+}|\\w+(\\:\\:\\w*)*)"};var g=[hljs.BE,d,b,a];var f={b:"->",c:[{b:hljs.IR},{b:"{",e:"}"}]};var e=[b,a,hljs.HCM,{cN:"comment",b:"^(__END__|__DATA__)",e:"\\n$",r:5},f,{cN:"string",b:"q[qwxr]?\\s*\\(",e:"\\)",c:g,r:5},{cN:"string",b:"q[qwxr]?\\s*\\[",e:"\\]",c:g,r:5},{cN:"string",b:"q[qwxr]?\\s*\\{",e:"\\}",c:g,r:5},{cN:"string",b:"q[qwxr]?\\s*\\|",e:"\\|",c:g,r:5},{cN:"string",b:"q[qwxr]?\\s*\\<",e:"\\>",c:g,r:5},{cN:"string",b:"qw\\s+q",e:"q",c:g,r:5},{cN:"string",b:"'",e:"'",c:[hljs.BE],r:0},{cN:"string",b:'"',e:'"',c:g,r:0},{cN:"string",b:"`",e:"`",c:[hljs.BE]},{cN:"string",b:"{\\w+}",r:0},{cN:"string",b:"-?\\w+\\s*\\=\\>",r:0},{cN:"number",b:"(\\b0[0-7_]+)|(\\b0x[0-9a-fA-F_]+)|(\\b[1-9][0-9_]*(\\.[0-9_]+)?)|[0_]\\b",r:0},{cN:"regexp",b:"(s|tr|y)/(\\\\.|[^/])*/(\\\\.|[^/])*/[a-z]*",r:10},{cN:"regexp",b:"(m|qr)?/",e:"/[a-z]*",c:[hljs.BE],r:0},{cN:"sub",b:"\\bsub\\b",e:"(\\s*\\(.*?\\))?[;{]",k:{sub:1},r:5},{cN:"operator",b:"-\\w\\b",r:0},{cN:"pod",b:"\\=\\w",e:"\\=cut"}];d.c=e;f.c[1].c=e;return{dM:{k:c,c:e}}}();hljs.LANGUAGES.cpp=function(){var b={keyword:{"false":1,"int":1,"float":1,"while":1,"private":1,"char":1,"catch":1,"export":1,virtual:1,operator:2,sizeof:2,dynamic_cast:2,typedef:2,const_cast:2,"const":1,struct:1,"for":1,static_cast:2,union:1,namespace:1,unsigned:1,"long":1,"throw":1,"volatile":2,"static":1,"protected":1,bool:1,template:1,mutable:1,"if":1,"public":1,friend:2,"do":1,"return":1,"goto":1,auto:1,"void":2,"enum":1,"else":1,"break":1,"new":1,extern:1,using:1,"true":1,"class":1,asm:1,"case":1,typeid:1,"short":1,reinterpret_cast:2,"default":1,"double":1,register:1,explicit:1,signed:1,typename:1,"try":1,"this":1,"switch":1,"continue":1,wchar_t:1,inline:1,"delete":1,alignof:1,char16_t:1,char32_t:1,constexpr:1,decltype:1,noexcept:1,nullptr:1,static_assert:1,thread_local:1},built_in:{std:1,string:1,cin:1,cout:1,cerr:1,clog:1,stringstream:1,istringstream:1,ostringstream:1,auto_ptr:1,deque:1,list:1,queue:1,stack:1,vector:1,map:1,set:1,bitset:1,multiset:1,multimap:1,unordered_set:1,unordered_map:1,unordered_multiset:1,unordered_multimap:1,array:1,shared_ptr:1}};var a={cN:"stl_container",b:"\\b(deque|list|queue|stack|vector|map|set|bitset|multiset|multimap|unordered_map|unordered_set|unordered_multiset|unordered_multimap|array)\\s*<",e:">",k:b,r:10};a.c=[a];return{dM:{k:b,i:"</",c:[hljs.CLCM,hljs.CBLCLM,hljs.QSM,{cN:"string",b:"'",e:"[^\\\\]'",i:"[^\\\\][^']"},hljs.CNM,{cN:"preprocessor",b:"#",e:"$"},a]}}}(); No newline at end of file |
@@ -0,0 +1,32 b'' | |||||
|
1 | // From https://gist.github.com/1343518 | |||
|
2 | // Modified by Hakim to handle Markdown indented with tabs | |||
|
3 | (function(){ | |||
|
4 | ||||
|
5 | if( typeof Showdown === 'undefined' ) { | |||
|
6 | throw 'The reveal.js Markdown plugin requires Showdown to be loaded'; | |||
|
7 | } | |||
|
8 | ||||
|
9 | var sections = document.querySelectorAll( '[data-markdown]' ); | |||
|
10 | ||||
|
11 | for( var i = 0, len = sections.length; i < len; i++ ) { | |||
|
12 | var section = sections[i]; | |||
|
13 | ||||
|
14 | var template = section.querySelector( 'script' ); | |||
|
15 | ||||
|
16 | // strip leading whitespace so it isn't evaluated as code | |||
|
17 | var text = ( template || section ).innerHTML; | |||
|
18 | ||||
|
19 | var leadingWs = text.match(/^\n?(\s*)/)[1].length, | |||
|
20 | leadingTabs = text.match(/^\n?(\t*)/)[1].length; | |||
|
21 | ||||
|
22 | if( leadingTabs > 0 ) { | |||
|
23 | text = text.replace( new RegExp('\\n?\\t{' + leadingTabs + '}','g'), '\n' ); | |||
|
24 | } | |||
|
25 | else if( leadingWs > 1 ) { | |||
|
26 | text = text.replace( new RegExp('\\n? {' + leadingWs + '}','g'), '\n' ); | |||
|
27 | } | |||
|
28 | ||||
|
29 | section.innerHTML = (new Showdown.converter()).makeHtml(text); | |||
|
30 | } | |||
|
31 | ||||
|
32 | })(); No newline at end of file |
@@ -0,0 +1,62 b'' | |||||
|
1 | // | |||
|
2 | // showdown.js -- A javascript port of Markdown. | |||
|
3 | // | |||
|
4 | // Copyright (c) 2007 John Fraser. | |||
|
5 | // | |||
|
6 | // Original Markdown Copyright (c) 2004-2005 John Gruber | |||
|
7 | // <http://daringfireball.net/projects/markdown/> | |||
|
8 | // | |||
|
9 | // Redistributable under a BSD-style open source license. | |||
|
10 | // See license.txt for more information. | |||
|
11 | // | |||
|
12 | // The full source distribution is at: | |||
|
13 | // | |||
|
14 | // A A L | |||
|
15 | // T C A | |||
|
16 | // T K B | |||
|
17 | // | |||
|
18 | // <http://www.attacklab.net/> | |||
|
19 | // | |||
|
20 | // | |||
|
21 | // Wherever possible, Showdown is a straight, line-by-line port | |||
|
22 | // of the Perl version of Markdown. | |||
|
23 | // | |||
|
24 | // This is not a normal parser design; it's basically just a | |||
|
25 | // series of string substitutions. It's hard to read and | |||
|
26 | // maintain this way, but keeping Showdown close to the original | |||
|
27 | // design makes it easier to port new features. | |||
|
28 | // | |||
|
29 | // More importantly, Showdown behaves like markdown.pl in most | |||
|
30 | // edge cases. So web applications can do client-side preview | |||
|
31 | // in Javascript, and then build identical HTML on the server. | |||
|
32 | // | |||
|
33 | // This port needs the new RegExp functionality of ECMA 262, | |||
|
34 | // 3rd Edition (i.e. Javascript 1.5). Most modern web browsers | |||
|
35 | // should do fine. Even with the new regular expression features, | |||
|
36 | // We do a lot of work to emulate Perl's regex functionality. | |||
|
37 | // The tricky changes in this file mostly have the "attacklab:" | |||
|
38 | // label. Major or self-explanatory changes don't. | |||
|
39 | // | |||
|
40 | // Smart diff tools like Araxis Merge will be able to match up | |||
|
41 | // this file with markdown.pl in a useful way. A little tweaking | |||
|
42 | // helps: in a copy of markdown.pl, replace "#" with "//" and | |||
|
43 | // replace "$text" with "text". Be sure to ignore whitespace | |||
|
44 | // and line endings. | |||
|
45 | // | |||
|
46 | // | |||
|
47 | // Showdown usage: | |||
|
48 | // | |||
|
49 | // var text = "Markdown *rocks*."; | |||
|
50 | // | |||
|
51 | // var converter = new Showdown.converter(); | |||
|
52 | // var html = converter.makeHtml(text); | |||
|
53 | // | |||
|
54 | // alert(html); | |||
|
55 | // | |||
|
56 | // Note: move the sample code to the bottom of this | |||
|
57 | // file before uncommenting it. | |||
|
58 | // | |||
|
59 | // | |||
|
60 | // Showdown namespace | |||
|
61 | // | |||
|
62 | var Showdown={};Showdown.converter=function(){var a,b,c,d=0;this.makeHtml=function(d){return a=new Array,b=new Array,c=new Array,d=d.replace(/~/g,"~T"),d=d.replace(/\$/g,"~D"),d=d.replace(/\r\n/g,"\n"),d=d.replace(/\r/g,"\n"),d="\n\n"+d+"\n\n",d=F(d),d=d.replace(/^[ \t]+$/mg,""),d=f(d),d=e(d),d=h(d),d=D(d),d=d.replace(/~D/g,"$$"),d=d.replace(/~T/g,"~"),d};var e=function(c){var c=c.replace(/^[ ]{0,3}\[(.+)\]:[ \t]*\n?[ \t]*<?(\S+?)>?[ \t]*\n?[ \t]*(?:(\n*)["(](.+?)[")][ \t]*)?(?:\n+|\Z)/gm,function(c,d,e,f,g){return d=d.toLowerCase(),a[d]=z(e),f?f+g:(g&&(b[d]=g.replace(/"/g,""")),"")});return c},f=function(a){a=a.replace(/\n/g,"\n\n");var b="p|div|h[1-6]|blockquote|pre|table|dl|ol|ul|script|noscript|form|fieldset|iframe|math|ins|del",c="p|div|h[1-6]|blockquote|pre|table|dl|ol|ul|script|noscript|form|fieldset|iframe|math";return a=a.replace(/^(<(p|div|h[1-6]|blockquote|pre|table|dl|ol|ul|script|noscript|form|fieldset|iframe|math|ins|del)\b[^\r]*?\n<\/\2>[ \t]*(?=\n+))/gm,g),a=a.replace(/^(<(p|div|h[1-6]|blockquote|pre|table|dl|ol|ul|script|noscript|form|fieldset|iframe|math)\b[^\r]*?.*<\/\2>[ \t]*(?=\n+)\n)/gm,g),a=a.replace(/(\n[ ]{0,3}(<(hr)\b([^<>])*?\/?>)[ \t]*(?=\n{2,}))/g,g),a=a.replace(/(\n\n[ ]{0,3}<!(--[^\r]*?--\s*)+>[ \t]*(?=\n{2,}))/g,g),a=a.replace(/(?:\n\n)([ ]{0,3}(?:<([?%])[^\r]*?\2>)[ \t]*(?=\n{2,}))/g,g),a=a.replace(/\n\n/g,"\n"),a},g=function(a,b){var d=b;return d=d.replace(/\n\n/g,"\n"),d=d.replace(/^\n/,""),d=d.replace(/\n+$/g,""),d="\n\n~K"+(c.push(d)-1)+"K\n\n",d},h=function(a){a=o(a);var b=t("<hr />");return a=a.replace(/^[ ]{0,2}([ ]?\*[ ]?){3,}[ \t]*$/gm,b),a=a.replace(/^[ ]{0,2}([ ]?\-[ ]?){3,}[ \t]*$/gm,b),a=a.replace(/^[ ]{0,2}([ ]?\_[ ]?){3,}[ \t]*$/gm,b),a=q(a),a=s(a),a=r(a),a=x(a),a=f(a),a=y(a),a},i=function(a){return a=u(a),a=j(a),a=A(a),a=m(a),a=k(a),a=B(a),a=z(a),a=w(a),a=a.replace(/ +\n/g," <br />\n"),a},j=function(a){var b=/(<[a-z\/!$]("[^"]*"|'[^']*'|[^'">])*>|<!(--.*?--\s*)+>)/gi;return a=a.replace(b,function(a){var b=a.replace(/(.)<\/?code>(?=.)/g,"$1`");return b=G(b,"\\`*_"),b}),a},k=function(a){return a=a.replace(/(\[((?:\[[^\]]*\]|[^\[\]])*)\][ ]?(?:\n[ ]*)?\[(.*?)\])()()()()/g,l),a=a.replace(/(\[((?:\[[^\]]*\]|[^\[\]])*)\]\([ \t]*()<?(.*?)>?[ \t]*((['"])(.*?)\6[ \t]*)?\))/g,l),a=a.replace(/(\[([^\[\]]+)\])()()()()()/g,l),a},l=function(c,d,e,f,g,h,i,j){j==undefined&&(j="");var k=d,l=e,m=f.toLowerCase(),n=g,o=j;if(n==""){m==""&&(m=l.toLowerCase().replace(/ ?\n/g," ")),n="#"+m;if(a[m]!=undefined)n=a[m],b[m]!=undefined&&(o=b[m]);else{if(!(k.search(/\(\s*\)$/m)>-1))return k;n=""}}n=G(n,"*_");var p='<a href="'+n+'"';return o!=""&&(o=o.replace(/"/g,"""),o=G(o,"*_"),p+=' title="'+o+'"'),p+=">"+l+"</a>",p},m=function(a){return a=a.replace(/(!\[(.*?)\][ ]?(?:\n[ ]*)?\[(.*?)\])()()()()/g,n),a=a.replace(/(!\[(.*?)\]\s?\([ \t]*()<?(\S+?)>?[ \t]*((['"])(.*?)\6[ \t]*)?\))/g,n),a},n=function(c,d,e,f,g,h,i,j){var k=d,l=e,m=f.toLowerCase(),n=g,o=j;o||(o="");if(n==""){m==""&&(m=l.toLowerCase().replace(/ ?\n/g," ")),n="#"+m;if(a[m]==undefined)return k;n=a[m],b[m]!=undefined&&(o=b[m])}l=l.replace(/"/g,"""),n=G(n,"*_");var p='<img src="'+n+'" alt="'+l+'"';return o=o.replace(/"/g,"""),o=G(o,"*_"),p+=' title="'+o+'"',p+=" />",p},o=function(a){function b(a){return a.replace(/[^\w]/g,"").toLowerCase()}return a=a.replace(/^(.+)[ \t]*\n=+[ \t]*\n+/gm,function(a,c){return t('<h1 id="'+b(c)+'">'+i(c)+"</h1>")}),a=a.replace(/^(.+)[ \t]*\n-+[ \t]*\n+/gm,function(a,c){return t('<h2 id="'+b(c)+'">'+i(c)+"</h2>")}),a=a.replace(/^(\#{1,6})[ \t]*(.+?)[ \t]*\#*\n+/gm,function(a,c,d){var e=c.length;return t("<h"+e+' id="'+b(d)+'">'+i(d)+"</h"+e+">")}),a},p,q=function(a){a+="~0";var b=/^(([ ]{0,3}([*+-]|\d+[.])[ \t]+)[^\r]+?(~0|\n{2,}(?=\S)(?![ \t]*(?:[*+-]|\d+[.])[ \t]+)))/gm;return d?a=a.replace(b,function(a,b,c){var d=b,e=c.search(/[*+-]/g)>-1?"ul":"ol";d=d.replace(/\n{2,}/g,"\n\n\n");var f=p(d);return f=f.replace(/\s+$/,""),f="<"+e+">"+f+"</"+e+">\n",f}):(b=/(\n\n|^\n?)(([ ]{0,3}([*+-]|\d+[.])[ \t]+)[^\r]+?(~0|\n{2,}(?=\S)(?![ \t]*(?:[*+-]|\d+[.])[ \t]+)))/g,a=a.replace(b,function(a,b,c,d){var e=b,f=c,g=d.search(/[*+-]/g)>-1?"ul":"ol",f=f.replace(/\n{2,}/g,"\n\n\n"),h=p(f);return h=e+"<"+g+">\n"+h+"</"+g+">\n",h})),a=a.replace(/~0/,""),a};p=function(a){return d++,a=a.replace(/\n{2,}$/,"\n"),a+="~0",a=a.replace(/(\n)?(^[ \t]*)([*+-]|\d+[.])[ \t]+([^\r]+?(\n{1,2}))(?=\n*(~0|\2([*+-]|\d+[.])[ \t]+))/gm,function(a,b,c,d,e){var f=e,g=b,j=c;return g||f.search(/\n{2,}/)>-1?f=h(E(f)):(f=q(E(f)),f=f.replace(/\n$/,""),f=i(f)),"<li>"+f+"</li>\n"}),a=a.replace(/~0/g,""),d--,a};var r=function(a){return a+="~0",a=a.replace(/(?:\n\n|^)((?:(?:[ ]{4}|\t).*\n+)+)(\n*[ ]{0,3}[^ \t\n]|(?=~0))/g,function(a,b,c){var d=b,e=c;return d=v(E(d)),d=F(d),d=d.replace(/^\n+/g,""),d=d.replace(/\n+$/g,""),d="<pre><code>"+d+"\n</code></pre>",t(d)+e}),a=a.replace(/~0/,""),a},s=function(a){return a+="~0",a=a.replace(/\n```(.*)\n([^`]+)\n```/g,function(a,b,c){var d=b,e=c;return e=v(e),e=F(e),e=e.replace(/^\n+/g,""),e=e.replace(/\n+$/g,""),e="<pre><code class="+d+">"+e+"\n</code></pre>",t(e)}),a=a.replace(/~0/,""),a},t=function(a){return a=a.replace(/(^\n+|\n+$)/g,""),"\n\n~K"+(c.push(a)-1)+"K\n\n"},u=function(a){return a=a.replace(/(^|[^\\])(`+)([^\r]*?[^`])\2(?!`)/gm,function(a,b,c,d,e){var f=d;return f=f.replace(/^([ \t]*)/g,""),f=f.replace(/[ \t]*$/g,""),f=v(f),b+"<code>"+f+"</code>"}),a},v=function(a){return a=a.replace(/&/g,"&"),a=a.replace(/</g,"<"),a=a.replace(/>/g,">"),a=G(a,"*_{}[]\\",!1),a},w=function(a){return a=a.replace(/(\*\*|__)(?=\S)([^\r]*?\S[*_]*)\1/g,"<strong>$2</strong>"),a=a.replace(/(\*|_)(?=\S)([^\r]*?\S)\1/g,"<em>$2</em>"),a},x=function(a){return a=a.replace(/((^[ \t]*>[ \t]?.+\n(.+\n)*\n*)+)/gm,function(a,b){var c=b;return c=c.replace(/^[ \t]*>[ \t]?/gm,"~0"),c=c.replace(/~0/g,""),c=c.replace(/^[ \t]+$/gm,""),c=h(c),c=c.replace(/(^|\n)/g,"$1 "),c=c.replace(/(\s*<pre>[^\r]+?<\/pre>)/gm,function(a,b){var c=b;return c=c.replace(/^ /mg,"~0"),c=c.replace(/~0/g,""),c}),t("<blockquote>\n"+c+"\n</blockquote>")}),a},y=function(a){a=a.replace(/^\n+/g,""),a=a.replace(/\n+$/g,"");var b=a.split(/\n{2,}/g),d=new Array,e=b.length;for(var f=0;f<e;f++){var g=b[f];g.search(/~K(\d+)K/g)>=0?d.push(g):g.search(/\S/)>=0&&(g=i(g),g=g.replace(/^([ \t]*)/g,"<p>"),g+="</p>",d.push(g))}e=d.length;for(var f=0;f<e;f++)while(d[f].search(/~K(\d+)K/)>=0){var h=c[RegExp.$1];h=h.replace(/\$/g,"$$$$"),d[f]=d[f].replace(/~K\d+K/,h)}return d.join("\n\n")},z=function(a){return a=a.replace(/&(?!#?[xX]?(?:[0-9a-fA-F]+|\w+);)/g,"&"),a=a.replace(/<(?![a-z\/?\$!])/gi,"<"),a},A=function(a){return a=a.replace(/\\(\\)/g,H),a=a.replace(/\\([`*_{}\[\]()>#+-.!])/g,H),a},B=function(a){return a=a.replace(/<((https?|ftp|dict):[^'">\s]+)>/gi,'<a href="$1">$1</a>'),a=a.replace(/<(?:mailto:)?([-.\w]+\@[-a-z0-9]+(\.[-a-z0-9]+)*\.[a-z]+)>/gi,function(a,b){return C(D(b))}),a},C=function(a){function b(a){var b="0123456789ABCDEF",c=a.charCodeAt(0);return b.charAt(c>>4)+b.charAt(c&15)}var c=[function(a){return"&#"+a.charCodeAt(0)+";"},function(a){return"&#x"+b(a)+";"},function(a){return a}];return a="mailto:"+a,a=a.replace(/./g,function(a){if(a=="@")a=c[Math.floor(Math.random()*2)](a);else if(a!=":"){var b=Math.random();a=b>.9?c[2](a):b>.45?c[1](a):c[0](a)}return a}),a='<a href="'+a+'">'+a+"</a>",a=a.replace(/">.+:/g,'">'),a},D=function(a){return a=a.replace(/~E(\d+)E/g,function(a,b){var c=parseInt(b);return String.fromCharCode(c)}),a},E=function(a){return a=a.replace(/^(\t|[ ]{1,4})/gm,"~0"),a=a.replace(/~0/g,""),a},F=function(a){return a=a.replace(/\t(?=\t)/g," "),a=a.replace(/\t/g,"~A~B"),a=a.replace(/~B(.+?)~A/g,function(a,b,c){var d=b,e=4-d.length%4;for(var f=0;f<e;f++)d+=" ";return d}),a=a.replace(/~A/g," "),a=a.replace(/~B/g,""),a},G=function(a,b,c){var d="(["+b.replace(/([\[\]\\])/g,"\\$1")+"])";c&&(d="\\\\"+d);var e=new RegExp(d,"g");return a=a.replace(e,H),a},H=function(a,b){var c=b.charCodeAt(0);return"~E"+c+"E"}},typeof exports!="undefined"&&(exports=Showdown); No newline at end of file |
@@ -0,0 +1,57 b'' | |||||
|
1 | (function() { | |||
|
2 | // don't emit events from inside the previews themselves | |||
|
3 | if ( window.location.search.match( /receiver/gi ) ) { return; } | |||
|
4 | ||||
|
5 | var socket = io.connect(window.location.origin); | |||
|
6 | var socketId = Math.random().toString().slice(2); | |||
|
7 | ||||
|
8 | console.log('View slide notes at ' + window.location.origin + '/notes/' + socketId); | |||
|
9 | window.open(window.location.origin + '/notes/' + socketId, 'notes-' + socketId); | |||
|
10 | ||||
|
11 | // Fires when a fragment is shown | |||
|
12 | Reveal.addEventListener( 'fragmentshown', function( event ) { | |||
|
13 | var fragmentData = { | |||
|
14 | fragment : 'next', | |||
|
15 | socketId : socketId | |||
|
16 | }; | |||
|
17 | socket.emit('fragmentchanged', fragmentData); | |||
|
18 | } ); | |||
|
19 | ||||
|
20 | // Fires when a fragment is hidden | |||
|
21 | Reveal.addEventListener( 'fragmenthidden', function( event ) { | |||
|
22 | var fragmentData = { | |||
|
23 | fragment : 'previous', | |||
|
24 | socketId : socketId | |||
|
25 | }; | |||
|
26 | socket.emit('fragmentchanged', fragmentData); | |||
|
27 | } ); | |||
|
28 | ||||
|
29 | // Fires when slide is changed | |||
|
30 | Reveal.addEventListener( 'slidechanged', function( event ) { | |||
|
31 | var nextindexh; | |||
|
32 | var nextindexv; | |||
|
33 | var slideElement = event.currentSlide; | |||
|
34 | ||||
|
35 | if (slideElement.nextElementSibling && slideElement.parentNode.nodeName == 'SECTION') { | |||
|
36 | nextindexh = event.indexh; | |||
|
37 | nextindexv = event.indexv + 1; | |||
|
38 | } else { | |||
|
39 | nextindexh = event.indexh + 1; | |||
|
40 | nextindexv = 0; | |||
|
41 | } | |||
|
42 | ||||
|
43 | var notes = slideElement.querySelector('aside.notes'); | |||
|
44 | var slideData = { | |||
|
45 | notes : notes ? notes.innerHTML : '', | |||
|
46 | indexh : event.indexh, | |||
|
47 | indexv : event.indexv, | |||
|
48 | nextindexh : nextindexh, | |||
|
49 | nextindexv : nextindexv, | |||
|
50 | socketId : socketId, | |||
|
51 | markdown : notes ? typeof notes.getAttribute('data-markdown') === 'string' : false | |||
|
52 | ||||
|
53 | }; | |||
|
54 | ||||
|
55 | socket.emit('slidechanged', slideData); | |||
|
56 | } ); | |||
|
57 | }()); |
@@ -0,0 +1,58 b'' | |||||
|
1 | var express = require('express'); | |||
|
2 | var fs = require('fs'); | |||
|
3 | var io = require('socket.io'); | |||
|
4 | var _ = require('underscore'); | |||
|
5 | var Mustache = require('mustache'); | |||
|
6 | ||||
|
7 | var app = express.createServer(); | |||
|
8 | var staticDir = express.static; | |||
|
9 | ||||
|
10 | io = io.listen(app); | |||
|
11 | ||||
|
12 | var opts = { | |||
|
13 | port : 1947, | |||
|
14 | baseDir : __dirname + '/../../' | |||
|
15 | }; | |||
|
16 | ||||
|
17 | io.sockets.on('connection', function(socket) { | |||
|
18 | socket.on('slidechanged', function(slideData) { | |||
|
19 | socket.broadcast.emit('slidedata', slideData); | |||
|
20 | }); | |||
|
21 | socket.on('fragmentchanged', function(fragmentData) { | |||
|
22 | socket.broadcast.emit('fragmentdata', fragmentData); | |||
|
23 | }); | |||
|
24 | }); | |||
|
25 | ||||
|
26 | app.configure(function() { | |||
|
27 | [ 'css', 'js', 'images', 'plugin', 'lib' ].forEach(function(dir) { | |||
|
28 | app.use('/' + dir, staticDir(opts.baseDir + dir)); | |||
|
29 | }); | |||
|
30 | }); | |||
|
31 | ||||
|
32 | app.get("/", function(req, res) { | |||
|
33 | fs.createReadStream(opts.baseDir + '/index.html').pipe(res); | |||
|
34 | }); | |||
|
35 | ||||
|
36 | app.get("/notes/:socketId", function(req, res) { | |||
|
37 | ||||
|
38 | fs.readFile(opts.baseDir + 'plugin/notes-server/notes.html', function(err, data) { | |||
|
39 | res.send(Mustache.to_html(data.toString(), { | |||
|
40 | socketId : req.params.socketId | |||
|
41 | })); | |||
|
42 | }); | |||
|
43 | // fs.createReadStream(opts.baseDir + 'notes-server/notes.html').pipe(res); | |||
|
44 | }); | |||
|
45 | ||||
|
46 | // Actually listen | |||
|
47 | app.listen(opts.port || null); | |||
|
48 | ||||
|
49 | var brown = '\033[33m', | |||
|
50 | green = '\033[32m', | |||
|
51 | reset = '\033[0m'; | |||
|
52 | ||||
|
53 | var slidesLocation = "http://localhost" + ( opts.port ? ( ':' + opts.port ) : '' ); | |||
|
54 | ||||
|
55 | console.log( brown + "reveal.js - Speaker Notes" + reset ); | |||
|
56 | console.log( "1. Open the slides at " + green + slidesLocation + reset ); | |||
|
57 | console.log( "2. Click on the link your JS console to go to the notes page" ); | |||
|
58 | console.log( "3. Advance through your slides and your notes will advance automatically" ); |
@@ -0,0 +1,139 b'' | |||||
|
1 | <!doctype html> | |||
|
2 | <html lang="en"> | |||
|
3 | <head> | |||
|
4 | <meta charset="utf-8"> | |||
|
5 | ||||
|
6 | <title>reveal.js - Slide Notes</title> | |||
|
7 | ||||
|
8 | <style> | |||
|
9 | body { | |||
|
10 | font-family: Helvetica; | |||
|
11 | } | |||
|
12 | ||||
|
13 | #notes { | |||
|
14 | font-size: 24px; | |||
|
15 | width: 640px; | |||
|
16 | margin-top: 5px; | |||
|
17 | } | |||
|
18 | ||||
|
19 | #wrap-current-slide { | |||
|
20 | width: 640px; | |||
|
21 | height: 512px; | |||
|
22 | float: left; | |||
|
23 | overflow: hidden; | |||
|
24 | } | |||
|
25 | ||||
|
26 | #current-slide { | |||
|
27 | width: 1280px; | |||
|
28 | height: 1024px; | |||
|
29 | border: none; | |||
|
30 | ||||
|
31 | -webkit-transform-origin: 0 0; | |||
|
32 | -moz-transform-origin: 0 0; | |||
|
33 | -ms-transform-origin: 0 0; | |||
|
34 | -o-transform-origin: 0 0; | |||
|
35 | transform-origin: 0 0; | |||
|
36 | ||||
|
37 | -webkit-transform: scale(0.5); | |||
|
38 | -moz-transform: scale(0.5); | |||
|
39 | -ms-transform: scale(0.5); | |||
|
40 | -o-transform: scale(0.5); | |||
|
41 | transform: scale(0.5); | |||
|
42 | } | |||
|
43 | ||||
|
44 | #wrap-next-slide { | |||
|
45 | width: 448px; | |||
|
46 | height: 358px; | |||
|
47 | float: left; | |||
|
48 | margin: 0 0 0 10px; | |||
|
49 | overflow: hidden; | |||
|
50 | } | |||
|
51 | ||||
|
52 | #next-slide { | |||
|
53 | width: 1280px; | |||
|
54 | height: 1024px; | |||
|
55 | border: none; | |||
|
56 | ||||
|
57 | -webkit-transform-origin: 0 0; | |||
|
58 | -moz-transform-origin: 0 0; | |||
|
59 | -ms-transform-origin: 0 0; | |||
|
60 | -o-transform-origin: 0 0; | |||
|
61 | transform-origin: 0 0; | |||
|
62 | ||||
|
63 | -webkit-transform: scale(0.35); | |||
|
64 | -moz-transform: scale(0.35); | |||
|
65 | -ms-transform: scale(0.35); | |||
|
66 | -o-transform: scale(0.35); | |||
|
67 | transform: scale(0.35); | |||
|
68 | } | |||
|
69 | ||||
|
70 | .slides { | |||
|
71 | position: relative; | |||
|
72 | margin-bottom: 10px; | |||
|
73 | border: 1px solid black; | |||
|
74 | border-radius: 2px; | |||
|
75 | background: rgb(28, 30, 32); | |||
|
76 | } | |||
|
77 | ||||
|
78 | .slides span { | |||
|
79 | position: absolute; | |||
|
80 | top: 3px; | |||
|
81 | left: 3px; | |||
|
82 | font-weight: bold; | |||
|
83 | font-size: 14px; | |||
|
84 | color: rgba( 255, 255, 255, 0.9 ); | |||
|
85 | } | |||
|
86 | </style> | |||
|
87 | </head> | |||
|
88 | ||||
|
89 | <body> | |||
|
90 | ||||
|
91 | <div id="wrap-current-slide" class="slides"> | |||
|
92 | <iframe src="/?receiver" width="1280" height="1024" id="current-slide"></iframe> | |||
|
93 | </div> | |||
|
94 | ||||
|
95 | <div id="wrap-next-slide" class="slides"> | |||
|
96 | <iframe src="/?receiver" width="640" height="512" id="next-slide"></iframe> | |||
|
97 | <span>UPCOMING:</span> | |||
|
98 | </div> | |||
|
99 | <div id="notes"></div> | |||
|
100 | ||||
|
101 | <script src="/socket.io/socket.io.js"></script> | |||
|
102 | <script src="/plugin/markdown/showdown.js"></script> | |||
|
103 | ||||
|
104 | <script> | |||
|
105 | var socketId = '{{socketId}}'; | |||
|
106 | var socket = io.connect(window.location.origin); | |||
|
107 | var notes = document.getElementById('notes'); | |||
|
108 | var currentSlide = document.getElementById('current-slide'); | |||
|
109 | var nextSlide = document.getElementById('next-slide'); | |||
|
110 | ||||
|
111 | socket.on('slidedata', function(data) { | |||
|
112 | // ignore data from sockets that aren't ours | |||
|
113 | if (data.socketId !== socketId) { return; } | |||
|
114 | ||||
|
115 | if (data.markdown) { | |||
|
116 | notes.innerHTML = (new Showdown.converter()).makeHtml(data.notes); | |||
|
117 | } | |||
|
118 | else { | |||
|
119 | notes.innerHTML = data.notes; | |||
|
120 | } | |||
|
121 | ||||
|
122 | currentSlide.contentWindow.Reveal.slide(data.indexh, data.indexv); | |||
|
123 | nextSlide.contentWindow.Reveal.slide(data.nextindexh, data.nextindexv); | |||
|
124 | }); | |||
|
125 | socket.on('fragmentdata', function(data) { | |||
|
126 | // ignore data from sockets that aren't ours | |||
|
127 | if (data.socketId !== socketId) { return; } | |||
|
128 | ||||
|
129 | if (data.fragment === 'next') { | |||
|
130 | currentSlide.contentWindow.Reveal.nextFragment(); | |||
|
131 | } | |||
|
132 | else if (data.fragment === 'previous') { | |||
|
133 | currentSlide.contentWindow.Reveal.prevFragment(); | |||
|
134 | } | |||
|
135 | }); | |||
|
136 | </script> | |||
|
137 | ||||
|
138 | </body> | |||
|
139 | </html> |
@@ -0,0 +1,142 b'' | |||||
|
1 | <!doctype html> | |||
|
2 | <html lang="en"> | |||
|
3 | <head> | |||
|
4 | <meta charset="utf-8"> | |||
|
5 | ||||
|
6 | <title>reveal.js - Slide Notes</title> | |||
|
7 | ||||
|
8 | <style> | |||
|
9 | body { | |||
|
10 | font-family: Helvetica; | |||
|
11 | } | |||
|
12 | ||||
|
13 | #notes { | |||
|
14 | font-size: 24px; | |||
|
15 | width: 640px; | |||
|
16 | margin-top: 5px; | |||
|
17 | } | |||
|
18 | ||||
|
19 | #wrap-current-slide { | |||
|
20 | width: 640px; | |||
|
21 | height: 512px; | |||
|
22 | float: left; | |||
|
23 | overflow: hidden; | |||
|
24 | } | |||
|
25 | ||||
|
26 | #current-slide { | |||
|
27 | width: 1280px; | |||
|
28 | height: 1024px; | |||
|
29 | border: none; | |||
|
30 | ||||
|
31 | -webkit-transform-origin: 0 0; | |||
|
32 | -moz-transform-origin: 0 0; | |||
|
33 | -ms-transform-origin: 0 0; | |||
|
34 | -o-transform-origin: 0 0; | |||
|
35 | transform-origin: 0 0; | |||
|
36 | ||||
|
37 | -webkit-transform: scale(0.5); | |||
|
38 | -moz-transform: scale(0.5); | |||
|
39 | -ms-transform: scale(0.5); | |||
|
40 | -o-transform: scale(0.5); | |||
|
41 | transform: scale(0.5); | |||
|
42 | } | |||
|
43 | ||||
|
44 | #wrap-next-slide { | |||
|
45 | width: 448px; | |||
|
46 | height: 358px; | |||
|
47 | float: left; | |||
|
48 | margin: 0 0 0 10px; | |||
|
49 | overflow: hidden; | |||
|
50 | } | |||
|
51 | ||||
|
52 | #next-slide { | |||
|
53 | width: 1280px; | |||
|
54 | height: 1024px; | |||
|
55 | border: none; | |||
|
56 | ||||
|
57 | -webkit-transform-origin: 0 0; | |||
|
58 | -moz-transform-origin: 0 0; | |||
|
59 | -ms-transform-origin: 0 0; | |||
|
60 | -o-transform-origin: 0 0; | |||
|
61 | transform-origin: 0 0; | |||
|
62 | ||||
|
63 | -webkit-transform: scale(0.35); | |||
|
64 | -moz-transform: scale(0.35); | |||
|
65 | -ms-transform: scale(0.35); | |||
|
66 | -o-transform: scale(0.35); | |||
|
67 | transform: scale(0.35); | |||
|
68 | } | |||
|
69 | ||||
|
70 | .slides { | |||
|
71 | position: relative; | |||
|
72 | margin-bottom: 10px; | |||
|
73 | border: 1px solid black; | |||
|
74 | border-radius: 2px; | |||
|
75 | background: rgb(28, 30, 32); | |||
|
76 | } | |||
|
77 | ||||
|
78 | .slides span { | |||
|
79 | position: absolute; | |||
|
80 | top: 3px; | |||
|
81 | left: 3px; | |||
|
82 | font-weight: bold; | |||
|
83 | font-size: 14px; | |||
|
84 | color: rgba( 255, 255, 255, 0.9 ); | |||
|
85 | } | |||
|
86 | </style> | |||
|
87 | </head> | |||
|
88 | ||||
|
89 | <body> | |||
|
90 | ||||
|
91 | <div id="wrap-current-slide" class="slides"> | |||
|
92 | <iframe src="../../index.html" width="1280" height="1024" id="current-slide"></iframe> | |||
|
93 | </div> | |||
|
94 | ||||
|
95 | <div id="wrap-next-slide" class="slides"> | |||
|
96 | <iframe src="../../index.html" width="640" height="512" id="next-slide"></iframe> | |||
|
97 | <span>UPCOMING:</span> | |||
|
98 | </div> | |||
|
99 | <div id="notes"></div> | |||
|
100 | ||||
|
101 | <script src="../../plugin/markdown/showdown.js"></script> | |||
|
102 | <script> | |||
|
103 | window.addEventListener( 'load', function() { | |||
|
104 | ||||
|
105 | (function( window, undefined ) { | |||
|
106 | var notes = document.getElementById( 'notes' ), | |||
|
107 | currentSlide = document.getElementById( 'current-slide' ), | |||
|
108 | nextSlide = document.getElementById( 'next-slide' ); | |||
|
109 | ||||
|
110 | window.addEventListener( 'message', function( event ) { | |||
|
111 | var data = JSON.parse( event.data ); | |||
|
112 | // No need for updating the notes in case of fragment changes | |||
|
113 | if ( data.notes !== undefined) { | |||
|
114 | if( data.markdown ) { | |||
|
115 | notes.innerHTML = (new Showdown.converter()).makeHtml( data.notes ); | |||
|
116 | } | |||
|
117 | else { | |||
|
118 | notes.innerHTML = data.notes; | |||
|
119 | } | |||
|
120 | } | |||
|
121 | ||||
|
122 | // Update the note slides | |||
|
123 | currentSlide.contentWindow.Reveal.slide( data.indexh, data.indexv ); | |||
|
124 | nextSlide.contentWindow.Reveal.slide( data.nextindexh, data.nextindexv ); | |||
|
125 | ||||
|
126 | // Showing and hiding fragments | |||
|
127 | if( data.fragment === 'next' ) { | |||
|
128 | currentSlide.contentWindow.Reveal.nextFragment(); | |||
|
129 | } | |||
|
130 | else if( data.fragment === 'prev' ) { | |||
|
131 | currentSlide.contentWindow.Reveal.prevFragment(); | |||
|
132 | } | |||
|
133 | ||||
|
134 | }, false ); | |||
|
135 | ||||
|
136 | })( window ); | |||
|
137 | ||||
|
138 | }, false ); | |||
|
139 | ||||
|
140 | </script> | |||
|
141 | </body> | |||
|
142 | </html> |
@@ -0,0 +1,98 b'' | |||||
|
1 | /** | |||
|
2 | * Handles opening of and synchronization with the reveal.js | |||
|
3 | * notes window. | |||
|
4 | */ | |||
|
5 | var RevealNotes = (function() { | |||
|
6 | ||||
|
7 | function openNotes() { | |||
|
8 | var notesPopup = window.open( 'plugin/notes/notes.html', 'reveal.js - Notes', 'width=1120,height=850' ); | |||
|
9 | ||||
|
10 | // Fires when slide is changed | |||
|
11 | Reveal.addEventListener( 'slidechanged', function( event ) { | |||
|
12 | post('slidechanged'); | |||
|
13 | } ); | |||
|
14 | ||||
|
15 | // Fires when a fragment is shown | |||
|
16 | Reveal.addEventListener( 'fragmentshown', function( event ) { | |||
|
17 | post('fragmentshown'); | |||
|
18 | } ); | |||
|
19 | ||||
|
20 | // Fires when a fragment is hidden | |||
|
21 | Reveal.addEventListener( 'fragmenthidden', function( event ) { | |||
|
22 | post('fragmenthidden'); | |||
|
23 | } ); | |||
|
24 | ||||
|
25 | /** | |||
|
26 | * Posts the current slide data to the notes window | |||
|
27 | * | |||
|
28 | * @param {String} eventType Expecting 'slidechanged', 'fragmentshown' | |||
|
29 | * or 'fragmenthidden' set in the events above to define the needed | |||
|
30 | * slideDate. | |||
|
31 | */ | |||
|
32 | function post( eventType ) { | |||
|
33 | var slideElement = Reveal.getCurrentSlide(), | |||
|
34 | messageData; | |||
|
35 | ||||
|
36 | if( eventType === 'slidechanged' ) { | |||
|
37 | var notes = slideElement.querySelector( 'aside.notes' ), | |||
|
38 | indexh = Reveal.getIndices().h, | |||
|
39 | indexv = Reveal.getIndices().v, | |||
|
40 | nextindexh, | |||
|
41 | nextindexv; | |||
|
42 | ||||
|
43 | if( slideElement.nextElementSibling && slideElement.parentNode.nodeName == 'SECTION' ) { | |||
|
44 | nextindexh = indexh; | |||
|
45 | nextindexv = indexv + 1; | |||
|
46 | } else { | |||
|
47 | nextindexh = indexh + 1; | |||
|
48 | nextindexv = 0; | |||
|
49 | } | |||
|
50 | ||||
|
51 | messageData = { | |||
|
52 | notes : notes ? notes.innerHTML : '', | |||
|
53 | indexh : indexh, | |||
|
54 | indexv : indexv, | |||
|
55 | nextindexh : nextindexh, | |||
|
56 | nextindexv : nextindexv, | |||
|
57 | markdown : notes ? typeof notes.getAttribute( 'data-markdown' ) === 'string' : false | |||
|
58 | }; | |||
|
59 | } | |||
|
60 | else if( eventType === 'fragmentshown' ) { | |||
|
61 | messageData = { | |||
|
62 | fragment : 'next' | |||
|
63 | }; | |||
|
64 | } | |||
|
65 | else if( eventType === 'fragmenthidden' ) { | |||
|
66 | messageData = { | |||
|
67 | fragment : 'prev' | |||
|
68 | }; | |||
|
69 | } | |||
|
70 | ||||
|
71 | notesPopup.postMessage( JSON.stringify( messageData ), '*' ); | |||
|
72 | } | |||
|
73 | ||||
|
74 | // Navigate to the current slide when the notes are loaded | |||
|
75 | notesPopup.addEventListener( 'load', function( event ) { | |||
|
76 | post('slidechanged'); | |||
|
77 | }, false ); | |||
|
78 | } | |||
|
79 | ||||
|
80 | // If the there's a 'notes' query set, open directly | |||
|
81 | if( window.location.search.match( /(\?|\&)notes/gi ) !== null ) { | |||
|
82 | openNotes(); | |||
|
83 | } | |||
|
84 | ||||
|
85 | // Open the notes when the 's' key is hit | |||
|
86 | document.addEventListener( 'keydown', function( event ) { | |||
|
87 | // Disregard the event if the target is editable or a | |||
|
88 | // modifier is present | |||
|
89 | if ( document.querySelector( ':focus' ) !== null || event.shiftKey || event.altKey || event.ctrlKey || event.metaKey ) return; | |||
|
90 | ||||
|
91 | if( event.keyCode === 83 ) { | |||
|
92 | event.preventDefault(); | |||
|
93 | openNotes(); | |||
|
94 | } | |||
|
95 | }, false ); | |||
|
96 | ||||
|
97 | return { open: openNotes }; | |||
|
98 | })(); |
@@ -0,0 +1,251 b'' | |||||
|
1 | // Custom reveal.js integration | |||
|
2 | (function(){ | |||
|
3 | document.querySelector( '.reveal' ).addEventListener( 'click', function( event ) { | |||
|
4 | if( event.altKey ) { | |||
|
5 | event.preventDefault(); | |||
|
6 | zoom.to({ element: event.target, pan: false }); | |||
|
7 | } | |||
|
8 | } ); | |||
|
9 | })(); | |||
|
10 | ||||
|
11 | /*! | |||
|
12 | * zoom.js 0.2 (modified version for use with reveal.js) | |||
|
13 | * http://lab.hakim.se/zoom-js | |||
|
14 | * MIT licensed | |||
|
15 | * | |||
|
16 | * Copyright (C) 2011-2012 Hakim El Hattab, http://hakim.se | |||
|
17 | */ | |||
|
18 | var zoom = (function(){ | |||
|
19 | ||||
|
20 | // The current zoom level (scale) | |||
|
21 | var level = 1; | |||
|
22 | ||||
|
23 | // The current mouse position, used for panning | |||
|
24 | var mouseX = 0, | |||
|
25 | mouseY = 0; | |||
|
26 | ||||
|
27 | // Timeout before pan is activated | |||
|
28 | var panEngageTimeout = -1, | |||
|
29 | panUpdateInterval = -1; | |||
|
30 | ||||
|
31 | var currentOptions = null; | |||
|
32 | ||||
|
33 | // Check for transform support so that we can fallback otherwise | |||
|
34 | var supportsTransforms = 'WebkitTransform' in document.body.style || | |||
|
35 | 'MozTransform' in document.body.style || | |||
|
36 | 'msTransform' in document.body.style || | |||
|
37 | 'OTransform' in document.body.style || | |||
|
38 | 'transform' in document.body.style; | |||
|
39 | ||||
|
40 | if( supportsTransforms ) { | |||
|
41 | // The easing that will be applied when we zoom in/out | |||
|
42 | document.body.style.transition = 'transform 0.8s ease'; | |||
|
43 | document.body.style.OTransition = '-o-transform 0.8s ease'; | |||
|
44 | document.body.style.msTransition = '-ms-transform 0.8s ease'; | |||
|
45 | document.body.style.MozTransition = '-moz-transform 0.8s ease'; | |||
|
46 | document.body.style.WebkitTransition = '-webkit-transform 0.8s ease'; | |||
|
47 | } | |||
|
48 | ||||
|
49 | // Zoom out if the user hits escape | |||
|
50 | document.addEventListener( 'keyup', function( event ) { | |||
|
51 | if( level !== 1 && event.keyCode === 27 ) { | |||
|
52 | zoom.out(); | |||
|
53 | } | |||
|
54 | }, false ); | |||
|
55 | ||||
|
56 | // Monitor mouse movement for panning | |||
|
57 | document.addEventListener( 'mousemove', function( event ) { | |||
|
58 | if( level !== 1 ) { | |||
|
59 | mouseX = event.clientX; | |||
|
60 | mouseY = event.clientY; | |||
|
61 | } | |||
|
62 | }, false ); | |||
|
63 | ||||
|
64 | /** | |||
|
65 | * Applies the CSS required to zoom in, prioritizes use of CSS3 | |||
|
66 | * transforms but falls back on zoom for IE. | |||
|
67 | * | |||
|
68 | * @param {Number} pageOffsetX | |||
|
69 | * @param {Number} pageOffsetY | |||
|
70 | * @param {Number} elementOffsetX | |||
|
71 | * @param {Number} elementOffsetY | |||
|
72 | * @param {Number} scale | |||
|
73 | */ | |||
|
74 | function magnify( pageOffsetX, pageOffsetY, elementOffsetX, elementOffsetY, scale ) { | |||
|
75 | ||||
|
76 | if( supportsTransforms ) { | |||
|
77 | var origin = pageOffsetX +'px '+ pageOffsetY +'px', | |||
|
78 | transform = 'translate('+ -elementOffsetX +'px,'+ -elementOffsetY +'px) scale('+ scale +')'; | |||
|
79 | ||||
|
80 | document.body.style.transformOrigin = origin; | |||
|
81 | document.body.style.OTransformOrigin = origin; | |||
|
82 | document.body.style.msTransformOrigin = origin; | |||
|
83 | document.body.style.MozTransformOrigin = origin; | |||
|
84 | document.body.style.WebkitTransformOrigin = origin; | |||
|
85 | ||||
|
86 | document.body.style.transform = transform; | |||
|
87 | document.body.style.OTransform = transform; | |||
|
88 | document.body.style.msTransform = transform; | |||
|
89 | document.body.style.MozTransform = transform; | |||
|
90 | document.body.style.WebkitTransform = transform; | |||
|
91 | } | |||
|
92 | else { | |||
|
93 | // Reset all values | |||
|
94 | if( scale === 1 ) { | |||
|
95 | document.body.style.position = ''; | |||
|
96 | document.body.style.left = ''; | |||
|
97 | document.body.style.top = ''; | |||
|
98 | document.body.style.width = ''; | |||
|
99 | document.body.style.height = ''; | |||
|
100 | document.body.style.zoom = ''; | |||
|
101 | } | |||
|
102 | // Apply scale | |||
|
103 | else { | |||
|
104 | document.body.style.position = 'relative'; | |||
|
105 | document.body.style.left = ( - ( pageOffsetX + elementOffsetX ) / scale ) + 'px'; | |||
|
106 | document.body.style.top = ( - ( pageOffsetY + elementOffsetY ) / scale ) + 'px'; | |||
|
107 | document.body.style.width = ( scale * 100 ) + '%'; | |||
|
108 | document.body.style.height = ( scale * 100 ) + '%'; | |||
|
109 | document.body.style.zoom = scale; | |||
|
110 | } | |||
|
111 | } | |||
|
112 | ||||
|
113 | level = scale; | |||
|
114 | ||||
|
115 | if( level !== 1 && document.documentElement.classList ) { | |||
|
116 | document.documentElement.classList.add( 'zoomed' ); | |||
|
117 | } | |||
|
118 | else { | |||
|
119 | document.documentElement.classList.remove( 'zoomed' ); | |||
|
120 | } | |||
|
121 | } | |||
|
122 | ||||
|
123 | /** | |||
|
124 | * Pan the document when the mosue cursor approaches the edges | |||
|
125 | * of the window. | |||
|
126 | */ | |||
|
127 | function pan() { | |||
|
128 | var range = 0.12, | |||
|
129 | rangeX = window.innerWidth * range, | |||
|
130 | rangeY = window.innerHeight * range, | |||
|
131 | scrollOffset = getScrollOffset(); | |||
|
132 | ||||
|
133 | // Up | |||
|
134 | if( mouseY < rangeY ) { | |||
|
135 | window.scroll( scrollOffset.x, scrollOffset.y - ( 1 - ( mouseY / rangeY ) ) * ( 14 / level ) ); | |||
|
136 | } | |||
|
137 | // Down | |||
|
138 | else if( mouseY > window.innerHeight - rangeY ) { | |||
|
139 | window.scroll( scrollOffset.x, scrollOffset.y + ( 1 - ( window.innerHeight - mouseY ) / rangeY ) * ( 14 / level ) ); | |||
|
140 | } | |||
|
141 | ||||
|
142 | // Left | |||
|
143 | if( mouseX < rangeX ) { | |||
|
144 | window.scroll( scrollOffset.x - ( 1 - ( mouseX / rangeX ) ) * ( 14 / level ), scrollOffset.y ); | |||
|
145 | } | |||
|
146 | // Right | |||
|
147 | else if( mouseX > window.innerWidth - rangeX ) { | |||
|
148 | window.scroll( scrollOffset.x + ( 1 - ( window.innerWidth - mouseX ) / rangeX ) * ( 14 / level ), scrollOffset.y ); | |||
|
149 | } | |||
|
150 | } | |||
|
151 | ||||
|
152 | function getScrollOffset() { | |||
|
153 | return { | |||
|
154 | x: window.scrollX !== undefined ? window.scrollX : window.pageXOffset, | |||
|
155 | y: window.scrollY !== undefined ? window.scrollY : window.pageXYffset | |||
|
156 | } | |||
|
157 | } | |||
|
158 | ||||
|
159 | return { | |||
|
160 | /** | |||
|
161 | * Zooms in on either a rectangle or HTML element. | |||
|
162 | * | |||
|
163 | * @param {Object} options | |||
|
164 | * - element: HTML element to zoom in on | |||
|
165 | * OR | |||
|
166 | * - x/y: coordinates in non-transformed space to zoom in on | |||
|
167 | * - width/height: the portion of the screen to zoom in on | |||
|
168 | * - scale: can be used instead of width/height to explicitly set scale | |||
|
169 | */ | |||
|
170 | to: function( options ) { | |||
|
171 | // Due to an implementation limitation we can't zoom in | |||
|
172 | // to another element without zooming out first | |||
|
173 | if( level !== 1 ) { | |||
|
174 | zoom.out(); | |||
|
175 | } | |||
|
176 | else { | |||
|
177 | options.x = options.x || 0; | |||
|
178 | options.y = options.y || 0; | |||
|
179 | ||||
|
180 | // If an element is set, that takes precedence | |||
|
181 | if( !!options.element ) { | |||
|
182 | // Space around the zoomed in element to leave on screen | |||
|
183 | var padding = 20; | |||
|
184 | ||||
|
185 | options.width = options.element.getBoundingClientRect().width + ( padding * 2 ); | |||
|
186 | options.height = options.element.getBoundingClientRect().height + ( padding * 2 ); | |||
|
187 | options.x = options.element.getBoundingClientRect().left - padding; | |||
|
188 | options.y = options.element.getBoundingClientRect().top - padding; | |||
|
189 | } | |||
|
190 | ||||
|
191 | // If width/height values are set, calculate scale from those values | |||
|
192 | if( options.width !== undefined && options.height !== undefined ) { | |||
|
193 | options.scale = Math.max( Math.min( window.innerWidth / options.width, window.innerHeight / options.height ), 1 ); | |||
|
194 | } | |||
|
195 | ||||
|
196 | if( options.scale > 1 ) { | |||
|
197 | options.x *= options.scale; | |||
|
198 | options.y *= options.scale; | |||
|
199 | ||||
|
200 | var scrollOffset = getScrollOffset(); | |||
|
201 | ||||
|
202 | if( options.element ) { | |||
|
203 | scrollOffset.x -= ( window.innerWidth - ( options.width * options.scale ) ) / 2; | |||
|
204 | } | |||
|
205 | ||||
|
206 | magnify( scrollOffset.x, scrollOffset.y, options.x, options.y, options.scale ); | |||
|
207 | ||||
|
208 | if( options.pan !== false ) { | |||
|
209 | ||||
|
210 | // Wait with engaging panning as it may conflict with the | |||
|
211 | // zoom transition | |||
|
212 | panEngageTimeout = setTimeout( function() { | |||
|
213 | panUpdateInterval = setInterval( pan, 1000 / 60 ); | |||
|
214 | }, 800 ); | |||
|
215 | ||||
|
216 | } | |||
|
217 | } | |||
|
218 | ||||
|
219 | currentOptions = options; | |||
|
220 | } | |||
|
221 | }, | |||
|
222 | ||||
|
223 | /** | |||
|
224 | * Resets the document zoom state to its default. | |||
|
225 | */ | |||
|
226 | out: function() { | |||
|
227 | clearTimeout( panEngageTimeout ); | |||
|
228 | clearInterval( panUpdateInterval ); | |||
|
229 | ||||
|
230 | var scrollOffset = getScrollOffset(); | |||
|
231 | ||||
|
232 | if( currentOptions && currentOptions.element ) { | |||
|
233 | scrollOffset.x -= ( window.innerWidth - ( currentOptions.width * currentOptions.scale ) ) / 2; | |||
|
234 | } | |||
|
235 | ||||
|
236 | magnify( scrollOffset.x, scrollOffset.y, 0, 0, 1 ); | |||
|
237 | ||||
|
238 | level = 1; | |||
|
239 | }, | |||
|
240 | ||||
|
241 | // Alias | |||
|
242 | magnify: function( options ) { this.to( options ) }, | |||
|
243 | reset: function() { this.out() }, | |||
|
244 | ||||
|
245 | zoomLevel: function() { | |||
|
246 | return level; | |||
|
247 | } | |||
|
248 | } | |||
|
249 | ||||
|
250 | })(); | |||
|
251 |
@@ -6,7 +6,7 b' init_mathjax = function() {' | |||||
6 | inlineMath: [ ['$','$'], ["\\(","\\)"] ], |
|
6 | inlineMath: [ ['$','$'], ["\\(","\\)"] ], | |
7 | displayMath: [ ['$$','$$'], ["\\[","\\]"] ] |
|
7 | displayMath: [ ['$$','$$'], ["\\[","\\]"] ] | |
8 | }, |
|
8 | }, | |
9 |
displayAlign: ' |
|
9 | displayAlign: 'center', // Change this to 'center' to center equations. | |
10 | "HTML-CSS": { |
|
10 | "HTML-CSS": { | |
11 | styles: {'.MathJax_Display': {"margin": 0}} |
|
11 | styles: {'.MathJax_Display': {"margin": 0}} | |
12 | } |
|
12 | } |
@@ -22,6 +22,7 b' from converters.bloggerhtml import ConverterBloggerHTML' | |||||
22 | from converters.rst import ConverterRST |
|
22 | from converters.rst import ConverterRST | |
23 | from converters.latex import ConverterLaTeX |
|
23 | from converters.latex import ConverterLaTeX | |
24 | from converters.python import ConverterPy |
|
24 | from converters.python import ConverterPy | |
|
25 | from converters.slider import ConverterSlider | |||
25 |
|
26 | |||
26 |
|
27 | |||
27 | # When adding a new format, make sure to add it to the `converters` |
|
28 | # When adding a new format, make sure to add it to the `converters` | |
@@ -36,6 +37,7 b' converters = {' | |||||
36 | 'blogger-html': ConverterBloggerHTML, |
|
37 | 'blogger-html': ConverterBloggerHTML, | |
37 | 'latex': ConverterLaTeX, |
|
38 | 'latex': ConverterLaTeX, | |
38 | 'py': ConverterPy, |
|
39 | 'py': ConverterPy, | |
|
40 | 'slider': ConverterSlider, | |||
39 | } |
|
41 | } | |
40 |
|
42 | |||
41 | default_format = 'rst' |
|
43 | default_format = 'rst' |
General Comments 0
You need to be logged in to leave comments.
Login now